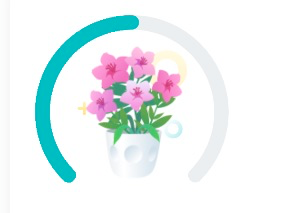
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
<canvas id="mycanvas" class="mycanvas" width="462" height="462"></canvas>
</body>
<script>
const x0 = 231 / 2 * 2 // 圆心坐标
const y0 = 231 / 2 * 2 // 圆心坐标
const r1 = (231 / 2 - 16) * 2 // 外圆半径
const r2 = (231 / 2 - 25) * 2 // 内圆半径
const startAng = 135 // 起始角度
const endAng = 45 // 终点角度
function getPointX(r, ao) {
return x0 + r * Math.cos(ao * Math.PI / 180)
}
function getPointY(r, ao) {
return y0 + r * Math.sin(ao * Math.PI / 180)
}
var canvas = document.getElementById('mycanvas');
var ctx = canvas.getContext("2d");
ctx.beginPath();
// ctx.moveTo(231/2, 231/2)
// ---------1 画大圆------------
ctx.arc(x0, y0, r1, (Math.PI / 180) * startAng, (Math.PI / 180) * endAng, false);
// ctx.lineCap = "round";
// ctx.lineWidth = '32';
ctx.strokeStyle = 'blue';
ctx.stroke();
// 获取大圆上的点 与 边界圆交点
let x1 = this.getPointX(r1, startAng)
let y1 = this.getPointY(r1, startAng)
// 获取小圆上的点 与 边界圆交点
let x2 = this.getPointX(r2, startAng)
let y2 = this.getPointY(r2, startAng)
// 边界圆上的圆心
let x3 = (x2 - x1) / 2 + x1
let y3 = (y1 - y2) / 2 + y2
// 边界圆上的半径
let r3 = (r1 - r2) / 2
// ---------2 画小圆与校园两个边界的的左侧圆------------
ctx.moveTo(x2, y2)
ctx.arc(x3, y3, r3, (Math.PI/180)*315, (Math.PI/180)*startAng, false);
ctx.stroke();
// ---------3 画小圆-----------
ctx.moveTo(x2, y2)
ctx.arc(x0, y0, r2, (Math.PI/180)*startAng, (Math.PI/180)*endAng, false);
ctx.stroke();
// 获取大圆上的点 与 边界圆交点
let xx1 = this.getPointX(r1, endAng)
let yy1 = this.getPointY(r1, endAng)
// 获取小圆上的点 与 边界圆交点
let xx2 = this.getPointX(r2, endAng)
let yy2 = this.getPointY(r2, endAng)
// 边界圆上的圆心
let xx3 = (xx1 - xx2) / 2 + xx2
let yy3 = (yy1 - yy2) / 2 + y2
// 边界圆上的半径
let rr3 = (r1 - r2) / 2
// ---------2 画小圆与校园两个边界的的左侧圆------------
ctx.moveTo(xx1, yy1)
ctx.arc(xx3, yy3, rr3, (Math.PI/180)*45, (Math.PI/180)*235, false);
ctx.stroke();
ctx.closePath()
ctx.fillStyle="green";
// ctx.fill()
</script>
<style>
.mycanvas {
231px;
height: 231px;
}
</style>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
<canvas id="mycanvas" class="mycanvas" width="462" height="462"></canvas>
</body>
<script>
const x0 = 231 / 2 * 2 // 圆心坐标
const y0 = 231 / 2 * 2 // 圆心坐标
const r1 = (231 / 2 - 16) * 2 // 外圆半径
const r2 = (231 / 2 - 25) * 2 // 内圆半径
const startAng = 135 // 起始角度
const endAng = 45 // 终点角度
var canvas = document.getElementById('mycanvas');
var ctx = canvas.getContext("2d");
ctx.beginPath();
// ---------1 画大圆------------
ctx.arc(x0, y0, r1, (Math.PI / 180) * startAng, (Math.PI / 180) * endAng, false);
ctx.lineCap = "round";
ctx.lineWidth = '32';
ctx.strokeStyle = '#ebeff0';
ctx.stroke();
// 获取大圆上的点 与 边界圆交点
let x1 = this.getPointX(r1, startAng)
let y1 = this.getPointY(r1, startAng)
ctx.moveTo(x1, y1)
var temp = startAng
window.requestAnimationFrame(() => {
return this.animDraw(ctx, temp)
});
function drawColorArc(ctx, endAng) {
ctx.beginPath();
ctx.arc(x0, y0, r1, (Math.PI / 180) * startAng, (Math.PI / 180) * endAng, false);
ctx.lineCap = "round";
ctx.lineWidth = '32';
ctx.strokeStyle = '#1dbdc4';
ctx.stroke();
}
function animDraw(ctx, temp) {
temp++
if (temp < 270) {
window.requestAnimationFrame(() => {
return this.animDraw(ctx, temp)
});
}
this.drawColorArc(ctx, temp)
}
function getPointX(r, ao) {
return x0 + r * Math.cos(ao * Math.PI /180 )
}
function getPointY(r,ao) {
return y0 + r * Math.sin(ao * Math.PI /180 )
}
</script>
<style>
.mycanvas {
231px;
height: 231px;
}
</style>
</html>