[洛谷] P1126 机器人搬重物(BFS搜图好题)
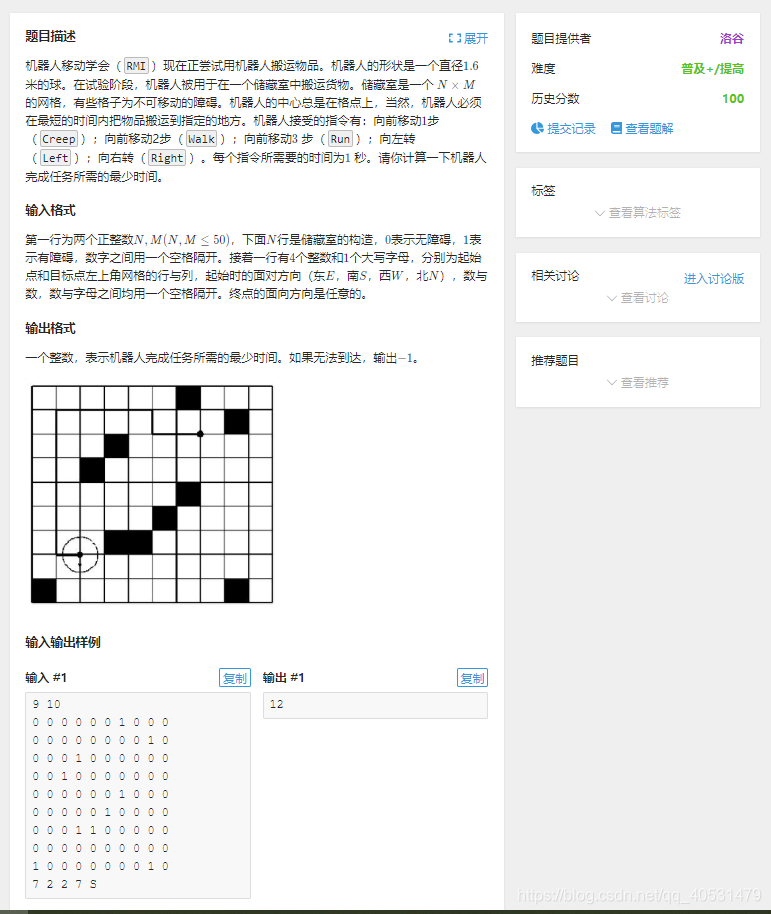
#include <bits/stdc++.h>
using namespace std;
int maze[100][100],sx,sy,ex,ey,m,n,sdir;
bool vis[100][100][4];
char ddd[3];
struct Node{
int x,y,step,dir;
Node(int _x=0,int _y=0,int _step=0,int _dir=0):x(_x),y(_y),step(_step),dir(_dir){}
}node;
bool ok(int x,int y,int dir);
bool out(int x,int y);
pair<int,int> move(int x,int y,int dir,int len);
bool checkCreep(int xx,int yy,int dir,int &nx,int &ny);
bool checkWalk(int xx,int yy,int dir,int& nx,int& ny);
bool checkRun(int xx,int yy,int dir,int& nx,int &ny);
inline int toRight(int dir){return (dir+1)%4;}
inline int toLeft(int dir){return (dir+3)%4;}
void bfs();
int main()
{
int ca = 0;
while(cin>>n>>m)
{
if(ca++) memset(vis,0,sizeof(vis));
for(int i=1; i<=n; ++i)
for(int j=1;j<=m;++j)
scanf("%d",&maze[i][j]);
scanf("%d%d%d%d%s",&sx,&sy,&ex,&ey,ddd);
char sss[] = "ESWN666";
for(int i=0;i<4;++i)
if(sss[i]==ddd[0]) sdir = i;
bfs();
}
return 0;
}
bool ok(int x,int y,int dir)
{
return (x>0&&x<=n&&y>0&&y<=m&&!vis[x][y][dir]&&maze[x][y]==0);
}
bool out(int x,int y)
{
return (x<=0||x>n||y<=0||y>m);
}
pair<int,int> move(int x,int y,int dir,int len)
{
switch (dir)
{
case 0: return make_pair(x,y+len);
