////////////////////////////////////效果图///////////////////////////
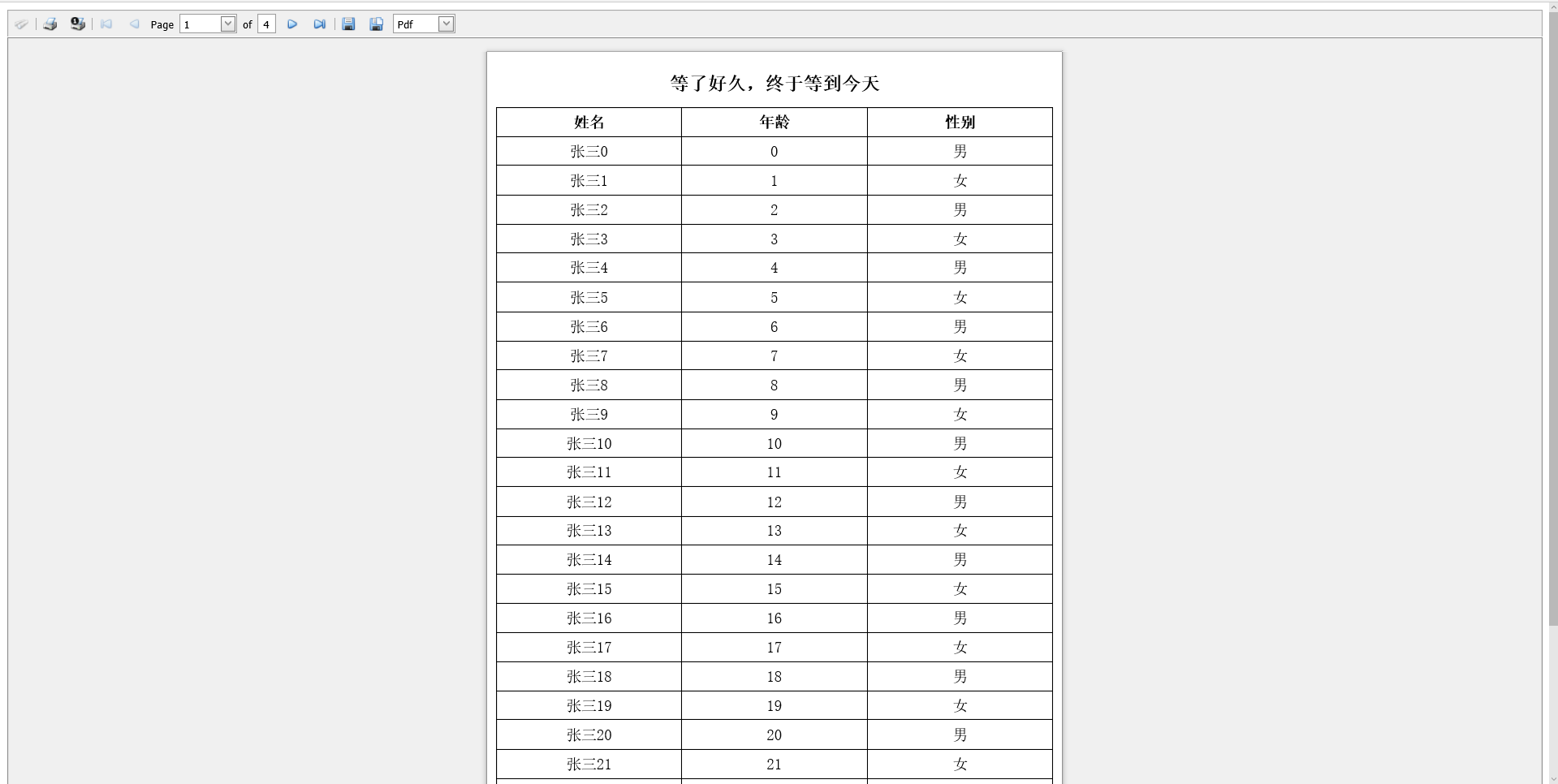
////////////////////////////////////代码////////////////////////////
using System; using System.Drawing; using System.Collections; using System.ComponentModel; using DevExpress.XtraReports.UI; using System.Data; using MvcApplication1.Models; using System.Collections.Generic; /// <summary> /// Summary description for XtraReport3 /// </summary> public class XtraReport2 : DevExpress.XtraReports.UI.XtraReport { private DevExpress.XtraReports.UI.DetailBand Detail; private DevExpress.XtraReports.UI.TopMarginBand TopMargin; private DevExpress.XtraReports.UI.BottomMarginBand BottomMargin; protected int XRTableCellHeight = 5; private ReportHeaderBand ReportHeader; private XRLabel xrLabel2; /// <summary> /// Required designer variable. /// </summary> private System.ComponentModel.IContainer components = null; public XtraReport2() { InitializeComponent(); XtraReport rpt = this;// 建立报表实例 InitBands(rpt);//添加带区(Bands) InitDetailsBasedonXRTable(rpt, GetDataSource());//用XRTable显示报表 } public IList<StudentModel> GetDataSource() { List<StudentModel> model = new List<StudentModel>(); for (int i = 0; i < 100; i++) { model.Add(new StudentModel { StudentName = "张三" + i, Age = i, Sex = i % 2 == 0 ? "男" : "女" }); } return model; } public void InitBands(XtraReport rpt) { DetailBand detail = new DetailBand(); PageHeaderBand pageHeader = new PageHeaderBand(); ReportFooterBand reportFooter = new ReportFooterBand(); detail.Height = XRTableCellHeight; reportFooter.Height = 380; pageHeader.Height = XRTableCellHeight; rpt.Bands.AddRange(new DevExpress.XtraReports.UI.Band[] { detail, pageHeader, reportFooter }); } public XRTableCell GetHeaderCell(string text, int colWidth) { XRTableCell headerCell = new XRTableCell(); headerCell.Width = colWidth; headerCell.Borders = DevExpress.XtraPrinting.BorderSide.All; headerCell.Text = text; return headerCell; } public XRTableCell GetDetailCell(string text, int colWidth) { XRTableCell detailCell = new XRTableCell(); detailCell.Width = colWidth; detailCell.Text = text; detailCell.Borders = DevExpress.XtraPrinting.BorderSide.Left | DevExpress.XtraPrinting.BorderSide.Right | DevExpress.XtraPrinting.BorderSide.Bottom; return detailCell; } public void InitDetailsBasedonXRTable(XtraReport