题目定义:
实现一个二叉搜索树迭代器。你将使用二叉搜索树的根节点初始化迭代器。
调用 next() 将返回二叉搜索树中的下一个最小的数。
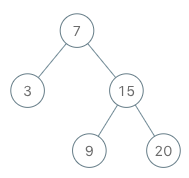
BSTIterator iterator = new BSTIterator(root);
iterator.next(); // 返回 3
iterator.next(); // 返回 7
iterator.hasNext(); // 返回 true
iterator.next(); // 返回 9
iterator.hasNext(); // 返回 true
iterator.next(); // 返回 15
iterator.hasNext(); // 返回 true
iterator.next(); // 返回 20
iterator.hasNext(); // 返回 false
方式一(运用栈):
class BSTIterator {
private Deque<TreeNode> stack;
public BSTIterator(TreeNode root) {
stack = new ArrayDeque<>();
TreeNode cur = root;
while(cur != null){
if(cur.left == null){
stack.add(new TreeNode(cur.val));
cur = cur.right;
}else{
TreeNode pre = cur.left;
while(pre.right != null && pre.right != cur){
pre = pre.right;
}
if(pre.right == null){
pre.right = cur;
cur = cur.left;
}else{
pre.right = null;
stack.add(new TreeNode(cur.val));
cur = cur.right;
}
}
}
}
public int next() {
return stack.pop().val;
}
public boolean hasNext() {
return !stack.isEmpty();
}
}
方式二(中序遍历):
class BSTIterator {
private List<Integer> inOrder;
private int index;
public BSTIterator(TreeNode root) {
inOrder = new ArrayList<>();
index = -1;
inOrderTravel(root);
}
private void inOrderTravel(TreeNode root){
if(root == null)
return;
inOrderTravel(root.left);
this.inOrder.add(root.val);
inOrderTravel(root.right);
}
public int next() {
return inOrder.get(++index);
}
public boolean hasNext() {
return inOrder.size() - index - 1 > 0;
}
}
方式三(只保存左子树节点):
class BSTIterator {
private Stack<TreeNode> stack;
public BSTIterator(TreeNode root) {
stack = new Stack<>();
this.leftMostInOrder(root);
}
private void leftMostInOrder(TreeNode root){
while(root != null){
stack.push(root);
root = root.left;
}
}
public int next() {
TreeNode node = stack.pop();
if(node.right != null)
leftMostInOrder(node.right);
return node.val;
}
public boolean hasNext() {
return !stack.isEmpty();
}
}
参考:
https://leetcode-cn.com/problems/binary-search-tree-iterator/solution/er-cha-sou-suo-shu-die-dai-qi-by-leetcode/