1 collections系列
方法如下

1 class Counter(dict): 2 '''Dict subclass for counting hashable items. Sometimes called a bag 3 or multiset. Elements are stored as dictionary keys and their counts 4 are stored as dictionary values. 5 6 >>> c = Counter('abcdeabcdabcaba') # count elements from a string 7 8 >>> c.most_common(3) # three most common elements 9 [('a', 5), ('b', 4), ('c', 3)] 10 >>> sorted(c) # list all unique elements 11 ['a', 'b', 'c', 'd', 'e'] 12 >>> ''.join(sorted(c.elements())) # list elements with repetitions 13 'aaaaabbbbcccdde' 14 >>> sum(c.values()) # total of all counts 15 15 16 17 >>> c['a'] # count of letter 'a' 18 5 19 >>> for elem in 'shazam': # update counts from an iterable 20 ... c[elem] += 1 # by adding 1 to each element's count 21 >>> c['a'] # now there are seven 'a' 22 7 23 >>> del c['b'] # remove all 'b' 24 >>> c['b'] # now there are zero 'b' 25 0 26 27 >>> d = Counter('simsalabim') # make another counter 28 >>> c.update(d) # add in the second counter 29 >>> c['a'] # now there are nine 'a' 30 9 31 32 >>> c.clear() # empty the counter 33 >>> c 34 Counter() 35 36 Note: If a count is set to zero or reduced to zero, it will remain 37 in the counter until the entry is deleted or the counter is cleared: 38 39 >>> c = Counter('aaabbc') 40 >>> c['b'] -= 2 # reduce the count of 'b' by two 41 >>> c.most_common() # 'b' is still in, but its count is zero 42 [('a', 3), ('c', 1), ('b', 0)] 43 44 ''' 45 # References: 46 # http://en.wikipedia.org/wiki/Multiset 47 # http://www.gnu.org/software/smalltalk/manual-base/html_node/Bag.html 48 # http://www.demo2s.com/Tutorial/Cpp/0380__set-multiset/Catalog0380__set-multiset.htm 49 # http://code.activestate.com/recipes/259174/ 50 # Knuth, TAOCP Vol. II section 4.6.3 51 52 def __init__(*args, **kwds): 53 '''Create a new, empty Counter object. And if given, count elements 54 from an input iterable. Or, initialize the count from another mapping 55 of elements to their counts. 56 57 >>> c = Counter() # a new, empty counter 58 >>> c = Counter('gallahad') # a new counter from an iterable 59 >>> c = Counter({'a': 4, 'b': 2}) # a new counter from a mapping 60 >>> c = Counter(a=4, b=2) # a new counter from keyword args 61 62 ''' 63 if not args: 64 raise TypeError("descriptor '__init__' of 'Counter' object " 65 "needs an argument") 66 self = args[0] 67 args = args[1:] 68 if len(args) > 1: 69 raise TypeError('expected at most 1 arguments, got %d' % len(args)) 70 super(Counter, self).__init__() 71 self.update(*args, **kwds) 72 73 def __missing__(self, key): 74 'The count of elements not in the Counter is zero.' 75 # Needed so that self[missing_item] does not raise KeyError 76 return 0 77 78 def most_common(self, n=None): 79 '''List the n most common elements and their counts from the most 80 common to the least. If n is None, then list all element counts. 81 82 >>> Counter('abcdeabcdabcaba').most_common(3) 83 [('a', 5), ('b', 4), ('c', 3)] 84 85 ''' 86 # Emulate Bag.sortedByCount from Smalltalk 87 if n is None: 88 return sorted(self.iteritems(), key=_itemgetter(1), reverse=True) 89 return _heapq.nlargest(n, self.iteritems(), key=_itemgetter(1)) 90 91 def elements(self): 92 '''Iterator over elements repeating each as many times as its count. 93 94 >>> c = Counter('ABCABC') 95 >>> sorted(c.elements()) 96 ['A', 'A', 'B', 'B', 'C', 'C'] 97 98 # Knuth's example for prime factors of 1836: 2**2 * 3**3 * 17**1 99 >>> prime_factors = Counter({2: 2, 3: 3, 17: 1}) 100 >>> product = 1 101 >>> for factor in prime_factors.elements(): # loop over factors 102 ... product *= factor # and multiply them 103 >>> product 104 1836 105 106 Note, if an element's count has been set to zero or is a negative 107 number, elements() will ignore it. 108 109 ''' 110 # Emulate Bag.do from Smalltalk and Multiset.begin from C++. 111 return _chain.from_iterable(_starmap(_repeat, self.iteritems())) 112 113 # Override dict methods where necessary 114 115 @classmethod 116 def fromkeys(cls, iterable, v=None): 117 # There is no equivalent method for counters because setting v=1 118 # means that no element can have a count greater than one. 119 raise NotImplementedError( 120 'Counter.fromkeys() is undefined. Use Counter(iterable) instead.') 121 122 def update(*args, **kwds): 123 '''Like dict.update() but add counts instead of replacing them. 124 125 Source can be an iterable, a dictionary, or another Counter instance. 126 127 >>> c = Counter('which') 128 >>> c.update('witch') # add elements from another iterable 129 >>> d = Counter('watch') 130 >>> c.update(d) # add elements from another counter 131 >>> c['h'] # four 'h' in which, witch, and watch 132 4 133 134 ''' 135 # The regular dict.update() operation makes no sense here because the 136 # replace behavior results in the some of original untouched counts 137 # being mixed-in with all of the other counts for a mismash that 138 # doesn't have a straight-forward interpretation in most counting 139 # contexts. Instead, we implement straight-addition. Both the inputs 140 # and outputs are allowed to contain zero and negative counts. 141 142 if not args: 143 raise TypeError("descriptor 'update' of 'Counter' object " 144 "needs an argument") 145 self = args[0] 146 args = args[1:] 147 if len(args) > 1: 148 raise TypeError('expected at most 1 arguments, got %d' % len(args)) 149 iterable = args[0] if args else None 150 if iterable is not None: 151 if isinstance(iterable, Mapping): 152 if self: 153 self_get = self.get 154 for elem, count in iterable.iteritems(): 155 self[elem] = self_get(elem, 0) + count 156 else: 157 super(Counter, self).update(iterable) # fast path when counter is empty 158 else: 159 self_get = self.get 160 for elem in iterable: 161 self[elem] = self_get(elem, 0) + 1 162 if kwds: 163 self.update(kwds) 164 165 def subtract(*args, **kwds): 166 '''Like dict.update() but subtracts counts instead of replacing them. 167 Counts can be reduced below zero. Both the inputs and outputs are 168 allowed to contain zero and negative counts. 169 170 Source can be an iterable, a dictionary, or another Counter instance. 171 172 >>> c = Counter('which') 173 >>> c.subtract('witch') # subtract elements from another iterable 174 >>> c.subtract(Counter('watch')) # subtract elements from another counter 175 >>> c['h'] # 2 in which, minus 1 in witch, minus 1 in watch 176 0 177 >>> c['w'] # 1 in which, minus 1 in witch, minus 1 in watch 178 -1 179 180 ''' 181 if not args: 182 raise TypeError("descriptor 'subtract' of 'Counter' object " 183 "needs an argument") 184 self = args[0] 185 args = args[1:] 186 if len(args) > 1: 187 raise TypeError('expected at most 1 arguments, got %d' % len(args)) 188 iterable = args[0] if args else None 189 if iterable is not None: 190 self_get = self.get 191 if isinstance(iterable, Mapping): 192 for elem, count in iterable.items(): 193 self[elem] = self_get(elem, 0) - count 194 else: 195 for elem in iterable: 196 self[elem] = self_get(elem, 0) - 1 197 if kwds: 198 self.subtract(kwds) 199 200 def copy(self): 201 'Return a shallow copy.' 202 return self.__class__(self) 203 204 def __reduce__(self): 205 return self.__class__, (dict(self),) 206 207 def __delitem__(self, elem): 208 'Like dict.__delitem__() but does not raise KeyError for missing values.' 209 if elem in self: 210 super(Counter, self).__delitem__(elem) 211 212 def __repr__(self): 213 if not self: 214 return '%s()' % self.__class__.__name__ 215 items = ', '.join(map('%r: %r'.__mod__, self.most_common())) 216 return '%s({%s})' % (self.__class__.__name__, items) 217 218 # Multiset-style mathematical operations discussed in: 219 # Knuth TAOCP Volume II section 4.6.3 exercise 19 220 # and at http://en.wikipedia.org/wiki/Multiset 221 # 222 # Outputs guaranteed to only include positive counts. 223 # 224 # To strip negative and zero counts, add-in an empty counter: 225 # c += Counter() 226 227 def __add__(self, other): 228 '''Add counts from two counters. 229 230 >>> Counter('abbb') + Counter('bcc') 231 Counter({'b': 4, 'c': 2, 'a': 1}) 232 233 ''' 234 if not isinstance(other, Counter): 235 return NotImplemented 236 result = Counter() 237 for elem, count in self.items(): 238 newcount = count + other[elem] 239 if newcount > 0: 240 result[elem] = newcount 241 for elem, count in other.items(): 242 if elem not in self and count > 0: 243 result[elem] = count 244 return result 245 246 def __sub__(self, other): 247 ''' Subtract count, but keep only results with positive counts. 248 249 >>> Counter('abbbc') - Counter('bccd') 250 Counter({'b': 2, 'a': 1}) 251 252 ''' 253 if not isinstance(other, Counter): 254 return NotImplemented 255 result = Counter() 256 for elem, count in self.items(): 257 newcount = count - other[elem] 258 if newcount > 0: 259 result[elem] = newcount 260 for elem, count in other.items(): 261 if elem not in self and count < 0: 262 result[elem] = 0 - count 263 return result 264 265 def __or__(self, other): 266 '''Union is the maximum of value in either of the input counters. 267 268 >>> Counter('abbb') | Counter('bcc') 269 Counter({'b': 3, 'c': 2, 'a': 1}) 270 271 ''' 272 if not isinstance(other, Counter): 273 return NotImplemented 274 result = Counter() 275 for elem, count in self.items(): 276 other_count = other[elem] 277 newcount = other_count if count < other_count else count 278 if newcount > 0: 279 result[elem] = newcount 280 for elem, count in other.items(): 281 if elem not in self and count > 0: 282 result[elem] = count 283 return result 284 285 def __and__(self, other): 286 ''' Intersection is the minimum of corresponding counts. 287 288 >>> Counter('abbb') & Counter('bcc') 289 Counter({'b': 1}) 290 291 ''' 292 if not isinstance(other, Counter): 293 return NotImplemented 294 result = Counter() 295 for elem, count in self.items(): 296 other_count = other[elem] 297 newcount = count if count < other_count else other_count 298 if newcount > 0: 299 result[elem] = newcount 300 return result 301 302 303 if __name__ == '__main__': 304 # verify that instances can be pickled 305 from cPickle import loads, dumps 306 Point = namedtuple('Point', 'x, y', True) 307 p = Point(x=10, y=20) 308 assert p == loads(dumps(p)) 309 310 # test and demonstrate ability to override methods 311 class Point(namedtuple('Point', 'x y')): 312 __slots__ = () 313 @property 314 def hypot(self): 315 return (self.x ** 2 + self.y ** 2) ** 0.5 316 def __str__(self): 317 return 'Point: x=%6.3f y=%6.3f hypot=%6.3f' % (self.x, self.y, self.hypot) 318 319 for p in Point(3, 4), Point(14, 5/7.): 320 print p 321 322 class Point(namedtuple('Point', 'x y')): 323 'Point class with optimized _make() and _replace() without error-checking' 324 __slots__ = () 325 _make = classmethod(tuple.__new__) 326 def _replace(self, _map=map, **kwds): 327 return self._make(_map(kwds.get, ('x', 'y'), self)) 328 329 print Point(11, 22)._replace(x=100) 330 331 Point3D = namedtuple('Point3D', Point._fields + ('z',)) 332 print Point3D.__doc__ 333 334 import doctest 335 TestResults = namedtuple('TestResults', 'failed attempted') 336 print TestResults(*doctest.testmod())
常用方法如下:
1,计数器

1 >>> import collections 2 >>> c1 = collections.Counter('aabbccddww') 3 >>> c1 4 Counter({'a': 2, 'c': 2, 'b': 2, 'd': 2, 'w': 2}) 5 >>> c1.most_common(3) 取出前三个 6 [('a', 2), ('c', 2), ('b', 2)] 7 >>> c2 = collections.Counter('aabbttyy') 8 >>> c2 9 Counter({'a': 2, 'y': 2, 'b': 2, 't': 2}) 10 >>> c1.update(c2) 合并,c2的元素合并到了c1 11 >>> c1 12 Counter({'a': 4, 'b': 4, 'c': 2, 'd': 2, 't': 2, 'w': 2, 'y': 13 14 2}) 15 >>> c1['a'] 取出计数器中的元素,没有返回0 16 4 17 >>> c1['g'] 18 0 19 >>> c2.clear() 清除 20 >>> c1.elements() 返回一个迭代器 21 <itertools.chain object at 0x015A63F0> 22 >>> for item in c1.elements(): 23 ... print item 24 ... 25 a 26 a 27 a 28 a 29 c 30 c 31 b 32 b 33 b 34 b 35 d 36 d 37 t 38 t 39 w 40 w 41 y 42 y
2 有序字典
方法如下