case 1: return make_pair(x+len,y);
case 2: return make_pair(x,y-len);
case 3: return make_pair(x-len,y);
}
return make_pair(0,0);
}
bool checkCreep(int xx,int yy,int dir,int &nx,int &ny)
{
pair<int,int> pr = move(xx,yy,dir,1);
int x = pr.first, y = pr.second;
nx = x, ny = y;
if(out(x,y)||out(x+1,y)||out(x,y+1)||out(x+1,y+1)) return false;
if(maze[x][y]||maze[x+1][y]||maze[x][y+1]||maze[x+1][y+1]) return false;
return !vis[x][y][dir];
}
bool checkWalk(int xx,int yy,int dir,int& nx,int& ny)
{
pair<int,int> pr = move(xx,yy,dir,1);
int x = pr.first, y = pr.second;
if(out(x,y)||out(x+1,y)||out(x,y+1)||out(x+1,y+1)) return false;
if(maze[x][y]||maze[x+1][y]||maze[x][y+1]||maze[x+1][y+1]) return false;
pr = move(xx,yy,dir,2);
x = pr.first, y = pr.second;
if(out(x,y)||out(x+1,y)||out(x,y+1)||out(x+1,y+1)) return false;
if(maze[x][y]||maze[x+1][y]||maze[x][y+1]||maze[x+1][y+1]) return false;
nx = x, ny = y;
return !vis[x][y][dir];
}
bool checkRun(int xx,int yy,int dir,int& nx,int &ny)
{
pair<int,int> pr = move(xx,yy,dir,1);
int x = pr.first, y = pr.second;
if(out(x,y)||out(x+1,y)||out(x,y+1)||out(x+1,y+1)) return false;
if(maze[x][y]||maze[x+1][y]||maze[x][y+1]||maze[x+1][y+1]) return false;
