2020蓝桥杯校内模拟赛(一) -- 比较简单
前四题基础填空比较水为 计算器算就行
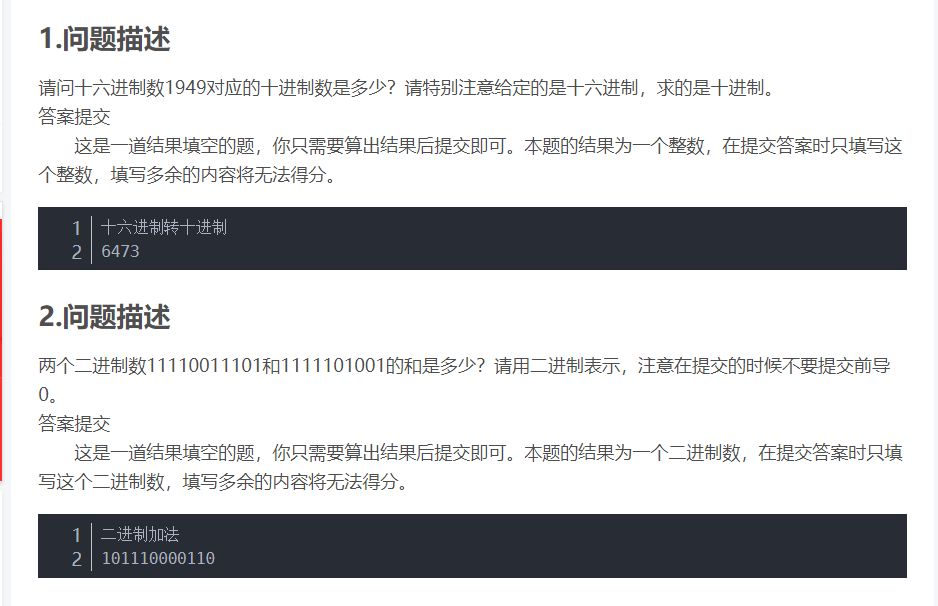
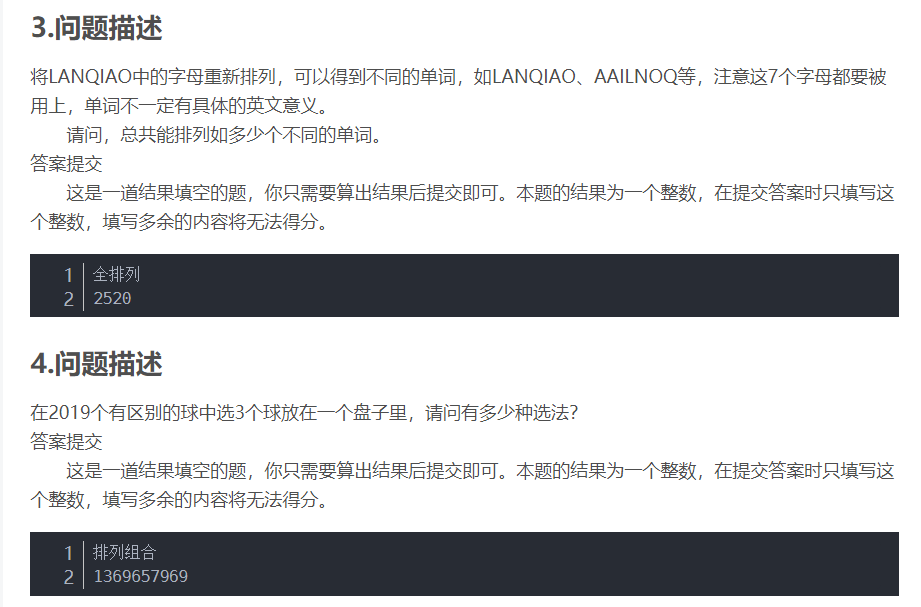
程序代码题
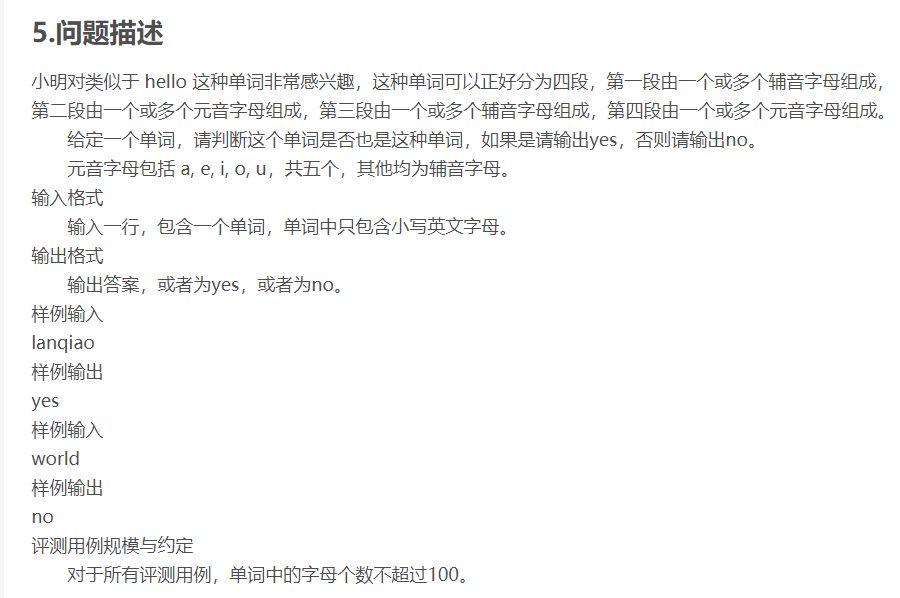
#include <string>
#include <iostream>
using namespace std;
char c[5] = { 'a','e','i','o','u' };
int check(char s) {
int tag = 0;
for (int i = 0; i < 5; i++) {
if (s == c[i]) {
tag = 1;
}
}
if (tag == 1) return 1;
return 0;
}
int main() {
string s;
cin >> s;
int i = 0, j;
int tag = 0, cnt = 0;//0为辅音
while (i < s.size()) {
while (check(s[i]) == tag && i < s.size()) {
i++;
}
if (tag) tag = 0;//状态翻转
else
tag = 1;
cnt++;
}
if (cnt == 4) {
printf("Yes
");
}
else printf("No
");
return 0;
}
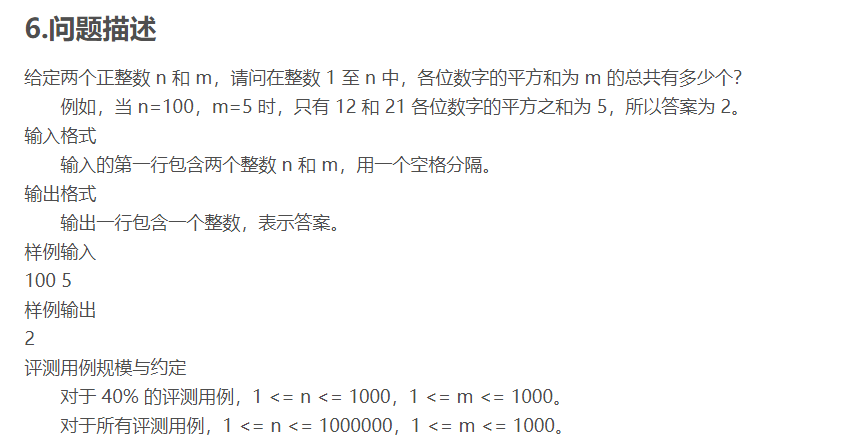
#include <string>
#include <iostream>
using namespace std;
int Sum(int a) {
int sum = 0;
while (a) {
