D. Legacy
Rick and his co-workers have made a new radioactive formula and a lot of bad guys are after them. So Rick wants to give his legacy to Morty before bad guys catch them.
There are n planets in their universe numbered from 1 to n. Rick is in planet number s (the earth) and he doesn't know where Morty is. As we all know, Rick owns a portal gun. With this gun he can open one-way portal from a planet he is in to any other planet (including that planet). But there are limits on this gun because he's still using its free trial.
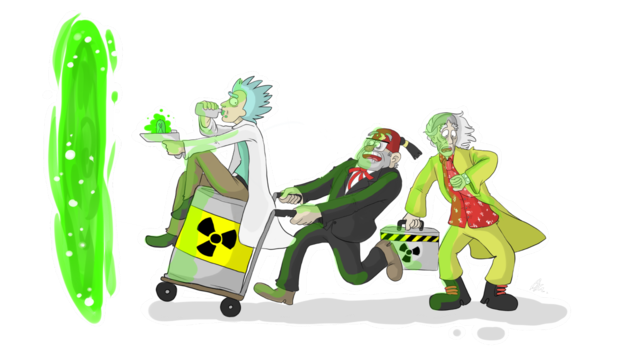
By default he can not open any portal by this gun. There are q plans in the website that sells these guns. Every time you purchase a plan you can only use it once but you can purchase it again if you want to use it more.
Plans on the website have three types:
- With a plan of this type you can open a portal from planet v to planet u.
- With a plan of this type you can open a portal from planet v to any planet with index in range [l, r].
- With a plan of this type you can open a portal from any planet with index in range [l, r] to planet v.
Rick doesn't known where Morty is, but Unity is going to inform him and he wants to be prepared for when he finds and start his journey immediately. So for each planet (including earth itself) he wants to know the minimum amount of money he needs to get from earth to that planet.
Input
The first line of input contains three integers n, q and s (1 ≤ n, q ≤ 105, 1 ≤ s ≤ n) — number of planets, number of plans and index of earth respectively.
The next q lines contain the plans. Each line starts with a number t, type of that plan (1 ≤ t ≤ 3). If t = 1 then it is followed by three integers v, u and w where w is the cost of that plan (1 ≤ v, u ≤ n, 1 ≤ w ≤ 109). Otherwise it is followed by four integers v, l, r and w where w is the cost of that plan (1 ≤ v ≤ n, 1 ≤ l ≤ r ≤ n, 1 ≤ w ≤ 109).
Output
In the first and only line of output print n integers separated by spaces. i-th of them should be minimum money to get from earth to i-th planet, or - 1 if it's impossible to get to that planet.
Examples
input
3 5 1
2 3 2 3 17
2 3 2 2 16
2 2 2 3 3
3 3 1 1 12
1 3 3 17
output
0 28 12
input
4 3 1
3 4 1 3 12
2 2 3 4 10
1 2 4 16
output
0 -1 -1 12
Note
In the first sample testcase, Rick can purchase 4th plan once and then 2nd plan in order to get to get to planet number 2.
题意:有n个点,q个询问,每次询问有一种操作。操作1:u→[l,r](即u到l,l+1,l+2,...,r距离均为w)的距离为w;操作2:[l,r]→u的距离为w;操作3:u到v的距离为w;求起点到其他点的最短距离,到达不了输出-1。
思路:这道题目如果老老实实的去建图的话,边会多到爆炸。所以我们需要另外想一个建图方法。
由于边的起点或者终点涉及到了区间的情况,我们可以使用线段树建图的套路。
对于整段区间,我们用把它划分成一颗线段树。
对于整个图,我们可以构造两颗线段树A和B。
在A线段树上,我们从底部往上连权值为0的边。对于B线段树来说我们从顶部往下连边权值为0的边。
然后我们把B线段树底部的所有点向A线段树底部的点连权值为0得边。
对于type=1的边,我们从A线段树底部的点向B线段树底部的点做权值为w的边。
对于type=2的边,我们从A线段树底部的点向B线段树对应的区间段连权值为w的一些边。
对于type=3的边,我们从A线段树对应的区间段向B线段树底部对应的点连权值为w的一些边。
然后跑最短路就行了。
