Description
Dao was a simple two-player board game designed by Jeff Pickering and Ben van Buskirk at 1999. A variation of it, called S-Dao, is a one-player game. In S-Dao, the game board is a 4 * 4 square with 16 cells. There are 4 black stones and 4 white stones placed on the game board randomly in the beginning. The player is given a final position and asked to play the game using the following rules such that the final position is reached using the minimum number of moves: 1. You first move a white stone, and then a black stone. You then alternatively move a white stone and a black stone. 2. A stone can be moved horizontally, vertically or diagonally. A stone must be moved in a direction until the boarder or another stone is encountered. There is no capture or jump. 3. During each move, you need to move a stone of the right color. You cannot pass. An example of a sequence of legal moves is shown in the following figure. This move sequence takes 4 moves. This is not a sequence of legal moves

using the least number of moves assume the leftmost board is the initial position and the rightmost board is the final position. A sequence of moves using only 3 moves is shown below.
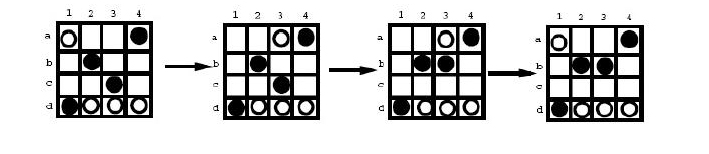
Given an initial position and a final position, your task is to report the minimum number of moves from the initial position to the final position.
Input
The first line contains the number of test cases w, w <= 6. Then the w test cases are listed one by one. Each test case consists of 8 lines, 4 characters per line. The first 4 lines are the initial board position. The remaining 4 lines are the final board position. The i-th line of a board is the board at the i-th row. A character 'b' means a black stone, a character 'w' means a white stone, and a '*' means an empty cell.
Output
For each test case, output the minimum number of moves in one line. If it is impossible to move from the initial position to the final position, then output -1.
Sample Input
2 w**b *wb* *bw* b**w w**b *wb* *bw* bw** w**b *b** **b* bwww w**b *bb* **** bwww
Sample Output
1 3
Hint
Doing simple exhaustive search without planning ahead will most likely get you into troubles.
Source
写了快200行的题目,跟前面一道题目 poj2046 很相似,用hash来保存整个图的状态,然后剩下的就是bfs的控制能力了。

1 #include<iostream> 2 #include<cstdio> 3 #include<cstring> 4 #include<queue> 5 #include<algorithm> 6 #include<stdlib.h> 7 using namespace std; 8 #define ll long long 9 #define M 1000007 10 ll hash[M]; 11 ll goal[5][5]; 12 ll base[17]={1}; 13 ll aimNum; 14 ll flag; 15 ll white; 16 ll black; 17 struct Node{ 18 ll x,y; 19 ll mp[5][5]; 20 ll step; 21 ll whitePx[5]; 22 ll whitePy[5]; 23 ll blackPx[5]; 24 ll blackPy[5]; 25 26 }st; 27 bool inserNum(ll ans){//hash的插入,看看是否跟之前的状态相同,其实跟vis数组标记一个意思 28 ll val=ans%M; 29 while(hash[val]!=-1 && hash[val]!