代码:
MainActivity代码:
package com.example.contactsch;
import java.util.ArrayList; import java.util.HashMap; import java.util.Map;
import android.app.Activity; import android.app.LauncherActivity.ListItem; import android.content.Intent; import android.database.Cursor; import android.database.sqlite.SQLiteDatabase; import android.net.LocalSocketAddress.Namespace; import android.os.Bundle; import android.support.v4.widget.CursorAdapter; import android.support.v4.widget.SimpleCursorAdapter; import android.util.Log; import android.view.Menu; import android.view.MenuInflater; import android.view.MenuItem; import android.view.View; import android.view.View.OnClickListener; import android.widget.AdapterView; import android.widget.AdapterView.OnItemClickListener; import android.widget.Button; import android.widget.ListView; import android.widget.SimpleAdapter; import android.widget.TextView;
public class MainActivity extends Activity { private ListView lView; private MyOpenHelper dbHelper; private Button delb,addb; SQLiteDatabase db; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); delb=(Button)findViewById(R.id.del); addb=(Button)findViewById(R.id.add); lView=(ListView)findViewById(R.id.listV); Log.i("test", "111111111111111111111"); dbHelper=new MyOpenHelper(MainActivity.this, "personal_contacts.db", null, 1); db=dbHelper.getReadableDatabase(); Cursor cursor=db.rawQuery("select * from contacts", null); Log.i("test", "2222222222222222222222"); inflateList(cursor); Log.i("test", "3333333333333333333333"); addb.setOnClickListener(new OnClickListener() { @Override public void onClick(View arg0) { // TODO Auto-generated method stub Bundle bundle=new Bundle(); bundle.putString("name", ""); Intent intent=new Intent(); intent.setClass(MainActivity.this, detail_main.class); intent.putExtras(bundle); startActivity(intent); } }); lView.setOnItemClickListener(new OnItemClickListener() {
@Override public void onItemClick(AdapterView<?> arg0, View arg1, int arg2, long arg3) { // TODO Auto-generated method stub TextView ser=(TextView)arg1.findViewById(R.id.tView1); String se=ser.getText().toString(); Intent intent=new Intent(MainActivity.this,detail_main.class); Bundle bundle=new Bundle(); bundle.putString("name", se); intent.putExtras(bundle); startActivity(intent); } });
}
private void inflateList(Cursor cursor) { // TODO Auto-generated method stub int c=cursor.getCount(); String[] nameS=new String[c]; String[] phoneS=new String[c]; int co=0; while(cursor.moveToNext()){ nameS[co]=cursor.getString(cursor.getColumnIndex("name")); phoneS[co]=cursor.getString(cursor.getColumnIndex("phone")); co++; } final ArrayList<HashMap<String, Object>> listItem=new ArrayList<HashMap<String,Object>>(); for (int i = 1; i <= c; i++) { HashMap<String, Object> map=new HashMap<String, Object>(); map.put("ItemTitle", nameS[i-1]); map.put("ItemText", phoneS[i-1]); listItem.add(map); } Log.i("test", "66666666666666666666666666666"); if (cursor.getCount()!=0) { Log.i("test", "1000000000000000000000000000"); SimpleAdapter sAdapter=new SimpleAdapter(MainActivity.this, listItem, R.layout.link, new String[]{"ItemTitle","ItemText"} , new int[]{R.id.tView1,R.id.tView2}); Log.i("test", "10101010101010101010101010101"); lView.setAdapter(sAdapter); } Log.i("test", "4444444444444444"); Log.i("test", "55555555555555555555"); }
@Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. MenuInflater inflater=new MenuInflater(this); inflater.inflate(R.menu.main, menu); return super.onCreateOptionsMenu(menu); }
}
DetailActivity代码:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" >
