1. Write a program in Java to print the Floyd's Triangle.
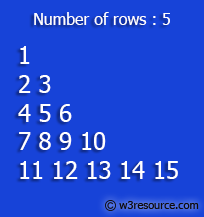
package com.w3resource;
import java.util.Scanner;
public class FloydTriangle {
public static void main(String[] args) {
int i, j, n, p, q;
System.out.print("Input number of rows: ");
Scanner in = new Scanner(System.in);
n = in.nextInt();
for (i = 1; i <= n; i++) {
if (i % 2 == 0) {
p = 1;
q = 0;
} else {
p = 0;
q = 1;
}
for(j = 1; j <= i; j++) {
if (j % 2 == 0)
System.out.print(p);
else
System.out.print(q);
}
System.out.println();
}
}
}
Output
Input number of rows: 5
1
01
101
0101
10101
2. Write a program in Java to display the n terms of odd natural number and their sum.
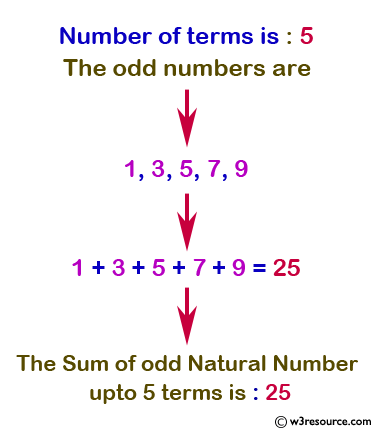
package com.w3resource;
import java.util.Scanner;
public class PrintOddAndSum {
public static void main(String[] args) {
int i, n, sum = 0;
System.out.print("Input number of terms is : ");
Scanner in = new Scanner(System.in);
n = in.nextInt();
System.out.println("
The odd numbers are : ");
for (i = 1; i <= n; i++) {
System.out.println(2 * i - 1);
sum += 2 * i - 1;
}
System.out.println("The Sum of odd Natural Number upto " + n + " terms is : " + sum);
}
}
Output
Input number of terms is: 5
The odd numbers are:
1
3
5
7
9
The Sum of odd Natural Number upto 5terms is : 25
3. Write a program in Java to make such a pattern like right angle triangle with a number which will repeat a number in a row. The pattern is as follows
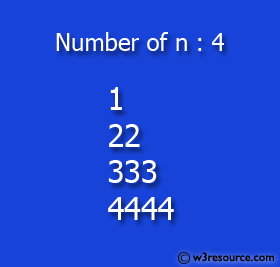
package com.w3resource;
import java.util.Scanner;
public class PrintRepeatNumber {
public static void main(String[] args) {
int i, j, n;
System.out.print("Input number of n : ");
Scanner in = new Scanner(System.in);
n = in.nextInt();
for (i = 1; i <= n; i++) {
for (j = 1; j <= i; j++) {
System.out.print(i);
}
System.out.println();
}
}
}
Output
Input number of n : 4
1
22
333
4444
4. Write a program in Java to make such a pattern like right angle triangle with number increased by 1.
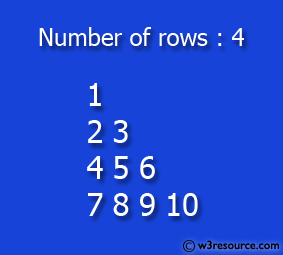
package com.w3resource;
import java.util.Scanner;
public class IncrementNumber {
public static void main(String[] args) {
int i, j, n, k = 1;
System.out.print("Input number of rows: ");
Scanner in = new Scaner(System.in);
n = in.nextInt();
for (i = 1; i <= n; i++) {
for (j = 1; j <= i; j++) {
System.out.print(k++);
}
System.out.println();
}
}
}
Output
Input number of rows : 4
1
23
456
78910
5. Write a program in Java to make such a pattern like a pyramid with a number which will repeat the number in the same row.
