大二这么久了,第一个学期课程实在太少,而且全都不是CS的专业课,无奈,若是自己不勤奋一点,过了半年,用不到半年,就又变回小白了。所以自己最近也在重新巩固数据结构的知识。刚好看到二叉树这里,觉得二叉树应用广泛,复习的比较细致。
刚开始就是二叉树的建立了,大多数地方都是先序的递归创建,于是便想着试试非递归的代码,倒腾了个把小时,基本上弄出代码了,本渣渣水平有限,有错误的地方,望路过的大神不吝赐教~~不胜感激!
主要的思路是:
按照先序的顺序输入二叉树,若是遇到空子树,用字符‘#‘表示,例如
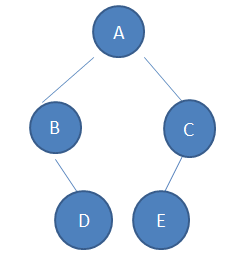
( 1)若第一次输入就是’#‘,则创建的是空树。
(2)每次创建一个新的结点p,看栈顶元素的flag,若为0,则取出(不出栈)栈顶指针R,并将其flag修改为1.R->child=p;
若flag本身就是1,则直接取栈顶(出栈)!R-rchild=p;
然后将p入栈。入栈的flag初始值是0;
(3)当栈为空时,二叉树就建好了。
代码:
1 #include<stdio.h> 2 #include<iostream> 3 #include<malloc.h> 4 using namespace std; 5 typedef struct BiTNode 6 { 7 char c; 8 struct BiTNode *lchild,*rchild; 9 }BiTNode,*BiTree; 10 typedef struct 11 { 12 BiTree x; 13 int flag; 14 }snode; 15 typedef struct 16 { 17 snode sdata[100]; 18 int top; 19 }Stack; 20 int Empty(Stack s) 21 { 22 if(s.top==-1) return 1; 23 else return 0; 24 } 25 void Pop(Stack &s,BiTree R) //取栈顶元素,flag=1标志已经被取过一次 26 { 27 R=s.sdata[s.top].x; 28 s.sdata[s.top].flag=1; 29 } 30 void Out(Stack &s,BiTree R) //取栈顶元素,并且栈顶元素出栈 31 { 32 R=s.sdata[s.top].x; 33 s.top--; 34 } 35 void In(Stack &s,BiTree R) //指针入栈,flag=0标志从未被取过 36 { 37 s.top++; 38 s.sdata[s.top].x=R; 39 s.sdata[s.top].flag=0; 40 } 41 void CreateBiTree(BiTree T) 42 { 43 char ch; 44 Stack s; 45 T=NULL; 46 s.top=-1; 47 BiTree R=T,p; 48 do 49 { 50 cin>>ch; 51 if(Empty(s)&&ch=='#') 52 return; 53 else if(ch!='#') 54 { 55 p=(BiTree)malloc(sizeof(BiTNode)); 56 p->c=ch; 57 if(Empty(s)) 58 { 59 T=p; 60 R=p; 61 } 62 else if(s.sdata[s.top].flag==0) 63 { 64 Pop(s,R); 65 R->lchild=p; 66 } 67 else 68 { 69 Out(s,R); 70 R->rchild=p; 71 } 72 In(s,p); 73 } 74 else 75 { 76 if(s.sdata[s.top].flag==0) 77 { 78 Pop(s,R); 79 80 R->lchild=NULL; 81 } 82 else 83 { 84 Out(s,R); 85 R->rchild=NULL; 86 } 87 } 88 }while(s.top!=-1); 89 } 90 int main() 91 { 92 BiTree T; 93 CreateBiTree(T); 94 }