1 class OrderedDict(dict): 2 'Dictionary that remembers insertion order' 3 # An inherited dict maps keys to values. 4 # The inherited dict provides __getitem__, __len__, __contains__, and get. 5 # The remaining methods are order-aware. 6 # Big-O running times for all methods are the same as regular dictionaries. 7 8 # The internal self.__map dict maps keys to links in a doubly linked list. 9 # The circular doubly linked list starts and ends with a sentinel element. 10 # The sentinel element never gets deleted (this simplifies the algorithm). 11 # Each link is stored as a list of length three: [PREV, NEXT, KEY]. 12 13 def __init__(*args, **kwds): 14 '''Initialize an ordered dictionary. The signature is the same as 15 regular dictionaries, but keyword arguments are not recommended because 16 their insertion order is arbitrary. 17 18 ''' 19 if not args: 20 raise TypeError("descriptor '__init__' of 'OrderedDict' object " 21 "needs an argument") 22 self = args[0] 23 args = args[1:] 24 if len(args) > 1: 25 raise TypeError('expected at most 1 arguments, got %d' % len(args)) 26 try: 27 self.__root 28 except AttributeError: 29 self.__root = root = [] # sentinel node 30 root[:] = [root, root, None] 31 self.__map = {} 32 self.__update(*args, **kwds) 33 34 def __setitem__(self, key, value, dict_setitem=dict.__setitem__): 35 'od.__setitem__(i, y) <==> od[i]=y' 36 # Setting a new item creates a new link at the end of the linked list, 37 # and the inherited dictionary is updated with the new key/value pair. 38 if key not in self: 39 root = self.__root 40 last = root[0] 41 last[1] = root[0] = self.__map[key] = [last, root, key] 42 return dict_setitem(self, key, value) 43 44 def __delitem__(self, key, dict_delitem=dict.__delitem__): 45 'od.__delitem__(y) <==> del od[y]' 46 # Deleting an existing item uses self.__map to find the link which gets 47 # removed by updating the links in the predecessor and successor nodes. 48 dict_delitem(self, key) 49 link_prev, link_next, _ = self.__map.pop(key) 50 link_prev[1] = link_next # update link_prev[NEXT] 51 link_next[0] = link_prev # update link_next[PREV] 52 53 def __iter__(self): 54 'od.__iter__() <==> iter(od)' 55 # Traverse the linked list in order. 56 root = self.__root 57 curr = root[1] # start at the first node 58 while curr is not root: 59 yield curr[2] # yield the curr[KEY] 60 curr = curr[1] # move to next node 61 62 def __reversed__(self): 63 'od.__reversed__() <==> reversed(od)' 64 # Traverse the linked list in reverse order. 65 root = self.__root 66 curr = root[0] # start at the last node 67 while curr is not root: 68 yield curr[2] # yield the curr[KEY] 69 curr = curr[0] # move to previous node 70 71 def clear(self): 72 'od.clear() -> None. Remove all items from od.' 73 root = self.__root 74 root[:] = [root, root, None] 75 self.__map.clear() 76 dict.clear(self) 77 78 # -- the following methods do not depend on the internal structure -- 79 80 def keys(self): 81 'od.keys() -> list of keys in od' 82 return list(self) 83 84 def values(self): 85 'od.values() -> list of values in od' 86 return [self[key] for key in self] 87 88 def items(self): 89 'od.items() -> list of (key, value) pairs in od' 90 return [(key, self[key]) for key in self] 91 92 def iterkeys(self): 93 'od.iterkeys() -> an iterator over the keys in od' 94 return iter(self) 95 96 def itervalues(self): 97 'od.itervalues -> an iterator over the values in od' 98 for k in self: 99 yield self[k] 100 101 def iteritems(self): 102 'od.iteritems -> an iterator over the (key, value) pairs in od' 103 for k in self: 104 yield (k, self[k]) 105 106 update = MutableMapping.update 107 108 __update = update # let subclasses override update without breaking __init__ 109 110 __marker = object() 111 112 def pop(self, key, default=__marker): 113 '''od.pop(k[,d]) -> v, remove specified key and return the corresponding 114 value. If key is not found, d is returned if given, otherwise KeyError 115 is raised. 116 117 ''' 118 if key in self: 119 result = self[key] 120 del self[key] 121 return result 122 if default is self.__marker: 123 raise KeyError(key) 124 return default 125 126 def setdefault(self, key, default=None): 127 'od.setdefault(k[,d]) -> od.get(k,d), also set od[k]=d if k not in od' 128 if key in self: 129 return self[key] 130 self[key] = default 131 return default 132 133 def popitem(self, last=True): 134 '''od.popitem() -> (k, v), return and remove a (key, value) pair. 135 Pairs are returned in LIFO order if last is true or FIFO order if false. 136 137 ''' 138 if not self: 139 raise KeyError('dictionary is empty') 140 key = next(reversed(self) if last else iter(self)) 141 value = self.pop(key) 142 return key, value 143 144 def __repr__(self, _repr_running={}): 145 'od.__repr__() <==> repr(od)' 146 call_key = id(self), _get_ident() 147 if call_key in _repr_running: 148 return '...' 149 _repr_running[call_key] = 1 150 try: 151 if not self: 152 return '%s()' % (self.__class__.__name__,) 153 return '%s(%r)' % (self.__class__.__name__, self.items()) 154 finally: 155 del _repr_running[call_key] 156 157 def __reduce__(self): 158 'Return state information for pickling' 159 items = [[k, self[k]] for k in self] 160 inst_dict = vars(self).copy() 161 for k in vars(OrderedDict()): 162 inst_dict.pop(k, None) 163 if inst_dict: 164 return (self.__class__, (items,), inst_dict) 165 return self.__class__, (items,) 166 167 def copy(self): 168 'od.copy() -> a shallow copy of od' 169 return self.__class__(self) 170 171 @classmethod 172 def fromkeys(cls, iterable, value=None): 173 '''OD.fromkeys(S[, v]) -> New ordered dictionary with keys from S. 174 If not specified, the value defaults to None. 175 176 ''' 177 self = cls() 178 for key in iterable: 179 self[key] = value 180 return self 181 182 def __eq__(self, other): 183 '''od.__eq__(y) <==> od==y. Comparison to another OD is order-sensitive 184 while comparison to a regular mapping is order-insensitive. 185 186 ''' 187 if isinstance(other, OrderedDict): 188 return dict.__eq__(self, other) and all(_imap(_eq, self, other)) 189 return dict.__eq__(self, other) 190 191 def __ne__(self, other): 192 'od.__ne__(y) <==> od!=y' 193 return not self == other 194 195 # -- the following methods support python 3.x style dictionary views -- 196 197 def viewkeys(self): 198 "od.viewkeys() -> a set-like object providing a view on od's keys" 199 return KeysView(self) 200 201 def viewvalues(self): 202 "od.viewvalues() -> an object providing a view on od's values" 203 return ValuesView(self) 204 205 def viewitems(self): 206 "od.viewitems() -> a set-like object providing a view on od's items" 207 return ItemsView(self) 208 209 210 ################################################################################ 211 ### namedtuple 212 ################################################################################ 213 214 _class_template = ''' 215 class {typename}(tuple): 216 '{typename}({arg_list})' 217 218 __slots__ = () 219 220 _fields = {field_names!r} 221 222 def __new__(_cls, {arg_list}): 223 'Create new instance of {typename}({arg_list})' 224 return _tuple.__new__(_cls, ({arg_list})) 225 226 @classmethod 227 def _make(cls, iterable, new=tuple.__new__, len=len): 228 'Make a new {typename} object from a sequence or iterable' 229 result = new(cls, iterable) 230 if len(result) != {num_fields:d}: 231 raise TypeError('Expected {num_fields:d} arguments, got %d' % len(result)) 232 return result 233 234 def __repr__(self): 235 'Return a nicely formatted representation string' 236 return '{typename}({repr_fmt})' % self 237 238 def _asdict(self): 239 'Return a new OrderedDict which maps field names to their values' 240 return OrderedDict(zip(self._fields, self)) 241 242 def _replace(_self, **kwds): 243 'Return a new {typename} object replacing specified fields with new values' 244 result = _self._make(map(kwds.pop, {field_names!r}, _self)) 245 if kwds: 246 raise ValueError('Got unexpected field names: %r' % kwds.keys()) 247 return result 248 249 def __getnewargs__(self): 250 'Return self as a plain tuple. Used by copy and pickle.' 251 return tuple(self) 252 253 __dict__ = _property(_asdict) 254 255 def __getstate__(self): 256 'Exclude the OrderedDict from pickling' 257 pass 258 259 {field_defs} 260 ''' 261 262 _repr_template = '{name}=%r' 263 264 _field_template = ''' 265 {name} = _property(_itemgetter({index:d}), doc='Alias for field number {index:d}') 266 ''' 267 268 def namedtuple(typename, field_names, verbose=False, rename=False): 269 """Returns a new subclass of tuple with named fields. 270 271 >>> Point = namedtuple('Point', ['x', 'y']) 272 >>> Point.__doc__ # docstring for the new class 273 'Point(x, y)' 274 >>> p = Point(11, y=22) # instantiate with positional args or keywords 275 >>> p[0] + p[1] # indexable like a plain tuple 276 33 277 >>> x, y = p # unpack like a regular tuple 278 >>> x, y 279 (11, 22) 280 >>> p.x + p.y # fields also accessable by name 281 33 282 >>> d = p._asdict() # convert to a dictionary 283 >>> d['x'] 284 11 285 >>> Point(**d) # convert from a dictionary 286 Point(x=11, y=22) 287 >>> p._replace(x=100) # _replace() is like str.replace() but targets named fields 288 Point(x=100, y=22) 289 290 """ 291 292 # Validate the field names. At the user's option, either generate an error 293 # message or automatically replace the field name with a valid name. 294 if isinstance(field_names, basestring): 295 field_names = field_names.replace(',', ' ').split() 296 field_names = map(str, field_names) 297 typename = str(typename) 298 if rename: 299 seen = set() 300 for index, name in enumerate(field_names): 301 if (not all(c.isalnum() or c=='_' for c in name) 302 or _iskeyword(name) 303 or not name 304 or name[0].isdigit() 305 or name.startswith('_') 306 or name in seen): 307 field_names[index] = '_%d' % index 308 seen.add(name) 309 for name in [typename] + field_names: 310 if type(name) != str: 311 raise TypeError('Type names and field names must be strings') 312 if not all(c.isalnum() or c=='_' for c in name): 313 raise ValueError('Type names and field names can only contain ' 314 'alphanumeric characters and underscores: %r' % name) 315 if _iskeyword(name): 316 raise ValueError('Type names and field names cannot be a ' 317 'keyword: %r' % name) 318 if name[0].isdigit(): 319 raise ValueError('Type names and field names cannot start with ' 320 'a number: %r' % name) 321 seen = set() 322 for name in field_names: 323 if name.startswith('_') and not rename: 324 raise ValueError('Field names cannot start with an underscore: ' 325 '%r' % name) 326 if name in seen: 327 raise ValueError('Encountered duplicate field name: %r' % name) 328 seen.add(name) 329 330 # Fill-in the class template 331 class_definition = _class_template.format( 332 typename = typename, 333 field_names = tuple(field_names), 334 num_fields = len(field_names), 335 arg_list = repr(tuple(field_names)).replace("'", "")[1:-1], 336 repr_fmt = ', '.join(_repr_template.format(name=name) 337 for name in field_names), 338 field_defs = ' '.join(_field_template.format(index=index, name=name) 339 for index, name in enumerate(field_names)) 340 ) 341 if verbose: 342 print class_definition 343 344 # Execute the template string in a temporary namespace and support 345 # tracing utilities by setting a value for frame.f_globals['__name__'] 346 namespace = dict(_itemgetter=_itemgetter, __name__='namedtuple_%s' % typename, 347 OrderedDict=OrderedDict, _property=property, _tuple=tuple) 348 try: 349 exec class_definition in namespace 350 except SyntaxError as e: 351 raise SyntaxError(e.message + ': ' + class_definition) 352 result = namespace[typename] 353 354 # For pickling to work, the __module__ variable needs to be set to the frame 355 # where the named tuple is created. Bypass this step in environments where 356 # sys._getframe is not defined (Jython for example) or sys._getframe is not 357 # defined for arguments greater than 0 (IronPython). 358 try: 359 result.__module__ = _sys._getframe(1).f_globals.get('__name__', '__main__') 360 except (AttributeError, ValueError): 361 pass 362 363 return result 364 365 366 ######################################################################## 367 ### Counter 368 ######################################################################## 369 370 class Counter(dict): 371 '''Dict subclass for counting hashable items. Sometimes called a bag 372 or multiset. Elements are stored as dictionary keys and their counts 373 are stored as dictionary values. 374 375 >>> c = Counter('abcdeabcdabcaba') # count elements from a string 376 377 >>> c.most_common(3) # three most common elements 378 [('a', 5), ('b', 4), ('c', 3)] 379 >>> sorted(c) # list all unique elements 380 ['a', 'b', 'c', 'd', 'e'] 381 >>> ''.join(sorted(c.elements())) # list elements with repetitions 382 'aaaaabbbbcccdde' 383 >>> sum(c.values()) # total of all counts 384 15 385 386 >>> c['a'] # count of letter 'a' 387 5 388 >>> for elem in 'shazam': # update counts from an iterable 389 ... c[elem] += 1 # by adding 1 to each element's count 390 >>> c['a'] # now there are seven 'a' 391 7 392 >>> del c['b'] # remove all 'b' 393 >>> c['b'] # now there are zero 'b' 394 0 395 396 >>> d = Counter('simsalabim') # make another counter 397 >>> c.update(d) # add in the second counter 398 >>> c['a'] # now there are nine 'a' 399 9 400 401 >>> c.clear() # empty the counter 402 >>> c 403 Counter() 404 405 Note: If a count is set to zero or reduced to zero, it will remain 406 in the counter until the entry is deleted or the counter is cleared: 407 408 >>> c = Counter('aaabbc') 409 >>> c['b'] -= 2 # reduce the count of 'b' by two 410 >>> c.most_common() # 'b' is still in, but its count is zero 411 [('a', 3), ('c', 1), ('b', 0)] 412 413 ''' 414 # References: 415 # http://en.wikipedia.org/wiki/Multiset 416 # http://www.gnu.org/software/smalltalk/manual-base/html_node/Bag.html 417 # http://www.demo2s.com/Tutorial/Cpp/0380__set-multiset/Catalog0380__set-multiset.htm 418 # http://code.activestate.com/recipes/259174/ 419 # Knuth, TAOCP Vol. II section 4.6.3 420 421 def __init__(*args, **kwds): 422 '''Create a new, empty Counter object. And if given, count elements 423 from an input iterable. Or, initialize the count from another mapping 424 of elements to their counts. 425 426 >>> c = Counter() # a new, empty counter 427 >>> c = Counter('gallahad') # a new counter from an iterable 428 >>> c = Counter({'a': 4, 'b': 2}) # a new counter from a mapping 429 >>> c = Counter(a=4, b=2) # a new counter from keyword args 430 431 ''' 432 if not args: 433 raise TypeError("descriptor '__init__' of 'Counter' object " 434 "needs an argument") 435 self = args[0] 436 args = args[1:] 437 if len(args) > 1: 438 raise TypeError('expected at most 1 arguments, got %d' % len(args)) 439 super(Counter, self).__init__() 440 self.update(*args, **kwds) 441 442 def __missing__(self, key): 443 'The count of elements not in the Counter is zero.' 444 # Needed so that self[missing_item] does not raise KeyError 445 return 0 446 447 def most_common(self, n=None): 448 '''List the n most common elements and their counts from the most 449 common to the least. If n is None, then list all element counts. 450 451 >>> Counter('abcdeabcdabcaba').most_common(3) 452 [('a', 5), ('b', 4), ('c', 3)] 453 454 ''' 455 # Emulate Bag.sortedByCount from Smalltalk 456 if n is None: 457 return sorted(self.iteritems(), key=_itemgetter(1), reverse=True) 458 return _heapq.nlargest(n, self.iteritems(), key=_itemgetter(1)) 459 460 def elements(self): 461 '''Iterator over elements repeating each as many times as its count. 462 463 >>> c = Counter('ABCABC') 464 >>> sorted(c.elements()) 465 ['A', 'A', 'B', 'B', 'C', 'C'] 466 467 # Knuth's example for prime factors of 1836: 2**2 * 3**3 * 17**1 468 >>> prime_factors = Counter({2: 2, 3: 3, 17: 1}) 469 >>> product = 1 470 >>> for factor in prime_factors.elements(): # loop over factors 471 ... product *= factor # and multiply them 472 >>> product 473 1836 474 475 Note, if an element's count has been set to zero or is a negative 476 number, elements() will ignore it. 477 478 ''' 479 # Emulate Bag.do from Smalltalk and Multiset.begin from C++. 480 return _chain.from_iterable(_starmap(_repeat, self.iteritems())) 481 482 # Override dict methods where necessary 483 484 @classmethod 485 def fromkeys(cls, iterable, v=None): 486 # There is no equivalent method for counters because setting v=1 487 # means that no element can have a count greater than one. 488 raise NotImplementedError( 489 'Counter.fromkeys() is undefined. Use Counter(iterable) instead.') 490 491 def update(*args, **kwds): 492 '''Like dict.update() but add counts instead of replacing them. 493 494 Source can be an iterable, a dictionary, or another Counter instance. 495 496 >>> c = Counter('which') 497 >>> c.update('witch') # add elements from another iterable 498 >>> d = Counter('watch') 499 >>> c.update(d) # add elements from another counter 500 >>> c['h'] # four 'h' in which, witch, and watch 501 4 502 503 ''' 504 # The regular dict.update() operation makes no sense here because the 505 # replace behavior results in the some of original untouched counts 506 # being mixed-in with all of the other counts for a mismash that 507 # doesn't have a straight-forward interpretation in most counting 508 # contexts. Instead, we implement straight-addition. Both the inputs 509 # and outputs are allowed to contain zero and negative counts. 510 511 if not args: 512 raise TypeError("descriptor 'update' of 'Counter' object " 513 "needs an argument") 514 self = args[0] 515 args = args[1:] 516 if len(args) > 1: 517 raise TypeError('expected at most 1 arguments, got %d' % len(args)) 518 iterable = args[0] if args else None 519 if iterable is not None: 520 if isinstance(iterable, Mapping): 521 if self: 522 self_get = self.get 523 for elem, count in iterable.iteritems(): 524 self[elem] = self_get(elem, 0) + count 525 else: 526 super(Counter, self).update(iterable) # fast path when counter is empty 527 else: 528 self_get = self.get 529 for elem in iterable: 530 self[elem] = self_get(elem, 0) + 1 531 if kwds: 532 self.update(kwds) 533 534 def subtract(*args, **kwds): 535 '''Like dict.update() but subtracts counts instead of replacing them. 536 Counts can be reduced below zero. Both the inputs and outputs are 537 allowed to contain zero and negative counts. 538 539 Source can be an iterable, a dictionary, or another Counter instance. 540 541 >>> c = Counter('which') 542 >>> c.subtract('witch') # subtract elements from another iterable 543 >>> c.subtract(Counter('watch')) # subtract elements from another counter 544 >>> c['h'] # 2 in which, minus 1 in witch, minus 1 in watch 545 0 546 >>> c['w'] # 1 in which, minus 1 in witch, minus 1 in watch 547 -1 548 549 ''' 550 if not args: 551 raise TypeError("descriptor 'subtract' of 'Counter' object " 552 "needs an argument") 553 self = args[0] 554 args = args[1:] 555 if len(args) > 1: 556 raise TypeError('expected at most 1 arguments, got %d' % len(args)) 557 iterable = args[0] if args else None 558 if iterable is not None: 559 self_get = self.get 560 if isinstance(iterable, Mapping): 561 for elem, count in iterable.items(): 562 self[elem] = self_get(elem, 0) - count 563 else: 564 for elem in iterable: 565 self[elem] = self_get(elem, 0) - 1 566 if kwds: 567 self.subtract(kwds) 568 569 def copy(self): 570 'Return a shallow copy.' 571 return self.__class__(self) 572 573 def __reduce__(self): 574 return self.__class__, (dict(self),) 575 576 def __delitem__(self, elem): 577 'Like dict.__delitem__() but does not raise KeyError for missing values.' 578 if elem in self: 579 super(Counter, self).__delitem__(elem) 580 581 def __repr__(self): 582 if not self: 583 return '%s()' % self.__class__.__name__ 584 items = ', '.join(map('%r: %r'.__mod__, self.most_common())) 585 return '%s({%s})' % (self.__class__.__name__, items) 586 587 # Multiset-style mathematical operations discussed in: 588 # Knuth TAOCP Volume II section 4.6.3 exercise 19 589 # and at http://en.wikipedia.org/wiki/Multiset 590 # 591 # Outputs guaranteed to only include positive counts. 592 # 593 # To strip negative and zero counts, add-in an empty counter: 594 # c += Counter() 595 596 def __add__(self, other): 597 '''Add counts from two counters. 598 599 >>> Counter('abbb') + Counter('bcc') 600 Counter({'b': 4, 'c': 2, 'a': 1}) 601 602 ''' 603 if not isinstance(other, Counter): 604 return NotImplemented 605 result = Counter() 606 for elem, count in self.items(): 607 newcount = count + other[elem] 608 if newcount > 0: 609 result[elem] = newcount 610 for elem, count in other.items(): 611 if elem not in self and count > 0: 612 result[elem] = count 613 return result 614 615 def __sub__(self, other): 616 ''' Subtract count, but keep only results with positive counts. 617 618 >>> Counter('abbbc') - Counter('bccd') 619 Counter({'b': 2, 'a': 1}) 620 621 ''' 622 if not isinstance(other, Counter): 623 return NotImplemented 624 result = Counter() 625 for elem, count in self.items(): 626 newcount = count - other[elem] 627 if newcount > 0: 628 result[elem] = newcount 629 for elem, count in other.items(): 630 if elem not in self and count < 0: 631 result[elem] = 0 - count 632 return result 633 634 def __or__(self, other): 635 '''Union is the maximum of value in either of the input counters. 636 637 >>> Counter('abbb') | Counter('bcc') 638 Counter({'b': 3, 'c': 2, 'a': 1}) 639 640 ''' 641 if not isinstance(other, Counter): 642 return NotImplemented 643 result = Counter() 644 for elem, count in self.items(): 645 other_count = other[elem] 646 newcount = other_count if count < other_count else count 647 if newcount > 0: 648 result[elem] = newcount 649 for elem, count in other.items(): 650 if elem not in self and count > 0: 651 result[elem] = count 652 return result 653 654 def __and__(self, other): 655 ''' Intersection is the minimum of corresponding counts. 656 657 >>> Counter('abbb') & Counter('bcc') 658 Counter({'b': 1}) 659 660 ''' 661 if not isinstance(other, Counter): 662 return NotImplemented 663 result = Counter() 664 for elem, count in self.items(): 665 other_count = other[elem] 666 newcount = count if count < other_count else other_count 667 if newcount > 0: 668 result[elem] = newcount 669 return result 670 671 672 if __name__ == '__main__': 673 # verify that instances can be pickled 674 from cPickle import loads, dumps 675 Point = namedtuple('Point', 'x, y', True) 676 p = Point(x=10, y=20) 677 assert p == loads(dumps(p)) 678 679 # test and demonstrate ability to override methods 680 class Point(namedtuple('Point', 'x y')): 681 __slots__ = () 682 @property 683 def hypot(self): 684 return (self.x ** 2 + self.y ** 2) ** 0.5 685 def __str__(self): 686 return 'Point: x=%6.3f y=%6.3f hypot=%6.3f' % (self.x, self.y, self.hypot) 687 688 for p in Point(3, 4), Point(14, 5/7.): 689 print p 690 691 class Point(namedtuple('Point', 'x y')): 692 'Point class with optimized _make() and _replace() without error-checking' 693 __slots__ = () 694 _make = classmethod(tuple.__new__) 695 def _replace(self, _map=map, **kwds): 696 return self._make(_map(kwds.get, ('x', 'y'), self)) 697 698 print Point(11, 22)._replace(x=100) 699 700 Point3D = namedtuple('Point3D', Point._fields + ('z',)) 701 print Point3D.__doc__ 702 703 import doctest 704 TestResults = namedtuple('TestResults', 'failed attempted') 705 print TestResults(*doctest.testmod())
普通字典是无序的,这里是介绍有序字典,具体方法如下

1 >>> import collections 2 >>> c1 = collections.OrderedDict() 3 >>> c1['k1'] = 1 4 >>> c1['k2'] = 2 5 >>> c1['k3'] = 3 6 >>> c1 7 OrderedDict([('k1', 1), ('k2', 2), ('k3', 3)]) 有序 8 >>> c2 = {} 9 >>> c2['k1'] = 1 10 >>> c2['k2'] = 2 11 >>> c2['k3'] = 3 12 >>> c2 13 {'k3': 3, 'k2': 2, 'k1': 1} 无序
3默认字典
defaultdict

1 class defaultdict(dict): 2 """ 3 defaultdict(default_factory[, ...]) --> dict with default factory 4 5 The default factory is called without arguments to produce 6 a new value when a key is not present, in __getitem__ only. 7 A defaultdict compares equal to a dict with the same items. 8 All remaining arguments are treated the same as if they were 9 passed to the dict constructor, including keyword arguments. 10 """ 11 def copy(self): # real signature unknown; restored from __doc__ 12 """ D.copy() -> a shallow copy of D. """ 13 pass 14 15 def __copy__(self, *args, **kwargs): # real signature unknown 16 """ D.copy() -> a shallow copy of D. """ 17 pass 18 19 def __getattribute__(self, name): # real signature unknown; restored from __doc__ 20 """ x.__getattribute__('name') <==> x.name """ 21 pass 22 23 def __init__(self, default_factory=None, **kwargs): # known case of _collections.defaultdict.__init__ 24 """ 25 defaultdict(default_factory[, ...]) --> dict with default factory 26 27 The default factory is called without arguments to produce 28 a new value when a key is not present, in __getitem__ only. 29 A defaultdict compares equal to a dict with the same items. 30 All remaining arguments are treated the same as if they were 31 passed to the dict constructor, including keyword arguments. 32 33 # (copied from class doc) 34 """ 35 pass 36 37 def __missing__(self, key): # real signature unknown; restored from __doc__ 38 """ 39 __missing__(key) # Called by __getitem__ for missing key; pseudo-code: 40 if self.default_factory is None: raise KeyError((key,)) 41 self[key] = value = self.default_factory() 42 return value 43 """ 44 pass 45 46 def __reduce__(self, *args, **kwargs): # real signature unknown 47 """ Return state information for pickling. """ 48 pass 49 50 def __repr__(self): # real signature unknown; restored from __doc__ 51 """ x.__repr__() <==> repr(x) """ 52 pass 53 54 default_factory = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 55 """Factory for default value called by __missing__().""" 56 57 58 59 class deque(object): 60 """ 61 deque([iterable[, maxlen]]) --> deque object 62 63 Build an ordered collection with optimized access from its endpoints. 64 """ 65 def append(self, *args, **kwargs): # real signature unknown 66 """ Add an element to the right side of the deque. """ 67 pass 68 69 def appendleft(self, *args, **kwargs): # real signature unknown 70 """ Add an element to the left side of the deque. """ 71 pass 72 73 def clear(self, *args, **kwargs): # real signature unknown 74 """ Remove all elements from the deque. """ 75 pass 76 77 def count(self, value): # real signature unknown; restored from __doc__ 78 """ D.count(value) -> integer -- return number of occurrences of value """ 79 return 0 80 81 def extend(self, *args, **kwargs): # real signature unknown 82 """ Extend the right side of the deque with elements from the iterable """ 83 pass 84 85 def extendleft(self, *args, **kwargs): # real signature unknown 86 """ Extend the left side of the deque with elements from the iterable """ 87 pass 88 89 def pop(self, *args, **kwargs): # real signature unknown 90 """ Remove and return the rightmost element. """ 91 pass 92 93 def popleft(self, *args, **kwargs): # real signature unknown 94 """ Remove and return the leftmost element. """ 95 pass 96 97 def remove(self, value): # real signature unknown; restored from __doc__ 98 """ D.remove(value) -- remove first occurrence of value. """ 99 pass 100 101 def reverse(self): # real signature unknown; restored from __doc__ 102 """ D.reverse() -- reverse *IN PLACE* """ 103 pass 104 105 def rotate(self, *args, **kwargs): # real signature unknown 106 """ Rotate the deque n steps to the right (default n=1). If n is negative, rotates left. """ 107 pass 108 109 def __copy__(self, *args, **kwargs): # real signature unknown 110 """ Return a shallow copy of a deque. """ 111 pass 112 113 def __delitem__(self, y): # real signature unknown; restored from __doc__ 114 """ x.__delitem__(y) <==> del x[y] """ 115 pass 116 117 def __eq__(self, y): # real signature unknown; restored from __doc__ 118 """ x.__eq__(y) <==> x==y """ 119 pass 120 121 def __getattribute__(self, name): # real signature unknown; restored from __doc__ 122 """ x.__getattribute__('name') <==> x.name """ 123 pass 124 125 def __getitem__(self, y): # real signature unknown; restored from __doc__ 126 """ x.__getitem__(y) <==> x[y] """ 127 pass 128 129 def __ge__(self, y): # real signature unknown; restored from __doc__ 130 """ x.__ge__(y) <==> x>=y """ 131 pass 132 133 def __gt__(self, y): # real signature unknown; restored from __doc__ 134 """ x.__gt__(y) <==> x>y """ 135 pass 136 137 def __iadd__(self, y): # real signature unknown; restored from __doc__ 138 """ x.__iadd__(y) <==> x+=y """ 139 pass 140 141 def __init__(self, iterable=(), maxlen=None): # known case of _collections.deque.__init__ 142 """ 143 deque([iterable[, maxlen]]) --> deque object 144 145 Build an ordered collection with optimized access from its endpoints. 146 # (copied from class doc) 147 """ 148 pass 149 150 def __iter__(self): # real signature unknown; restored from __doc__ 151 """ x.__iter__() <==> iter(x) """ 152 pass 153 154 def __len__(self): # real signature unknown; restored from __doc__ 155 """ x.__len__() <==> len(x) """ 156 pass 157 158 def __le__(self, y): # real signature unknown; restored from __doc__ 159 """ x.__le__(y) <==> x<=y """ 160 pass 161 162 def __lt__(self, y): # real signature unknown; restored from __doc__ 163 """ x.__lt__(y) <==> x<y """ 164 pass 165 166 @staticmethod # known case of __new__ 167 def __new__(S, *more): # real signature unknown; restored from __doc__ 168 """ T.__new__(S, ...) -> a new object with type S, a subtype of T """ 169 pass 170 171 def __ne__(self, y): # real signature unknown; restored from __doc__ 172 """ x.__ne__(y) <==> x!=y """ 173 pass 174 175 def __reduce__(self, *args, **kwargs): # real signature unknown 176 """ Return state information for pickling. """ 177 pass 178 179 def __repr__(self): # real signature unknown; restored from __doc__ 180 """ x.__repr__() <==> repr(x) """ 181 pass 182 183 def __reversed__(self): # real signature unknown; restored from __doc__ 184 """ D.__reversed__() -- return a reverse iterator over the deque """ 185 pass 186 187 def __setitem__(self, i, y): # real signature unknown; restored from __doc__ 188 """ x.__setitem__(i, y) <==> x[i]=y """ 189 pass 190 191 def __sizeof__(self): # real signature unknown; restored from __doc__ 192 """ D.__sizeof__() -- size of D in memory, in bytes """ 193 pass 194 195 maxlen = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 196 """maximum size of a deque or None if unbounded""" 197 198 199 __hash__ = None
默认字典与原生字典比较实例