pr = move(xx,yy,dir,2);
x = pr.first, y = pr.second;
if(out(x,y)||out(x+1,y)||out(x,y+1)||out(x+1,y+1)) return false;
if(maze[x][y]||maze[x+1][y]||maze[x][y+1]||maze[x+1][y+1]) return false;
pr = move(xx,yy,dir,3);
x = pr.first, y = pr.second;
if(out(x,y)||out(x+1,y)||out(x,y+1)||out(x+1,y+1)) return false;
if(maze[x][y]||maze[x+1][y]||maze[x][y+1]||maze[x+1][y+1]) return false;
nx = x, ny = y;
return !vis[x][y][dir];
}
void bfs()
{
queue<Node> Q;
Q.push(Node(sx,sy,0,sdir));
vis[sx][sy][sdir] = true;
int ans = -1;
while(!Q.empty())
{
node = Q.front();
Q.pop();
int x = node.x, y = node.y;
int step = node.step, dir = node.dir;
if(x==ex&&y==ey)
{
ans = step;
break;
}
int nx=0,ny=0;
if(checkRun(x,y,dir,nx,ny))
{
vis[nx][ny][dir] = true;
Q.push(Node(nx,ny,step+1,dir));
}
if(checkWalk(x,y,dir,nx,ny))
{
vis[nx][ny][dir] = true;
Q.push(Node(nx,ny,step+1,dir));
}
if(checkCreep(x,y,dir,nx,ny))
{
vis[nx][ny][dir] = true;
Q.push(Node(nx,ny,step+1,dir));
}
if(!vis[x][y][toLeft(dir)])
{
vis[x][y][toLeft(dir)] = true;
Q.push(Node(x,y,step+1,toLeft(dir)));
}
if(!vis[x][y][toRight(dir)])
{
vis[x][y][toRight(dir)] = true;
Q.push(Node(x,y,step+1,toRight(dir)));
}
}
printf("%d
",ans);
}
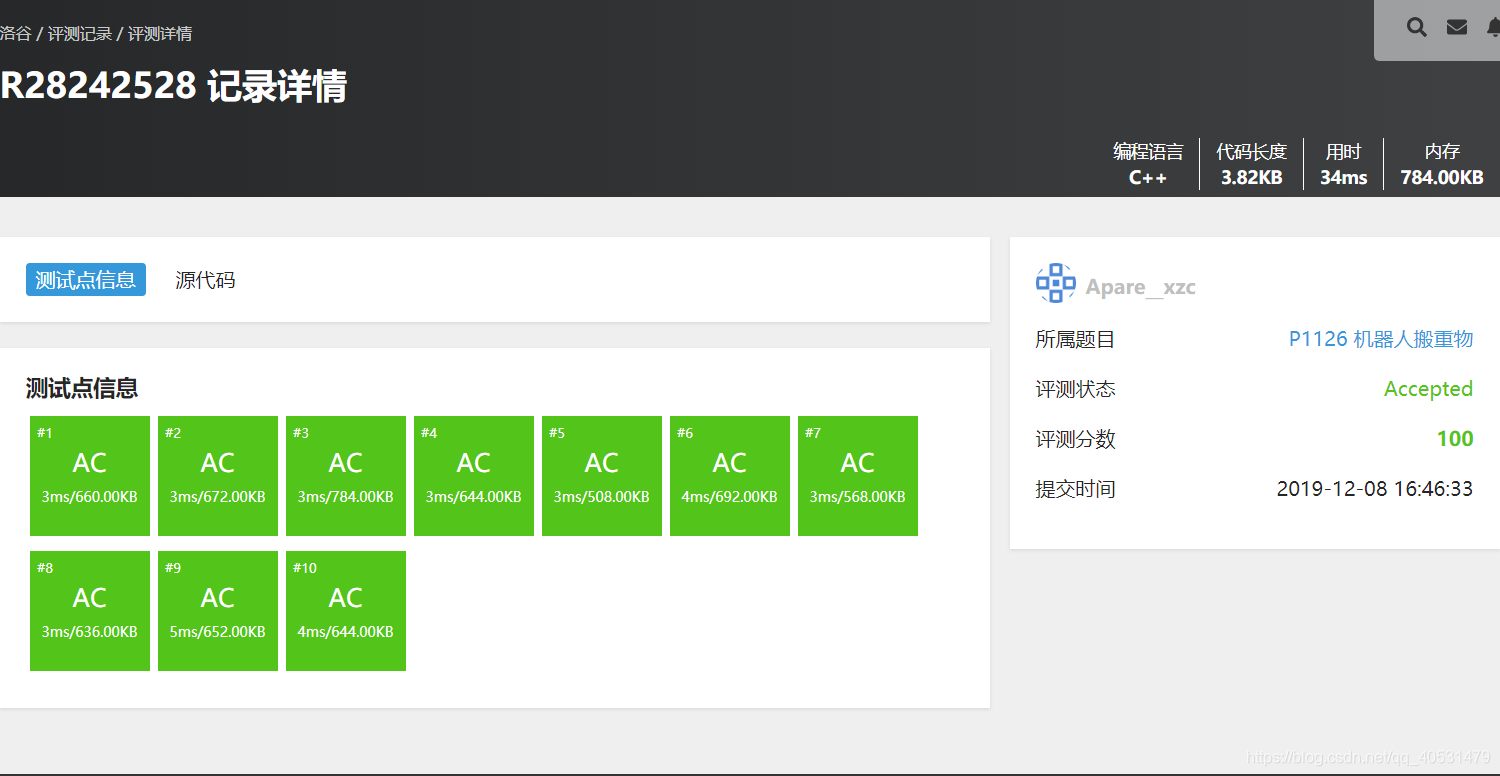