1 //2017-09-04 2 #include <cstdio> 3 #include <cstring> 4 #include <iostream> 5 #include <algorithm> 6 #include <vector> 7 #include <queue> 8 #define ll long long 9 #define lson (id<<1) 10 #define rson ((id<<1)|1) 11 12 using namespace std; 13 14 const int N = 110000; 15 const ll INF = 0x3f3f3f3f3f3f3f3f; 16 17 int head[N<<4], tot; 18 struct Edge{ 19 int to, w, next; 20 }edge[N<<7]; 21 22 void init(){ 23 tot = 0; 24 memset(head, -1, sizeof(head)); 25 } 26 27 void add_edge(int u, int v, int w){ 28 edge[tot].w = w; 29 edge[tot].to = v; 30 edge[tot].next = head[u]; 31 head[u] = tot++; 32 } 33 34 vector<int> vec; 35 int tree[2][N<<4], idx; 36 //k为0表示从顶向下的线段树 37 //k为1表示从底向上的线段树 38 void build(int id, int l, int r, int k){ 39 tree[k][id] = ++idx; 40 if(l == r){ 41 if(k == 0) 42 add_edge(tree[k][id], l, 0); 43 else 44 add_edge(l, tree[k][id], 0); 45 return; 46 } 47 int mid = (l+r)>>1; 48 build(lson, l, mid, k); 49 build(rson, mid+1, r, k); 50 if(k == 0){ 51 add_edge(tree[k][id], tree[k][lson], 0); 52 add_edge(tree[k][id], tree[k][rson], 0); 53 }else{ 54 add_edge(tree[k][lson], tree[k][id], 0); 55 add_edge(tree[k][rson], tree[k][id], 0); 56 } 57 } 58 59 //获得[L, R]区间对应的节点集合 60 //l,r为辅助变量,k表示操作第k棵线段树 61 void get_interval(int id, int l, int r, int L, int R, int k){ 62 if(L <= l && r <= R){ 63 vec.push_back(tree[k][id]); 64 return; 65 } 66 int mid = (l+r)>>1; 67 if(mid >= L) 68 get_interval(lson, l, mid, L, R, k); 69 if(mid < R) 70 get_interval(rson, mid+1, r, L, R, k); 71 } 72 73 bool vis[N<<4]; 74 ll dis[N<<4]; 75 int cnt[N<<4]; 76 deque<int> dq; 77 78 bool spfa(int s, int n){ 79 memset(vis, 0, sizeof(vis)); 80 memset(cnt, 0, sizeof(cnt)); 81 for(int i = 1; i <= n; i++) 82 dis[i] = INF; 83 vis[s] = 1; 84 dis[s] = 0; 85 cnt[s] = 1; 86 deque<int> dq; 87 dq.push_back(s); 88 while(!dq.empty()){ 89 int u = dq.front(); 90 dq.pop_front(); 91 vis[u] = 0; 92 for(int i = head[u]; i != -1; i = edge[i].next){ 93 int v = edge[i].to; 94 if(dis[v] > dis[u] + edge[i].w){ 95 dis[v] = dis[u] + edge[i].w; 96 if(!vis[v]){ 97 vis[v] = 1; 98 dq.push_back(v); 99 if(++cnt[v] > n)return false; 100 } 101 } 102 } 103 } 104 return true; 105 } 106 int main() 107 { 108 //freopen("inputD.txt", "r", stdin); 109 int n, q, s; 110 while(scanf("%d%d%d", &n, &q, &s) != EOF){ 111 init(); 112 idx = n;//线段树上表示区间的点从n+1开始 113 build(1, 1, n, 0); 114 build(1, 1, n, 1); 115 int l, r, v, w, u, t; 116 while(q--){ 117 scanf("%d", &t); 118 if(t == 1){ 119 scanf("%d%d%d", &v, &u, &w); 120 add_edge(v, u, w); 121 }else if(t == 2){ 122 scanf("%d%d%d%d", &v, &l, &r, &w); 123 vec.clear(); 124 get_interval(1, 1, n, l, r, 0); 125 for(auto &u : vec) 126 add_edge(v, u, w); 127 }else if(t == 3){ 128 scanf("%d%d%d%d", &v, &l, &r, &w); 129 vec.clear(); 130 get_interval(1, 1, n, l, r, 1); 131 for(auto &u : vec) 132 add_edge(u, v, w); 133 } 134 } 135 spfa(s, 5*n); 136 for(int i = 1; i <= n; i++) 137 if(dis[i] == INF) 138 printf("-1 "); 139 else printf("%I64d ", dis[i]); 140 printf(" "); 141 } 142 143 return 0; 144 }