1 #include <stdio.h>
2 #define SEX 1
3 #define TALL 2
4 #define Fat 4
5 #define PRETTY 8
6 #define GLASS 16
7 //define不能加逗号
8 //自己的头文件用""
9 //系统头文件用<>
10 typedef union NODE
11 {
12 char a;
13 short b;
14 int c;
15 }node;
16 /*union
17 其中包含的所有变量从头开始存储
18 */
19 int main()
20 {
21 node aa;
22 printf("%d
",&aa.a);
23 printf("%d
",&aa.b);
24 printf("%d
",&aa.c);
25 printf("%d
",sizeof(node));
26 aa.a = 1;
27 aa.b = 2;
28 aa.c = 3;
29 printf("%d
",aa.a + aa.c);
30 //取反
31 //printf("%d
",~0);
32 //11111111 -1
33 //11111110 -2
34 //左移填0
35 //右移 无符号补0,有符号补符号位
36 //男女。高矮。胖瘦。好不好看。带不带眼镜
37 //char c = SEX|TALL|PRETTY;
38 //if(c&PRETTY)
39 //{
40 // printf("好看
");
41 //}
42 //else
43 //{
44 // printf("不好看
");
45 //}
46 }
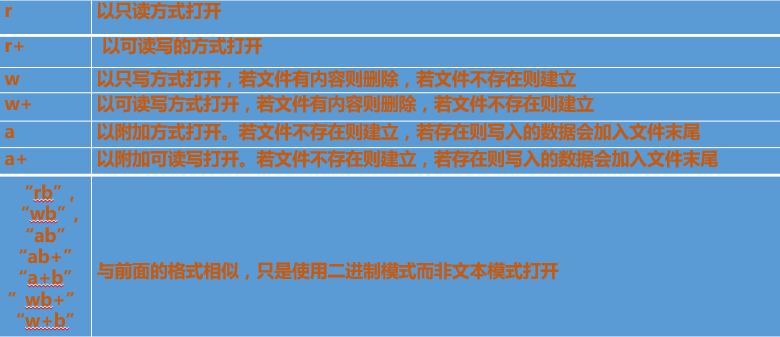
1 #include <stdio.h>
2 int main()
3 {
4 FILE* fp ;
5 FILE* fp2;
6 char buf[20]="qwerty" ;
7 char a ;
8 //fp = fopen("F:\a.txt","r");
9 //fopen_s(&fp,"F:\a.txt","w");//以只写方式打开,若文件有内容则删除,若文件不存在则建立
10 fopen_s(&fp,"F:\a.txt","r+");//r+覆盖原文件中前一部分
11 //fopen_s(&fp2,"F:\a.txt","w+");//w+清除原文件然后写入
12 //fopen_s(&fp,"F:\a.txt","a+");//a+在原文件末尾添加,重定位也从末尾添加
13 //fread(buf,5,1,fp);
14 //while( (a = getc(fp))!= EOF)//文件复制
15 //{
16 // fputc(a,fp2);
17 //}
18 fseek(fp,5,SEEK_END);//第二个参数offset偏移量,第三个参数base起始位置(从哪向后数起)
19 fwrite(buf,sizeof(char),4,fp);//将4个char型数据写入文件
20 fclose(fp);
21 //fclose(fp2);
22 //printf("%s
",buf);
23 return 0;
24
25 }
1 #include"stdio.h"
2 //枚举
3 typedef enum CS{石头,剪刀,布}cs;//不能使用中文逗号,格式无错,但为一个变量未隔开
4 //默认从0开始
5 //改变以后,下一个元素是前一个元素的值+1
6 //增加代码可读性
7 /*栈区:函数中声明的变量
8 作用域:整个函数
9 生命周期:函数结束
10
11 堆区:程序员申请程序员释放
12 生命周期:整个不释放就存在。例如:malloc
13
14 字符常量区:声明数组
15 char* a = "abcd";//字符常量区,不可以更改
16 char a[4] = "abcd";//从字符常量区复制到该区域,可以改变
17
18 const int p; 等价于 int const p;
19 const int *p; 等价于 int const *p;
20 const int *p //*p不可以改变,指向的可变但值不可以改变
21 const * int p //p不可以改变,指向的不变但值可以改变
22 */
23
24 int main()
25 {
26 cs a = 石头;
27 switch(a)
28 {
29 case 石头:
30 printf("石头
");
31 break;
32 case 剪刀:
33 printf("剪刀
");
34 break;
35 case 布:
36 printf("布
");
37 break;
38 }
39 }