开发指南针的思蹄很简单:程序先准备一张指南针图片,该图片上方向指引指同北方。接下来开发一个检测方向的传感器,程序检测到手机顶部绕z轴转过多少度,让指南针图片反向转过多少度即可。
main.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="#fff" android:orientation="vertical" > <TextView android:id="@+id/txtInfo" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <ImageView android:id="@+id/znzImage" android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@drawable/znz" /> </LinearLayout>
Compass.java
package org.crazyit.sensor; import android.app.Activity; import android.hardware.Sensor; import android.hardware.SensorEvent; import android.hardware.SensorEventListener; import android.hardware.SensorManager; import android.os.Bundle; import android.view.animation.Animation; import android.view.animation.RotateAnimation; import android.widget.ImageView; import android.widget.TextView; /** * Description: <br/> * site: <a href="http://www.crazyit.org">crazyit.org</a> <br/> * Copyright (C), 2001-2014, Yeeku.H.Lee <br/> * This program is protected by copyright laws. <br/> * Program Name: <br/> * Date: * * @author Yeeku.H.Lee kongyeeku@163.com * @version 1.0 */ public class Compass extends Activity implements SensorEventListener { // 定义显示指南针的图片 ImageView znzImage; TextView tv; // 记录指南针图片转过的角度 float currentDegree = 0f; // 定义Sensor管理器 SensorManager mSensorManager; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); // 获取界面中显示指南针的图片 tv = (TextView) findViewById(R.id.txtInfo); znzImage = (ImageView) findViewById(R.id.znzImage); // 获取传感器管理服务 mSensorManager = (SensorManager) getSystemService(SENSOR_SERVICE); } @Override protected void onResume() { super.onResume(); // 为系统的方向传感器注册监听器 mSensorManager.registerListener(this, mSensorManager.getDefaultSensor(Sensor.TYPE_ORIENTATION), SensorManager.SENSOR_DELAY_GAME); } @Override protected void onPause() { // 取消注册 mSensorManager.unregisterListener(this); super.onPause(); } @Override protected void onStop() { // 取消注册 mSensorManager.unregisterListener(this); super.onStop(); } @Override public void onSensorChanged(SensorEvent event) { // 获取触发event的传感器类型 int sensorType = event.sensor.getType(); switch (sensorType) { case Sensor.TYPE_ORIENTATION: // 获取绕Z轴转过的角度。 float degree = event.values[0]; // 创建旋转动画(反向转过degree度) RotateAnimation ra = new RotateAnimation(currentDegree, -degree, Animation.RELATIVE_TO_SELF, 0.5f, Animation.RELATIVE_TO_SELF, 0.5f); // 设置动画的持续时间 ra.setDuration(200); // 运行动画 znzImage.startAnimation(ra); currentDegree = -degree; tv.setText("角度:" + currentDegree); break; } } @Override public void onAccuracyChanged(Sensor sensor, int accuracy) { } }
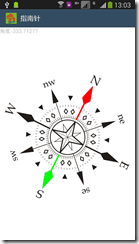