1.分布式简介 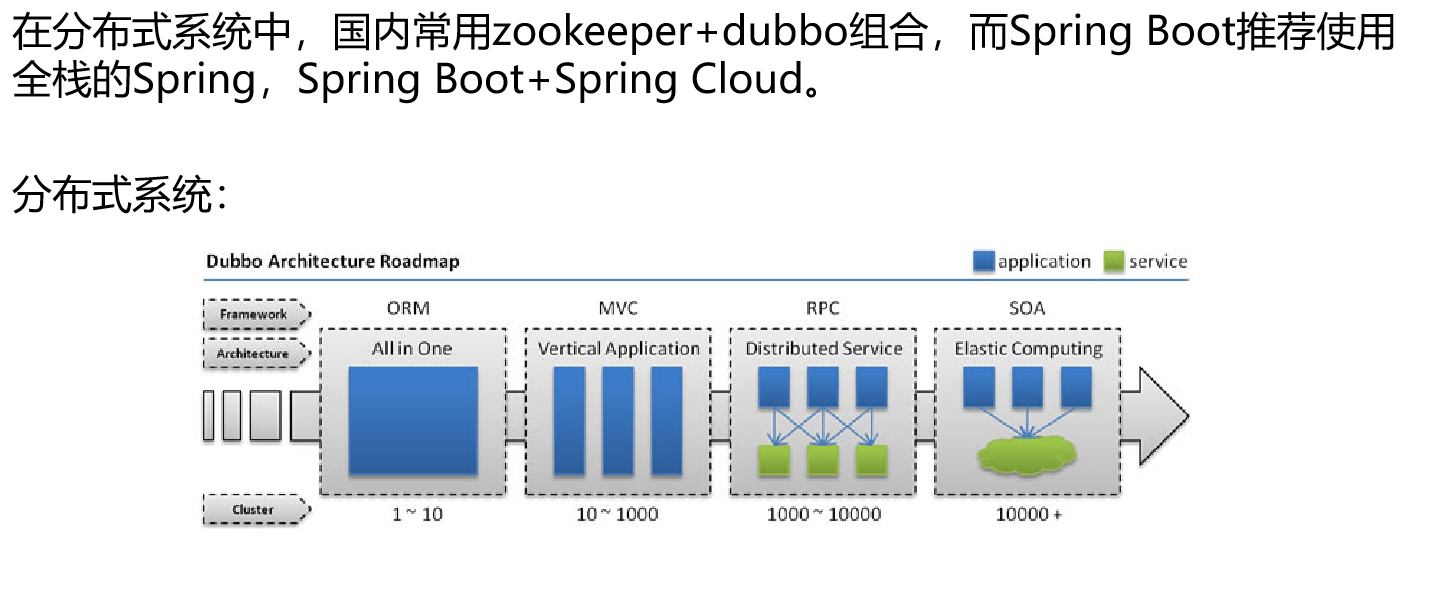
2.Zookeeper和Dubbo 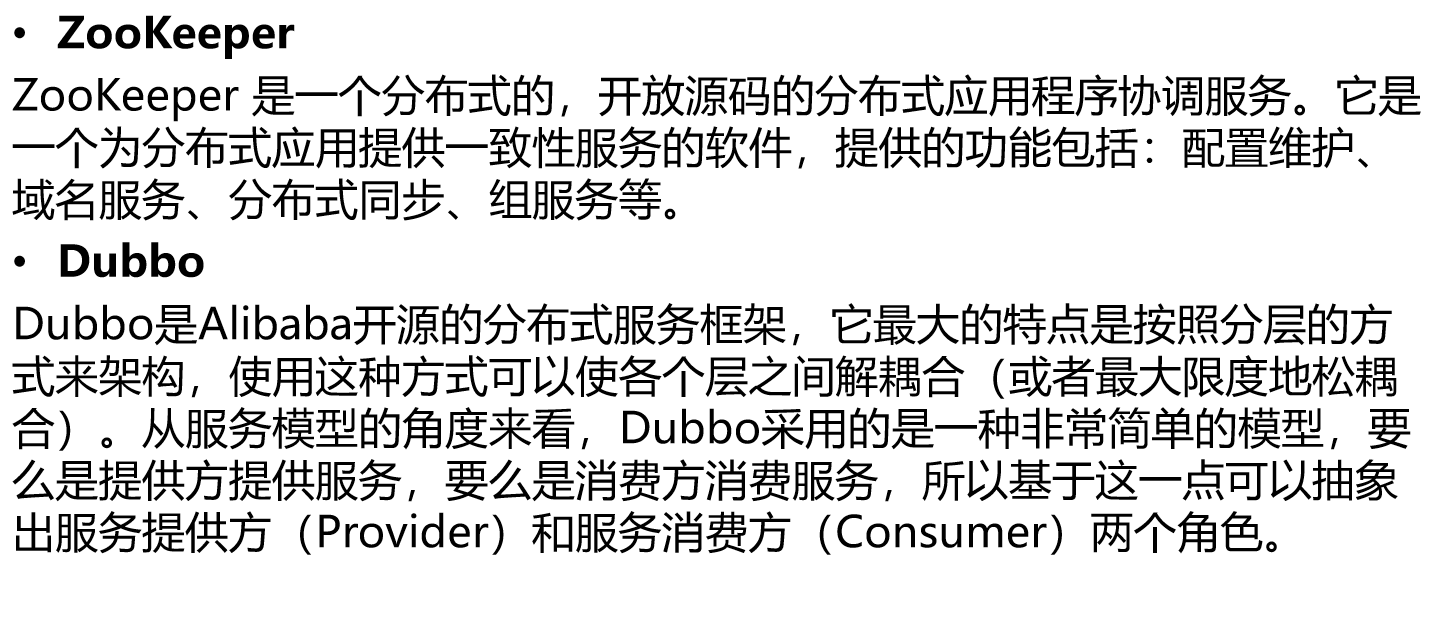
3.zookeeper
(1).zookeeper安装
官方文档:https://hub.docker.com/_/zookeeper?tab=description
在docker上安装zookeeper
[root@hosystem ~]# docker search zookeeper NAME DESCRIPTION STARS OFFICIAL AUTOMATED zookeeper Apache ZooKeeper is an open-source server wh… 947 [OK] [root@hosystem ~]# docker pull zookeeper:3.4.11 |
(2).zookeeper启动
[root@hosystem ~]# docker images REPOSITORY TAG IMAGE ID CREATED SIZE zookeeper 3.4.11 56d414270ae3 2 years ago 146MB [root@hosystem ~]# docker run --name ZK01 -p 2181:2181 --restart always -d 56d414270ae3 054d27805228d7d3cc6f8df96962a2dd9c24f3f5907554160854c661ef8d67dd [root@hosystem ~]# docker ps CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES 054d27805228 56d414270ae3 "/docker-entrypoint.…" 41 seconds ago Up 21 seconds 2888/tcp, 0.0.0.0:2181->2181/tcp, 3888/tcp ZK01
#将2181端口添加到防火墙 [root@hosystem ~]# firewall-cmd --permanent --zone=public --add-port=2181/tcp success [root@hosystem ~]# firewall-cmd --reload success |
(3).zookeeper整合dubbo
[1].创建项目
①.创建Empty Project
②.New Module
创建provider
创建consumer
4.Spring Boot和Spring Cloud
Martin Fowler 微服务原文 https://martinfowler.com/articles/microservices.html
(1).创建工程
[1].创建Empty Project
[2].创建eureka-server
[3].创建provider-ticket
[4].创建consumer-user
(2).配置eureka-server信息
[1].application.properties
server.port=8761 eureka.instance.hostname=eureka-server eureka.client.register-with-eureka=false eureka.client.fetch-registry=false eureka.client.service-url.defaultZone=http://localhost:8761/eureka/ |
[2].EurekaServerApplication.java
package com.hosystem.eurekaserver;
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer;
/** * 注册中心 * 1.配置Eureka信息 * 2.@EnableEurekaServer */ @EnableEurekaServer @SpringBootApplication public class EurekaServerApplication {
public static void main(String[] args) { SpringApplication.run(EurekaServerApplication.class, args); }
} |
访问eureka页面
(3).配置provider-ticket信息
[1].8001
①.TicketController.java
package com.hosystem.providerticket.controller;
import com.hosystem.providerticket.service.TicketService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController;
@RestController public class TicketController {
@Autowired TicketService ticketService;
@GetMapping("/ticket") public String getTicket(){ System.out.println("8001"); return ticketService.getTicket(); } } |
②.TicketService.java
package com.hosystem.providerticket.service;
import org.springframework.stereotype.Service;
@Service public class TicketService { public String getTicket(){ return "《模仿游戏》"; } } |
③.application.properties