1 import collections 2 >>> collections.defaultdict(list) 括号内加上list,会在创建字典的时候默认value是列表 3 defaultdict(<type 'list'>, {}) 4 >>> dic = collections.defaultdict(list) 5 >>> dic 6 defaultdict(<type 'list'>, {}) 7 >>> dic['k1'].append(1) 8 >>> dic 9 defaultdict(<type 'list'>, {'k1': [1]}) 10 >>> dic1={} 11 >>> dic1['k1'].append(1) 12 Traceback (most recent call last): 13 File "<stdin>", line 1, in <module> 14 KeyError: 'k1' 15 >>> dic1['k1']=[] 必须多一步指定value为列表 16 >>> dic1['k1'].append(1) 17 >>> dic1 18 {'k1': [1]} 19 >>>
4可命名元组namedtuple

1 def namedtuple(typename, field_names, verbose=False, rename=False): 2 """Returns a new subclass of tuple with named fields. 3 4 >>> Point = namedtuple('Point', ['x', 'y']) 5 >>> Point.__doc__ # docstring for the new class 6 'Point(x, y)' 7 >>> p = Point(11, y=22) # instantiate with positional args or keywords 8 >>> p[0] + p[1] # indexable like a plain tuple 9 33 10 >>> x, y = p # unpack like a regular tuple 11 >>> x, y 12 (11, 22) 13 >>> p.x + p.y # fields also accessable by name 14 33 15 >>> d = p._asdict() # convert to a dictionary 16 >>> d['x'] 17 11 18 >>> Point(**d) # convert from a dictionary 19 Point(x=11, y=22) 20 >>> p._replace(x=100) # _replace() is like str.replace() but targets named fields 21 Point(x=100, y=22) 22 23 """ 24 25 # Validate the field names. At the user's option, either generate an error 26 # message or automatically replace the field name with a valid name. 27 if isinstance(field_names, basestring): 28 field_names = field_names.replace(',', ' ').split() 29 field_names = map(str, field_names) 30 typename = str(typename) 31 if rename: 32 seen = set() 33 for index, name in enumerate(field_names): 34 if (not all(c.isalnum() or c=='_' for c in name) 35 or _iskeyword(name) 36 or not name 37 or name[0].isdigit() 38 or name.startswith('_') 39 or name in seen): 40 field_names[index] = '_%d' % index 41 seen.add(name) 42 for name in [typename] + field_names: 43 if type(name) != str: 44 raise TypeError('Type names and field names must be strings') 45 if not all(c.isalnum() or c=='_' for c in name): 46 raise ValueError('Type names and field names can only contain ' 47 'alphanumeric characters and underscores: %r' % name) 48 if _iskeyword(name): 49 raise ValueError('Type names and field names cannot be a ' 50 'keyword: %r' % name) 51 if name[0].isdigit(): 52 raise ValueError('Type names and field names cannot start with ' 53 'a number: %r' % name) 54 seen = set() 55 for name in field_names: 56 if name.startswith('_') and not rename: 57 raise ValueError('Field names cannot start with an underscore: ' 58 '%r' % name) 59 if name in seen: 60 raise ValueError('Encountered duplicate field name: %r' % name) 61 seen.add(name) 62 63 # Fill-in the class template 64 class_definition = _class_template.format( 65 typename = typename, 66 field_names = tuple(field_names), 67 num_fields = len(field_names), 68 arg_list = repr(tuple(field_names)).replace("'", "")[1:-1], 69 repr_fmt = ', '.join(_repr_template.format(name=name) 70 for name in field_names), 71 field_defs = ' '.join(_field_template.format(index=index, name=name) 72 for index, name in enumerate(field_names)) 73 ) 74 if verbose: 75 print class_definition 76 77 # Execute the template string in a temporary namespace and support 78 # tracing utilities by setting a value for frame.f_globals['__name__'] 79 namespace = dict(_itemgetter=_itemgetter, __name__='namedtuple_%s' % typename, 80 OrderedDict=OrderedDict, _property=property, _tuple=tuple) 81 try: 82 exec class_definition in namespace 83 except SyntaxError as e: 84 raise SyntaxError(e.message + ': ' + class_definition) 85 result = namespace[typename] 86 87 # For pickling to work, the __module__ variable needs to be set to the frame 88 # where the named tuple is created. Bypass this step in environments where 89 # sys._getframe is not defined (Jython for example) or sys._getframe is not 90 # defined for arguments greater than 0 (IronPython). 91 try: 92 result.__module__ = _sys._getframe(1).f_globals.get('__name__', '__main__') 93 except (AttributeError, ValueError): 94 pass 95 96 return result 97 98 99 ######################################################################## 100 ### Counter 101 ######################################################################## 102 103 class Counter(dict): 104 '''Dict subclass for counting hashable items. Sometimes called a bag 105 or multiset. Elements are stored as dictionary keys and their counts 106 are stored as dictionary values. 107 108 >>> c = Counter('abcdeabcdabcaba') # count elements from a string 109 110 >>> c.most_common(3) # three most common elements 111 [('a', 5), ('b', 4), ('c', 3)] 112 >>> sorted(c) # list all unique elements 113 ['a', 'b', 'c', 'd', 'e'] 114 >>> ''.join(sorted(c.elements())) # list elements with repetitions 115 'aaaaabbbbcccdde' 116 >>> sum(c.values()) # total of all counts 117 15 118 119 >>> c['a'] # count of letter 'a' 120 5 121 >>> for elem in 'shazam': # update counts from an iterable 122 ... c[elem] += 1 # by adding 1 to each element's count 123 >>> c['a'] # now there are seven 'a' 124 7 125 >>> del c['b'] # remove all 'b' 126 >>> c['b'] # now there are zero 'b' 127 0 128 129 >>> d = Counter('simsalabim') # make another counter 130 >>> c.update(d) # add in the second counter 131 >>> c['a'] # now there are nine 'a' 132 9 133 134 >>> c.clear() # empty the counter 135 >>> c 136 Counter() 137 138 Note: If a count is set to zero or reduced to zero, it will remain 139 in the counter until the entry is deleted or the counter is cleared: 140 141 >>> c = Counter('aaabbc') 142 >>> c['b'] -= 2 # reduce the count of 'b' by two 143 >>> c.most_common() # 'b' is still in, but its count is zero 144 [('a', 3), ('c', 1), ('b', 0)] 145 146 ''' 147 # References: 148 # http://en.wikipedia.org/wiki/Multiset 149 # http://www.gnu.org/software/smalltalk/manual-base/html_node/Bag.html 150 # http://www.demo2s.com/Tutorial/Cpp/0380__set-multiset/Catalog0380__set-multiset.htm 151 # http://code.activestate.com/recipes/259174/ 152 # Knuth, TAOCP Vol. II section 4.6.3 153 154 def __init__(*args, **kwds): 155 '''Create a new, empty Counter object. And if given, count elements 156 from an input iterable. Or, initialize the count from another mapping 157 of elements to their counts. 158 159 >>> c = Counter() # a new, empty counter 160 >>> c = Counter('gallahad') # a new counter from an iterable 161 >>> c = Counter({'a': 4, 'b': 2}) # a new counter from a mapping 162 >>> c = Counter(a=4, b=2) # a new counter from keyword args 163 164 ''' 165 if not args: 166 raise TypeError("descriptor '__init__' of 'Counter' object " 167 "needs an argument") 168 self = args[0] 169 args = args[1:] 170 if len(args) > 1: 171 raise TypeError('expected at most 1 arguments, got %d' % len(args)) 172 super(Counter, self).__init__() 173 self.update(*args, **kwds) 174 175 def __missing__(self, key): 176 'The count of elements not in the Counter is zero.' 177 # Needed so that self[missing_item] does not raise KeyError 178 return 0 179 180 def most_common(self, n=None): 181 '''List the n most common elements and their counts from the most 182 common to the least. If n is None, then list all element counts. 183 184 >>> Counter('abcdeabcdabcaba').most_common(3) 185 [('a', 5), ('b', 4), ('c', 3)] 186 187 ''' 188 # Emulate Bag.sortedByCount from Smalltalk 189 if n is None: 190 return sorted(self.iteritems(), key=_itemgetter(1), reverse=True) 191 return _heapq.nlargest(n, self.iteritems(), key=_itemgetter(1)) 192 193 def elements(self): 194 '''Iterator over elements repeating each as many times as its count. 195 196 >>> c = Counter('ABCABC') 197 >>> sorted(c.elements()) 198 ['A', 'A', 'B', 'B', 'C', 'C'] 199 200 # Knuth's example for prime factors of 1836: 2**2 * 3**3 * 17**1 201 >>> prime_factors = Counter({2: 2, 3: 3, 17: 1}) 202 >>> product = 1 203 >>> for factor in prime_factors.elements(): # loop over factors 204 ... product *= factor # and multiply them 205 >>> product 206 1836 207 208 Note, if an element's count has been set to zero or is a negative 209 number, elements() will ignore it. 210 211 ''' 212 # Emulate Bag.do from Smalltalk and Multiset.begin from C++. 213 return _chain.from_iterable(_starmap(_repeat, self.iteritems())) 214 215 # Override dict methods where necessary 216 217 @classmethod 218 def fromkeys(cls, iterable, v=None): 219 # There is no equivalent method for counters because setting v=1 220 # means that no element can have a count greater than one. 221 raise NotImplementedError( 222 'Counter.fromkeys() is undefined. Use Counter(iterable) instead.') 223 224 def update(*args, **kwds): 225 '''Like dict.update() but add counts instead of replacing them. 226 227 Source can be an iterable, a dictionary, or another Counter instance. 228 229 >>> c = Counter('which') 230 >>> c.update('witch') # add elements from another iterable 231 >>> d = Counter('watch') 232 >>> c.update(d) # add elements from another counter 233 >>> c['h'] # four 'h' in which, witch, and watch 234 4 235 236 ''' 237 # The regular dict.update() operation makes no sense here because the 238 # replace behavior results in the some of original untouched counts 239 # being mixed-in with all of the other counts for a mismash that 240 # doesn't have a straight-forward interpretation in most counting 241 # contexts. Instead, we implement straight-addition. Both the inputs 242 # and outputs are allowed to contain zero and negative counts. 243 244 if not args: 245 raise TypeError("descriptor 'update' of 'Counter' object " 246 "needs an argument") 247 self = args[0] 248 args = args[1:] 249 if len(args) > 1: 250 raise TypeError('expected at most 1 arguments, got %d' % len(args)) 251 iterable = args[0] if args else None 252 if iterable is not None: 253 if isinstance(iterable, Mapping): 254 if self: 255 self_get = self.get 256 for elem, count in iterable.iteritems(): 257 self[elem] = self_get(elem, 0) + count 258 else: 259 super(Counter, self).update(iterable) # fast path when counter is empty 260 else: 261 self_get = self.get 262 for elem in iterable: 263 self[elem] = self_get(elem, 0) + 1 264 if kwds: 265 self.update(kwds) 266 267 def subtract(*args, **kwds): 268 '''Like dict.update() but subtracts counts instead of replacing them. 269 Counts can be reduced below zero. Both the inputs and outputs are 270 allowed to contain zero and negative counts. 271 272 Source can be an iterable, a dictionary, or another Counter instance. 273 274 >>> c = Counter('which') 275 >>> c.subtract('witch') # subtract elements from another iterable 276 >>> c.subtract(Counter('watch')) # subtract elements from another counter 277 >>> c['h'] # 2 in which, minus 1 in witch, minus 1 in watch 278 0 279 >>> c['w'] # 1 in which, minus 1 in witch, minus 1 in watch 280 -1 281 282 ''' 283 if not args: 284 raise TypeError("descriptor 'subtract' of 'Counter' object " 285 "needs an argument") 286 self = args[0] 287 args = args[1:] 288 if len(args) > 1: 289 raise TypeError('expected at most 1 arguments, got %d' % len(args)) 290 iterable = args[0] if args else None 291 if iterable is not None: 292 self_get = self.get 293 if isinstance(iterable, Mapping): 294 for elem, count in iterable.items(): 295 self[elem] = self_get(elem, 0) - count 296 else: 297 for elem in iterable: 298 self[elem] = self_get(elem, 0) - 1 299 if kwds: 300 self.subtract(kwds) 301 302 def copy(self): 303 'Return a shallow copy.' 304 return self.__class__(self) 305 306 def __reduce__(self): 307 return self.__class__, (dict(self),) 308 309 def __delitem__(self, elem): 310 'Like dict.__delitem__() but does not raise KeyError for missing values.' 311 if elem in self: 312 super(Counter, self).__delitem__(elem) 313 314 def __repr__(self): 315 if not self: 316 return '%s()' % self.__class__.__name__ 317 items = ', '.join(map('%r: %r'.__mod__, self.most_common())) 318 return '%s({%s})' % (self.__class__.__name__, items) 319 320 # Multiset-style mathematical operations discussed in: 321 # Knuth TAOCP Volume II section 4.6.3 exercise 19 322 # and at http://en.wikipedia.org/wiki/Multiset 323 # 324 # Outputs guaranteed to only include positive counts. 325 # 326 # To strip negative and zero counts, add-in an empty counter: 327 # c += Counter() 328 329 def __add__(self, other): 330 '''Add counts from two counters. 331 332 >>> Counter('abbb') + Counter('bcc') 333 Counter({'b': 4, 'c': 2, 'a': 1}) 334 335 ''' 336 if not isinstance(other, Counter): 337 return NotImplemented 338 result = Counter() 339 for elem, count in self.items(): 340 newcount = count + other[elem] 341 if newcount > 0: 342 result[elem] = newcount 343 for elem, count in other.items(): 344 if elem not in self and count > 0: 345 result[elem] = count 346 return result 347 348 def __sub__(self, other): 349 ''' Subtract count, but keep only results with positive counts. 350 351 >>> Counter('abbbc') - Counter('bccd') 352 Counter({'b': 2, 'a': 1}) 353 354 ''' 355 if not isinstance(other, Counter): 356 return NotImplemented 357 result = Counter() 358 for elem, count in self.items(): 359 newcount = count - other[elem] 360 if newcount > 0: 361 result[elem] = newcount 362 for elem, count in other.items(): 363 if elem not in self and count < 0: 364 result[elem] = 0 - count 365 return result 366 367 def __or__(self, other): 368 '''Union is the maximum of value in either of the input counters. 369 370 >>> Counter('abbb') | Counter('bcc') 371 Counter({'b': 3, 'c': 2, 'a': 1}) 372 373 ''' 374 if not isinstance(other, Counter): 375 return NotImplemented 376 result = Counter() 377 for elem, count in self.items(): 378 other_count = other[elem] 379 newcount = other_count if count < other_count else count 380 if newcount > 0: 381 result[elem] = newcount 382 for elem, count in other.items(): 383 if elem not in self and count > 0: 384 result[elem] = count 385 return result 386 387 def __and__(self, other): 388 ''' Intersection is the minimum of corresponding counts. 389 390 >>> Counter('abbb') & Counter('bcc') 391 Counter({'b': 1}) 392 393 ''' 394 if not isinstance(other, Counter): 395 return NotImplemented 396 result = Counter() 397 for elem, count in self.items(): 398 other_count = other[elem] 399 newcount = count if count < other_count else other_count 400 if newcount > 0: 401 result[elem] = newcount 402 return result 403 404 405 if __name__ == '__main__': 406 # verify that instances can be pickled 407 from cPickle import loads, dumps 408 Point = namedtuple('Point', 'x, y', True) 409 p = Point(x=10, y=20) 410 assert p == loads(dumps(p)) 411 412 # test and demonstrate ability to override methods 413 class Point(namedtuple('Point', 'x y')): 414 __slots__ = () 415 @property 416 def hypot(self): 417 return (self.x ** 2 + self.y ** 2) ** 0.5 418 def __str__(self): 419 return 'Point: x=%6.3f y=%6.3f hypot=%6.3f' % (self.x, self.y, self.hypot) 420 421 for p in Point(3, 4), Point(14, 5/7.): 422 print p 423 424 class Point(namedtuple('Point', 'x y')): 425 'Point class with optimized _make() and _replace() without error-checking' 426 __slots__ = () 427 _make = classmethod(tuple.__new__) 428 def _replace(self, _map=map, **kwds): 429 return self._make(_map(kwds.get, ('x', 'y'), self)) 430 431 print Point(11, 22)._replace(x=100) 432 433 Point3D = namedtuple('Point3D', Point._fields + ('z',)) 434 print Point3D.__doc__ 435 436 import doctest 437 TestResults = namedtuple('TestResults', 'failed attempted') 438 print TestResults(*doctest.testmod())
主要是对于像坐标类似的,利于访问
x=1,y=2
具体实例如下:

1 >>> c = collections.namedtuple('c',['x','y']) 2 >>> a=c(1,2) 3 >>> a 4 c(x=1, y=2) 5 >>> a.x 6 1 7 >>> a.y 8 2 9 >>>
5队列
双向队列