sum += (a % 10) * (a % 10);
a /= 10;
}
return sum;
}
int main() {
int n, m;
int ans = 0;
cin >> n >> m;
for (int i = 1; i <= n; i++) {
if (Sum(i) == m) ans++;
}
cout << ans << endl;
return 0;
}
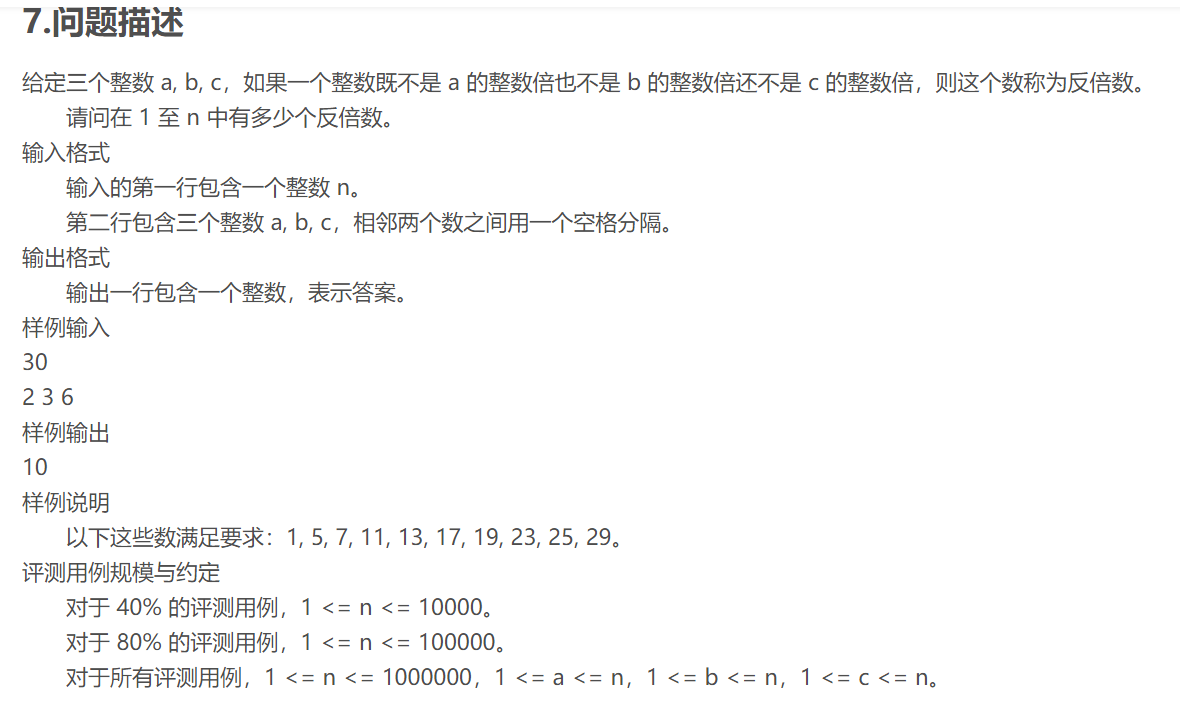
#include <string>
#include <iostream>
using namespace std;
int main() {
int n, a, b, c;
cin >> n;
cin >> a >> b >> c;
int cnt = 0;
for (int i = 1; i <= n; i++) {
if (i % a != 0 && i % b != 0 && i % c != 0) {
cnt++;
}
}
cout << cnt << endl;
return 0;
}
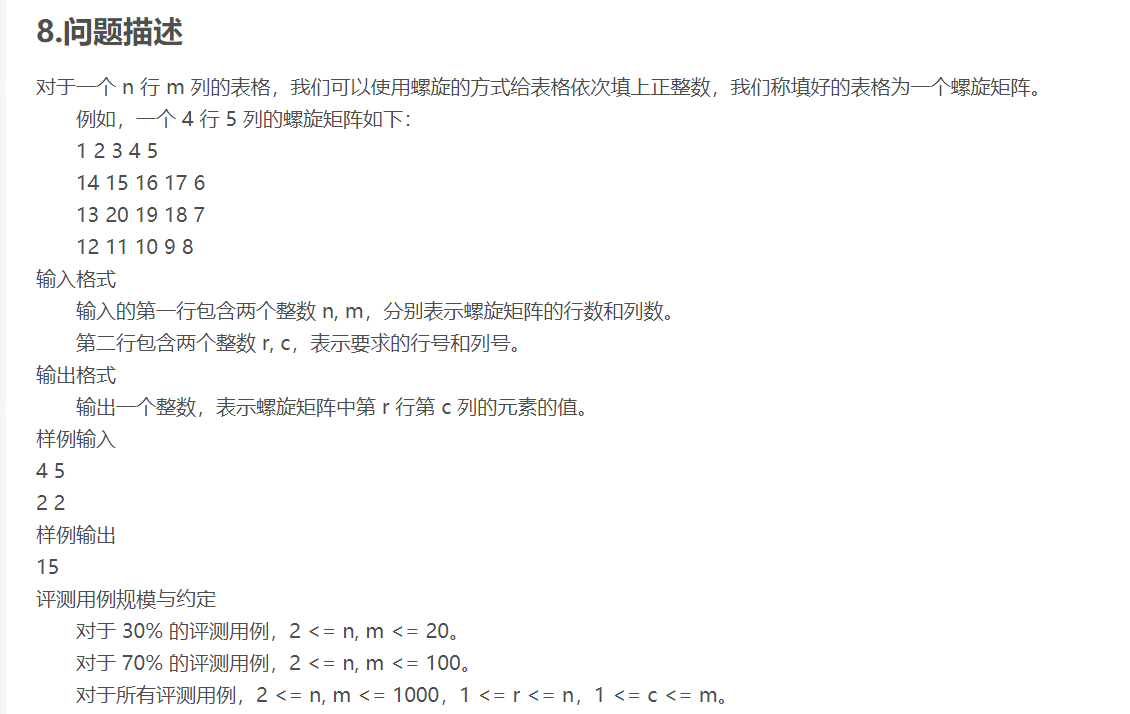
O(m * n)最坏时间复杂度
#include <string>
#include <iostream>
using namespace std;
int n, m;
int r, c;
bool in(int x, int y) {
if (x + 1 == c && y + 1 == r) {
return true;
}
else
return false;
}
int main() {
cin >> n >> m;
cin >> c >> r;
int x = 0, y = 0;//初始位置
int a = 0 , b = 0;
int s = 0;
