<template>
<div class="vux-toast">
<div class="weui-mask_transparent" v-show="isShowMask && show"></div>
<transition :name="currentTransition">
<div class="weui-toast" :style="{ width}" :class="toastClass" v-show="show">
<i class="weui-icon-success-no-circle weui-icon_toast" v-show="type !== 'text'"></i>
<p class="weui-toast__content" v-if="text" :style="style" v-html="text" ></p>
<p class="weui-toast__content" v-else :style="style">
<slot></slot>
</p>
</div>
</transition>
</div>
</template>
<script>
export default {
name: 'toast',
props: {
value: Boolean,
time: {
type: Number,
default: 2000
},
type: {
type: String,
default: 'success'
},
transition: String,
{
type: String,
default: '7.6em'
},
isShowMask: {
type: Boolean,
default: false
},
text: String,
position: String
},
data () {
return {
show: false
}
},
created () {
console.log(this.text,"text")
if (this.value) {
this.show = true
}
},
computed: {
currentTransition () {
if (this.transition) {
return this.transition
}
if (this.position === 'top') {
return 'vux-slide-from-top'
}
if (this.position === 'bottom') {
return 'vux-slide-from-bottom'
}
return 'vux-fade'
},
toastClass () {
return {
'weui-toast_forbidden': this.type === 'warn',
'weui-toast_cancel': this.type === 'cancel',
'weui-toast_success': this.type === 'success',
'weui-toast_text': this.type === 'text',
'vux-toast-top': this.position === 'top',
'vux-toast-bottom': this.position === 'bottom',
'vux-toast-middle': this.position === 'middle'
}
},
style () {
if (this.type === 'text' && this.width === 'auto') {
return { padding: '10px' }
}
}
},
watch: {
show (val) {
if (val) {
this.$emit('input', true)
this.$emit('on-show')
clearTimeout(this.timeout)
this.timeout = setTimeout(() => {
this.show = false
this.$emit('input', false)
this.$emit('on-hide')
}, this.time)
}
},
value (val) {
this.show = val
}
}
}
</script>
import ToastComponent from "@/components/Toast"
import { mergeOptions } from './plugin_helper'
import objectAssign from 'object-assign'
let $vm
let watcher
const plugin = {
install (vue, pluginOptions = {}) {
const Toast = vue.extend(ToastComponent)
if (!$vm) {
$vm = new Toast({
el: document.createElement('div')
})
document.body.appendChild($vm.$el)
}
console.log($vm.$options.props,"$vm.$options.props")
const defaults = {}
for (let i in $vm.$options.props) {
if (i !== 'value') {
defaults[i] = $vm.$options.props[i].default
}
}
console.log(defaults,"defaults")
const toast = {
show (options = {}) {
// destroy watcher
watcher && watcher()
console.log(options,"options")
console.log(typeof options,"options")
if (typeof options === 'string') {
mergeOptions($vm, objectAssign({}, pluginOptions, {text: options}))
} else if (typeof options === 'object') {
console.log(123)
mergeOptions($vm, objectAssign({}, pluginOptions, options))
}
if (typeof options === 'object' && options.onShow || options.onHide) {
console.log(456)
watcher = $vm.$watch('show', (val) => {
val && options.onShow && options.onShow($vm)
val === false && options.onHide && options.onHide($vm)
})
}
$vm.show = true
},
text (text, position = 'default') {
this.show({
type: 'text',
'auto',
position,
text
})
},
hide () {
$vm.show = false
},
isVisible () {
return $vm.show
}
}
vue.prototype.$alert = toast
}
}
export default plugin
export const install = plugin.install
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <script src="./vue.js"></script> <title>Document</title> </head> <style> </style> <body> <div id="app"> <base-component></base-component> <base-component1></base-component1> <base-component2></base-component2> <hr> </div> <div id="app1"> </div> <script> let baseOptions = { template: `<p> {{ firstName }} {{ lastName }} aka {{ alias }}</p>`, data () { return { firstName: '大漠', lastName: 'w3cplus', alias: '大漠_w3cplus' } } } let baseExtend = Vue.extend(baseOptions) let newbaseExtend =new baseExtend({ el:"#app1" });
// 等同于下面, 区别是 动态添加到body里,而不是固定的dom节点
let newbaseExtend =new baseExtend({
el:document.createElement("div")
});
document.body.appendChild(newbaseExtend.$el)
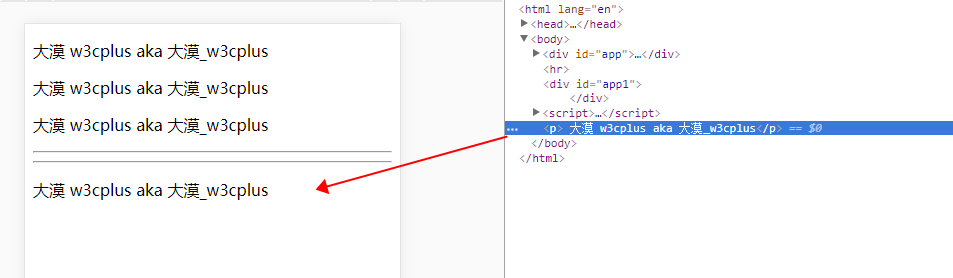
Vue.component('base-component',baseOptions) Vue.component('base-component1',baseExtend) Vue.component('base-component2',Vue.extend(baseOptions)) /** *Vue.component()会注册一个全局的组件, 其会自动判断第二个传进来的是Vue继续对象(Vue.extend)还是普通对象({...}), 如果传进来的是普能对象的话会自动调用Vue.extend, 所以你先继承再传,还是直接传普通对象对Vue.component()的最终结果是没差的。著作权归作者所有。 */ var vm = new Vue({ el:"#app" }) // console.log(baseExtend,"baseExtend") // console.log(Vue,"vue") console.log(vm,"vm") /** *ƒ VueComponent(options) { this._init(options); } "baseExtend" ƒ Vue(options) { if (!(this instanceof Vue) ) { warn('Vue is a constructor and should be called with the `new` keyword'); } this._init(options); } "vue" */ /* * Vue.extend()和Vue.component()的区别 https://www.w3cplus.com/vue/vue-extend.html */ </script> </body> </html>
* Vue.extend()和Vue.component()的区别
https://www.w3cplus.com/vue/vue-extend.html
*/