组合模式:将对象组合成树形结构以表示“部分-整体”的层次结构。
组合模式使得用户对单个对象和组合对象的使用具有一致性。
是一种结构型模式
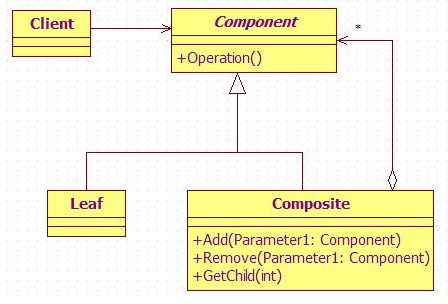
使用场景:
1、用于对象的部分-整体层次结构,如树形菜单、文件夹菜单、部门组织架构图等;
2、对用户隐藏组合对象与单个对象的不同,使得用户统一地使用组合结构中的所有对象。
1 #include <iostream> 2 #include <string> 3 #include <vector> 4 5 using namespace std; 6 7 class STComponent 8 { 9 public: 10 STComponent() 11 { 12 13 } 14 STComponent(string strName): m_strName(strName) 15 { 16 17 } 18 virtual ~STComponent() 19 { 20 21 } 22 23 /* 24 virtual void Add(STComponent* c); 25 virtual void Remove(STComponent* c) ; 26 virtual void Display(int iDepth); 27 */ 28 29 30 virtual void Add(STComponent* c) = 0; 31 virtual void Remove(STComponent* c) = 0; 32 virtual void Display(int iDepth) = 0; 33 34 string m_strName; 35 36 }; 37 38 class STLeaf: public STComponent 39 { 40 public: 41 STLeaf(string strName): STComponent(strName) 42 { 43 44 } 45 46 virtual void Add(STComponent* c) 47 { 48 cout<< "Cann't Add to a leaf"<< endl; 49 } 50 virtual void Remove(STComponent* c) 51 { 52 cout<< "Cann't Remove from a leaf"<< endl; 53 } 54 virtual void Display(int iDepth) 55 { 56 cout<< string(iDepth, '-')<< m_strName<< endl; 57 } 58 }; 59 60 class STComposite: public STComponent 61 { 62 public: 63 STComposite(string strName): STComponent(strName) 64 { 65 66 } 67 ~STComposite() 68 { 69 m_vecStComposite.clear(); 70 } 71 72 virtual void Add(STComponent* c) 73 { 74 m_vecStComposite.push_back(c); 75 } 76 77 virtual void Remove(STComponent* c) 78 { 79 for (typeof(m_vecStComposite.begin()) it = m_vecStComposite.begin(); it != m_vecStComposite.end();) 80 { 81 if (*it == c) 82 { 83 it = m_vecStComposite.erase(it); 84 cout<< "erase Succ: "<< (*it)->m_strName<< endl; 85 } 86 else 87 { 88 ++it; 89 } 90 } 91 } 92 93 virtual void Display(int iDepth) 94 { 95 cout<< string(iDepth, '-')<< m_strName<< endl; 96 for (size_t i = 0; i < m_vecStComposite.size(); ++i) 97 { 98 m_vecStComposite[i]->Display(iDepth+1); 99 } 100 } 101 102 vector<STComponent*> m_vecStComposite; 103 }; 104 105 int main(int argc, char* argv[]) 106 { 107 //STLeaf* pstLeaf = new STLeaf("leafA"); 108 //pstLeaf->Add(NULL); 109 //pstLeaf->Remove(NULL); 110 //pstLeaf->Display(10); 111 //delete pstLeaf; 112 113 STComposite* root = new STComposite("root"); 114 root->Add(new STLeaf("Leaf A")); 115 root->Add(new STLeaf("Leaf B")); 116 117 STComposite* comp = new STComposite("Composite X"); 118 comp->Add(new STLeaf("Leaf XA")); 119 comp->Add(new STLeaf("Leaf XB")); 120 root->Add(comp); 121 122 STComposite* comp2 = new STComposite("Composite XY"); 123 comp2->Add(new STLeaf("Leaf XYA")); 124 comp2->Add(new STLeaf("Leaf XYB")); 125 comp->Add(comp2); 126 127 STLeaf* pstLeaf = new STLeaf("leaf D"); 128 root->Add(pstLeaf); 129 root->Display(1); 130 131 // root->Remove(pstLeaf); 132 root->Remove(comp); 133 root->Display(1); 134 135 return 0; 136 } 137 ///////////////////////////// 138 [root@ ~/learn_code/design_pattern/16_composite]$ ./composite 139 -root 140 --Leaf A 141 --Leaf B 142 --Composite X 143 ---Leaf XA 144 ---Leaf XB 145 ---Composite XY 146 ----Leaf XYA 147 ----Leaf XYB 148 --leaf D 149 erase Succ: leaf D 150 -root 151 --Leaf A 152 --Leaf B 153 --leaf D