Time Limit: 3000MS | Memory Limit: Unknown | 64bit IO Format: %lld & %llu |
Description
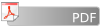
A robot has to patrol around a rectangular area which is in a form of mxn grid (m rows and n columns). The rows are labeled from 1 to m. The columns are labeled from 1 to n. A cell (i, j) denotes the cell in row i and column j in the grid. At each step, the robot can only move from one cell to an adjacent cell, i.e. from (x, y) to (x+ 1, y), (x, y + 1), (x - 1, y) or (x, y - 1). Some of the cells in the grid contain obstacles. In order to move to a cell containing obstacle, the robot has to switch to turbo mode. Therefore, the robot cannot move continuously to more than k cells containing obstacles.
Your task is to write a program to find the shortest path (with the minimum number of cells) from cell (1, 1) to cell (m, n). It is assumed that both these cells do not contain obstacles.
Input
The input consists of several data sets. The first line of the input file contains the number of data sets which is a positive integer and is not bigger than 20. The following lines describe the data sets.
For each data set, the first line contains two positive integer numbers m and n separated by space (1m, n
20). The second line contains an integer number k(0
k
20). The ith line of the next m lines contains n integer aij separated by space (i = 1, 2,..., m;j = 1, 2,..., n). The value of aij is 1 if there is an obstacle on the cell (i,j), and is 0 otherwise.
Output
For each data set, if there exists a way for the robot to reach the cell (m, n), write in one line the integer number s, which is the number of moves the robot has to make; -1 otherwise.
Sample Input
3 2 5 0 0 1 0 0 0 0 0 0 1 0 4 6 1 0 1 1 0 0 0 0 0 1 0 1 1 0 1 1 1 1 0 0 1 1 1 0 0 2 2 0 0 1 1 0
Sample Output
7 10 -1
一开始想用A*来着,g设为当前步数,h为曼哈顿距离,但似乎第三组死循环了……看来A*应用不够熟练啊,然后改为普通bfs,只用了二维的数组记录状态WA……抱着试一试的心态加了一维表示个当前点被穿过k步走过的记录,没想到A了……
代码:
#include<iostream> #include<algorithm> #include<cstdlib> #include<sstream> #include<cstring> #include<cstdio> #include<string> #include<deque> #include<stack> #include<cmath> #include<queue> #include<set> #include<map> using namespace std; #define INF 0x3f3f3f3f #define MM(x,y) memset(x,y,sizeof(x)) typedef pair<int,int> pii; typedef long long LL; const double PI=acos(-1.0); const int N=25; int m,n,k; int pos[N][N]; int vis[N][N][N]; struct info { int x; int y; int g; int kk; }; queue<info>Q; info direct[4]={{0,1,1,0},{1,0,1,0},{0,-1,1,0},{-1,0,1,0}}; inline info operator+(const info &a,const info &b) { info c; c.x=a.x+b.x; c.y=a.y+b.y; c.g=a.g+b.g; return c; } bool check(const info &a) { return (a.x>=0&&a.x<m&&a.y>=0&&a.y<n&&a.kk<=k); } void init() { MM(pos,0); MM(vis,0); while (!Q.empty()) Q.pop(); } int main(void) { int tcase,i,j; scanf("%d",&tcase); while (tcase--) { init(); scanf("%d%d",&m,&n); scanf("%d",&k); for (i=0; i<m; ++i) { for (j=0; j<n; ++j) scanf("%d",&pos[i][j]); } int r=-1; info S={0,0,0,0}; vis[S.x][S.y][S.kk]=1; Q.push(S); while (!Q.empty()) { info now=Q.front(); Q.pop(); if(now.x==m-1&&now.y==n-1) { r=now.g; break; } for (i=0; i<4; i++) { info v=now+direct[i]; if(!pos[v.x][v.y]) v.kk=0; else v.kk=now.kk+1; if(check(v)&&!vis[v.x][v.y][v.kk]) { Q.push(v); vis[v.x][v.y][v.kk]=1; } } } printf("%d ",r); } return 0; }
A*代码:
#include<iostream> #include<algorithm> #include<cstdlib> #include<sstream> #include<cstring> #include<cstdio> #include<string> #include<deque> #include<stack> #include<cmath> #include<queue> #include<set> #include<map> using namespace std; #define INF 0x3f3f3f3f #define MM(x,y) memset(x,y,sizeof(x)) typedef pair<int,int> pii; typedef long long LL; const double PI=acos(-1.0); const int N=25; int m,n,k; int pos[N][N]; int vis[N][N][N]; struct info { int x; int y; int g; int kk; int h; bool operator<(const info &b)const { if(h+g!=b.h+b.g) return h+g>b.h+b.g; if(g!=b.g) return g>b.g; return kk>b.kk; } }; priority_queue<info>Q; info direct[4]={{0,1,1,0,0},{1,0,1,0,0},{0,-1,1,0,0},{-1,0,1,0,0}}; inline info operator+(const info &a,const info &b) { info c; c.x=a.x+b.x; c.y=a.y+b.y; c.g=a.g+b.g; return c; } bool check(const info &a) { return (a.x>=0&&a.x<m&&a.y>=0&&a.y<n&&a.kk<=k&&!vis[a.x][a.y][a.kk]); } void init() { MM(pos,0); MM(vis,0); while (!Q.empty()) Q.pop(); } int main(void) { int tcase,i,j; scanf("%d",&tcase); while (tcase--) { init(); scanf("%d%d",&m,&n); scanf("%d",&k); for (i=0; i<m; ++i) { for (j=0; j<n; ++j) { scanf("%d",&pos[i][j]); } } int r=-1; info S={0,0,0,0,n+m-2}; vis[S.x][S.y][S.kk]=1; Q.push(S); while (!Q.empty()) { info now=Q.top(); Q.pop(); if(now.x==m-1&&now.y==n-1) { r=now.g; break; } for (i=0; i<4; i++) { info v=now+direct[i]; if(!pos[v.x][v.y]) v.kk=0; else v.kk=now.kk+1; if(check(v)) { v.h=m-1+n-1-v.x-v.y; Q.push(v); vis[v.x][v.y][v.kk]=1; } } } printf("%d ",r); } return 0; }