<LinearLayout android:layout_width="fill_parent" android:layout_height="wrap_content" android:orientation="horizontal" android:layout_marginTop="10dp">
<TextView android:id="@+id/name" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="姓名:" android:textSize="20sp" />
<EditText android:id="@+id/etname" android:layout_width="match_parent" android:layout_height="wrap_content" android:ems="10" android:textSize="25sp" android:background="#eeffffff" > </EditText> </LinearLayout>
<LinearLayout android:layout_width="fill_parent" android:layout_height="wrap_content" android:orientation="horizontal" android:layout_marginTop="10dp"> <TextView android:id="@+id/phone" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="固定电话:" android:textSize="20sp" />
<EditText android:id="@+id/etphone" android:layout_width="match_parent" android:layout_height="wrap_content" android:ems="10" android:textSize="25sp" android:background="#eeffffff"> </EditText> </LinearLayout>
<LinearLayout android:layout_width="fill_parent" android:layout_height="wrap_content" android:orientation="horizontal" android:layout_marginTop="10dp"> <TextView android:id="@+id/mobile" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="移动电话:" android:textSize="20sp" />
<EditText android:id="@+id/etmobile" android:layout_width="match_parent" android:layout_height="wrap_content" android:ems="10" android:textSize="25sp" android:background="#eeffffff"> </EditText> </LinearLayout>
<LinearLayout android:layout_width="fill_parent" android:layout_height="wrap_content" android:orientation="horizontal" android:layout_marginTop="10dp">
<TextView android:id="@+id/email" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="电子邮件:" android:textSize="20sp" />
<EditText android:id="@+id/etemail" android:layout_width="match_parent" android:layout_height="wrap_content" android:ems="10" android:textSize="25sp" android:background="#eeffffff"> </EditText> </LinearLayout>
<LinearLayout android:layout_width="fill_parent" android:layout_height="wrap_content" android:orientation="horizontal" android:layout_marginTop="10dp">
<TextView android:id="@+id/post" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="邮政编码:" android:textSize="20sp" />
<EditText android:id="@+id/etpost" android:layout_width="match_parent" android:layout_height="wrap_content" android:ems="10" android:textSize="25sp" android:background="#eeffffff"> </EditText> </LinearLayout>
<LinearLayout android:layout_width="fill_parent" android:layout_height="wrap_content" android:orientation="horizontal" android:layout_marginTop="10dp">
<TextView android:id="@+id/addr" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="通信地址:" android:textSize="20sp" />
<EditText android:id="@+id/etaddr" android:layout_width="match_parent" android:layout_height="wrap_content" android:ems="10" android:textSize="25sp" android:background="#eeffffff"> </EditText> </LinearLayout>
<LinearLayout android:layout_width="fill_parent" android:layout_height="wrap_content" android:orientation="horizontal" android:layout_marginTop="10dp">
<TextView android:id="@+id/comp" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="公司名称:" android:textSize="20sp" />
<EditText android:id="@+id/etcomp" android:layout_width="match_parent" android:layout_height="wrap_content" android:ems="10" android:textSize="25sp" android:background="#eeffffff"> </EditText> </LinearLayout>
<ImageButton android:id="@+id/bcButton1" android:layout_width="fill_parent" android:layout_height="wrap_content" android:src="@drawable/www" />
</LinearLayout>
运行结果:(截图)
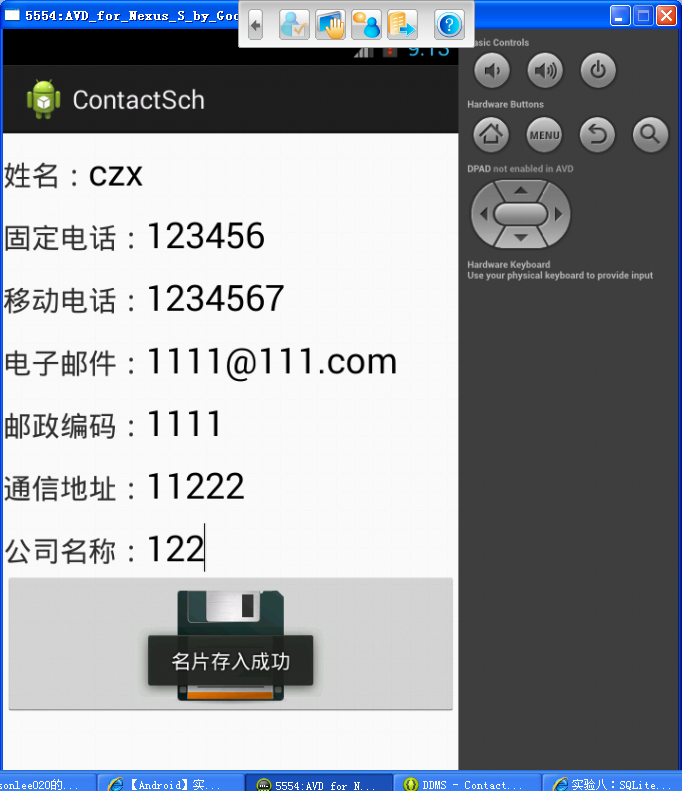

|