1 class deque(object): 2 """ 3 deque([iterable[, maxlen]]) --> deque object 4 5 Build an ordered collection with optimized access from its endpoints. 6 """ 7 def append(self, *args, **kwargs): # real signature unknown 8 """ Add an element to the right side of the deque. """ 9 pass 10 11 def appendleft(self, *args, **kwargs): # real signature unknown 12 """ Add an element to the left side of the deque. """ 13 pass 14 15 def clear(self, *args, **kwargs): # real signature unknown 16 """ Remove all elements from the deque. """ 17 pass 18 19 def count(self, value): # real signature unknown; restored from __doc__ 20 """ D.count(value) -> integer -- return number of occurrences of value """ 21 return 0 22 23 def extend(self, *args, **kwargs): # real signature unknown 24 """ Extend the right side of the deque with elements from the iterable """ 25 pass 26 27 def extendleft(self, *args, **kwargs): # real signature unknown 28 """ Extend the left side of the deque with elements from the iterable """ 29 pass 30 31 def pop(self, *args, **kwargs): # real signature unknown 32 """ Remove and return the rightmost element. """ 33 pass 34 35 def popleft(self, *args, **kwargs): # real signature unknown 36 """ Remove and return the leftmost element. """ 37 pass 38 39 def remove(self, value): # real signature unknown; restored from __doc__ 40 """ D.remove(value) -- remove first occurrence of value. """ 41 pass 42 43 def reverse(self): # real signature unknown; restored from __doc__ 44 """ D.reverse() -- reverse *IN PLACE* """ 45 pass 46 47 def rotate(self, *args, **kwargs): # real signature unknown 48 """ Rotate the deque n steps to the right (default n=1). If n is negative, rotates left. """ 49 pass 50 51 def __copy__(self, *args, **kwargs): # real signature unknown 52 """ Return a shallow copy of a deque. """ 53 pass 54 55 def __delitem__(self, y): # real signature unknown; restored from __doc__ 56 """ x.__delitem__(y) <==> del x[y] """ 57 pass 58 59 def __eq__(self, y): # real signature unknown; restored from __doc__ 60 """ x.__eq__(y) <==> x==y """ 61 pass 62 63 def __getattribute__(self, name): # real signature unknown; restored from __doc__ 64 """ x.__getattribute__('name') <==> x.name """ 65 pass 66 67 def __getitem__(self, y): # real signature unknown; restored from __doc__ 68 """ x.__getitem__(y) <==> x[y] """ 69 pass 70 71 def __ge__(self, y): # real signature unknown; restored from __doc__ 72 """ x.__ge__(y) <==> x>=y """ 73 pass 74 75 def __gt__(self, y): # real signature unknown; restored from __doc__ 76 """ x.__gt__(y) <==> x>y """ 77 pass 78 79 def __iadd__(self, y): # real signature unknown; restored from __doc__ 80 """ x.__iadd__(y) <==> x+=y """ 81 pass 82 83 def __init__(self, iterable=(), maxlen=None): # known case of _collections.deque.__init__ 84 """ 85 deque([iterable[, maxlen]]) --> deque object 86 87 Build an ordered collection with optimized access from its endpoints. 88 # (copied from class doc) 89 """ 90 pass 91 92 def __iter__(self): # real signature unknown; restored from __doc__ 93 """ x.__iter__() <==> iter(x) """ 94 pass 95 96 def __len__(self): # real signature unknown; restored from __doc__ 97 """ x.__len__() <==> len(x) """ 98 pass 99 100 def __le__(self, y): # real signature unknown; restored from __doc__ 101 """ x.__le__(y) <==> x<=y """ 102 pass 103 104 def __lt__(self, y): # real signature unknown; restored from __doc__ 105 """ x.__lt__(y) <==> x<y """ 106 pass 107 108 @staticmethod # known case of __new__ 109 def __new__(S, *more): # real signature unknown; restored from __doc__ 110 """ T.__new__(S, ...) -> a new object with type S, a subtype of T """ 111 pass 112 113 def __ne__(self, y): # real signature unknown; restored from __doc__ 114 """ x.__ne__(y) <==> x!=y """ 115 pass 116 117 def __reduce__(self, *args, **kwargs): # real signature unknown 118 """ Return state information for pickling. """ 119 pass 120 121 def __repr__(self): # real signature unknown; restored from __doc__ 122 """ x.__repr__() <==> repr(x) """ 123 pass 124 125 def __reversed__(self): # real signature unknown; restored from __doc__ 126 """ D.__reversed__() -- return a reverse iterator over the deque """ 127 pass 128 129 def __setitem__(self, i, y): # real signature unknown; restored from __doc__ 130 """ x.__setitem__(i, y) <==> x[i]=y """ 131 pass 132 133 def __sizeof__(self): # real signature unknown; restored from __doc__ 134 """ D.__sizeof__() -- size of D in memory, in bytes """ 135 pass 136 137 maxlen = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 138 """maximum size of a deque or None if unbounded""" 139 140 141 __hash__ = None
左右两边都可以操作,有一个线程安全
具体用法如下:

1 >>> import collections 2 >>> q = collections.deque() 创建 3 >>> q.append(1) 添加 4 >>> q.append(2) 5 >>> q.append(3) 6 >>> q.append(4) 7 >>> q 8 deque([1, 2, 3, 4]) 9 >>> q.appendleft(5) 左边添加 10 >>> q 11 deque([5, 1, 2, 3, 4]) 12 >>> q.count(1) 队列中某个值出现的次数 13 1 14 >>> q.pop() 返回删除的数字,并且删除 15 4 16 >>> q.remove(5) 17 >>> q 18 deque([1, 2, 3]) 19 >>> b=collections.deque() 20 >>> b.append(9) 21 >>> b.append(0) 22 >>> q.extend(b) q和b合并 23 >>> q 24 deque([1, 2, 3, 9, 0]) 25 >>> q.clear() 清除 26 >>> q 27 deque([]) 28 >>>
单项队列(fifo先进先出)

1 class Queue: 2 """Create a queue object with a given maximum size. 3 4 If maxsize is <= 0, the queue size is infinite. 5 """ 6 def __init__(self, maxsize=0): 7 self.maxsize = maxsize 8 self._init(maxsize) 9 # mutex must be held whenever the queue is mutating. All methods 10 # that acquire mutex must release it before returning. mutex 11 # is shared between the three conditions, so acquiring and 12 # releasing the conditions also acquires and releases mutex. 13 self.mutex = _threading.Lock() 14 # Notify not_empty whenever an item is added to the queue; a 15 # thread waiting to get is notified then. 16 self.not_empty = _threading.Condition(self.mutex) 17 # Notify not_full whenever an item is removed from the queue; 18 # a thread waiting to put is notified then. 19 self.not_full = _threading.Condition(self.mutex) 20 # Notify all_tasks_done whenever the number of unfinished tasks 21 # drops to zero; thread waiting to join() is notified to resume 22 self.all_tasks_done = _threading.Condition(self.mutex) 23 self.unfinished_tasks = 0 24 25 def task_done(self): 26 """Indicate that a formerly enqueued task is complete. 27 28 Used by Queue consumer threads. For each get() used to fetch a task, 29 a subsequent call to task_done() tells the queue that the processing 30 on the task is complete. 31 32 If a join() is currently blocking, it will resume when all items 33 have been processed (meaning that a task_done() call was received 34 for every item that had been put() into the queue). 35 36 Raises a ValueError if called more times than there were items 37 placed in the queue. 38 """ 39 self.all_tasks_done.acquire() 40 try: 41 unfinished = self.unfinished_tasks - 1 42 if unfinished <= 0: 43 if unfinished < 0: 44 raise ValueError('task_done() called too many times') 45 self.all_tasks_done.notify_all() 46 self.unfinished_tasks = unfinished 47 finally: 48 self.all_tasks_done.release() 49 50 def join(self): 51 """Blocks until all items in the Queue have been gotten and processed. 52 53 The count of unfinished tasks goes up whenever an item is added to the 54 queue. The count goes down whenever a consumer thread calls task_done() 55 to indicate the item was retrieved and all work on it is complete. 56 57 When the count of unfinished tasks drops to zero, join() unblocks. 58 """ 59 self.all_tasks_done.acquire() 60 try: 61 while self.unfinished_tasks: 62 self.all_tasks_done.wait() 63 finally: 64 self.all_tasks_done.release() 65 66 def qsize(self): 67 """Return the approximate size of the queue (not reliable!).""" 68 self.mutex.acquire() 69 n = self._qsize() 70 self.mutex.release() 71 return n 72 73 def empty(self): 74 """Return True if the queue is empty, False otherwise (not reliable!).""" 75 self.mutex.acquire() 76 n = not self._qsize() 77 self.mutex.release() 78 return n 79 80 def full(self): 81 """Return True if the queue is full, False otherwise (not reliable!).""" 82 self.mutex.acquire() 83 n = 0 < self.maxsize == self._qsize() 84 self.mutex.release() 85 return n 86 87 def put(self, item, block=True, timeout=None): 88 """Put an item into the queue. 89 90 If optional args 'block' is true and 'timeout' is None (the default), 91 block if necessary until a free slot is available. If 'timeout' is 92 a non-negative number, it blocks at most 'timeout' seconds and raises 93 the Full exception if no free slot was available within that time. 94 Otherwise ('block' is false), put an item on the queue if a free slot 95 is immediately available, else raise the Full exception ('timeout' 96 is ignored in that case). 97 """ 98 self.not_full.acquire() 99 try: 100 if self.maxsize > 0: 101 if not block: 102 if self._qsize() == self.maxsize: 103 raise Full 104 elif timeout is None: 105 while self._qsize() == self.maxsize: 106 self.not_full.wait() 107 elif timeout < 0: 108 raise ValueError("'timeout' must be a non-negative number") 109 else: 110 endtime = _time() + timeout 111 while self._qsize() == self.maxsize: 112 remaining = endtime - _time() 113 if remaining <= 0.0: 114 raise Full 115 self.not_full.wait(remaining) 116 self._put(item) 117 self.unfinished_tasks += 1 118 self.not_empty.notify() 119 finally: 120 self.not_full.release() 121 122 def put_nowait(self, item): 123 """Put an item into the queue without blocking. 124 125 Only enqueue the item if a free slot is immediately available. 126 Otherwise raise the Full exception. 127 """ 128 return self.put(item, False) 129 130 def get(self, block=True, timeout=None): 131 """Remove and return an item from the queue. 132 133 If optional args 'block' is true and 'timeout' is None (the default), 134 block if necessary until an item is available. If 'timeout' is 135 a non-negative number, it blocks at most 'timeout' seconds and raises 136 the Empty exception if no item was available within that time. 137 Otherwise ('block' is false), return an item if one is immediately 138 available, else raise the Empty exception ('timeout' is ignored 139 in that case). 140 """ 141 self.not_empty.acquire() 142 try: 143 if not block: 144 if not self._qsize(): 145 raise Empty 146 elif timeout is None: 147 while not self._qsize(): 148 self.not_empty.wait() 149 elif timeout < 0: 150 raise ValueError("'timeout' must be a non-negative number") 151 else: 152 endtime = _time() + timeout 153 while not self._qsize(): 154 remaining = endtime - _time() 155 if remaining <= 0.0: 156 raise Empty 157 self.not_empty.wait(remaining) 158 item = self._get() 159 self.not_full.notify() 160 return item 161 finally: 162 self.not_empty.release() 163 164 def get_nowait(self): 165 """Remove and return an item from the queue without blocking. 166 167 Only get an item if one is immediately available. Otherwise 168 raise the Empty exception. 169 """ 170 return self.get(False) 171 172 # Override these methods to implement other queue organizations 173 # (e.g. stack or priority queue). 174 # These will only be called with appropriate locks held 175 176 # Initialize the queue representation 177 def _init(self, maxsize): 178 self.queue = deque() 179 180 def _qsize(self, len=len): 181 return len(self.queue) 182 183 # Put a new item in the queue 184 def _put(self, item): 185 self.queue.append(item) 186 187 # Get an item from the queue 188 def _get(self): 189 return self.queue.popleft() 190 191 192 class PriorityQueue(Queue): 193 '''Variant of Queue that retrieves open entries in priority order (lowest first). 194 195 Entries are typically tuples of the form: (priority number, data). 196 ''' 197 198 def _init(self, maxsize): 199 self.queue = [] 200 201 def _qsize(self, len=len): 202 return len(self.queue) 203 204 def _put(self, item, heappush=heapq.heappush): 205 heappush(self.queue, item) 206 207 def _get(self, heappop=heapq.heappop): 208 return heappop(self.queue) 209 210 211 class LifoQueue(Queue): 212 '''Variant of Queue that retrieves most recently added entries first.''' 213 214 def _init(self, maxsize): 215 self.queue = [] 216 217 def _qsize(self, len=len): 218 return len(self.queue) 219 220 def _put(self, item): 221 self.queue.append(item) 222 223 def _get(self): 224 return self.queue.pop()
实例如下:

1 import Queue 2 q = Queue.Queue(10) 3 出队 4 q.put(1) 5 q.put(2) 6 q.put(3) 7 8 入队 9 q.get() 10 q.get() 11 q.get() 12 q.get() 13 取出过程中,如果取出全部,在继续取的话,会一直等待,直到有值放进去
2迭代器和生成器简介
迭代器为类序列对象提供了一个类序列的接口。python的迭代无缝地支持序列对象,而且它还允许程序员迭代非序列类型,包括用户定义的对象。迭代器用起来很灵巧,你可以迭代不是序列但表现处序列行为的对象,例如字典的键、一个文件的行,等等。迭代器的作用如下:
•提供了刻扩展的迭代器接口;
•对列表迭代带来了性能上的增强;
•在字典迭代中性能提升;
•创建真正的迭代接口,而不是原来的随即对象访问;
•与所有已经存在的用户定义的类以及扩展得模拟序列和映射的对象向后兼容;
•迭代非序列集合(例如映射和文件)时,可以创建更简洁可读的代码
当如果不存在,则报异常 StopIteration,最常用的是next方法和iter()函数
具体举例如下

1 >>> a = [1,2,3,4] 2 >>> a 3 [1, 2, 3, 4] 4 >>> b = iter(a) 5 >>> b.next() 迭代 6 1 7 >>> b.next() 8 2 9 >>> b.next() 10 3 11 >>> b.next() 12 4 13 >>> b.next() 不存在报错StopIteration 14 Traceback (most recent call last): 15 File "<stdin>", line 1, in <module> 16 StopIteration 17 >>> s = {'one':1,'two':2,'three':3} 18 >>> c = iter(s) 19 >>> c.next() 20 'three' 21 >>> c.next() 22 'two' 23 >>>
另外一种就是for循环

1 >>> for i in [11,22,33,44]: 2 ... print i 3 ... 4 11 5 22 6 33 7 44 8 >>>
生成器:
range不是生成器 和 xrange 是生成器
readlines不是生成器 和 xreadlines 是生成器
生成器内部基于yield创建,即:对于生成器只有使用时才创建,从而不避免内存浪费
yield 语句可以让普通函数变成一个生成器,并且相应的 __next__() 方法返回的是 yield 后面的值。一种更直观的解释是:程序执行到 yield 会返回值并暂停,再次调用 next() 时会从上次暂停的地方继续开始执行(暂时冻结函数)

1 def func1(): 2 yield 1 3 yield 2 4 yield 3 5 for i in func1(): 6 print i 7 yield可以临时冻结函数,会一个一个的生成 8 结果是:1 9 2 10 3
1 yield临时冻结函数 2 def mrang(arg): 3 seed = 0 4 while True: 5 seed = seed + 1 6 yield seed 7 8 for i in mrang(10): 9 print i
3冒泡排序小练习
原理:
经典排序算法 - 冒泡排序Bubble sort
原理是临近的数字两两进行比较,按照从小到大或者从大到小的顺序进行交换,这样一趟过去后,最大或最小的数字被交换到了最后一位,然后再从头开始进行两两比较交换,直到倒数第二位时结束。

1 对列表元素进行排序 2 li = [13,22,6,99,11] 3 两个两个的对比 4 5 for n in range(1,len(li)-1): 6 for m in range(len(li) -n): 7 num1=li[m] 8 num2=li[m+1] 9 #将较大的放在右侧 10 if num1>num2: 11 temp=li[m] 12 li[m]=num2 13 li[m+1]=temp 14 print li
或者是:

1 li = [13,22,6,99,11] 2 for m in range(len(li)-1): 3 4 for n in range(m+1, len(li)): 5 if li[m]> li[n]: 6 temp = li[n] 7 li[n] = li[m] 8 li[m] = temp 9 10 print li
4内置函数
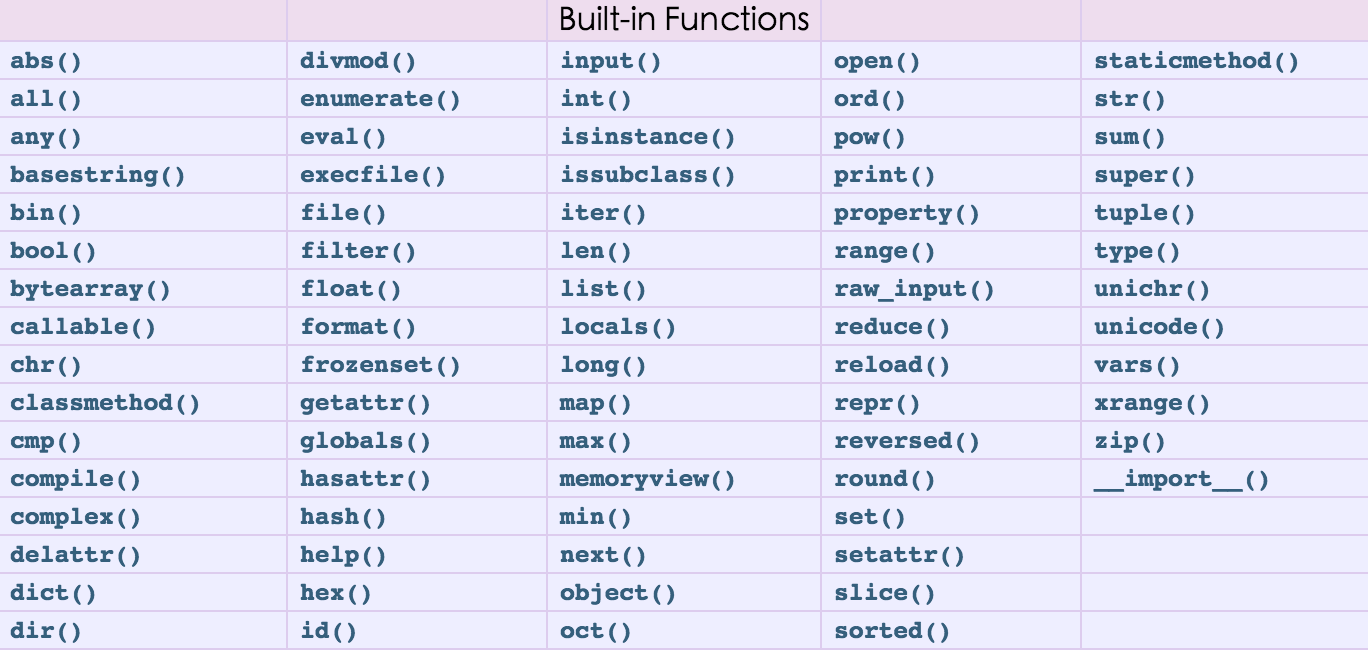
简要介绍一些没见到的:

1 >>> a = ['',1,2,3] 2 >>> all(a) #一假变假 3 False 4 >>> any(a) #一真变真 5 True 6 >>> b = -1 7 >>> abs(b) #求绝对值 8 1 9 >>> c = 10 10 >>> divmod(10,3) 求除数和余数 11 (3, 1) 12 >>> print vars() 列出某种类型的方法 13 {'a': ['', 1, 2, 3], 'c': 10, 'b': -1, '__builtins__': <module '__builtin__' (built-in)>, '__package__': None, '__name__': '__main__', '__doc__': None} 14 >>> b = [9,8,7,5,3] 15 >>> for i in enumerate(a): 16 ... print i 17 ... 18 (0, '') 19 (1, 1) 20 (2, 2) 21 (3, 3) 22 >>> ord('a') 23 97 24 >>> a = 1 25 >>> chr(a) 26 'x01' 27 >>> 28 print __name__ #函数的名字 29 print __doc__ #说明 30 例如 31 #!/usr/bin/env python 32 ''' 33 123456 34 ''' 35 print __doc__ 36 print __name__ 37 输出: 38 123456 39 40 __main__
1 print ord('c') 2 print chr(99) 3 print hex(10) #16进制 4 print oct(12) #八进制 5 print bin(2) #二进制
5文件操作
首先打开文件,然后对文件进行操作
1 文件句柄 = file(文件名称,模式) 2 文件句柄 = open(文件名称,模式) 3 推荐使用open
文件模式介绍:
开文件的模式有:
- r,只读模式(默认)。
- w,只写模式。【不可读;不存在则创建;存在则删除内容;】
- a,追加模式。【可读; 不存在则创建;存在则只追加内容;】
"+" 表示可以同时读写某个文件
- r+,可读写文件。【可读;可写;可追加】
- w+,写读
- a+,同a
"U"表示在读取时,可以将 自动转换成 (与 r 或 r+ 模式同使用)
- rU
- r+U
"b"表示处理二进制文件(如:FTP发送上传ISO镜像文件,linux可忽略,windows处理二进制文件时需标注)
- rb
- wb
- ab
文件的方法如下:

