要求:
- 1 补充课上没有完成的作业
- 2 参考15.3节,用自己完成的队列(链队,循环数组队列)实现模拟票务柜台排队功能
- 3 用JDB或IDEA单步跟踪排队情况,画出队列变化图,包含自己的学号信息
- 4 把代码推送到代码托管平台
- 5 把完成过程写一篇博客:重点是单步跟踪过程和遇到的问题及解决过程
- 6 提交博客链接
补充代码:
public class CircularArrayQueue<T> implements Queue<T>
{
private final int DEFAULT_CAPACITY = 10000;
private int front, rear, size;
private T[] queue;
//-----------------------------------------------------------------
// Creates an empty queue using the default capacity.
//-----------------------------------------------------------------
public CircularArrayQueue()
{
front = rear = size = 0;
queue = (T[]) (new Object[DEFAULT_CAPACITY]);
}
//-----------------------------------------------------------------
// Adds the specified element to the rear of this queue, expanding
// the capacity of the queue array if necessary.
//-----------------------------------------------------------------
public void enqueue (T element)
{
if (size == queue.length)
expandCapacity();
queue[rear] = element;
rear = (rear+1) % queue.length;
size++;
}
public void expandCapacity()
{
T[] larger = (T[])(new Object[queue.length*2]);
for (int index = 0; index < size; index++)
larger[index] = queue[(front+index) % queue.length];
front = 0;
rear = size;
queue = larger;
}
@Override
public T dequeue()
{
if (size == 0)
throw new EmptyCollectionException("queue");
T d = queue[front];
queue[front] = null;
front = (front+1)%queue.length;
size--;
return d;
}
@Override
public T first() {
return queue[front];
}
@Override
public boolean isEmpty() {
boolean course = false;
if(size ==0){
course = true;
}
return course;
}
@Override
public int size() {
return size;
}
@Override
public String toString(){
String result = "";
int scan = 0;
while(scan < size)
{
if(queue[scan]!=null)
{
result += queue[scan].toString()+"
";
}
scan++;
}
return result;
}
}
单步跟踪:
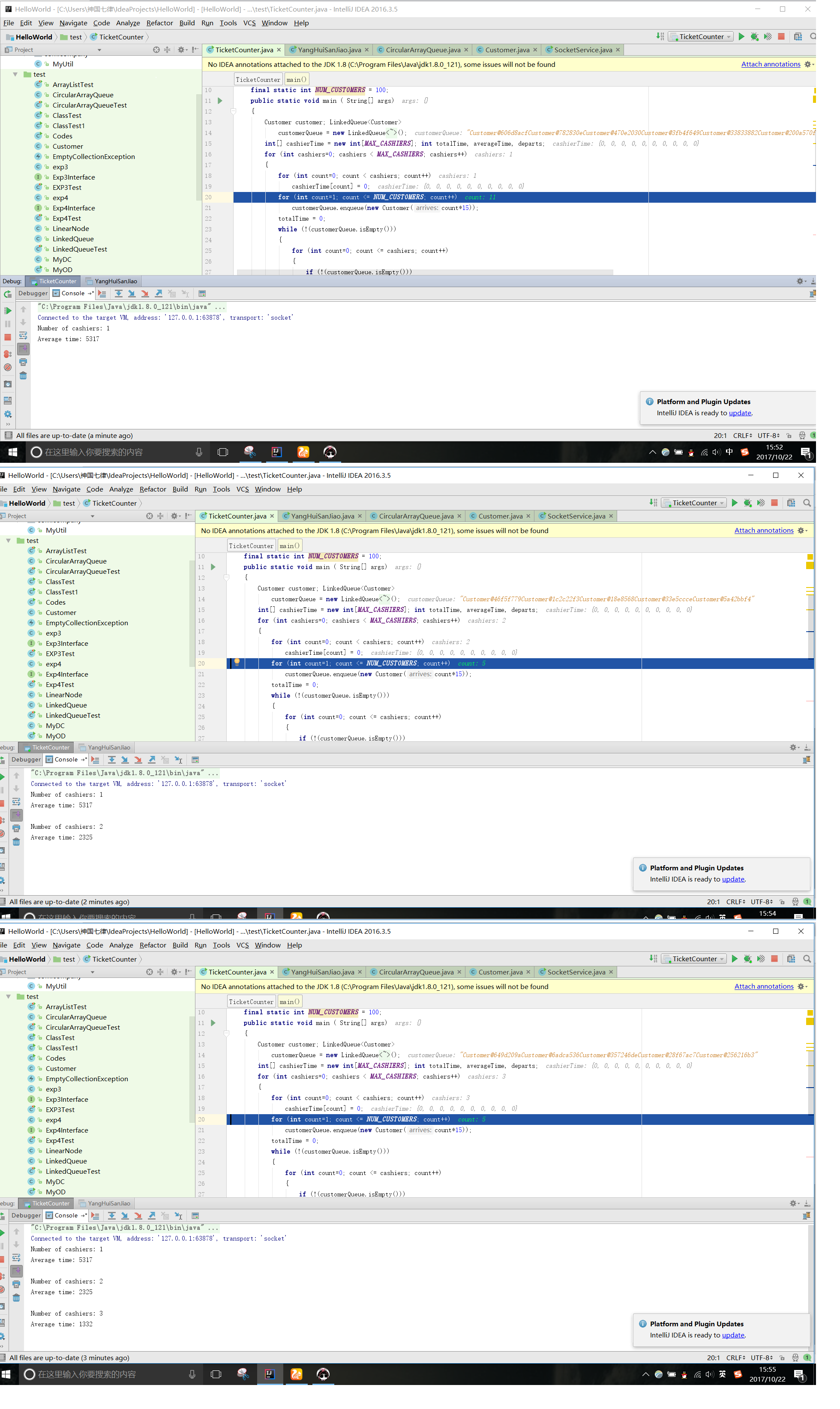
队列变化图:
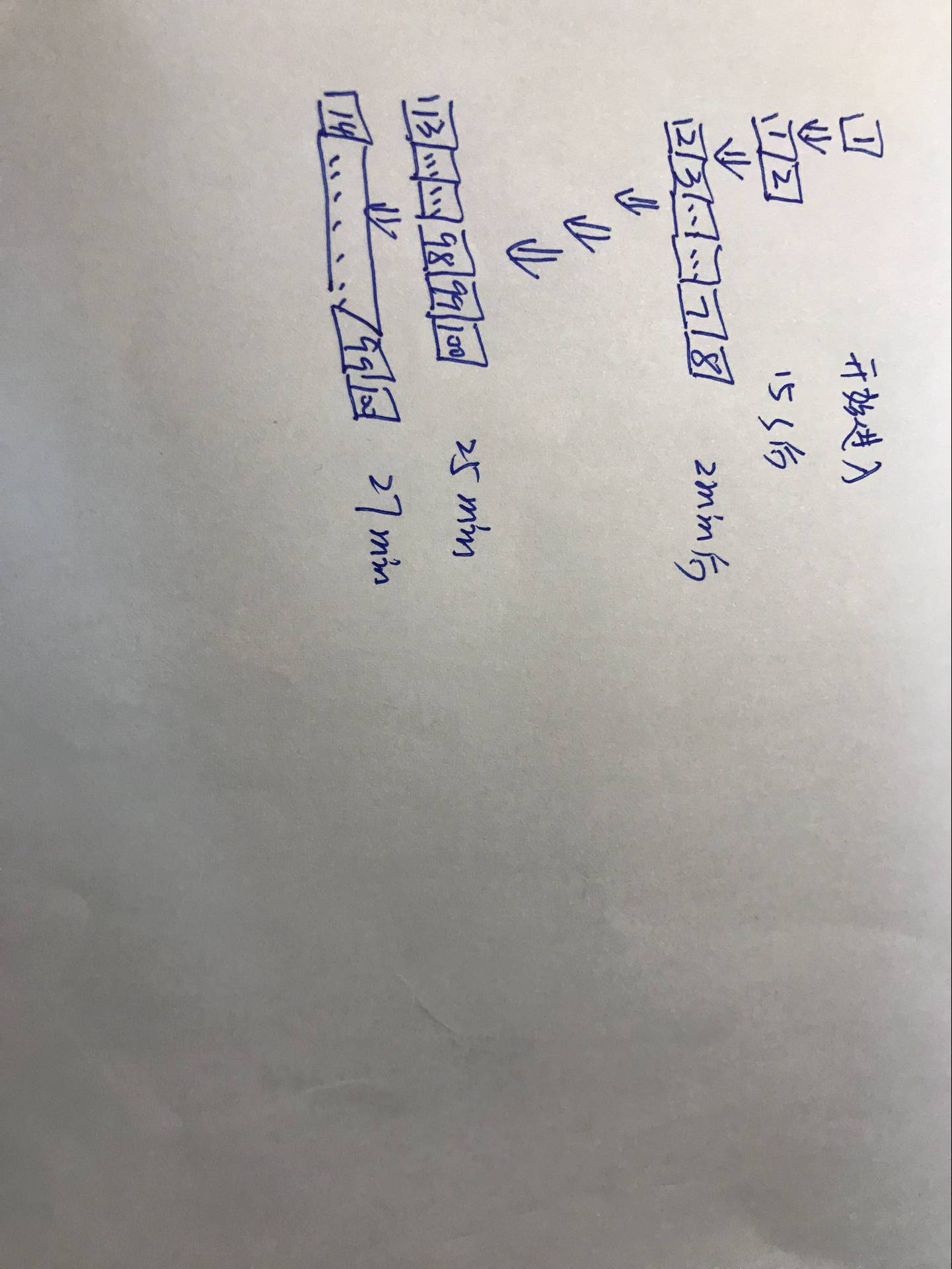