server.port=8001 spring.application.name=provider-ticket #注册服务的时候使用服务的ip地址 eureka.instance.prefer-ip-address=true eureka.client.service-url.defaultZone=http://localhost:8761/eureka/ |
[2].8002
①.TicketController.java
package com.hosystem.providerticket.controller;
import com.hosystem.providerticket.service.TicketService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController;
@RestController public class TicketController {
@Autowired TicketService ticketService;
@GetMapping("/ticket") public String getTicket(){ System.out.println("8002"); return ticketService.getTicket(); } } |
②.TicketService.java
package com.hosystem.providerticket.service;
import org.springframework.stereotype.Service;
@Service public class TicketService { public String getTicket(){ return "《模仿游戏》"; } } |
③.application.properties
server.port=8002 spring.application.name=provider-ticket #注册服务的时候使用服务的ip地址 eureka.instance.prefer-ip-address=true eureka.client.service-url.defaultZone=http://localhost:8761/eureka/ |
(4).provider-ticket
[1].package
打包provider-ticket两次,一个端口为8001一次端口为8002
[2].启动provider
(5).consumer-user
[1]application.properties
server.port=8200 spring.application.name=consumer-user #注册服务的时候使用服务的ip地址 eureka.instance.prefer-ip-address=true eureka.client.service-url.defaultZone=http://localhost:8761/eureka/ |
[2].ConsumerUserApplication.java
package com.hosystem.consumeruser;
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.cloud.client.discovery.EnableDiscoveryClient; import org.springframework.cloud.client.loadbalancer.LoadBalanced; import org.springframework.context.annotation.Bean; import org.springframework.web.client.RestTemplate;
@EnableDiscoveryClient //开启发现功能 @SpringBootApplication public class ConsumerUserApplication {
public static void main(String[] args) { SpringApplication.run(ConsumerUserApplication.class, args); }
@LoadBalanced //使用负载均衡机制 @Bean public RestTemplate restTemplate(){ return new RestTemplate(); }
} |
[3].UserController.java
package com.hosystem.consumeruser.controller;
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; import org.springframework.web.client.RestTemplate;
@RestController public class UserController {
@Autowired RestTemplate restTemplate;
@GetMapping("/buy") public String buyTicket(String name){ String s = restTemplate.getForObject("http://PROVIDER-TICKET/ticket",String.class); return name+"观看了"+s; } } |
注意,观察加@LoadBalanced和不加@LoadBalanced的区别。加了@LoadBalanced的可以实现负载均衡。
参考文档:
https://github.com/alibaba/dubbo
https://hub.docker.com/_/zookeeper?tab=description
https://github.com/apache/dubbo/
https://mvnrepository.com/artifact/com.github.sgroschupf/zkclient