1 class file(object): 2 """ 3 file(name[, mode[, buffering]]) -> file object 4 5 Open a file. The mode can be 'r', 'w' or 'a' for reading (default), 6 writing or appending. The file will be created if it doesn't exist 7 when opened for writing or appending; it will be truncated when 8 opened for writing. Add a 'b' to the mode for binary files. 9 Add a '+' to the mode to allow simultaneous reading and writing. 10 If the buffering argument is given, 0 means unbuffered, 1 means line 11 buffered, and larger numbers specify the buffer size. The preferred way 12 to open a file is with the builtin open() function. 13 Add a 'U' to mode to open the file for input with universal newline 14 support. Any line ending in the input file will be seen as a ' ' 15 in Python. Also, a file so opened gains the attribute 'newlines'; 16 the value for this attribute is one of None (no newline read yet), 17 ' ', ' ', ' ' or a tuple containing all the newline types seen. 18 19 'U' cannot be combined with 'w' or '+' mode. 20 """ 21 def close(self): # real signature unknown; restored from __doc__ 22 """ 23 close() -> None or (perhaps) an integer. Close the file. 24 25 Sets data attribute .closed to True. A closed file cannot be used for 26 further I/O operations. close() may be called more than once without 27 error. Some kinds of file objects (for example, opened by popen()) 28 may return an exit status upon closing. 29 """ 30 pass 31 32 def fileno(self): # real signature unknown; restored from __doc__ 33 """ 34 fileno() -> integer "file descriptor". 35 36 This is needed for lower-level file interfaces, such os.read(). 37 """ 38 return 0 39 40 def flush(self): # real signature unknown; restored from __doc__ 41 """ flush() -> None. Flush the internal I/O buffer. """ 42 pass 43 44 def isatty(self): # real signature unknown; restored from __doc__ 45 """ isatty() -> true or false. True if the file is connected to a tty device. """ 46 return False 47 48 def next(self): # real signature unknown; restored from __doc__ 49 """ x.next() -> the next value, or raise StopIteration """ 50 pass 51 52 def read(self, size=None): # real signature unknown; restored from __doc__ 53 """ 54 read([size]) -> read at most size bytes, returned as a string. 55 56 If the size argument is negative or omitted, read until EOF is reached. 57 Notice that when in non-blocking mode, less data than what was requested 58 may be returned, even if no size parameter was given. 59 """ 60 pass 61 62 def readinto(self): # real signature unknown; restored from __doc__ 63 """ readinto() -> Undocumented. Don't use this; it may go away. """ 64 pass 65 66 def readline(self, size=None): # real signature unknown; restored from __doc__ 67 """ 68 readline([size]) -> next line from the file, as a string. 69 70 Retain newline. A non-negative size argument limits the maximum 71 number of bytes to return (an incomplete line may be returned then). 72 Return an empty string at EOF. 73 """ 74 pass 75 76 def readlines(self, size=None): # real signature unknown; restored from __doc__ 77 """ 78 readlines([size]) -> list of strings, each a line from the file. 79 80 Call readline() repeatedly and return a list of the lines so read. 81 The optional size argument, if given, is an approximate bound on the 82 total number of bytes in the lines returned. 83 """ 84 return [] 85 86 def seek(self, offset, whence=None): # real signature unknown; restored from __doc__ 87 """ 88 seek(offset[, whence]) -> None. Move to new file position. 89 90 Argument offset is a byte count. Optional argument whence defaults to 91 0 (offset from start of file, offset should be >= 0); other values are 1 92 (move relative to current position, positive or negative), and 2 (move 93 relative to end of file, usually negative, although many platforms allow 94 seeking beyond the end of a file). If the file is opened in text mode, 95 only offsets returned by tell() are legal. Use of other offsets causes 96 undefined behavior. 97 Note that not all file objects are seekable. 98 """ 99 pass 100 101 def tell(self): # real signature unknown; restored from __doc__ 102 """ tell() -> current file position, an integer (may be a long integer). """ 103 pass 104 105 def truncate(self, size=None): # real signature unknown; restored from __doc__ 106 """ 107 truncate([size]) -> None. Truncate the file to at most size bytes. 108 109 Size defaults to the current file position, as returned by tell(). 110 """ 111 pass 112 113 def write(self, p_str): # real signature unknown; restored from __doc__ 114 """ 115 write(str) -> None. Write string str to file. 116 117 Note that due to buffering, flush() or close() may be needed before 118 the file on disk reflects the data written. 119 """ 120 pass 121 122 def writelines(self, sequence_of_strings): # real signature unknown; restored from __doc__ 123 """ 124 writelines(sequence_of_strings) -> None. Write the strings to the file. 125 126 Note that newlines are not added. The sequence can be any iterable object 127 producing strings. This is equivalent to calling write() for each string. 128 """ 129 pass 130 131 def xreadlines(self): # real signature unknown; restored from __doc__ 132 """ 133 xreadlines() -> returns self. 134 135 For backward compatibility. File objects now include the performance 136 optimizations previously implemented in the xreadlines module. 137 """ 138 pass 139 140 def __delattr__(self, name): # real signature unknown; restored from __doc__ 141 """ x.__delattr__('name') <==> del x.name """ 142 pass 143 144 def __enter__(self): # real signature unknown; restored from __doc__ 145 """ __enter__() -> self. """ 146 return self 147 148 def __exit__(self, *excinfo): # real signature unknown; restored from __doc__ 149 """ __exit__(*excinfo) -> None. Closes the file. """ 150 pass 151 152 def __getattribute__(self, name): # real signature unknown; restored from __doc__ 153 """ x.__getattribute__('name') <==> x.name """ 154 pass 155 156 def __init__(self, name, mode=None, buffering=None): # real signature unknown; restored from __doc__ 157 pass 158 159 def __iter__(self): # real signature unknown; restored from __doc__ 160 """ x.__iter__() <==> iter(x) """ 161 pass 162 163 @staticmethod # known case of __new__ 164 def __new__(S, *more): # real signature unknown; restored from __doc__ 165 """ T.__new__(S, ...) -> a new object with type S, a subtype of T """ 166 pass 167 168 def __repr__(self): # real signature unknown; restored from __doc__ 169 """ x.__repr__() <==> repr(x) """ 170 pass 171 172 def __setattr__(self, name, value): # real signature unknown; restored from __doc__ 173 """ x.__setattr__('name', value) <==> x.name = value """ 174 pass 175 176 closed = property(lambda self: True) 177 """True if the file is closed 178 179 :type: bool 180 """ 181 182 encoding = property(lambda self: '') 183 """file encoding 184 185 :type: string 186 """ 187 188 errors = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 189 """Unicode error handler""" 190 191 mode = property(lambda self: '') 192 """file mode ('r', 'U', 'w', 'a', possibly with 'b' or '+' added) 193 194 :type: string 195 """ 196 197 name = property(lambda self: '') 198 """file name 199 200 :type: string 201 """ 202 203 newlines = property(lambda self: '') 204 """end-of-line convention used in this file 205 206 :type: string 207 """ 208 209 softspace = property(lambda self: True) 210 """flag indicating that a space needs to be printed; used by print 211 212 :type: bool 213 """ 214 215 216 217 class float(object): 218 """ 219 float(x) -> floating point number 220 221 Convert a string or number to a floating point number, if possible. 222 """ 223 def as_integer_ratio(self): # real signature unknown; restored from __doc__ 224 """ 225 float.as_integer_ratio() -> (int, int) 226 227 Return a pair of integers, whose ratio is exactly equal to the original 228 float and with a positive denominator. 229 Raise OverflowError on infinities and a ValueError on NaNs. 230 231 >>> (10.0).as_integer_ratio() 232 (10, 1) 233 >>> (0.0).as_integer_ratio() 234 (0, 1) 235 >>> (-.25).as_integer_ratio() 236 (-1, 4) 237 """ 238 pass 239 240 def conjugate(self, *args, **kwargs): # real signature unknown 241 """ Return self, the complex conjugate of any float. """ 242 pass 243 244 def fromhex(self, string): # real signature unknown; restored from __doc__ 245 """ 246 float.fromhex(string) -> float 247 248 Create a floating-point number from a hexadecimal string. 249 >>> float.fromhex('0x1.ffffp10') 250 2047.984375 251 >>> float.fromhex('-0x1p-1074') 252 -4.9406564584124654e-324 253 """ 254 return 0.0 255 256 def hex(self): # real signature unknown; restored from __doc__ 257 """ 258 float.hex() -> string 259 260 Return a hexadecimal representation of a floating-point number. 261 >>> (-0.1).hex() 262 '-0x1.999999999999ap-4' 263 >>> 3.14159.hex() 264 '0x1.921f9f01b866ep+1' 265 """ 266 return "" 267 268 def is_integer(self, *args, **kwargs): # real signature unknown 269 """ Return True if the float is an integer. """ 270 pass 271 272 def __abs__(self): # real signature unknown; restored from __doc__ 273 """ x.__abs__() <==> abs(x) """ 274 pass 275 276 def __add__(self, y): # real signature unknown; restored from __doc__ 277 """ x.__add__(y) <==> x+y """ 278 pass 279 280 def __coerce__(self, y): # real signature unknown; restored from __doc__ 281 """ x.__coerce__(y) <==> coerce(x, y) """ 282 pass 283 284 def __divmod__(self, y): # real signature unknown; restored from __doc__ 285 """ x.__divmod__(y) <==> divmod(x, y) """ 286 pass 287 288 def __div__(self, y): # real signature unknown; restored from __doc__ 289 """ x.__div__(y) <==> x/y """ 290 pass 291 292 def __eq__(self, y): # real signature unknown; restored from __doc__ 293 """ x.__eq__(y) <==> x==y """ 294 pass 295 296 def __float__(self): # real signature unknown; restored from __doc__ 297 """ x.__float__() <==> float(x) """ 298 pass 299 300 def __floordiv__(self, y): # real signature unknown; restored from __doc__ 301 """ x.__floordiv__(y) <==> x//y """ 302 pass 303 304 def __format__(self, format_spec): # real signature unknown; restored from __doc__ 305 """ 306 float.__format__(format_spec) -> string 307 308 Formats the float according to format_spec. 309 """ 310 return "" 311 312 def __getattribute__(self, name): # real signature unknown; restored from __doc__ 313 """ x.__getattribute__('name') <==> x.name """ 314 pass 315 316 def __getformat__(self, typestr): # real signature unknown; restored from __doc__ 317 """ 318 float.__getformat__(typestr) -> string 319 320 You probably don't want to use this function. It exists mainly to be 321 used in Python's test suite. 322 323 typestr must be 'double' or 'float'. This function returns whichever of 324 'unknown', 'IEEE, big-endian' or 'IEEE, little-endian' best describes the 325 format of floating point numbers used by the C type named by typestr. 326 """ 327 return "" 328 329 def __getnewargs__(self, *args, **kwargs): # real signature unknown 330 pass 331 332 def __ge__(self, y): # real signature unknown; restored from __doc__ 333 """ x.__ge__(y) <==> x>=y """ 334 pass 335 336 def __gt__(self, y): # real signature unknown; restored from __doc__ 337 """ x.__gt__(y) <==> x>y """ 338 pass 339 340 def __hash__(self): # real signature unknown; restored from __doc__ 341 """ x.__hash__() <==> hash(x) """ 342 pass 343 344 def __init__(self, x): # real signature unknown; restored from __doc__ 345 pass 346 347 def __int__(self): # real signature unknown; restored from __doc__ 348 """ x.__int__() <==> int(x) """ 349 pass 350 351 def __le__(self, y): # real signature unknown; restored from __doc__ 352 """ x.__le__(y) <==> x<=y """ 353 pass 354 355 def __long__(self): # real signature unknown; restored from __doc__ 356 """ x.__long__() <==> long(x) """ 357 pass 358 359 def __lt__(self, y): # real signature unknown; restored from __doc__ 360 """ x.__lt__(y) <==> x<y """ 361 pass 362 363 def __mod__(self, y): # real signature unknown; restored from __doc__ 364 """ x.__mod__(y) <==> x%y """ 365 pass 366 367 def __mul__(self, y): # real signature unknown; restored from __doc__ 368 """ x.__mul__(y) <==> x*y """ 369 pass 370 371 def __neg__(self): # real signature unknown; restored from __doc__ 372 """ x.__neg__() <==> -x """ 373 pass 374 375 @staticmethod # known case of __new__ 376 def __new__(S, *more): # real signature unknown; restored from __doc__ 377 """ T.__new__(S, ...) -> a new object with type S, a subtype of T """ 378 pass 379 380 def __ne__(self, y): # real signature unknown; restored from __doc__ 381 """ x.__ne__(y) <==> x!=y """ 382 pass 383 384 def __nonzero__(self): # real signature unknown; restored from __doc__ 385 """ x.__nonzero__() <==> x != 0 """ 386 pass 387 388 def __pos__(self): # real signature unknown; restored from __doc__ 389 """ x.__pos__() <==> +x """ 390 pass 391 392 def __pow__(self, y, z=None): # real signature unknown; restored from __doc__ 393 """ x.__pow__(y[, z]) <==> pow(x, y[, z]) """ 394 pass 395 396 def __radd__(self, y): # real signature unknown; restored from __doc__ 397 """ x.__radd__(y) <==> y+x """ 398 pass 399 400 def __rdivmod__(self, y): # real signature unknown; restored from __doc__ 401 """ x.__rdivmod__(y) <==> divmod(y, x) """ 402 pass 403 404 def __rdiv__(self, y): # real signature unknown; restored from __doc__ 405 """ x.__rdiv__(y) <==> y/x """ 406 pass 407 408 def __repr__(self): # real signature unknown; restored from __doc__ 409 """ x.__repr__() <==> repr(x) """ 410 pass 411 412 def __rfloordiv__(self, y): # real signature unknown; restored from __doc__ 413 """ x.__rfloordiv__(y) <==> y//x """ 414 pass 415 416 def __rmod__(self, y): # real signature unknown; restored from __doc__ 417 """ x.__rmod__(y) <==> y%x """ 418 pass 419 420 def __rmul__(self, y): # real signature unknown; restored from __doc__ 421 """ x.__rmul__(y) <==> y*x """ 422 pass 423 424 def __rpow__(self, x, z=None): # real signature unknown; restored from __doc__ 425 """ y.__rpow__(x[, z]) <==> pow(x, y[, z]) """ 426 pass 427 428 def __rsub__(self, y): # real signature unknown; restored from __doc__ 429 """ x.__rsub__(y) <==> y-x """ 430 pass 431 432 def __rtruediv__(self, y): # real signature unknown; restored from __doc__ 433 """ x.__rtruediv__(y) <==> y/x """ 434 pass 435 436 def __setformat__(self, typestr, fmt): # real signature unknown; restored from __doc__ 437 """ 438 float.__setformat__(typestr, fmt) -> None 439 440 You probably don't want to use this function. It exists mainly to be 441 used in Python's test suite. 442 443 typestr must be 'double' or 'float'. fmt must be one of 'unknown', 444 'IEEE, big-endian' or 'IEEE, little-endian', and in addition can only be 445 one of the latter two if it appears to match the underlying C reality. 446 447 Override the automatic determination of C-level floating point type. 448 This affects how floats are converted to and from binary strings. 449 """ 450 pass 451 452 def __str__(self): # real signature unknown; restored from __doc__ 453 """ x.__str__() <==> str(x) """ 454 pass 455 456 def __sub__(self, y): # real signature unknown; restored from __doc__ 457 """ x.__sub__(y) <==> x-y """ 458 pass 459 460 def __truediv__(self, y): # real signature unknown; restored from __doc__ 461 """ x.__truediv__(y) <==> x/y """ 462 pass 463 464 def __trunc__(self, *args, **kwargs): # real signature unknown 465 """ Return the Integral closest to x between 0 and x. """ 466 pass 467 468 imag = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 469 """the imaginary part of a complex number""" 470 471 real = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 472 """the real part of a complex number""" 473 474 475 476 class frozenset(object): 477 """ 478 frozenset() -> empty frozenset object 479 frozenset(iterable) -> frozenset object 480 481 Build an immutable unordered collection of unique elements. 482 """ 483 def copy(self, *args, **kwargs): # real signature unknown 484 """ Return a shallow copy of a set. """ 485 pass 486 487 def difference(self, *args, **kwargs): # real signature unknown 488 """ 489 Return the difference of two or more sets as a new set. 490 491 (i.e. all elements that are in this set but not the others.) 492 """ 493 pass 494 495 def intersection(self, *args, **kwargs): # real signature unknown 496 """ 497 Return the intersection of two or more sets as a new set. 498 499 (i.e. elements that are common to all of the sets.) 500 """ 501 pass 502 503 def isdisjoint(self, *args, **kwargs): # real signature unknown 504 """ Return True if two sets have a null intersection. """ 505 pass 506 507 def issubset(self, *args, **kwargs): # real signature unknown 508 """ Report whether another set contains this set. """ 509 pass 510 511 def issuperset(self, *args, **kwargs): # real signature unknown 512 """ Report whether this set contains another set. """ 513 pass 514 515 def symmetric_difference(self, *args, **kwargs): # real signature unknown 516 """ 517 Return the symmetric difference of two sets as a new set. 518 519 (i.e. all elements that are in exactly one of the sets.) 520 """ 521 pass 522 523 def union(self, *args, **kwargs): # real signature unknown 524 """ 525 Return the union of sets as a new set. 526 527 (i.e. all elements that are in either set.) 528 """ 529 pass 530 531 def __and__(self, y): # real signature unknown; restored from __doc__ 532 """ x.__and__(y) <==> x&y """ 533 pass 534 535 def __cmp__(self, y): # real signature unknown; restored from __doc__ 536 """ x.__cmp__(y) <==> cmp(x,y) """ 537 pass 538 539 def __contains__(self, y): # real signature unknown; restored from __doc__ 540 """ x.__contains__(y) <==> y in x. """ 541 pass 542 543 def __eq__(self, y): # real signature unknown; restored from __doc__ 544 """ x.__eq__(y) <==> x==y """ 545 pass 546 547 def __getattribute__(self, name): # real signature unknown; restored from __doc__ 548 """ x.__getattribute__('name') <==> x.name """ 549 pass 550 551 def __ge__(self, y): # real signature unknown; restored from __doc__ 552 """ x.__ge__(y) <==> x>=y """ 553 pass 554 555 def __gt__(self, y): # real signature unknown; restored from __doc__ 556 """ x.__gt__(y) <==> x>y """ 557 pass 558 559 def __hash__(self): # real signature unknown; restored from __doc__ 560 """ x.__hash__() <==> hash(x) """ 561 pass 562 563 def __init__(self, seq=()): # known special case of frozenset.__init__ 564 """ x.__init__(...) initializes x; see help(type(x)) for signature """ 565 pass 566 567 def __iter__(self): # real signature unknown; restored from __doc__ 568 """ x.__iter__() <==> iter(x) """ 569 pass 570 571 def __len__(self): # real signature unknown; restored from __doc__ 572 """ x.__len__() <==> len(x) """ 573 pass 574 575 def __le__(self, y): # real signature unknown; restored from __doc__ 576 """ x.__le__(y) <==> x<=y """ 577 pass 578 579 def __lt__(self, y): # real signature unknown; restored from __doc__ 580 """ x.__lt__(y) <==> x<y """ 581 pass 582 583 @staticmethod # known case of __new__ 584 def __new__(S, *more): # real signature unknown; restored from __doc__ 585 """ T.__new__(S, ...) -> a new object with type S, a subtype of T """ 586 pass 587 588 def __ne__(self, y): # real signature unknown; restored from __doc__ 589 """ x.__ne__(y) <==> x!=y """ 590 pass 591 592 def __or__(self, y): # real signature unknown; restored from __doc__ 593 """ x.__or__(y) <==> x|y """ 594 pass 595 596 def __rand__(self, y): # real signature unknown; restored from __doc__ 597 """ x.__rand__(y) <==> y&x """ 598 pass 599 600 def __reduce__(self, *args, **kwargs): # real signature unknown 601 """ Return state information for pickling. """ 602 pass 603 604 def __repr__(self): # real signature unknown; restored from __doc__ 605 """ x.__repr__() <==> repr(x) """ 606 pass 607 608 def __ror__(self, y): # real signature unknown; restored from __doc__ 609 """ x.__ror__(y) <==> y|x """ 610 pass 611 612 def __rsub__(self, y): # real signature unknown; restored from __doc__ 613 """ x.__rsub__(y) <==> y-x """ 614 pass 615 616 def __rxor__(self, y): # real signature unknown; restored from __doc__ 617 """ x.__rxor__(y) <==> y^x """ 618 pass 619 620 def __sizeof__(self): # real signature unknown; restored from __doc__ 621 """ S.__sizeof__() -> size of S in memory, in bytes """ 622 pass 623 624 def __sub__(self, y): # real signature unknown; restored from __doc__ 625 """ x.__sub__(y) <==> x-y """ 626 pass 627 628 def __xor__(self, y): # real signature unknown; restored from __doc__ 629 """ x.