# PySimpleGUI:
# 在PySimpleGUI中,小部件(widgets)被称为element,element的名称与编码中使用的名称保持一致。
# PySimpleGUI中有两种类型的窗口:1) one-shot window 2) persistent window(multiple reads using an event loop)
# 一、两种窗口模式:
# 1--one-shot window:
# 特点:read and then closes
# 语法:sg.Window("window Title",layout) 其中 layout 为三个一维数组构成的二维数组
# layout=[[sg.Text("my one-shot window")],[sg.InputText()],[sg.Submit(),sg.Cancel()]]
# 例 1:
from pathlib import Path
import PySimpleGUI as sg
layout=[[sg.Text("my one-shot window")],[sg.InputText()],[sg.Submit(),sg.Cancel()]]
Window=sg.Window("Window Title",layout)
event,values=Window.Read() # event的值为 所点击的 button的名称,values为字典,字典的值为输入的内容
Window.Close() # 不可少
print(event) # "submit" or "cancel"
print(values) # {0: 'hello world!'}
print(values[0]) # 'hello world!'
# 2--Persistent window (multiple reads using an event loop)
# 特点:该模式实现多次读取
# 语法:
# 例 1:
from pathlib import Path
import PySimpleGUI as sg
# layout=[[sg.Text("my persistent window")],[sg.InputText(do_not_clear=True)],[sg.Submit(),sg.Exit()]]
# layout=[[sg.Text("my persistent window")],[sg.InputText(do_not_clear=True)],[sg.Button("Submit"),sg.Exit()]]
layout=[[sg.Text("my persistent window")],[sg.InputText(do_not_clear=True)],[sg.Button("Read"),sg.Exit()]]
# do_not_clear默认为 True
while True:
event,values=Window.Read() # event的值为 所点击的 button的名称,values为字典,字典的值为输入的内容
if event is None or event == "Exit":
break
print(event,values)
Window.Close() # 不可少
# 例 2:
from pathlib import Path
import PySimpleGUI as sg
layout=[[sg.Text("my persistent window")],[sg.InputText(do_not_clear=False)],[sg.Submit(),sg.Exit()]]
Window=sg.Window("Window Title",layout)
while True:
event,values=Window.Read() # event的值为 所点击的 button的名称,values为字典,字典的值为输入的内容
if event is None or event == "Exit":
break
print(event,values)
Window.Close() # 不可少
# 二、应用:
# 1--创建数据表:
# layout里有多个 sg.Text()
from pathlib import Path
import PySimpleGUI as sg
layout=[
[sg.Text("Please enter Name,Address,Phone")],
[sg.Text("Name",size=(15,1)),sg.InputText()], # key为 0
[sg.Text("Address",size=(15,1)),sg.InputText("辽宁省黄泥川",key="address")], # 可以有默认值,可以指定 key
[sg.Text("Phone",size=(15,1)),sg.InputText()], # 因为 address指定了 key,phone的 key就变为 1了。
[sg.Submit(),sg.Cancel()]]
Window=sg.Window('Simple data entry window',layout)
event,values=Window.Read()
Window.Close()
print(event,values[0],values["address"],values[1]) # Submit Collin 辽宁省黄泥川 17763230890
# 2--文件浏览器:
# 例1:
from pathlib import Path
import PySimpleGUI as sg
layout = [[sg.Text('请选择一个文件')],
[sg.InputText(), sg.FileBrowse()],
[sg.Submit(), sg.Cancel()]]
# with sg.Window('SHA-1 & 256 Hash', layout) as window:
# (event, (source_filename,)) = window.Read()
# print(event, source_filename)
Window=sg.Window('文件浏览器',layout)
event,value = Window.Read()
Window.Close()
print(value[0]) # C:/Users/XuYunPeng/Desktop/工具/Collin_test/log/20200721140356.log
# 例2:
layout = [[sg.Text('Enter 2 files to comare')],
[sg.Text('File 1', size=(8, 1)), sg.InputText(), sg.FileBrowse()],
[sg.Text('File 2', size=(8, 1)), sg.InputText(), sg.FileBrowse()],
[sg.Submit(), sg.Cancel()]]
window = sg.Window('File Compare', layout)
event, values = window.Read()
window.Close()
print(event, values)
# 例3:
import PySimpleGUI as sg
import sys
layout = [[sg.Text('Document to open')],[sg.In(), sg.FileBrowse()], [sg.CloseButton('Open'), sg.CloseButton('Cancel')]]
if len(sys.argv) == 1:
Window=sg.Window('My Script',layout)
event, (fname,) = Window.Read()
Window.Close()
else:
fname = sys.argv[1]
