Description
Imagine you are standing inside a two-dimensional maze composed of square cells which may or may not be filled with rock. You can move north, south, east or west one cell at a step. These moves are called walks.
One of the empty cells contains a box which can be moved to an adjacent free cell by standing next to the box and then moving in the direction of the box. Such a move is called a push. The box cannot be moved in any other way than by pushing, which means that if you push it into a corner you can never get it out of the corner again.
One of the empty cells is marked as the target cell. Your job is to bring the box to the target cell by a sequence of walks and pushes. As the box is very heavy, you would like to minimize the number of pushes. Can you write a program that will work out the best such sequence?
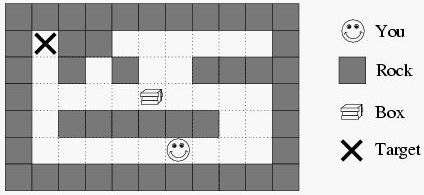
One of the empty cells contains a box which can be moved to an adjacent free cell by standing next to the box and then moving in the direction of the box. Such a move is called a push. The box cannot be moved in any other way than by pushing, which means that if you push it into a corner you can never get it out of the corner again.
One of the empty cells is marked as the target cell. Your job is to bring the box to the target cell by a sequence of walks and pushes. As the box is very heavy, you would like to minimize the number of pushes. Can you write a program that will work out the best such sequence?
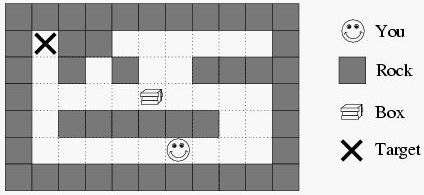
Input
The
input contains the descriptions of several mazes. Each maze description
starts with a line containing two integers r and c (both <= 20)
representing the number of rows and columns of the maze.
Following this are r lines each containing c characters. Each character describes one cell of the maze. A cell full of rock is indicated by a `#' and an empty cell is represented by a `.'. Your starting position is symbolized by `S', the starting position of the box by `B' and the target cell by `T'.
Input is terminated by two zeroes for r and c.
Following this are r lines each containing c characters. Each character describes one cell of the maze. A cell full of rock is indicated by a `#' and an empty cell is represented by a `.'. Your starting position is symbolized by `S', the starting position of the box by `B' and the target cell by `T'.
Input is terminated by two zeroes for r and c.
Output
For
each maze in the input, first print the number of the maze, as shown in
the sample output. Then, if it is impossible to bring the box to the
target cell, print ``Impossible.''.
Otherwise, output a sequence that minimizes the number of pushes. If there is more than one such sequence, choose the one that minimizes the number of total moves (walks and pushes). If there is still more than one such sequence, any one is acceptable.
Print the sequence as a string of the characters N, S, E, W, n, s, e and w where uppercase letters stand for pushes, lowercase letters stand for walks and the different letters stand for the directions north, south, east and west.
Output a single blank line after each test case.
Otherwise, output a sequence that minimizes the number of pushes. If there is more than one such sequence, choose the one that minimizes the number of total moves (walks and pushes). If there is still more than one such sequence, any one is acceptable.
Print the sequence as a string of the characters N, S, E, W, n, s, e and w where uppercase letters stand for pushes, lowercase letters stand for walks and the different letters stand for the directions north, south, east and west.
Output a single blank line after each test case.
Sample Input
1 7 SB....T 1 7 SB..#.T 7 11 ########### #T##......# #.#.#..#### #....B....# #.######..# #.....S...# ########### 8 4 .... .##. .#.. .#.. .#.B .##S .... ###T 0 0
Sample Output
Maze #1 EEEEE Maze #2 Impossible. Maze #3 eennwwWWWWeeeeeesswwwwwwwnNN Maze #4 swwwnnnnnneeesssSSS
题目大意:推箱子。不过要求的是推的步数最少的路径,如果相同,则找到总步数最少的路径。
题目分析:因为涉及到路径的优先权问题,毫无疑问就要用到优先队列。接下来就是解这道题的核心步骤了,定义状态和状态转移。显然(其实,这个“显然”是花了老长时间想明白的),状态参量就是“你”和箱子的坐标、走过的路径和已经“推”过的步数和总步数。对于这道题,状态转移反而要比定义状态容易做到。
因为箱子的位置也是变化的,所以状态参量中要有箱子坐标。一开始没注意到这一点或者说就没想明白,导致瞎耽误了很多工夫!!!
代码如下:
1 # include<iostream> 2 # include<cstdio> 3 # include<queue> 4 # include<cstring> 5 # include<algorithm> 6 using namespace std; 7 struct node 8 { 9 int mx,my,bx,by,pt,t,h; 10 string s; 11 node(int _mx,int _my,int _bx,int _by,int _pt,int _t,string _s):mx(_mx),my(_my),bx(_bx),by(_by),pt(_pt),t(_t),s(_s){} 12 bool operator < (const node &a) const { 13 if(pt==a.pt) 14 return t>a.t; 15 return pt>a.pt; 16 } 17 }; 18 int r,c,vis[25][25][25][25]; 19 char mp[25][25]; 20 string f[2]={"nesw","NESW"}; 21 int d[4][2]={{-1,0},{0,1},{1,0},{0,-1}}; 22 bool ok(int x,int y) 23 { 24 if(x>=0&&x<r&&y>=0&&y<c) 25 return true; 26 return false; 27 } 28 void bfs(int mx,int my,int bx,int by) 29 { 30 priority_queue<node>q; 31 memset(vis,0,sizeof(vis)); 32 vis[mx][my][bx][by]=1; 33 q.push(node(mx,my,bx,by,0,0,"")); 34 while(!q.empty()) 35 { 36 node u=q.top(); 37 q.pop(); 38 if(mp[u.bx][u.by]=='T'){ 39 cout<<u.s<<endl; 40 return ; 41 } 42 for(int i=0;i<4;++i){ 43 int nx=u.mx+d[i][0],ny=u.my+d[i][1]; 44 if(ok(nx,ny)&&mp[nx][ny]!='#'){ 45 if(nx==u.bx&&ny==u.by){ 46 int nbx=u.bx+d[i][0],nby=u.by+d[i][1]; 47 if(ok(nbx,nby)&&mp[nbx][nby]!='#'&&!vis[nx][ny][nbx][nby]){ 48 vis[nx][ny][nbx][nby]=1; 49 string s=u.s; 50 s+=f[1][i]; 51 q.push(node(nx,ny,nbx,nby,u.pt+1,u.t+1,s)); 52 } 53 }else{ 54 if(!vis[nx][ny][u.bx][u.by]){ 55 vis[nx][ny][u.bx][u.by]=1; 56 string s=u.s; 57 s+=f[0][i]; 58 q.push(node(nx,ny,u.bx,u.by,u.pt,u.t+1,s)); 59 } 60 } 61 } 62 } 63 } 64 printf("Impossible. "); 65 } 66 int main() 67 { 68 int cas=0,mx,my,bx,by; 69 while(scanf("%d%d",&r,&c),r+c) 70 { 71 for(int i=0;i<r;++i){ 72 scanf("%s",mp[i]); 73 for(int j=0;j<c;++j){ 74 if(mp[i][j]=='S') 75 mx=i,my=j; 76 if(mp[i][j]=='B') 77 bx=i,by=j; 78 } 79 } 80 printf("Maze #%d ",++cas); 81 bfs(mx,my,bx,by); 82 printf(" "); 83 } 84 return 0; 85 }
做后感:一定要在想明白思路后再写代码,否则,多写无益处!!!