while (1) {//模拟过程
for (int i = 0; i < m - 2 * a; i++) { // 右走
s++;
if (in(x, y)) {
cout << s << endl;
return 0;
}
y++;
}
y--;
x++;
for (int i = 0; i < n - 2 * a - 1; i++) {// 下走
s++;
if (in(x, y)) {
cout << s << endl;
return 0;
}
x++;
}
x--;
y--;
for (int i = 0; i < m - 2 * a - 1; i++) { // 左走
s++;
if (in(x, y)) {
cout << s << endl;
return 0;
}
y--;
}
y++;
x--;
for (int i = 0; i < n - 2 * a - 2; i++) { // 上走
s++;
if (in(x, y)) {
cout << s << endl;
return 0;
}
x--;
}
x++;
y++;
a++; //进入下一个模拟
}
return 0;
}
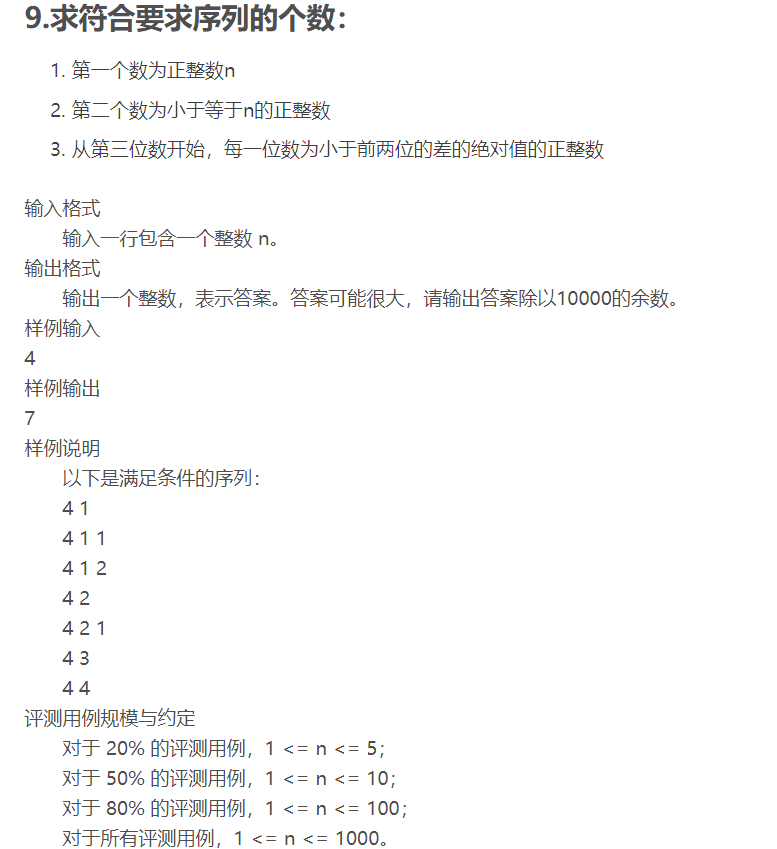
非DP做法
#include <string>
#include <iostream>
#include <vector>
#include <math.h>
using namespace std;
int num = 0;
void dfs( int a, int b);
void f( int i, int n)
{
num++;
num = num % 10000;
if (abs(n - i) > 1)
dfs( n, i);
}
void dfs( int a, int b)
{
