Description
Assume the coasting is an infinite straight line. Land is in one side of coasting, sea in the other. Each small island is a point locating in the sea side. And any radar installation, locating on the coasting, can only cover d distance, so an island in the sea can be covered by a radius installation, if the distance between them is at most d.
We use Cartesian coordinate system, defining the coasting is the x-axis. The sea side is above x-axis, and the land side below. Given the position of each island in the sea, and given the distance of the coverage of the radar installation, your task is to write a program to find the minimal number of radar installations to cover all the islands. Note that the position of an island is represented by its x-y coordinates.
Figure A Sample Input of Radar Installations
We use Cartesian coordinate system, defining the coasting is the x-axis. The sea side is above x-axis, and the land side below. Given the position of each island in the sea, and given the distance of the coverage of the radar installation, your task is to write a program to find the minimal number of radar installations to cover all the islands. Note that the position of an island is represented by its x-y coordinates.
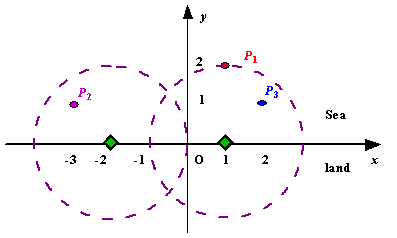
Figure A Sample Input of Radar Installations
Input
The
input consists of several test cases. The first line of each case
contains two integers n (1<=n<=1000) and d, where n is the number
of islands in the sea and d is the distance of coverage of the radar
installation. This is followed by n lines each containing two integers
representing the coordinate of the position of each island. Then a blank
line follows to separate the cases.
The input is terminated by a line containing pair of zeros
The input is terminated by a line containing pair of zeros
Output
For
each test case output one line consisting of the test case number
followed by the minimal number of radar installations needed. "-1"
installation means no solution for that case.
Sample Input
3 2 1 2 -3 1 2 1 1 2 0 2 0 0
Sample Output
Case 1: 2 Case 2: 1
题解:贪心
按x从小到大排序
首先是要卡边界
对于当前点,如果能被之前的覆盖就直接覆盖
如果不能,那么如果覆盖当前点的圆心(能覆盖到的最右端)的横坐标小于当前圆心横坐标,那么就更新当前圆心坐标,否则ans++
1 #include<iostream> 2 #include<cstdio> 3 #include<cstdlib> 4 #include<cstring> 5 #include<algorithm> 6 #include<cmath> 7 #define ll long long 8 using namespace std; 9 10 const int N = 1010; 11 12 int n,d,ans,tot,flg; 13 double pos; 14 15 struct Node { 16 double x,y; 17 bool operator < (const Node a) const { 18 return x<a.x; 19 } 20 }p[N]; 21 22 int gi() { 23 int x=0,o=1; char ch=getchar(); 24 while(ch!='-' && (ch<'0' || ch>'9')) ch=getchar(); 25 if(ch=='-') o=-1,ch=getchar(); 26 while(ch>='0' && ch<='9') x=x*10+ch-'0',ch=getchar(); 27 return o*x; 28 } 29 30 int main() { 31 while(scanf("%d%d", &n, &d) && n+d) { 32 ans=1,tot++,flg=0; 33 for(int i=1; i<=n; i++) { 34 p[i].x=gi(),p[i].y=gi(); 35 } 36 for(int i=1; i<=n; i++) { 37 if(p[i].y>d) {flg=1;break;} 38 } 39 if(flg) {printf("Case %d: %d ",tot,-1);continue;} 40 sort(p+1,p+n+1); 41 pos=p[1].x+sqrt(d*d-p[1].y*p[1].y); 42 for(int i=2; i<=n; i++) { 43 if((pos-p[i].x)*(pos-p[i].x)+p[i].y*p[i].y<=d*d) continue; 44 double pos1=p[i].x+sqrt(d*d-p[i].y*p[i].y); 45 if(pos1>pos) ans++; 46 pos=pos1; 47 } 48 printf("Case %d: %d ",tot,ans); 49 } 50 return 0; 51 }