rpt, IList<StudentModel> model) { int colCount = model.Count; int colWidth = (rpt.PageWidth - (rpt.Margins.Left + rpt.Margins.Right)) / colCount; // Create a table to represent headers XRTable tableHeader = new XRTable(); tableHeader.Width = (rpt.PageWidth - (rpt.Margins.Left + rpt.Margins.Right)); tableHeader.TextAlignment = DevExpress.XtraPrinting.TextAlignment.MiddleCenter; tableHeader.Font = new System.Drawing.Font("宋体", 12.75F, System.Drawing.FontStyle.Bold); // Create a table to display data XRTable tableDetail = new XRTable(); tableDetail.TextAlignment = DevExpress.XtraPrinting.TextAlignment.MiddleCenter; tableDetail.Width = (rpt.PageWidth - (rpt.Margins.Left + rpt.Margins.Right)); tableDetail.Font = new System.Drawing.Font("宋体", 12.75F, System.Drawing.FontStyle.Regular); XRTableRow headerRow = new XRTableRow(); headerRow.Cells.Add(GetHeaderCell("姓名", colWidth)); headerRow.Cells.Add(GetHeaderCell("年龄", colWidth)); headerRow.Cells.Add(GetHeaderCell("性别", colWidth)); headerRow.Width = tableHeader.Width; tableHeader.Rows.Add(headerRow); foreach (StudentModel item in model) { XRTableRow detailRow = new XRTableRow(); detailRow.Cells.Add(GetDetailCell(item.StudentName, colWidth)); detailRow.Cells.Add(GetDetailCell(Convert.ToString(item.Age), colWidth)); detailRow.Cells.Add(GetDetailCell(item.Sex, colWidth)); detailRow.Width = tableDetail.Width; tableDetail.Rows.Add(detailRow); } rpt.Bands[BandKind.PageHeader].Controls.Add(tableHeader); rpt.Bands[BandKind.Detail].Controls.Add(tableDetail); tableDetail.Padding = new DevExpress.XtraPrinting.PaddingInfo(0, 0, XRTableCellHeight, XRTableCellHeight); tableHeader.Padding = new DevExpress.XtraPrinting.PaddingInfo(0, 0, XRTableCellHeight, XRTableCellHeight); } /// <summary> /// Clean up any resources being used. /// </summary> /// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param> protected override void Dispose(bool disposing) { if (disposing && (components != null)) { components.Dispose(); } base.Dispose(disposing); } #region Designer generated code /// <summary> /// Required method for Designer support - do not modify /// the contents of this method with the code editor. /// </summary> private void InitializeComponent() { this.Detail = new DevExpress.XtraReports.UI.DetailBand(); this.TopMargin = new DevExpress.XtraReports.UI.TopMarginBand(); this.BottomMargin = new DevExpress.XtraReports.UI.BottomMarginBand(); this.ReportHeader = new DevExpress.XtraReports.UI.ReportHeaderBand(); this.xrLabel2 = new DevExpress.XtraReports.UI.XRLabel(); ((System.ComponentModel.ISupportInitialize)(this)).BeginInit(); // // Detail // this.Detail.HeightF = 71.875F; this.Detail.MultiColumn.Layout = DevExpress.XtraPrinting.ColumnLayout.AcrossThenDown; this.Detail.MultiColumn.Mode = DevExpress.XtraReports.UI.MultiColumnMode.UseColumnCount; this.Detail.Name = "Detail"; this.Detail.StylePriority.UsePadding = false; this.Detail.StylePriority.UseTextAlignment = false; this.Detail.TextAlignment = DevExpress.XtraPrinting.TextAlignment.MiddleCenter; // // TopMargin // this.TopMargin.HeightF = 36.45833F; this.TopMargin.Name = "TopMargin"; this.TopMargin.Padding = new DevExpress.XtraPrinting.PaddingInfo(0, 0, 0, 0, 100F); this.TopMargin.TextAlignment = DevExpress.XtraPrinting.TextAlignment.TopLeft; // // BottomMargin // this.BottomMargin.HeightF = 36.45833F; this.BottomMargin.Name = "BottomMargin"; this.BottomMargin.Padding = new DevExpress.XtraPrinting.PaddingInfo(0, 0, 0, 0, 100F); this.BottomMargin.TextAlignment = DevExpress.XtraPrinting.TextAlignment.TopLeft; // // ReportHeader // this.ReportHeader.Controls.AddRange(new DevExpress.XtraReports.UI.XRControl[] { this.xrLabel2}); this.ReportHeader.HeightF = 54.25002F; this.ReportHeader.Name = "ReportHeader"; // // xrLabel2 // this.xrLabel2.Font = new System.Drawing.Font("宋体", 15.75F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((byte)(0))); this.xrLabel2.LocationFloat = new DevExpress.Utils.PointFloat(0F, 0F); this.xrLabel2.Name = "xrLabel2"; this.xrLabel2.Padding = new DevExpress.XtraPrinting.PaddingInfo(2, 2, 0, 0, 100F); this.xrLabel2.SizeF = new System.Drawing.SizeF(650F, 54.25002F); this.xrLabel2.StylePriority.UseFont = false; this.xrLabel2.StylePriority.UseTextAlignment = false; this.xrLabel2.Text = "等了好久,终于等到今天"; this.xrLabel2.TextAlignment = DevExpress.XtraPrinting.TextAlignment.MiddleCenter; // // XtraReport2 // this.Bands.AddRange(new DevExpress.XtraReports.UI.Band[] { this.Detail, this.TopMargin, this.BottomMargin, this.ReportHeader}); this.Margins = new System.Drawing.Printing.Margins(100, 100, 36, 36); this.Version = "15.2"; ((System.ComponentModel.ISupportInitialize)(this)).EndInit(); } #endregion }