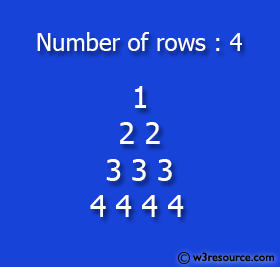
package com.w3resource;
import java.util.Scanner;
public class PrintRepeatElement {
public static void main(String[] args) {
int i, j, n, s, x;
System.out.print("Input number of rows : ");
Scanner in = new Scanner(System.in);
n = in.nextInt();
s = n + 4 - 1;
for (i = 1; i <= n; i++) {
for (x = s; x != 0; x--) {
System.out.print(" ");
}
for (j = 1; j <= i; j++) {
System.out.print(i + " ");
}
System.out.println();
s--;
}
}
}
Output
Input number of rows : 4
1
2 2
3 3 3
4 4 4 4
6. Write a Java program that accepts two floatingpoint numbers and checks whether they are the same up to two decimal places.
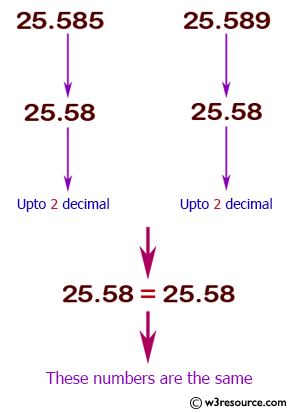
package com.w3resource;
import java.util.Scanner;
public class Exercise {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Input first floatingpoint number: ");
double num1 = input.nextDouble();
System.out.print("Input second floatingpoint number: ");
double num2 = input.nextDouble();
input.close();
if (Math.abs(num1 - num2) <= 0.01) {
System.out.println("These numbers are the same.");
} else {
System.out.println("THese numbers are different.");
}
}
}
Output
Input first floatingpoint number : 20.569
Input second floatingpoint number : 20.567
These numbers are the same.
7. Write a Java program to display the following character rhombus structure.
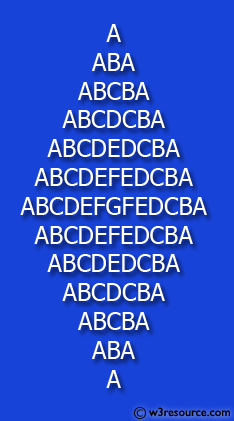
package com.w3resource;
import java.util.Scanner;
public class RhombusStructure {
public static void main(String args[]) {
Scanner sc = new Scanner(System.in);
System.out.println("Input the number: ");
int n = sc.nextInt();
int count = 1;
int count2 = 1;
char ch = 'A';
for (int i = 1; i < (n * 2); i++) {
for (int space = n - count2; space > 0; space--)
{
System.out.print(" ");
}
if (i < n) {
count2++;
} else {
count2--;
}
for (int j = 0; j < count; j++) {
System.out.print(ch);//print Character
if (j < count / 2) {
ch++;
} else {
ch--;
}
}
if (i < n) {
count = count + 2;
} else {
count = count - 2;
}
ch = 'A';
System.out.println();
}
}
}
Output
Input the number:
