使用Python中的turtle模块绘制五角星
代码
# 画国旗上的五角星
import turtle
import math
import numpy as np
# 按照下面网址的国旗上五个五角星的相对位置绘制
# https://wenku.baidu.com/view/799890eb4afe04a1b071ded9.html
# HEIGHT 和半径相关,是网址中矩形的高度
HEIGHT = 200
width = HEIGHT * 1.5
mid_star_radius = HEIGHT * (3/10)
small_star_radius = mid_star_radius * (1/3)
# 四个小的五角星相对于中心五角星的位置
move_vectors = [(5, 3), (7, 1), (7, -2), (5, -4)]
move_vectors = [(item[0] * small_star_radius, item[1] * small_star_radius) for item in move_vectors]
def calculate_coordinate(radius):
# 默认圆心在坐标原点
# 给半出径计算五角星顶点的坐标
RADIUS = radius
# 顶点dot0为坐标为(0, RADIUM)
dots = [None for x in range(0, 5)]
dots[0] = (0, RADIUS)
# 顶点编号dot0, dot1, dot2, dot3, dot4为顺时针顺序
# 两个点之间的夹角为
angle = 2 * math.pi / 5
# dot1与x轴正向(1, 0)的夹角
angle_dot1_1_0 = math.pi / 2 - angle
dots[1] = (RADIUS * math.cos(angle_dot1_1_0), RADIUS * math.sin(angle_dot1_1_0))
# dot4 与 dot1 关于y轴对称
dots[4] = (-dots[1][0], dots[1][1])
# dot2 与 Y轴负方向的夹角
angle_dot2_0_negative_1 = math.pi - 2 * angle
dots[2] = (RADIUS * math.sin(angle_dot2_0_negative_1), - RADIUS * math.cos(angle_dot2_0_negative_1))
# dot3 与 dot2 关于y轴对称
dots[3] = (- dots[2][0], dots[2][1])
return dots
def draw_five_point_star(dots):
# 传入五个点的坐标,绘制五角星
turtle.penup()
turtle.goto(dots[0])
turtle.pendown()
turtle.begin_fill()
for i in [2, 4, 1, 3, 0]:
turtle.goto(dots[i])
turtle.end_fill()
turtle.hideturtle()
def move_rotate_star(dots, x, y, angle):
# 移动和旋转五角星
move_matrix = np.array([[x], [y]])
# 旋转矩阵
rotation_matrix = np.array([[math.cos(angle), -math.sin(angle)], [math.sin(angle), math.cos(angle)]])
dots_matrix_list = [np.array(dot).reshape(2,1) for dot in dots]
# 旋转后的坐标
rotated_dots = [np.matmul(rotation_matrix, dot) for dot in dots_matrix_list]
# 根据x,y移动后的坐标
dots_new = [dot + move_matrix for dot in rotated_dots]
dots_new = [tuple(dot.reshape(1,2).tolist()[0]) for dot in dots_new]
return dots_new
star_center = calculate_coordinate(mid_star_radius)
star_small = calculate_coordinate(small_star_radius)
turtle.setup(width*2, HEIGHT *2 )
turtle.bgcolor("red")
turtle.color("yellow", "yellow")
# 画出中间的五角星
draw_five_point_star(star_center)
# 计算出四个小的五角星顶点坐标后,根据上述网址的相对位置移动和旋转五角星
star_num = 1
for move_args in move_vectors:
if star_num < 3:
angle = math.pi - math.atan(move_args[0]/move_args[1])
else:
angle = - math.atan(move_args[0]/move_args[1])
star_small_move = move_rotate_star(star_small, *move_args, angle)
draw_five_point_star(star_small_move)
star_num += 1
turtle.done()
# todo 按照国旗上的位置,需要将图案整体向左上角移动
结果
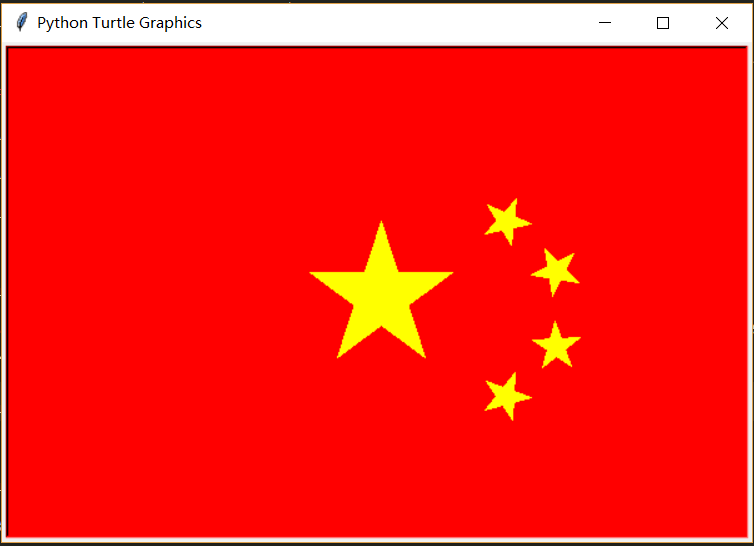