=ans){ 30 val=(val+10)%M; 31 } 32 if(hash[val]==-1){ 33 hash[val]=ans; 34 return true;//可以插入返回true 35 } 36 return false;//否则返回false 37 } 38 39 bool work(Node cnt){ 40 ll ans=0; 41 for(ll i=0;i<4;i++){ 42 for(ll j=0;j<4;j++){ 43 ans=ans+cnt.mp[i][j]*base[i*4+j];//ans为整张图的hash值 44 } 45 } 46 if(ans==aimNum){ 47 flag=1; 48 } 49 if(inserNum(ans)) 50 return true; 51 return false; 52 } 53 ll dirx[]={0,0,-1,1,-1,-1,1,1}; 54 ll diry[]={-1,1,0,0,-1,1,-1,1}; 55 ll bfs(){ 56 queue<Node>q; 57 q.push(st); 58 Node t1,t2; 59 while(!q.empty()){ 60 t1=q.front(); 61 q.pop(); 62 if(t1.step%2==0){ 63 for(ll i=0;i<4;i++){ 64 65 ll sx=t1.whitePx[i]; 66 ll sy=t1.whitePy[i]; 67 //printf("%I64d %I64d ",sx,sy); 68 for(ll j=0;j<8;j++){ 69 t2=t1; 70 t2.x=sx+dirx[j]; 71 t2.y=sy+diry[j]; 72 if(t2.mp[t2.x][t2.y]==1 || t2.mp[t2.x][t2.y]==2) continue; 73 t2.step=t1.step+1; 74 while(t2.x>=0 && t2.y>=0 && t2.x<4 && t2.y<4 && t2.mp[t2.x][t2.y]==3){ 75 t2.x=t2.x+dirx[j]; 76 t2.y=t2.y+diry[j]; 77 } 78 t2.x=t2.x-dirx[j]; 79 t2.y=t2.y-diry[j]; 80 swap(t2.mp[t2.x][t2.y],t2.mp[sx][sy]); 81 if(work(t2)){ 82 t2.whitePx[i]=t2.x; 83 t2.whitePy[i]=t2.y; 84 q.push(t2); 85 if(flag){ 86 return t2.step; 87 } 88 } 89 } 90 } 91 } 92 else{ 93 for(ll i=0;i<4;i++){ 94 ll sx=t1.blackPx[i]; 95 ll sy=t1.blackPy[i]; 96 for(ll j=0;j<8;j++){ 97 t2=t1; 98 t2.x=sx+dirx[j]; 99 t2.y=sy+diry[j]; 100 if(t2.mp[t2.x][t2.y]==1 || t2.mp[t2.x][t2.y]==2) continue; 101 t2.step=t1.step+1; 102 while(t2.x>=0 && t2.y>=0 && t2.x<4 && t2.y<4 && t2.mp[t2.x][t2.y]==3){ 103 t2.x=t2.x+dirx[j]; 104 t2.y=t2.y+diry[j]; 105 } 106 t2.x=t2.x-dirx[j]; 107 t2.y=t2.y-diry[j]; 108 swap(t2.mp[t2.x][t2.y],t2.mp[sx][sy]); 109 if(work(t2)){ 110 t2.blackPx[i]=t2.x; 111 t2.blackPy[i]=t2.y; 112 q.push(t2); 113 if(flag){ 114 return t2.step; 115 } 116 } 117 } 118 } 119 } 120 } 121 return -1; 122 } 123 ll calGoal(){ 124 ll ans=0; 125 for(ll i=0;i<4;i++){ 126 for(ll j=0;j<4;j++){ 127 ans=ans+goal[i][j]*base[i*4+j]; 128 } 129 } 130 return ans; 131 } 132 133 134 135 int main() 136 { 137 for(ll i=1;i<17;i++){ 138 base[i]=base[i-1]*2; 139 } 140 ll t; 141 scanf("%I64d",&t); 142 while(t--){ 143 memset(hash,-1,sizeof(hash)); 144 white=0; 145 black=0; 146 char s[10]; 147 for(ll i=0;i<4;i++){ 148 scanf("%s",s); 149 for(ll j=0;j<4;j++){ 150 if(s[j]=='w'){ 151 st.x=i; 152 st.y=j; 153 st.mp[i][j]=1; 154 st.whitePx[white]=i; 155 st.whitePy[white++]=j; 156 } 157 else if(s[j]=='b'){ 158 st.x=i; 159 st.y=j; 160 st.mp[i][j]=2; 161 st.blackPx[black]=i; 162 st.blackPy[black++]=j; 163 } 164 else if(s[j]=='*'){ 165 st.mp[i][j]=3; 166 } 167 } 168 } 169 for(ll i=0;i<4;i++){ 170 scanf("%s",s); 171 for(ll j=0;j<4;j++){ 172 if(s[j]=='w'){ 173 goal[i][j]=1; 174 } 175 else if(s[j]=='b'){ 176 goal[i][j]=2; 177 } 178 else if(s[j]=='*'){ 179 goal[i][j]=3; 180 } 181 } 182 } 183 184 aimNum=calGoal(); 185 flag=0; 186 st.step=0; 187 work(st); 188 if(flag){ 189 printf("0 "); 190 } 191 else{ 192 printf("%I64d ",bfs()); 193 } 194 195 } 196 return 0; 197 }