__xor__(y) <==> x^y """ 630 pass 631 632 633 class list(object): 634 """ 635 list() -> new empty list 636 list(iterable) -> new list initialized from iterable's items 637 """ 638 def append(self, p_object): # real signature unknown; restored from __doc__ 639 """ L.append(object) -- append object to end """ 640 pass 641 642 def count(self, value): # real signature unknown; restored from __doc__ 643 """ L.count(value) -> integer -- return number of occurrences of value """ 644 return 0 645 646 def extend(self, iterable): # real signature unknown; restored from __doc__ 647 """ L.extend(iterable) -- extend list by appending elements from the iterable """ 648 pass 649 650 def index(self, value, start=None, stop=None): # real signature unknown; restored from __doc__ 651 """ 652 L.index(value, [start, [stop]]) -> integer -- return first index of value. 653 Raises ValueError if the value is not present. 654 """ 655 return 0 656 657 def insert(self, index, p_object): # real signature unknown; restored from __doc__ 658 """ L.insert(index, object) -- insert object before index """ 659 pass 660 661 def pop(self, index=None): # real signature unknown; restored from __doc__ 662 """ 663 L.pop([index]) -> item -- remove and return item at index (default last). 664 Raises IndexError if list is empty or index is out of range. 665 """ 666 pass 667 668 def remove(self, value): # real signature unknown; restored from __doc__ 669 """ 670 L.remove(value) -- remove first occurrence of value. 671 Raises ValueError if the value is not present. 672 """ 673 pass 674 675 def reverse(self): # real signature unknown; restored from __doc__ 676 """ L.reverse() -- reverse *IN PLACE* """ 677 pass 678 679 def sort(self, cmp=None, key=None, reverse=False): # real signature unknown; restored from __doc__ 680 """ 681 L.sort(cmp=None, key=None, reverse=False) -- stable sort *IN PLACE*; 682 cmp(x, y) -> -1, 0, 1 683 """ 684 pass 685 686 def __add__(self, y): # real signature unknown; restored from __doc__ 687 """ x.__add__(y) <==> x+y """ 688 pass 689 690 def __contains__(self, y): # real signature unknown; restored from __doc__ 691 """ x.__contains__(y) <==> y in x """ 692 pass 693 694 def __delitem__(self, y): # real signature unknown; restored from __doc__ 695 """ x.__delitem__(y) <==> del x[y] """ 696 pass 697 698 def __delslice__(self, i, j): # real signature unknown; restored from __doc__ 699 """ 700 x.__delslice__(i, j) <==> del x[i:j] 701 702 Use of negative indices is not supported. 703 """ 704 pass 705 706 def __eq__(self, y): # real signature unknown; restored from __doc__ 707 """ x.__eq__(y) <==> x==y """ 708 pass 709 710 def __getattribute__(self, name): # real signature unknown; restored from __doc__ 711 """ x.__getattribute__('name') <==> x.name """ 712 pass 713 714 def __getitem__(self, y): # real signature unknown; restored from __doc__ 715 """ x.__getitem__(y) <==> x[y] """ 716 pass 717 718 def __getslice__(self, i, j): # real signature unknown; restored from __doc__ 719 """ 720 x.__getslice__(i, j) <==> x[i:j] 721 722 Use of negative indices is not supported. 723 """ 724 pass 725 726 def __ge__(self, y): # real signature unknown; restored from __doc__ 727 """ x.__ge__(y) <==> x>=y """ 728 pass 729 730 def __gt__(self, y): # real signature unknown; restored from __doc__ 731 """ x.__gt__(y) <==> x>y """ 732 pass 733 734 def __iadd__(self, y): # real signature unknown; restored from __doc__ 735 """ x.__iadd__(y) <==> x+=y """ 736 pass 737 738 def __imul__(self, y): # real signature unknown; restored from __doc__ 739 """ x.__imul__(y) <==> x*=y """ 740 pass 741 742 def __init__(self, seq=()): # known special case of list.__init__ 743 """ 744 list() -> new empty list 745 list(iterable) -> new list initialized from iterable's items 746 # (copied from class doc) 747 """ 748 pass 749 750 def __iter__(self): # real signature unknown; restored from __doc__ 751 """ x.__iter__() <==> iter(x) """ 752 pass 753 754 def __len__(self): # real signature unknown; restored from __doc__ 755 """ x.__len__() <==> len(x) """ 756 pass 757 758 def __le__(self, y): # real signature unknown; restored from __doc__ 759 """ x.__le__(y) <==> x<=y """ 760 pass 761 762 def __lt__(self, y): # real signature unknown; restored from __doc__ 763 """ x.__lt__(y) <==> x<y """ 764 pass 765 766 def __mul__(self, n): # real signature unknown; restored from __doc__ 767 """ x.__mul__(n) <==> x*n """ 768 pass 769 770 @staticmethod # known case of __new__ 771 def __new__(S, *more): # real signature unknown; restored from __doc__ 772 """ T.__new__(S, ...) -> a new object with type S, a subtype of T """ 773 pass 774 775 def __ne__(self, y): # real signature unknown; restored from __doc__ 776 """ x.__ne__(y) <==> x!=y """ 777 pass 778 779 def __repr__(self): # real signature unknown; restored from __doc__ 780 """ x.__repr__() <==> repr(x) """ 781 pass 782 783 def __reversed__(self): # real signature unknown; restored from __doc__ 784 """ L.__reversed__() -- return a reverse iterator over the list """ 785 pass 786 787 def __rmul__(self, n): # real signature unknown; restored from __doc__ 788 """ x.__rmul__(n) <==> n*x """ 789 pass 790 791 def __setitem__(self, i, y): # real signature unknown; restored from __doc__ 792 """ x.__setitem__(i, y) <==> x[i]=y """ 793 pass 794 795 def __setslice__(self, i, j, y): # real signature unknown; restored from __doc__ 796 """ 797 x.__setslice__(i, j, y) <==> x[i:j]=y 798 799 Use of negative indices is not supported. 800 """ 801 pass 802 803 def __sizeof__(self): # real signature unknown; restored from __doc__ 804 """ L.__sizeof__() -- size of L in memory, in bytes """ 805 pass 806 807 __hash__ = None 808 809 810 class long(object): 811 """ 812 long(x=0) -> long 813 long(x, base=10) -> long 814 815 Convert a number or string to a long integer, or return 0L if no arguments 816 are given. If x is floating point, the conversion truncates towards zero. 817 818 If x is not a number or if base is given, then x must be a string or 819 Unicode object representing an integer literal in the given base. The 820 literal can be preceded by '+' or '-' and be surrounded by whitespace. 821 The base defaults to 10. Valid bases are 0 and 2-36. Base 0 means to 822 interpret the base from the string as an integer literal. 823 >>> int('0b100', base=0) 824 4L 825 """ 826 def bit_length(self): # real signature unknown; restored from __doc__ 827 """ 828 long.bit_length() -> int or long 829 830 Number of bits necessary to represent self in binary. 831 >>> bin(37L) 832 '0b100101' 833 >>> (37L).bit_length() 834 6 835 """ 836 return 0 837 838 def conjugate(self, *args, **kwargs): # real signature unknown 839 """ Returns self, the complex conjugate of any long. """ 840 pass 841 842 def __abs__(self): # real signature unknown; restored from __doc__ 843 """ x.__abs__() <==> abs(x) """ 844 pass 845 846 def __add__(self, y): # real signature unknown; restored from __doc__ 847 """ x.__add__(y) <==> x+y """ 848 pass 849 850 def __and__(self, y): # real signature unknown; restored from __doc__ 851 """ x.__and__(y) <==> x&y """ 852 pass 853 854 def __cmp__(self, y): # real signature unknown; restored from __doc__ 855 """ x.__cmp__(y) <==> cmp(x,y) """ 856 pass 857 858 def __coerce__(self, y): # real signature unknown; restored from __doc__ 859 """ x.__coerce__(y) <==> coerce(x, y) """ 860 pass 861 862 def __divmod__(self, y): # real signature unknown; restored from __doc__ 863 """ x.__divmod__(y) <==> divmod(x, y) """ 864 pass 865 866 def __div__(self, y): # real signature unknown; restored from __doc__ 867 """ x.__div__(y) <==> x/y """ 868 pass 869 870 def __float__(self): # real signature unknown; restored from __doc__ 871 """ x.__float__() <==> float(x) """ 872 pass 873 874 def __floordiv__(self, y): # real signature unknown; restored from __doc__ 875 """ x.__floordiv__(y) <==> x//y """ 876 pass 877 878 def __format__(self, *args, **kwargs): # real signature unknown 879 pass 880 881 def __getattribute__(self, name): # real signature unknown; restored from __doc__ 882 """ x.__getattribute__('name') <==> x.name """ 883 pass 884 885 def __getnewargs__(self, *args, **kwargs): # real signature unknown 886 pass 887 888 def __hash__(self): # real signature unknown; restored from __doc__ 889 """ x.__hash__() <==> hash(x) """ 890 pass 891 892 def __hex__(self): # real signature unknown; restored from __doc__ 893 """ x.__hex__() <==> hex(x) """ 894 pass 895 896 def __index__(self): # real signature unknown; restored from __doc__ 897 """ x[y:z] <==> x[y.__index__():z.__index__()] """ 898 pass 899 900 def __init__(self, x=0): # real signature unknown; restored from __doc__ 901 pass 902 903 def __int__(self): # real signature unknown; restored from __doc__ 904 """ x.__int__() <==> int(x) """ 905 pass 906 907 def __invert__(self): # real signature unknown; restored from __doc__ 908 """ x.__invert__() <==> ~x """ 909 pass 910 911 def __long__(self): # real signature unknown; restored from __doc__ 912 """ x.__long__() <==> long(x) """ 913 pass 914 915 def __lshift__(self, y): # real signature unknown; restored from __doc__ 916 """ x.__lshift__(y) <==> x<<y """ 917 pass 918 919 def __mod__(self, y): # real signature unknown; restored from __doc__ 920 """ x.__mod__(y) <==> x%y """ 921 pass 922 923 def __mul__(self, y): # real signature unknown; restored from __doc__ 924 """ x.__mul__(y) <==> x*y """ 925 pass 926 927 def __neg__(self): # real signature unknown; restored from __doc__ 928 """ x.__neg__() <==> -x """ 929 pass 930 931 @staticmethod # known case of __new__ 932 def __new__(S, *more): # real signature unknown; restored from __doc__ 933 """ T.__new__(S, ...) -> a new object with type S, a subtype of T """ 934 pass 935 936 def __nonzero__(self): # real signature unknown; restored from __doc__ 937 """ x.__nonzero__() <==> x != 0 """ 938 pass 939 940 def __oct__(self): # real signature unknown; restored from __doc__ 941 """ x.__oct__() <==> oct(x) """ 942 pass 943 944 def __or__(self, y): # real signature unknown; restored from __doc__ 945 """ x.__or__(y) <==> x|y """ 946 pass 947 948 def __pos__(self): # real signature unknown; restored from __doc__ 949 """ x.__pos__() <==> +x """ 950 pass 951 952 def __pow__(self, y, z=None): # real signature unknown; restored from __doc__ 953 """ x.__pow__(y[, z]) <==> pow(x, y[, z]) """ 954 pass 955 956 def __radd__(self, y): # real signature unknown; restored from __doc__ 957 """ x.__radd__(y) <==> y+x """ 958 pass 959 960 def __rand__(self, y): # real signature unknown; restored from __doc__ 961 """ x.__rand__(y) <==> y&x """ 962 pass 963 964 def __rdivmod__(self, y): # real signature unknown; restored from __doc__ 965 """ x.__rdivmod__(y) <==> divmod(y, x) """ 966 pass 967 968 def __rdiv__(self, y): # real signature unknown; restored from __doc__ 969 """ x.__rdiv__(y) <==> y/x """ 970 pass 971 972 def __repr__(self): # real signature unknown; restored from __doc__ 973 """ x.__repr__() <==> repr(x) """ 974 pass 975 976 def __rfloordiv__(self, y): # real signature unknown; restored from __doc__ 977 """ x.__rfloordiv__(y) <==> y//x """ 978 pass 979 980 def __rlshift__(self, y): # real signature unknown; restored from __doc__ 981 """ x.__rlshift__(y) <==> y<<x """ 982 pass 983 984 def __rmod__(self, y): # real signature unknown; restored from __doc__ 985 """ x.__rmod__(y) <==> y%x """ 986 pass 987 988 def __rmul__(self, y): # real signature unknown; restored from __doc__ 989 """ x.__rmul__(y) <==> y*x """ 990 pass 991 992 def __ror__(self, y): # real signature unknown; restored from __doc__ 993 """ x.__ror__(y) <==> y|x """ 994 pass 995 996 def __rpow__(self, x, z=None): # real signature unknown; restored from __doc__ 997 """ y.__rpow__(x[, z]) <==> pow(x, y[, z]) """ 998 pass 999 1000 def __rrshift__(self, y): # real signature unknown; restored from __doc__ 1001 """ x.__rrshift__(y) <==> y>>x """ 1002 pass 1003 1004 def __rshift__(self, y): # real signature unknown; restored from __doc__ 1005 """ x.__rshift__(y) <==> x>>y """ 1006 pass 1007 1008 def __rsub__(self, y): # real signature unknown; restored from __doc__ 1009 """ x.__rsub__(y) <==> y-x """ 1010 pass 1011 1012 def __rtruediv__(self, y): # real signature unknown; restored from __doc__ 1013 """ x.__rtruediv__(y) <==> y/x """ 1014 pass 1015 1016 def __rxor__(self, y): # real signature unknown; restored from __doc__ 1017 """ x.__rxor__(y) <==> y^x """ 1018 pass 1019 1020 def __sizeof__(self, *args, **kwargs): # real signature unknown 1021 """ Returns size in memory, in bytes """ 1022 pass 1023 1024 def __str__(self): # real signature unknown; restored from __doc__ 1025 """ x.__str__() <==> str(x) """ 1026 pass 1027 1028 def __sub__(self, y): # real signature unknown; restored from __doc__ 1029 """ x.__sub__(y) <==> x-y """ 1030 pass 1031 1032 def __truediv__(self, y): # real signature unknown; restored from __doc__ 1033 """ x.__truediv__(y) <==> x/y """ 1034 pass 1035 1036 def __trunc__(self, *args, **kwargs): # real signature unknown 1037 """ Truncating an Integral returns itself. """ 1038 pass 1039 1040 def __xor__(self, y): # real signature unknown; restored from __doc__ 1041 """ x.__xor__(y) <==> x^y """ 1042 pass 1043 1044 denominator = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 1045 """the denominator of a rational number in lowest terms""" 1046 1047 imag = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 1048 """the imaginary part of a complex number""" 1049 1050 numerator = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 1051 """the numerator of a rational number in lowest terms""" 1052 1053 real = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 1054 """the real part of a complex number""" 1055 1056 1057 1058 class memoryview(object): 1059 """ 1060 memoryview(object) 1061 1062 Create a new memoryview object which references the given object. 1063 """ 1064 def tobytes(self, *args, **kwargs): # real signature unknown 1065 pass 1066 1067 def tolist(self, *args, **kwargs): # real signature unknown 1068 pass 1069 1070 def __delitem__(self, y): # real signature unknown; restored from __doc__ 1071 """ x.__delitem__(y) <==> del x[y] """ 1072 pass 1073 1074 def __eq__(self, y): # real signature unknown; restored from __doc__ 1075 """ x.__eq__(y) <==> x==y """ 1076 pass 1077 1078 def __getattribute__(self, name): # real signature unknown; restored from __doc__ 1079 """ x.__getattribute__('name') <==> x.name """ 1080 pass 1081 1082 def __getitem__(self, y): # real signature unknown; restored from __doc__ 1083 """ x.__getitem__(y) <==> x[y] """ 1084 pass 1085 1086 def __ge__(self, y): # real signature unknown; restored from __doc__ 1087 """ x.__ge__(y) <==> x>=y """ 1088 pass 1089 1090 def __gt__(self, y): # real signature unknown; restored from __doc__ 1091 """ x.__gt__(y) <==> x>y """ 1092 pass 1093 1094 def __init__(self, p_object): # real signature unknown; restored from __doc__ 1095 pass 1096 1097 def __len__(self): # real signature unknown; restored from __doc__ 1098 """ x.__len__() <==> len(x) """ 1099 pass 1100 1101 def __le__(self, y): # real signature unknown; restored from __doc__ 1102 """ x.__le__(y) <==> x<=y """ 1103 pass 1104 1105 def __lt__(self, y): # real signature unknown; restored from __doc__ 1106 """ x.__lt__(y) <==> x<y """ 1107 pass 1108 1109 @staticmethod # known case of __new__ 1110 def __new__(S, *more): # real signature unknown; restored from __doc__ 1111 """ T.__new__(S, ...) -> a new object with type S, a subtype of T """ 1112 pass 1113 1114 def __ne__(self, y): # real signature unknown; restored from __doc__ 1115 """ x.__ne__(y) <==> x!=y """ 1116 pass 1117 1118 def __repr__(self): # real signature unknown; restored from __doc__ 1119 """ x.__repr__() <==> repr(x) """ 1120 pass 1121 1122 def __setitem__(self, i, y): # real signature unknown; restored from __doc__ 1123 """ x.__setitem__(i, y) <==> x[i]=y """ 1124 pass 1125 1126 format = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 1127 1128 itemsize = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 1129 1130 ndim = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 1131 1132 readonly = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 1133 1134 shape = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 1135 1136 strides = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 1137 1138 suboffsets = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 1139 1140 1141 1142 class property(object): 1143 """ 1144 property(fget=None, fset=None, fdel=None, doc=None) -> property attribute 1145 1146 fget is a function to be used for getting an attribute value, and likewise 1147 fset is a function for setting, and fdel a function for del'ing, an 1148 attribute. Typical use is to define a managed attribute x: 1149 1150 class C(object): 1151 def getx(self): return self._x 1152 def setx(self, value): self._x = value 1153 def delx(self): del self._x 1154 x = property(getx, setx, delx, "I'm the 'x' property.") 1155 1156 Decorators make defining new properties or modifying existing ones easy: 1157 1158 class C(object): 1159 @property 1160 def x(self): 1161 "I am the 'x' property." 1162 return self._x 1163 @x.setter 1164 def x(self, value): 1165 self._x = value 1166 @x.deleter 1167 def x(self): 1168 del self._x 1169 """ 1170 def deleter(self, *args, **kwargs): # real signature unknown 1171 """ Descriptor to change the deleter on a property. """ 1172 pass 1173 1174 def getter(self, *args, **kwargs): # real signature unknown 1175 """ Descriptor to change the getter on a property. """ 1176 pass 1177 1178 def setter(self, *args, **kwargs): # real signature unknown 1179 """ Descriptor to change the setter on a property. """ 1180 pass 1181 1182 def __delete__(self, obj): # real signature unknown; restored from __doc__ 1183 """ descr.__delete__(obj) """ 1184 pass 1185 1186 def __getattribute__(self, name): # real signature unknown; restored from __doc__ 1187 """ x.__getattribute__('name') <==> x.name """ 1188 pass 1189 1190 def __get__(self, obj, type=None): # real signature unknown; restored from __doc__ 1191 """ descr.__get__(obj[, type]) -> value """ 1192 pass 1193 1194 def __init__(self, fget=None, fset=None, fdel=None, doc=None): # known special case of property.__init__ 1195 """ 1196 property(fget=None, fset=None, fdel=None, doc=None) -> property attribute 1197 1198 fget is a function to be used for getting an attribute value, and likewise 1199 fset is a function for setting, and fdel a function for del'ing, an 1200 attribute. Typical use is to define a managed attribute x: 1201 1202 class C(object): 1203 def getx(self): return self._x 1204 def setx(self, value): self._x = value 1205 def delx(self): del self._x 1206 x = property(getx, setx, delx, "I'm the 'x' property.") 1207 1208 Decorators make defining new properties or modifying existing ones easy: 1209 1210 class C(object): 1211 @property 1212 def x(self): 1213 "I am the 'x' property." 1214 return self._x 1215 @x.setter 1216 def x(self, value): 1217 self._x = value 1218 @x.deleter 1219 def x(self): 1220 del self._x 1221 1222 # (copied from class doc) 1223 """ 1224 pass 1225 1226 @staticmethod # known case of __new__ 1227 def __new__(S, *more): # real signature unknown; restored from __doc__ 1228 """ T.__new__(S, ...) -> a new object with type S, a subtype of T """ 1229 pass 1230 1231 def __set__(self, obj, value): # real signature unknown; restored from __doc__ 1232 """ descr.__set__(obj, value) """ 1233 pass 1234 1235 fdel = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 1236 1237 fget = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 1238 1239 fset = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 1240 1241 1242 1243 class reversed(object): 1244 """ 1245 reversed(sequence) -> reverse iterator over values of the sequence 1246 1247 Return a reverse iterator 1248 """ 1249 def next(self): # real signature unknown; restored from __doc__ 1250 """ x.next() -> the next value, or raise StopIteration """ 1251 pass 1252 1253 def __getattribute__(self, name): # real signature unknown; restored from __doc__ 1254 """ x.__getattribute__('name') <==> x.name """ 1255 pass 1256 1257 def __init__(self, sequence): # real signature unknown; restored from __doc__ 1258 pass 1259 1260 def __iter__(self): # real signature unknown; restored from __doc__ 1261 """ x.__iter__() <==> iter(x) """ 1262 pass 1263 1264 def __length_hint__(self, *args, **kwargs): # real signature unknown 1265 """ Private method returning an estimate of len(list(it)). """ 1266 pass 1267 1268 @staticmethod # known case of __new__ 1269 def __new__(S, *more): # real signature unknown; restored from __doc__ 1270 """ T.