int c = abs(a - b);
for (int i = 1; i < c; i++)
{
f( i, b);
}
}
int main()
{
int n;
cin >> n;
for (int i = 1; i <= n; i++)
f( i, n);
cout << num;
return 0;
}
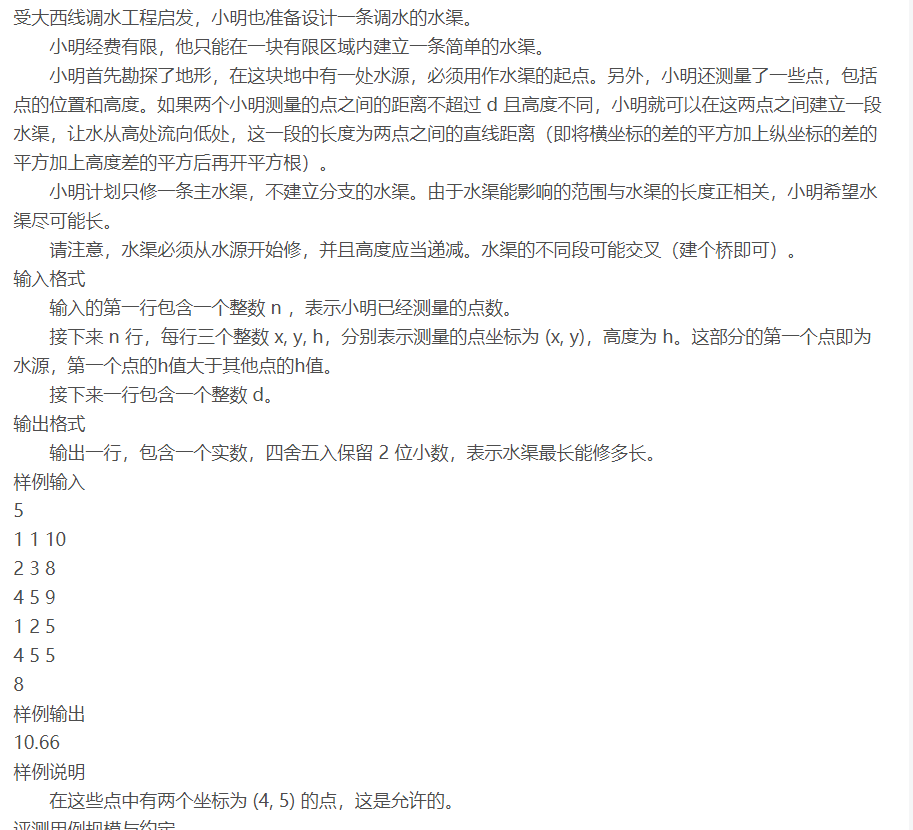
//dfs
#include <bits/stdc++.h>
using namespace std;
int n;double d;
struct s{
double x,y,h;
};
s ss[1001];
double ma=0;
int c(s a,s b)
{
return a.h>b.h;
}
void f(int a,double sum,double hh)
{
//cout<<a<<" "<<sum<<" "<<hh<<endl;
if(sum>ma)ma=sum;
for(int i=a+1;i<n;i++)
{
if(ss[i].h<ss[a].h)
{
double y=sqrt((ss[a].x-ss[i].x)*(ss[a].x-ss[i].x)+(ss[a].y-ss[i].y)*(ss[a].y-ss[i].y));
double x=sqrt((ss[a].x-ss[i].x)*(ss[a].x-ss[i].x)+(ss[a].y-ss[i].y)*(ss[a].y-ss[i].y)+(ss[a].h-ss[i].h)*(ss[a].h-ss[i].h));
if(x<=d)
{
f(i,sum+y,ss[i].h);
}
}
}
}
int main()
{
cin>>n;
for(int i=0;i<n;i++)
{
cin>>ss[i].x>>ss[i].y>>ss[i].h;
}
sort(ss,ss+n,c);
//cout<<ss[0].h<<endl;
cin>>d;
//for(int i=0;i<n;i++)
//{
f(0,0,ss[0].h);
//}
printf("%.2lf
",ma);
return 0;
}
DP做法
- 数组dp[i][j]储存以ij为前缀,能衍生出的子序列的个数。
- 例如,以41为前缀,可以衍生出411、412两种,再加上41本身就是3种。
- 递推关系:dp[i][j] = dp[j][1] + … + dp[j][abs(i-j)-1] + 1(ij本身)
#include <iostream>
#include <vector>
using namespace std;
const int N = 1001;
vector<vector<int>> dp(N, vector<int>(N, -1));
int countSeq(int first, int second)
{
if (dp[first][second] != -1)
return dp[first][second];
int t = 1;
for (int i = 1; i < abs(first - second); i++)
{
t += countSeq(second, i);
}
dp[first][second] = t % 10000;
return dp[first][second];
}
int main()
{
int n, ans(0);
cin >> n;
dp[1][1] = 1;
for (int i = 1; i <= n; i++)
{
ans = (ans + countSeq(n, i)) % 10000;
}
cout << ans << endl;
return 0;
}