02-线性结构4 Pop Sequence (25分)
Given a stack which can keep M numbers at most. Push N numbers in the order of 1, 2, 3, ..., N and pop randomly. You are supposed to tell if a given sequence of numbers is a possible pop sequence of the stack. For example, if M is 5 and N is 7, we can obtain 1, 2, 3, 4, 5, 6, 7 from the stack, but not 3, 2, 1, 7, 5, 6, 4.
Input Specification:
Each input file contains one test case. For each case, the first line contains 3 numbers (all no more than 1000): M (the maximum capacity of the stack), N (the length of push sequence), and K (the number of pop sequences to be checked). Then K lines follow, each contains a pop sequence of N numbers. All the numbers in a line are separated by a space.
Output Specification:
For each pop sequence, print in one line "YES" if it is indeed a possible pop sequence of the stack, or "NO" if not.
Sample Input:
5 7 5 1 2 3 4 5 6 7 3 2 1 7 5 6 4 7 6 5 4 3 2 1 5 6 4 3 7 2 1 1 7 6 5 4 3 2
Sample Output:
YES
NO
NO
YES
NO
提测代码:
#include <stdio.h> #include <stdlib.h> #define ERROR -1 typedef int Position; typedef int ElemType; struct SNode{ ElemType* Data; Position Top; int MaxSize; }; typedef struct SNode *Stack; Stack CreateStack(int MaxSize){ Stack S = (Stack)malloc(sizeof(struct SNode)); S->Data = (ElemType*)malloc(MaxSize * sizeof(ElemType)); S->Top = -1; S->MaxSize = MaxSize; return S; } void DestroyStack(Stack S){ if(S == NULL){ return; } if(S->Data != NULL){ free(S->Data); } free(S); } int IsEmpty(Stack S){ return (S->Top == -1); } int IsFull(Stack S){ return (S->Top + 1 == S->MaxSize); } int Push(Stack S, ElemType data){ if(IsFull(S)){ return ERROR; } S->Data[++S->Top] = data; return 1; } ElemType Pop(Stack S){ if(IsEmpty(S)){ return ERROR; } return S->Data[S->Top--]; } void Empty(Stack S){ S->Top = -1; } int main(){ int M, N, line; scanf("%d %d %d", &M, &N, &line); ElemType curData; int countor; int flag; Stack S = CreateStack(M); for(int i = 0; i < line; ++i){ countor = 1; flag = 1;
Empty(S); for(int j = 0; j < N; ++j){ scanf("%d", &curData); if(!flag) continue; //如果计数器小于当前值 if(countor < curData){ while(countor <= curData){ if(Push(S, countor++) == ERROR){ flag = 0; break; } } Pop(S); } else if(countor > curData){ if(Pop(S) != curData){ flag = 0; } } else{ countor++; } } if(flag == 1 && IsEmpty(S)){ printf("YES "); } else{ printf("NO "); } } return 0; }
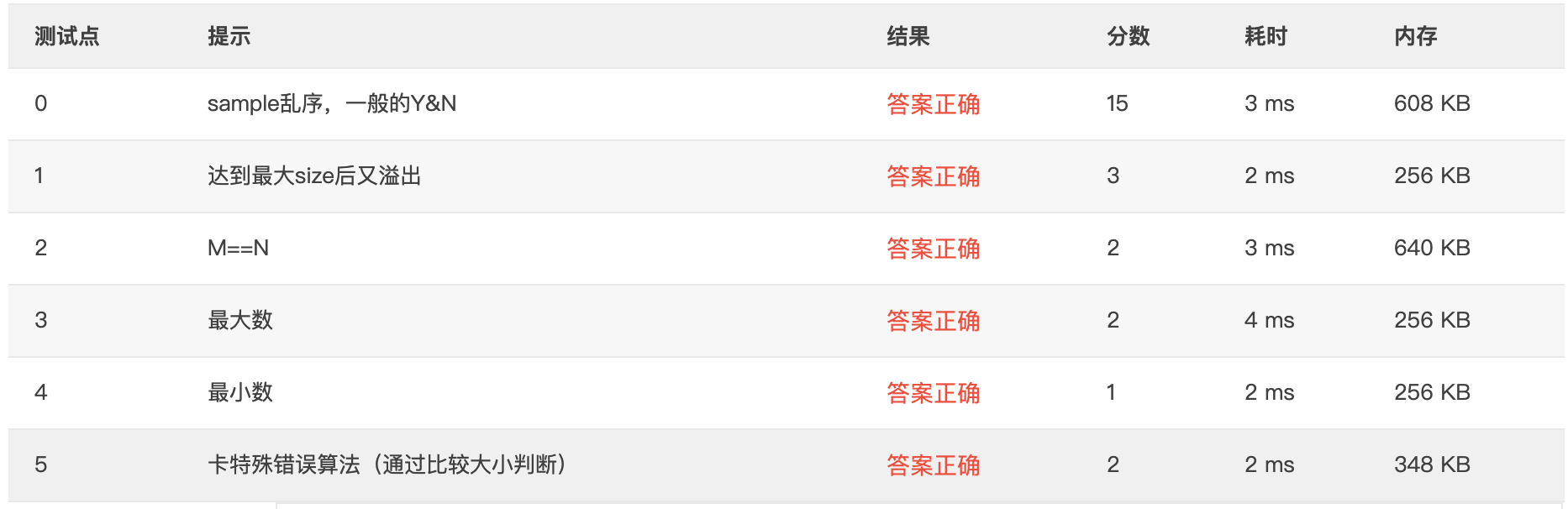