__new__(S, ...) -> a new object with type S, a subtype of T """ 1271 pass 1272 1273 1274 class set(object): 1275 """ 1276 set() -> new empty set object 1277 set(iterable) -> new set object 1278 1279 Build an unordered collection of unique elements. 1280 """ 1281 def add(self, *args, **kwargs): # real signature unknown 1282 """ 1283 Add an element to a set. 1284 1285 This has no effect if the element is already present. 1286 """ 1287 pass 1288 1289 def clear(self, *args, **kwargs): # real signature unknown 1290 """ Remove all elements from this set. """ 1291 pass 1292 1293 def copy(self, *args, **kwargs): # real signature unknown 1294 """ Return a shallow copy of a set. """ 1295 pass 1296 1297 def difference(self, *args, **kwargs): # real signature unknown 1298 """ 1299 Return the difference of two or more sets as a new set. 1300 1301 (i.e. all elements that are in this set but not the others.) 1302 """ 1303 pass 1304 1305 def difference_update(self, *args, **kwargs): # real signature unknown 1306 """ Remove all elements of another set from this set. """ 1307 pass 1308 1309 def discard(self, *args, **kwargs): # real signature unknown 1310 """ 1311 Remove an element from a set if it is a member. 1312 1313 If the element is not a member, do nothing. 1314 """ 1315 pass 1316 1317 def intersection(self, *args, **kwargs): # real signature unknown 1318 """ 1319 Return the intersection of two or more sets as a new set. 1320 1321 (i.e. elements that are common to all of the sets.) 1322 """ 1323 pass 1324 1325 def intersection_update(self, *args, **kwargs): # real signature unknown 1326 """ Update a set with the intersection of itself and another. """ 1327 pass 1328 1329 def isdisjoint(self, *args, **kwargs): # real signature unknown 1330 """ Return True if two sets have a null intersection. """ 1331 pass 1332 1333 def issubset(self, *args, **kwargs): # real signature unknown 1334 """ Report whether another set contains this set. """ 1335 pass 1336 1337 def issuperset(self, *args, **kwargs): # real signature unknown 1338 """ Report whether this set contains another set. """ 1339 pass 1340 1341 def pop(self, *args, **kwargs): # real signature unknown 1342 """ 1343 Remove and return an arbitrary set element. 1344 Raises KeyError if the set is empty. 1345 """ 1346 pass 1347 1348 def remove(self, *args, **kwargs): # real signature unknown 1349 """ 1350 Remove an element from a set; it must be a member. 1351 1352 If the element is not a member, raise a KeyError. 1353 """ 1354 pass 1355 1356 def symmetric_difference(self, *args, **kwargs): # real signature unknown 1357 """ 1358 Return the symmetric difference of two sets as a new set. 1359 1360 (i.e. all elements that are in exactly one of the sets.) 1361 """ 1362 pass 1363 1364 def symmetric_difference_update(self, *args, **kwargs): # real signature unknown 1365 """ Update a set with the symmetric difference of itself and another. """ 1366 pass 1367 1368 def union(self, *args, **kwargs): # real signature unknown 1369 """ 1370 Return the union of sets as a new set. 1371 1372 (i.e. all elements that are in either set.) 1373 """ 1374 pass 1375 1376 def update(self, *args, **kwargs): # real signature unknown 1377 """ Update a set with the union of itself and others. """ 1378 pass 1379 1380 def __and__(self, y): # real signature unknown; restored from __doc__ 1381 """ x.__and__(y) <==> x&y """ 1382 pass 1383 1384 def __cmp__(self, y): # real signature unknown; restored from __doc__ 1385 """ x.__cmp__(y) <==> cmp(x,y) """ 1386 pass 1387 1388 def __contains__(self, y): # real signature unknown; restored from __doc__ 1389 """ x.__contains__(y) <==> y in x. """ 1390 pass 1391 1392 def __eq__(self, y): # real signature unknown; restored from __doc__ 1393 """ x.__eq__(y) <==> x==y """ 1394 pass 1395 1396 def __getattribute__(self, name): # real signature unknown; restored from __doc__ 1397 """ x.__getattribute__('name') <==> x.name """ 1398 pass 1399 1400 def __ge__(self, y): # real signature unknown; restored from __doc__ 1401 """ x.__ge__(y) <==> x>=y """ 1402 pass 1403 1404 def __gt__(self, y): # real signature unknown; restored from __doc__ 1405 """ x.__gt__(y) <==> x>y """ 1406 pass 1407 1408 def __iand__(self, y): # real signature unknown; restored from __doc__ 1409 """ x.__iand__(y) <==> x&=y """ 1410 pass 1411 1412 def __init__(self, seq=()): # known special case of set.__init__ 1413 """ 1414 set() -> new empty set object 1415 set(iterable) -> new set object 1416 1417 Build an unordered collection of unique elements. 1418 # (copied from class doc) 1419 """ 1420 pass 1421 1422 def __ior__(self, y): # real signature unknown; restored from __doc__ 1423 """ x.__ior__(y) <==> x|=y """ 1424 pass 1425 1426 def __isub__(self, y): # real signature unknown; restored from __doc__ 1427 """ x.__isub__(y) <==> x-=y """ 1428 pass 1429 1430 def __iter__(self): # real signature unknown; restored from __doc__ 1431 """ x.__iter__() <==> iter(x) """ 1432 pass 1433 1434 def __ixor__(self, y): # real signature unknown; restored from __doc__ 1435 """ x.__ixor__(y) <==> x^=y """ 1436 pass 1437 1438 def __len__(self): # real signature unknown; restored from __doc__ 1439 """ x.__len__() <==> len(x) """ 1440 pass 1441 1442 def __le__(self, y): # real signature unknown; restored from __doc__ 1443 """ x.__le__(y) <==> x<=y """ 1444 pass 1445 1446 def __lt__(self, y): # real signature unknown; restored from __doc__ 1447 """ x.__lt__(y) <==> x<y """ 1448 pass 1449 1450 @staticmethod # known case of __new__ 1451 def __new__(S, *more): # real signature unknown; restored from __doc__ 1452 """ T.__new__(S, ...) -> a new object with type S, a subtype of T """ 1453 pass 1454 1455 def __ne__(self, y): # real signature unknown; restored from __doc__ 1456 """ x.__ne__(y) <==> x!=y """ 1457 pass 1458 1459 def __or__(self, y): # real signature unknown; restored from __doc__ 1460 """ x.__or__(y) <==> x|y """ 1461 pass 1462 1463 def __rand__(self, y): # real signature unknown; restored from __doc__ 1464 """ x.__rand__(y) <==> y&x """ 1465 pass 1466 1467 def __reduce__(self, *args, **kwargs): # real signature unknown 1468 """ Return state information for pickling. """ 1469 pass 1470 1471 def __repr__(self): # real signature unknown; restored from __doc__ 1472 """ x.__repr__() <==> repr(x) """ 1473 pass 1474 1475 def __ror__(self, y): # real signature unknown; restored from __doc__ 1476 """ x.__ror__(y) <==> y|x """ 1477 pass 1478 1479 def __rsub__(self, y): # real signature unknown; restored from __doc__ 1480 """ x.__rsub__(y) <==> y-x """ 1481 pass 1482 1483 def __rxor__(self, y): # real signature unknown; restored from __doc__ 1484 """ x.__rxor__(y) <==> y^x """ 1485 pass 1486 1487 def __sizeof__(self): # real signature unknown; restored from __doc__ 1488 """ S.__sizeof__() -> size of S in memory, in bytes """ 1489 pass 1490 1491 def __sub__(self, y): # real signature unknown; restored from __doc__ 1492 """ x.__sub__(y) <==> x-y """ 1493 pass 1494 1495 def __xor__(self, y): # real signature unknown; restored from __doc__ 1496 """ x.__xor__(y) <==> x^y """ 1497 pass 1498 1499 __hash__ = None 1500 1501 1502 class slice(object): 1503 """ 1504 slice(stop) 1505 slice(start, stop[, step]) 1506 1507 Create a slice object. This is used for extended slicing (e.g. a[0:10:2]). 1508 """ 1509 def indices(self, len): # real signature unknown; restored from __doc__ 1510 """ 1511 S.indices(len) -> (start, stop, stride) 1512 1513 Assuming a sequence of length len, calculate the start and stop 1514 indices, and the stride length of the extended slice described by 1515 S. Out of bounds indices are clipped in a manner consistent with the 1516 handling of normal slices. 1517 """ 1518 pass 1519 1520 def __cmp__(self, y): # real signature unknown; restored from __doc__ 1521 """ x.__cmp__(y) <==> cmp(x,y) """ 1522 pass 1523 1524 def __getattribute__(self, name): # real signature unknown; restored from __doc__ 1525 """ x.__getattribute__('name') <==> x.name """ 1526 pass 1527 1528 def __hash__(self): # real signature unknown; restored from __doc__ 1529 """ x.__hash__() <==> hash(x) """ 1530 pass 1531 1532 def __init__(self, stop): # real signature unknown; restored from __doc__ 1533 pass 1534 1535 @staticmethod # known case of __new__ 1536 def __new__(S, *more): # real signature unknown; restored from __doc__ 1537 """ T.__new__(S, ...) -> a new object with type S, a subtype of T """ 1538 pass 1539 1540 def __reduce__(self, *args, **kwargs): # real signature unknown 1541 """ Return state information for pickling. """ 1542 pass 1543 1544 def __repr__(self): # real signature unknown; restored from __doc__ 1545 """ x.__repr__() <==> repr(x) """ 1546 pass 1547 1548 start = property(lambda self: 0) 1549 """:type: int""" 1550 1551 step = property(lambda self: 0) 1552 """:type: int""" 1553 1554 stop = property(lambda self: 0) 1555 """:type: int""" 1556 1557 1558 1559 class staticmethod(object): 1560 """ 1561 staticmethod(function) -> method 1562 1563 Convert a function to be a static method. 1564 1565 A static method does not receive an implicit first argument. 1566 To declare a static method, use this idiom: 1567 1568 class C: 1569 def f(arg1, arg2, ...): ... 1570 f = staticmethod(f) 1571 1572 It can be called either on the class (e.g. C.f()) or on an instance 1573 (e.g. C().f()). The instance is ignored except for its class. 1574 1575 Static methods in Python are similar to those found in Java or C++. 1576 For a more advanced concept, see the classmethod builtin. 1577 """ 1578 def __getattribute__(self, name): # real signature unknown; restored from __doc__ 1579 """ x.__getattribute__('name') <==> x.name """ 1580 pass 1581 1582 def __get__(self, obj, type=None): # real signature unknown; restored from __doc__ 1583 """ descr.__get__(obj[, type]) -> value """ 1584 pass 1585 1586 def __init__(self, function): # real signature unknown; restored from __doc__ 1587 pass 1588 1589 @staticmethod # known case of __new__ 1590 def __new__(S, *more): # real signature unknown; restored from __doc__ 1591 """ T.__new__(S, ...) -> a new object with type S, a subtype of T """ 1592 pass 1593 1594 __func__ = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 1595 1596 1597 1598 class super(object): 1599 """ 1600 super(type, obj) -> bound super object; requires isinstance(obj, type) 1601 super(type) -> unbound super object 1602 super(type, type2) -> bound super object; requires issubclass(type2, type) 1603 Typical use to call a cooperative superclass method: 1604 class C(B): 1605 def meth(self, arg): 1606 super(C, self).meth(arg) 1607 """ 1608 def __getattribute__(self, name): # real signature unknown; restored from __doc__ 1609 """ x.__getattribute__('name') <==> x.name """ 1610 pass 1611 1612 def __get__(self, obj, type=None): # real signature unknown; restored from __doc__ 1613 """ descr.__get__(obj[, type]) -> value """ 1614 pass 1615 1616 def __init__(self, type1, type2=None): # known special case of super.__init__ 1617 """ 1618 super(type, obj) -> bound super object; requires isinstance(obj, type) 1619 super(type) -> unbound super object 1620 super(type, type2) -> bound super object; requires issubclass(type2, type) 1621 Typical use to call a cooperative superclass method: 1622 class C(B): 1623 def meth(self, arg): 1624 super(C, self).meth(arg) 1625 # (copied from class doc) 1626 """ 1627 pass 1628 1629 @staticmethod # known case of __new__ 1630 def __new__(S, *more): # real signature unknown; restored from __doc__ 1631 """ T.__new__(S, ...) -> a new object with type S, a subtype of T """ 1632 pass 1633 1634 def __repr__(self): # real signature unknown; restored from __doc__ 1635 """ x.__repr__() <==> repr(x) """ 1636 pass 1637 1638 __self_class__ = property(lambda self: type(object)) 1639 """the type of the instance invoking super(); may be None 1640 1641 :type: type 1642 """ 1643 1644 __self__ = property(lambda self: type(object)) 1645 """the instance invoking super(); may be None 1646 1647 :type: type 1648 """ 1649 1650 __thisclass__ = property(lambda self: type(object)) 1651 """the class invoking super() 1652 1653 :type: type 1654 """ 1655 1656 1657 1658 class tuple(object): 1659 """ 1660 tuple() -> empty tuple 1661 tuple(iterable) -> tuple initialized from iterable's items 1662 1663 If the argument is a tuple, the return value is the same object. 1664 """ 1665 def count(self, value): # real signature unknown; restored from __doc__ 1666 """ T.count(value) -> integer -- return number of occurrences of value """ 1667 return 0 1668 1669 def index(self, value, start=None, stop=None): # real signature unknown; restored from __doc__ 1670 """ 1671 T.index(value, [start, [stop]]) -> integer -- return first index of value. 1672 Raises ValueError if the value is not present. 1673 """ 1674 return 0 1675 1676 def __add__(self, y): # real signature unknown; restored from __doc__ 1677 """ x.__add__(y) <==> x+y """ 1678 pass 1679 1680 def __contains__(self, y): # real signature unknown; restored from __doc__ 1681 """ x.__contains__(y) <==> y in x """ 1682 pass 1683 1684 def __eq__(self, y): # real signature unknown; restored from __doc__ 1685 """ x.__eq__(y) <==> x==y """ 1686 pass 1687 1688 def __getattribute__(self, name): # real signature unknown; restored from __doc__ 1689 """ x.__getattribute__('name') <==> x.name """ 1690 pass 1691 1692 def __getitem__(self, y): # real signature unknown; restored from __doc__ 1693 """ x.__getitem__(y) <==> x[y] """ 1694 pass 1695 1696 def __getnewargs__(self, *args, **kwargs): # real signature unknown 1697 pass 1698 1699 def __getslice__(self, i, j): # real signature unknown; restored from __doc__ 1700 """ 1701 x.__getslice__(i, j) <==> x[i:j] 1702 1703 Use of negative indices is not supported. 1704 """ 1705 pass 1706 1707 def __ge__(self, y): # real signature unknown; restored from __doc__ 1708 """ x.__ge__(y) <==> x>=y """ 1709 pass 1710 1711 def __gt__(self, y): # real signature unknown; restored from __doc__ 1712 """ x.__gt__(y) <==> x>y """ 1713 pass 1714 1715 def __hash__(self): # real signature unknown; restored from __doc__ 1716 """ x.__hash__() <==> hash(x) """ 1717 pass 1718 1719 def __init__(self, seq=()): # known special case of tuple.__init__ 1720 """ 1721 tuple() -> empty tuple 1722 tuple(iterable) -> tuple initialized from iterable's items 1723 1724 If the argument is a tuple, the return value is the same object. 1725 # (copied from class doc) 1726 """ 1727 pass 1728 1729 def __iter__(self): # real signature unknown; restored from __doc__ 1730 """ x.__iter__() <==> iter(x) """ 1731 pass 1732 1733 def __len__(self): # real signature unknown; restored from __doc__ 1734 """ x.__len__() <==> len(x) """ 1735 pass 1736 1737 def __le__(self, y): # real signature unknown; restored from __doc__ 1738 """ x.__le__(y) <==> x<=y """ 1739 pass 1740 1741 def __lt__(self, y): # real signature unknown; restored from __doc__ 1742 """ x.__lt__(y) <==> x<y """ 1743 pass 1744 1745 def __mul__(self, n): # real signature unknown; restored from __doc__ 1746 """ x.__mul__(n) <==> x*n """ 1747 pass 1748 1749 @staticmethod # known case of __new__ 1750 def __new__(S, *more): # real signature unknown; restored from __doc__ 1751 """ T.__new__(S, ...) -> a new object with type S, a subtype of T """ 1752 pass 1753 1754 def __ne__(self, y): # real signature unknown; restored from __doc__ 1755 """ x.__ne__(y) <==> x!=y """ 1756 pass 1757 1758 def __repr__(self): # real signature unknown; restored from __doc__ 1759 """ x.__repr__() <==> repr(x) """ 1760 pass 1761 1762 def __rmul__(self, n): # real signature unknown; restored from __doc__ 1763 """ x.__rmul__(n) <==> n*x """ 1764 pass 1765 1766 1767 class type(object): 1768 """ 1769 type(object) -> the object's type 1770 type(name, bases, dict) -> a new type 1771 """ 1772 def mro(self): # real signature unknown; restored from __doc__ 1773 """ 1774 mro() -> list 1775 return a type's method resolution order 1776 """ 1777 return [] 1778 1779 def __call__(self, *more): # real signature unknown; restored from __doc__ 1780 """ x.__call__(...) <==> x(...) """ 1781 pass 1782 1783 def __delattr__(self, name): # real signature unknown; restored from __doc__ 1784 """ x.__delattr__('name') <==> del x.name """ 1785 pass 1786 1787 def __eq__(self, y): # real signature unknown; restored from __doc__ 1788 """ x.__eq__(y) <==> x==y """ 1789 pass 1790 1791 def __getattribute__(self, name): # real signature unknown; restored from __doc__ 1792 """ x.__getattribute__('name') <==> x.name """ 1793 pass 1794 1795 def __ge__(self, y): # real signature unknown; restored from __doc__ 1796 """ x.__ge__(y) <==> x>=y """ 1797 pass 1798 1799 def __gt__(self, y): # real signature unknown; restored from __doc__ 1800 """ x.__gt__(y) <==> x>y """ 1801 pass 1802 1803 def __hash__(self): # real signature unknown; restored from __doc__ 1804 """ x.__hash__() <==> hash(x) """ 1805 pass 1806 1807 def __init__(cls, what, bases=None, dict=None): # known special case of type.__init__ 1808 """ 1809 type(object) -> the object's type 1810 type(name, bases, dict) -> a new type 1811 # (copied from class doc) 1812 """ 1813 pass 1814 1815 def __instancecheck__(self): # real signature unknown; restored from __doc__ 1816 """ 1817 __instancecheck__() -> bool 1818 check if an object is an instance 1819 """ 1820 return False 1821 1822 def __le__(self, y): # real signature unknown; restored from __doc__ 1823 """ x.__le__(y) <==> x<=y """ 1824 pass 1825 1826 def __lt__(self, y): # real signature unknown; restored from __doc__ 1827 """ x.__lt__(y) <==> x<y """ 1828 pass 1829 1830 @staticmethod # known case of __new__ 1831 def __new__(S, *more): # real signature unknown; restored from __doc__ 1832 """ T.__new__(S, ...) -> a new object with type S, a subtype of T """ 1833 pass 1834 1835 def __ne__(self, y): # real signature unknown; restored from __doc__ 1836 """ x.__ne__(y) <==> x!=y """ 1837 pass 1838 1839 def __repr__(self): # real signature unknown; restored from __doc__ 1840 """ x.__repr__() <==> repr(x) """ 1841 pass 1842 1843 def __setattr__(self, name, value): # real signature unknown; restored from __doc__ 1844 """ x.__setattr__('name', value) <==> x.name = value """ 1845 pass 1846 1847 def __subclasscheck__(self): # real signature unknown; restored from __doc__ 1848 """ 1849 __subclasscheck__() -> bool 1850 check if a class is a subclass 1851 """ 1852 return False 1853 1854 def __subclasses__(self): # real signature unknown; restored from __doc__ 1855 """ __subclasses__() -> list of immediate subclasses """ 1856 return [] 1857 1858 __abstractmethods__ = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 1859 1860 1861 __bases__ = ( 1862 object, 1863 ) 1864 __base__ = object 1865 __basicsize__ = 436 1866 __dictoffset__ = 132 1867 __dict__ = None # (!) real value is '' 1868 __flags__ = -2146544149 1869 __itemsize__ = 20 1870 __mro__ = ( 1871 None, # (!) forward: type, real value is '' 1872 object, 1873 ) 1874 __name__ = 'type' 1875 __weakrefoffset__ = 184 1876 1877 1878 class unicode(basestring): 1879 """ 1880 unicode(object='') -> unicode object 1881 unicode(string[, encoding[, errors]]) -> unicode object 1882 1883 Create a new Unicode object from the given encoded string. 1884 encoding defaults to the current default string encoding. 1885 errors can be 'strict', 'replace' or 'ignore' and defaults to 'strict'. 1886 """ 1887 def capitalize(self): # real signature unknown; restored from __doc__ 1888 """ 1889 S.capitalize() -> unicode 1890 1891 Return a capitalized version of S, i.e. make the first character 1892 have upper case and the rest lower case. 1893 """ 1894 return u"" 1895 1896 def center(self, width, fillchar=None): # real signature unknown; restored from __doc__ 1897 """ 1898 S.center(width[, fillchar]) -> unicode 1899 1900 Return S centered in a Unicode string of length width. Padding is 1901 done using the specified fill character (default is a space) 1902 """ 1903 return u"" 1904 1905 def count(self, sub, start=None, end=None): # real signature unknown; restored from __doc__ 1906 """ 1907 S.count(sub[, start[, end]]) -> int 1908 1909 Return the number of non-overlapping occurrences of substring sub in 1910 Unicode string S[start:end]. Optional arguments start and end are 1911 interpreted as in slice notation. 