if not fname:
sg.Popup("Cancel", "No filename supplied")
raise SystemExit("Cancelling: no filename supplied")
print(event, fname)
# 3--创建可复选的表单
import PySimpleGUI as sg
# def window_op(title, layout):
# with sg.Window(title, layout) as window:
# event, values = window.Read()
# return event, values
def window_op(title, layout):
window=sg.Window(title, layout)
event, values = window.Read()
window.Close()
return event, values
layout = [[sg.Text('你喜欢的故事书是:')],
[sg.Text('故事书:'), sg.Checkbox('哈利波特', sg.OK()), sg.Checkbox('三体'), sg.OK()]]
window_op("test",layout) # ('OK', {0: True, 1: True})
# 4--pop 弹窗 (可以用来替代 print)
import PySimpleGUI as sg
v="坏蛋们"
sg.Popup('标题:',
'你们已经被包围了,"{}"'.format(v),
'快投降吧', "哈哈")
# 5--其他窗口元素:Listbox,Multiline,Menu,InputOptionMenu,Slider,InputText,Text,Frame,column等
import PySimpleGUI as sg
sg.ChangeLookAndFeel('GreenTan') # 绿色的配色方案
# ------ Menu Definition ------ #
menu_def = [['File', ['Open', 'Save', 'Exit', 'Properties']],
['Edit', ['Paste', ['Special', 'Normal', ], 'Undo'], ],
['Help', 'About...'], ]
# ------ Column Definition ------ #
column1 = [[sg.Text('Column 1', background_color='#F7F3EC', justification='center', size=(10, 1))],
[sg.Spin(values=('Spin Box 1', '2', '3'),
initial_value='Spin Box 1')],
[sg.Spin(values=('Spin Box 1', '2', '3'),
initial_value='Spin Box 2')],
[sg.Spin(values=('Spin Box 1', '2', '3'), initial_value='Spin Box 3')]]
layout = [
[sg.Menu(menu_def, tearoff=True)],
[sg.Text('All graphic widgets in one window!', size=(
30, 1), justification='center', font=("Helvetica", 25), relief=sg.RELIEF_RIDGE)],
[sg.Text('Here is some text.... and a place to enter text')],
[sg.InputText('This is my text')],
[sg.Frame(layout=[
[sg.Checkbox('Checkbox', size=(10, 1)), sg.Checkbox(
'My second checkbox!', default=True)],
[sg.Radio('My first Radio! ', "RADIO1", default=True, size=(10, 1)), sg.Radio('My second Radio!', "RADIO1")]], title='Options', title_color='red', relief=sg.RELIEF_SUNKEN, tooltip='Use these to set flags')],
[sg.Multiline(default_text='This is the default Text should you decide not to type anything', size=(35, 3)),
sg.Multiline(default_text='A second multi-line', size=(35, 3))],
[sg.InputCombo(('Combobox 1', 'Combobox 2'), size=(20, 1)),
sg.Slider(range=(1, 100), orientation='h', size=(34, 20), default_value=85)],
[sg.InputOptionMenu(('Menu Option 1', 'Menu Option 2', 'Menu Option 3'))],
[sg.Listbox(values=('Listbox 1', 'Listbox 2', 'Listbox 3'), size=(30, 3)),
sg.Frame('Labelled Group', [[
sg.Slider(range=(1, 100), orientation='v',
size=(5, 20), default_value=25),
sg.Slider(range=(1, 100), orientation='v',
size=(5, 20), default_value=75),
sg.Slider(range=(1, 100), orientation='v',
size=(5, 20), default_value=10),
sg.Column(column1, background_color='#F7F3EC')]])],
[sg.Text('_' * 80)],
[sg.Text('Choose A Folder', size=(35, 1))],
[sg.Text('Your Folder', size=(15, 1), auto_size_text=False, justification='right'),
sg.InputText('Default Folder'), sg.FolderBrowse()],
[sg.Submit(tooltip='Click to submit this window'), sg.Cancel()]
]
window = sg.Window('Everything bagel', layout,
default_element_size=(40, 1), grab_anywhere=False)
event, values = window.Read()
window.Close()
sg.Popup('Title',
'The results of the window.',
'The button clicked was "{}"'.format(event),
'The values are', values)
# 6--其他的例子:
https://www.jianshu.com/p/02f3839e143b
Author: Collin Wechat: pxy123abc Tel:17763230890
```Collin
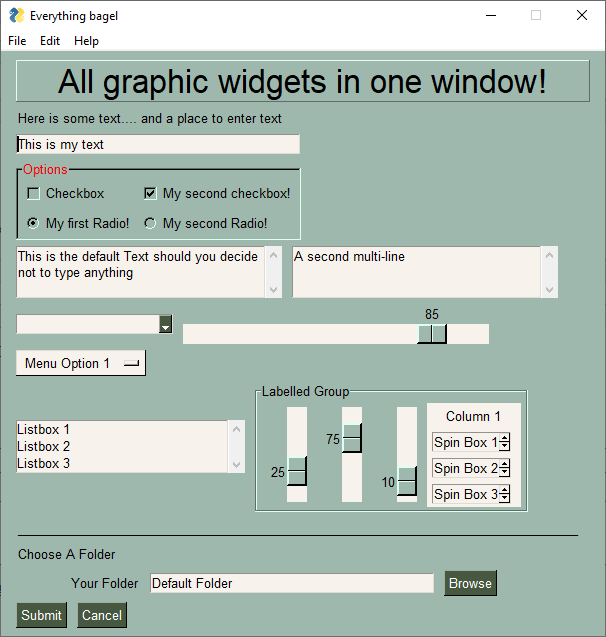