7
A
ABA
ABCBA
ABCDCBA
ABCDEDCBA
ABCDEFEDCBA
ABCDEFGFEDCBA
ABCDEFEDCBA
ABCDEDCBA
ABCDCBA
ABCBA
ABA
A
8. Write a Java program to display the number rhombus structure.
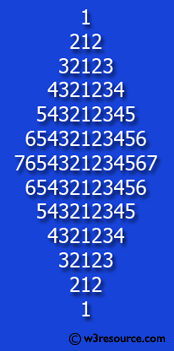
package com.w3resource;
import java.util.Scanner;
public class RhombusNumber {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
System.out.print("Input the number : ");
int n = in.nextInt();
int no_of_spaces = 1;
int start = 0;
int count = 1;
for (int i = 1; i < (n * 2); i++) {
for (int space = n - no_of_spaces; space > 0; space--) {
System.out.print(" ");
}
if (i < n) {
start = i; // for number
no_of_spaces++; // for spaces
} else {
start = n * 2 - i; // for number
no_of_spaces--; // for spaces
}
for (int j = 0; j < count; j++) {
System.out.print(start);
if (j < count / 2) {
start--;
} else {
start++;
}
}
if (i < n) {
count = count + 2;
} else {
count = count - 2;
}
System.out.println();
}
}
}
Output
Input the number : 7
1
212
32123
4321234
543212345
65432123456
7654321234567
65432123456
543212345
4321234
32123
212
1
9. Write a program in Java to display the pattern like right angle triangle with a number.
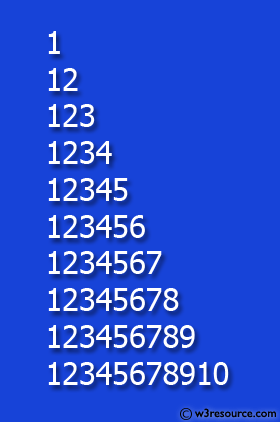
package com.programiz;
import java.util.Scanner;
public class PrintPattern {
public static void main(String[] args) {
int i, j, n;
System.out.print("Input number of rows : ");
Scanner in = new Scanner(System.in);
n = in.nextInt();
for (i = 1; i <= n; i++) {
for (j = 1; j <= i; j++) {
System.out.print(j);
}
System.out.println();
}
}
}
10. Write a Java program to find the number of days in a month.
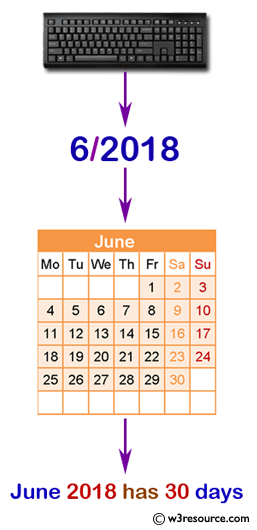
package com.w3resource;
import java.util.Scanner;
public class NumberOfDays {
public static void main(String[] strings) {
Scanner input = new Scanner(System.in);
int number_Of_DaysInMonth = 0;
String MonthOfName = "Unknown";
System.out.print("Input a month number: ");
int month = input.nextInt();
System.out.print("Input a year: ");
int year = input.nextInt();
switch (month) {
case 1:
MonthOfName = "January";
number_Of_DaysInMonth = 31;
break;
case 2:
MonthOfName = "February";
if ((year % 400 == 0) || ((year % 4 == 0) && (year % 100 != 0))) {
number_Of_DaysInMonth = 29;
} else {
number_Of_DaysInMonth = 28;
}
break;
case 3:
MonthOfName = "March";
number_Of_DaysInMonth = 31;
break;
case 4:
MonthOfName = "April";
number_Of_DaysInMonth = 30;
break;
case 5:
MonthOfName = "May";
number_Of_DaysInMonth = 31;
break;
case 6:
MonthOfName = "June";
number_Of_DaysInMonth = 30;
break;
case 7:
MonthOfName = "July";
number_Of_DaysInMonth = 31;
break;
case 8:
MonthOfName = "August";
number_Of_DaysInMonth = 31;
break;
case 9:
MonthOfName = "September";
number_Of_DaysInMonth = 30;
break;
case 10:
MonthOfName = "October";
number_Of_DaysInMonth = 31;
break;
case 11:
MonthOfName = "November";
number_Of_DaysInMonth = 30;
break;
case 12:
MonthOfName = "December";
number_Of_DaysInMonth = 31;
}
System.out.print(MonthOfName + " " + year + " has " + number_Of_DaysInMonth + " days
");
}
}
Output
Input a month number: 2
Input a year: 2020
February 2020 has 29 days