1912 """ 1913 return 0 1914 1915 def decode(self, encoding=None, errors=None): # real signature unknown; restored from __doc__ 1916 """ 1917 S.decode([encoding[,errors]]) -> string or unicode 1918 1919 Decodes S using the codec registered for encoding. encoding defaults 1920 to the default encoding. errors may be given to set a different error 1921 handling scheme. Default is 'strict' meaning that encoding errors raise 1922 a UnicodeDecodeError. Other possible values are 'ignore' and 'replace' 1923 as well as any other name registered with codecs.register_error that is 1924 able to handle UnicodeDecodeErrors. 1925 """ 1926 return "" 1927 1928 def encode(self, encoding=None, errors=None): # real signature unknown; restored from __doc__ 1929 """ 1930 S.encode([encoding[,errors]]) -> string or unicode 1931 1932 Encodes S using the codec registered for encoding. encoding defaults 1933 to the default encoding. errors may be given to set a different error 1934 handling scheme. Default is 'strict' meaning that encoding errors raise 1935 a UnicodeEncodeError. Other possible values are 'ignore', 'replace' and 1936 'xmlcharrefreplace' as well as any other name registered with 1937 codecs.register_error that can handle UnicodeEncodeErrors. 1938 """ 1939 return "" 1940 1941 def endswith(self, suffix, start=None, end=None): # real signature unknown; restored from __doc__ 1942 """ 1943 S.endswith(suffix[, start[, end]]) -> bool 1944 1945 Return True if S ends with the specified suffix, False otherwise. 1946 With optional start, test S beginning at that position. 1947 With optional end, stop comparing S at that position. 1948 suffix can also be a tuple of strings to try. 1949 """ 1950 return False 1951 1952 def expandtabs(self, tabsize=None): # real signature unknown; restored from __doc__ 1953 """ 1954 S.expandtabs([tabsize]) -> unicode 1955 1956 Return a copy of S where all tab characters are expanded using spaces. 1957 If tabsize is not given, a tab size of 8 characters is assumed. 1958 """ 1959 return u"" 1960 1961 def find(self, sub, start=None, end=None): # real signature unknown; restored from __doc__ 1962 """ 1963 S.find(sub [,start [,end]]) -> int 1964 1965 Return the lowest index in S where substring sub is found, 1966 such that sub is contained within S[start:end]. Optional 1967 arguments start and end are interpreted as in slice notation. 1968 1969 Return -1 on failure. 1970 """ 1971 return 0 1972 1973 def format(*args, **kwargs): # known special case of unicode.format 1974 """ 1975 S.format(*args, **kwargs) -> unicode 1976 1977 Return a formatted version of S, using substitutions from args and kwargs. 1978 The substitutions are identified by braces ('{' and '}'). 1979 """ 1980 pass 1981 1982 def index(self, sub, start=None, end=None): # real signature unknown; restored from __doc__ 1983 """ 1984 S.index(sub [,start [,end]]) -> int 1985 1986 Like S.find() but raise ValueError when the substring is not found. 1987 """ 1988 return 0 1989 1990 def isalnum(self): # real signature unknown; restored from __doc__ 1991 """ 1992 S.isalnum() -> bool 1993 1994 Return True if all characters in S are alphanumeric 1995 and there is at least one character in S, False otherwise. 1996 """ 1997 return False 1998 1999 def isalpha(self): # real signature unknown; restored from __doc__ 2000 """ 2001 S.isalpha() -> bool 2002 2003 Return True if all characters in S are alphabetic 2004 and there is at least one character in S, False otherwise. 2005 """ 2006 return False 2007 2008 def isdecimal(self): # real signature unknown; restored from __doc__ 2009 """ 2010 S.isdecimal() -> bool 2011 2012 Return True if there are only decimal characters in S, 2013 False otherwise. 2014 """ 2015 return False 2016 2017 def isdigit(self): # real signature unknown; restored from __doc__ 2018 """ 2019 S.isdigit() -> bool 2020 2021 Return True if all characters in S are digits 2022 and there is at least one character in S, False otherwise. 2023 """ 2024 return False 2025 2026 def islower(self): # real signature unknown; restored from __doc__ 2027 """ 2028 S.islower() -> bool 2029 2030 Return True if all cased characters in S are lowercase and there is 2031 at least one cased character in S, False otherwise. 2032 """ 2033 return False 2034 2035 def isnumeric(self): # real signature unknown; restored from __doc__ 2036 """ 2037 S.isnumeric() -> bool 2038 2039 Return True if there are only numeric characters in S, 2040 False otherwise. 2041 """ 2042 return False 2043 2044 def isspace(self): # real signature unknown; restored from __doc__ 2045 """ 2046 S.isspace() -> bool 2047 2048 Return True if all characters in S are whitespace 2049 and there is at least one character in S, False otherwise. 2050 """ 2051 return False 2052 2053 def istitle(self): # real signature unknown; restored from __doc__ 2054 """ 2055 S.istitle() -> bool 2056 2057 Return True if S is a titlecased string and there is at least one 2058 character in S, i.e. upper- and titlecase characters may only 2059 follow uncased characters and lowercase characters only cased ones. 2060 Return False otherwise. 2061 """ 2062 return False 2063 2064 def isupper(self): # real signature unknown; restored from __doc__ 2065 """ 2066 S.isupper() -> bool 2067 2068 Return True if all cased characters in S are uppercase and there is 2069 at least one cased character in S, False otherwise. 2070 """ 2071 return False 2072 2073 def join(self, iterable): # real signature unknown; restored from __doc__ 2074 """ 2075 S.join(iterable) -> unicode 2076 2077 Return a string which is the concatenation of the strings in the 2078 iterable. The separator between elements is S. 2079 """ 2080 return u"" 2081 2082 def ljust(self, width, fillchar=None): # real signature unknown; restored from __doc__ 2083 """ 2084 S.ljust(width[, fillchar]) -> int 2085 2086 Return S left-justified in a Unicode string of length width. Padding is 2087 done using the specified fill character (default is a space). 2088 """ 2089 return 0 2090 2091 def lower(self): # real signature unknown; restored from __doc__ 2092 """ 2093 S.lower() -> unicode 2094 2095 Return a copy of the string S converted to lowercase. 2096 """ 2097 return u"" 2098 2099 def lstrip(self, chars=None): # real signature unknown; restored from __doc__ 2100 """ 2101 S.lstrip([chars]) -> unicode 2102 2103 Return a copy of the string S with leading whitespace removed. 2104 If chars is given and not None, remove characters in chars instead. 2105 If chars is a str, it will be converted to unicode before stripping 2106 """ 2107 return u"" 2108 2109 def partition(self, sep): # real signature unknown; restored from __doc__ 2110 """ 2111 S.partition(sep) -> (head, sep, tail) 2112 2113 Search for the separator sep in S, and return the part before it, 2114 the separator itself, and the part after it. If the separator is not 2115 found, return S and two empty strings. 2116 """ 2117 pass 2118 2119 def replace(self, old, new, count=None): # real signature unknown; restored from __doc__ 2120 """ 2121 S.replace(old, new[, count]) -> unicode 2122 2123 Return a copy of S with all occurrences of substring 2124 old replaced by new. If the optional argument count is 2125 given, only the first count occurrences are replaced. 2126 """ 2127 return u"" 2128 2129 def rfind(self, sub, start=None, end=None): # real signature unknown; restored from __doc__ 2130 """ 2131 S.rfind(sub [,start [,end]]) -> int 2132 2133 Return the highest index in S where substring sub is found, 2134 such that sub is contained within S[start:end]. Optional 2135 arguments start and end are interpreted as in slice notation. 2136 2137 Return -1 on failure. 2138 """ 2139 return 0 2140 2141 def rindex(self, sub, start=None, end=None): # real signature unknown; restored from __doc__ 2142 """ 2143 S.rindex(sub [,start [,end]]) -> int 2144 2145 Like S.rfind() but raise ValueError when the substring is not found. 2146 """ 2147 return 0 2148 2149 def rjust(self, width, fillchar=None): # real signature unknown; restored from __doc__ 2150 """ 2151 S.rjust(width[, fillchar]) -> unicode 2152 2153 Return S right-justified in a Unicode string of length width. Padding is 2154 done using the specified fill character (default is a space). 2155 """ 2156 return u"" 2157 2158 def rpartition(self, sep): # real signature unknown; restored from __doc__ 2159 """ 2160 S.rpartition(sep) -> (head, sep, tail) 2161 2162 Search for the separator sep in S, starting at the end of S, and return 2163 the part before it, the separator itself, and the part after it. If the 2164 separator is not found, return two empty strings and S. 2165 """ 2166 pass 2167 2168 def rsplit(self, sep=None, maxsplit=None): # real signature unknown; restored from __doc__ 2169 """ 2170 S.rsplit([sep [,maxsplit]]) -> list of strings 2171 2172 Return a list of the words in S, using sep as the 2173 delimiter string, starting at the end of the string and 2174 working to the front. If maxsplit is given, at most maxsplit 2175 splits are done. If sep is not specified, any whitespace string 2176 is a separator. 2177 """ 2178 return [] 2179 2180 def rstrip(self, chars=None): # real signature unknown; restored from __doc__ 2181 """ 2182 S.rstrip([chars]) -> unicode 2183 2184 Return a copy of the string S with trailing whitespace removed. 2185 If chars is given and not None, remove characters in chars instead. 2186 If chars is a str, it will be converted to unicode before stripping 2187 """ 2188 return u"" 2189 2190 def split(self, sep=None, maxsplit=None): # real signature unknown; restored from __doc__ 2191 """ 2192 S.split([sep [,maxsplit]]) -> list of strings 2193 2194 Return a list of the words in S, using sep as the 2195 delimiter string. If maxsplit is given, at most maxsplit 2196 splits are done. If sep is not specified or is None, any 2197 whitespace string is a separator and empty strings are 2198 removed from the result. 2199 """ 2200 return [] 2201 2202 def splitlines(self, keepends=False): # real signature unknown; restored from __doc__ 2203 """ 2204 S.splitlines(keepends=False) -> list of strings 2205 2206 Return a list of the lines in S, breaking at line boundaries. 2207 Line breaks are not included in the resulting list unless keepends 2208 is given and true. 2209 """ 2210 return [] 2211 2212 def startswith(self, prefix, start=None, end=None): # real signature unknown; restored from __doc__ 2213 """ 2214 S.startswith(prefix[, start[, end]]) -> bool 2215 2216 Return True if S starts with the specified prefix, False otherwise. 2217 With optional start, test S beginning at that position. 2218 With optional end, stop comparing S at that position. 2219 prefix can also be a tuple of strings to try. 2220 """ 2221 return False 2222 2223 def strip(self, chars=None): # real signature unknown; restored from __doc__ 2224 """ 2225 S.strip([chars]) -> unicode 2226 2227 Return a copy of the string S with leading and trailing 2228 whitespace removed. 2229 If chars is given and not None, remove characters in chars instead. 2230 If chars is a str, it will be converted to unicode before stripping 2231 """ 2232 return u"" 2233 2234 def swapcase(self): # real signature unknown; restored from __doc__ 2235 """ 2236 S.swapcase() -> unicode 2237 2238 Return a copy of S with uppercase characters converted to lowercase 2239 and vice versa. 2240 """ 2241 return u"" 2242 2243 def title(self): # real signature unknown; restored from __doc__ 2244 """ 2245 S.title() -> unicode 2246 2247 Return a titlecased version of S, i.e. words start with title case 2248 characters, all remaining cased characters have lower case. 2249 """ 2250 return u"" 2251 2252 def translate(self, table): # real signature unknown; restored from __doc__ 2253 """ 2254 S.translate(table) -> unicode 2255 2256 Return a copy of the string S, where all characters have been mapped 2257 through the given translation table, which must be a mapping of 2258 Unicode ordinals to Unicode ordinals, Unicode strings or None. 2259 Unmapped characters are left untouched. Characters mapped to None 2260 are deleted. 2261 """ 2262 return u"" 2263 2264 def upper(self): # real signature unknown; restored from __doc__ 2265 """ 2266 S.upper() -> unicode 2267 2268 Return a copy of S converted to uppercase. 2269 """ 2270 return u"" 2271 2272 def zfill(self, width): # real signature unknown; restored from __doc__ 2273 """ 2274 S.zfill(width) -> unicode 2275 2276 Pad a numeric string S with zeros on the left, to fill a field 2277 of the specified width. The string S is never truncated. 2278 """ 2279 return u"" 2280 2281 def _formatter_field_name_split(self, *args, **kwargs): # real signature unknown 2282 pass 2283 2284 def _formatter_parser(self, *args, **kwargs): # real signature unknown 2285 pass 2286 2287 def __add__(self, y): # real signature unknown; restored from __doc__ 2288 """ x.__add__(y) <==> x+y """ 2289 pass 2290 2291 def __contains__(self, y): # real signature unknown; restored from __doc__ 2292 """ x.__contains__(y) <==> y in x """ 2293 pass 2294 2295 def __eq__(self, y): # real signature unknown; restored from __doc__ 2296 """ x.__eq__(y) <==> x==y """ 2297 pass 2298 2299 def __format__(self, format_spec): # real signature unknown; restored from __doc__ 2300 """ 2301 S.__format__(format_spec) -> unicode 2302 2303 Return a formatted version of S as described by format_spec. 2304 """ 2305 return u"" 2306 2307 def __getattribute__(self, name): # real signature unknown; restored from __doc__ 2308 """ x.__getattribute__('name') <==> x.name """ 2309 pass 2310 2311 def __getitem__(self, y): # real signature unknown; restored from __doc__ 2312 """ x.__getitem__(y) <==> x[y] """ 2313 pass 2314 2315 def __getnewargs__(self, *args, **kwargs): # real signature unknown 2316 pass 2317 2318 def __getslice__(self, i, j): # real signature unknown; restored from __doc__ 2319 """ 2320 x.__getslice__(i, j) <==> x[i:j] 2321 2322 Use of negative indices is not supported. 2323 """ 2324 pass 2325 2326 def __ge__(self, y): # real signature unknown; restored from __doc__ 2327 """ x.__ge__(y) <==> x>=y """ 2328 pass 2329 2330 def __gt__(self, y): # real signature unknown; restored from __doc__ 2331 """ x.__gt__(y) <==> x>y """ 2332 pass 2333 2334 def __hash__(self): # real signature unknown; restored from __doc__ 2335 """ x.__hash__() <==> hash(x) """ 2336 pass 2337 2338 def __init__(self, string=u'', encoding=None, errors='strict'): # known special case of unicode.__init__ 2339 """ 2340 unicode(object='') -> unicode object 2341 unicode(string[, encoding[, errors]]) -> unicode object 2342 2343 Create a new Unicode object from the given encoded string. 2344 encoding defaults to the current default string encoding. 2345 errors can be 'strict', 'replace' or 'ignore' and defaults to 'strict'. 2346 # (copied from class doc) 2347 """ 2348 pass 2349 2350 def __len__(self): # real signature unknown; restored from __doc__ 2351 """ x.__len__() <==> len(x) """ 2352 pass 2353 2354 def __le__(self, y): # real signature unknown; restored from __doc__ 2355 """ x.__le__(y) <==> x<=y """ 2356 pass 2357 2358 def __lt__(self, y): # real signature unknown; restored from __doc__ 2359 """ x.__lt__(y) <==> x<y """ 2360 pass 2361 2362 def __mod__(self, y): # real signature unknown; restored from __doc__ 2363 """ x.__mod__(y) <==> x%y """ 2364 pass 2365 2366 def __mul__(self, n): # real signature unknown; restored from __doc__ 2367 """ x.__mul__(n) <==> x*n """ 2368 pass 2369 2370 @staticmethod # known case of __new__ 2371 def __new__(S, *more): # real signature unknown; restored from __doc__ 2372 """ T.__new__(S, ...) -> a new object with type S, a subtype of T """ 2373 pass 2374 2375 def __ne__(self, y): # real signature unknown; restored from __doc__ 2376 """ x.__ne__(y) <==> x!=y """ 2377 pass 2378 2379 def __repr__(self): # real signature unknown; restored from __doc__ 2380 """ x.__repr__() <==> repr(x) """ 2381 pass 2382 2383 def __rmod__(self, y): # real signature unknown; restored from __doc__ 2384 """ x.__rmod__(y) <==> y%x """ 2385 pass 2386 2387 def __rmul__(self, n): # real signature unknown; restored from __doc__ 2388 """ x.__rmul__(n) <==> n*x """ 2389 pass 2390 2391 def __sizeof__(self): # real signature unknown; restored from __doc__ 2392 """ S.__sizeof__() -> size of S in memory, in bytes """ 2393 pass 2394 2395 def __str__(self): # real signature unknown; restored from __doc__ 2396 """ x.__str__() <==> str(x) """ 2397 pass 2398 2399 2400 class xrange(object): 2401 """ 2402 xrange(stop) -> xrange object 2403 xrange(start, stop[, step]) -> xrange object 2404 2405 Like range(), but instead of returning a list, returns an object that 2406 generates the numbers in the range on demand. For looping, this is 2407 slightly faster than range() and more memory efficient. 2408 """ 2409 def __getattribute__(self, name): # real signature unknown; restored from __doc__ 2410 """ x.__getattribute__('name') <==> x.name """ 2411 pass 2412 2413 def __getitem__(self, y): # real signature unknown; restored from __doc__ 2414 """ x.__getitem__(y) <==> x[y] """ 2415 pass 2416 2417 def __init__(self, stop): # real signature unknown; restored from __doc__ 2418 pass 2419 2420 def __iter__(self): # real signature unknown; restored from __doc__ 2421 """ x.__iter__() <==> iter(x) """ 2422 pass 2423 2424 def __len__(self): # real signature unknown; restored from __doc__ 2425 """ x.__len__() <==> len(x) """ 2426 pass 2427 2428 @staticmethod # known case of __new__ 2429 def __new__(S, *more): # real signature unknown; restored from __doc__ 2430 """ T.__new__(S, ...) -> a new object with type S, a subtype of T """ 2431 pass 2432 2433 def __reduce__(self, *args, **kwargs): # real signature unknown 2434 pass 2435 2436 def __repr__(self): # real signature unknown; restored from __doc__ 2437 """ x.__repr__() <==> repr(x) """ 2438 pass 2439 2440 def __reversed__(self, *args, **kwargs): # real signature unknown 2441 """ Returns a reverse iterator. """ 2442 pass 2443 2444 2445 # variables with complex values 2446 2447 Ellipsis = None # (!) real value is '' 2448 2449 NotImplemented = None # (!) real value is ''
具体方法举例如下:
1 file = open('log','r+') 2 file.close() #关闭文件 3 file.flush() #刷新文件内部缓冲区 4 file.next() #获取下一行数据,不存在,则报错 5 file.read() #读取指定字节数据 6 file.readline() #仅读取一行数据 7 file.readline() #仅读取一行数据 8 file.seek() #指定文件中指针位置 9 file.tell() #获取当前指针位置 10 file.truncate() #截断数据,仅保留指定之前数据 11 file.write() #写内容 12 file.readlines() #将一个字符串列表写入文件 13 file.xreadlines() #可用于逐行读取文件,非全部
常用文件打开方式
with open('文件',‘模式’) as f #内部会自动关闭并释放文件资源。
新功能:
2.7之后支持同时进行多个文件的打开操作
with
open
(
'log1'
) as obj1,
open
(
'log2'
) as obj2:
6函数
- 函数式:将某功能代码封装到函数中,日后便无需重复编写,仅调用函数即可
- 面向对象:对函数进行分类和封装,让开发“更快更好更强...”
函数式编程最重要的是增强代码的重用性和可读性
简单邮件告警
1 import smtplib 2 from email.mime.text import MIMEText 3 from email.utils import formataddr 4 msg = MIMEText('邮件内容', 'plain', 'utf-8') 5 msg['From'] = formataddr(["xxx名字",'发件人邮箱']) 6 msg['To'] = formataddr(["走人",'收件人邮箱']) 7 msg['Subject'] = "主题" 8 server = smtplib.SMTP("smtp.126.com", 25) 9 10 server.login("发件人邮箱", "发件人邮箱密码") 11 12 server.sendmail('发件人邮箱', ['收件人邮箱',], msg.as_string()) 13 14 server.quit()
函数:
1 def func(arg): 2 return arg 3 4执行: func()
1 def 定义函数关键字
2 函数名,日后通过函数名调用函数
3 函数声明,不自动执行,调用后才执行
4 函数的参数
5 函数的返回值
关于返回值:
1 return 返回值 2 1,未明确制定返回值,则返回None 3 2,返回值可以赋值给某个变量 4 5 6 8 #!/usr/bin/env python 9 # -*- coding:utf-8 -*- 10 def func(arg): 11 return True 12 def cc(arg): 13 print 'ok' 14 15 16 ret = func('33') #赋值 17 print ret #输出 18 19 20 bb = cc('kk') #同上 21 print bb 22 23 #输出的结果 24 True #return有返回值 25 ok 26 None #没有return,也就没有返回值,所以返回none
介绍函数的参数
1形式参数,就是一个形式而已,
例如def func(message):
pass
2实际参数,在函数调用时传入的参数
例如:
func('cpu报警')
3.默认参数
1,不传,则使用默认
2,默认参数,必须放到最后可以有多个
1 #!/usr/bin/env python 2 # -*- coding:utf-8 -*- 3 def func(arg='123'): 4 return arg 5 --------------------------------------------------------------------------------------- 6 #!/usr/bin/env python 7 # -*- coding:utf-8 -*- 8 def func(arg='123'): 9 return arg 10 def cc(arg): 11 print 'ok' 12 13 ret = func('456') #赋值 14 print ret #输出 15 #输出 16 456 17 ret = func() 18 print ret 19 #输出默认的 20 123
4.动态参数:
def func(*args):
print args
接收多个参数,内部自动构造元祖,序列 * 避免内部构造元祖

1 #!/usr/bin/env python 2 # -*- coding:utf-8 -*- 3 def func(*args): 4 print args 5 6 a=(1,2,3,4,5) 7 li = [11,22,33] 8 func(*li) 9 func(li) 10 func(a) 11 func(*a) 12 #输出如下:---------》在传入参数的时候序列加上 * 避免内部构造元祖 13 (11, 22, 33) 14 ([11, 22, 33],) 15 ((1, 2, 3, 4, 5),) 16 (1, 2, 3, 4, 5)
动态参数二
1 #!/usr/bin/env python 2 # -*- coding:utf-8 -*- 3 def func(**kwarg): 4 print kwarg 5 func(k1=123,k2='sss') 6 7 dic={'k1':123,'k2':'sss'} 8 #传入字典 9 func(**dic) 10 #输出 11 {'k2': 'sss', 'k1': 123} 12 {'k2': 'sss', 'k1': 123}