1003 Emergency (25分)
As an emergency rescue team leader of a city, you are given a special map of your country. The map shows several scattered cities connected by some roads. Amount of rescue teams in each city and the length of each road between any pair of cities are marked on the map. When there is an emergency call to you from some other city, your job is to lead your men to the place as quickly as possible, and at the mean time, call up as many hands on the way as possible.
Input Specification:
Each input file contains one test case. For each test case, the first line contains 4 positive integers: N (≤) - the number of cities (and the cities are numbered from 0 to N−1), M - the number of roads, C1 and C2 - the cities that you are currently in and that you must save, respectively. The next line contains N integers, where the i-th integer is the number of rescue teams in the i-th city. Then M lines follow, each describes a road with three integers c1, c2 and L, which are the pair of cities connected by a road and the length of that road, respectively. It is guaranteed that there exists at least one path from C1 to C2.
Output Specification:
For each test case, print in one line two numbers: the number of different shortest paths between C1 and C2, and the maximum amount of rescue teams you can possibly gather. All the numbers in a line must be separated by exactly one space, and there is no extra space allowed at the end of a line.
Sample Input:
5 6 0 2
1 2 1 5 3
0 1 1
0 2 2
0 3 1
1 2 1
2 4 1
3 4 1
Sample Output:
2 4
思路
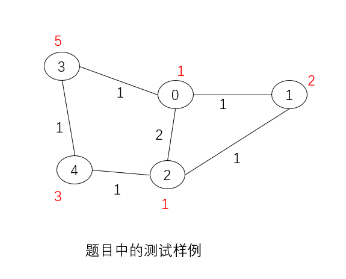
1 #include <iostream> 2 #include <cstdio> 3 #include <vector> 4 #include <cstring> 5 6 using namespace std; 7 8 #define INF 1000000000 9 10 int N, M, C1, C2; 11 int nums[510]; 12 int cost[510][510]; 13 int vis[510]; 14 vector<int> adjList[510]; //邻接表 15 int minCost = INF; // 最短路的代价 16 int maxNum = -1; // 最大的人数 17 int minCnt; // 最短路的数量 18 19 20 void dfs(int start, int c, int num) 21 { 22 // 剪枝,如果当前代价已经大于最小代价则返回,这里不剪枝也能过 23 if(c > minCost) 24 return; 25 26 if(start == C2) 27 { 28 if(minCost > c) 29 { 30 minCost = c; 31 minCnt = 1; 32 // maxNum此时要强制更新,不要用if判断,当时就是这里出错,找了很久很久的错因... 33 maxNum = num; 34 } 35 else if(minCost == c) 36 { 37 minCnt++; 38 // 这里一开始也写到 if(minCost > c) 里面去了,导致还有最后几个测试用例没过,找了很久很久原因... 39 if(maxNum < num) 40 maxNum = num; 41 } 42 43 44 return; 45 } 46 47 for(int i = 0; i < adjList[start].size(); ++i) 48 { 49 if(!vis[adjList[start][i]]) 50 { 51 vis[adjList[start][i]] = 1; 52 dfs(adjList[start][i], c+cost[start][adjList[start][i]], num+nums[adjList[start][i]]); 53 vis[adjList[start][i]] = 0; 54 } 55 } 56 57 } 58 59 60 int main() 61 { 62 63 scanf("%d%d%d%d", &N, &M, &C1, &C2); 64 for(int i = 0; i < N; ++i) 65 scanf("%d", &nums[i]); 66 67 int c1, c2, L; 68 for(int i = 0; i < M; ++i) 69 { 70 scanf("%d%d%d", &c1, &c2, &L); 71 72 // 处理重复输入同一路径,但代价不同的情况 73 if(cost[c1][c2] == 0) 74 { 75 cost[c1][c2] = cost[c2][c1] = L; 76 adjList[c1].push_back(c2); 77 adjList[c2].push_back(c1); 78 } 79 else if(cost[c1][c2] > L) 80 { 81 cost[c1][c2] = cost[c2][c1] = L; 82 } 83 84 } 85 86 vis[C1] = 1; 87 dfs(C1, 0, nums[C1]); 88 89 cout << minCnt << ' ' << maxNum; 90 91 return 0; 92 }