Theano 学习笔记(一)
为什么要定义共享变量?
定义共享变量的原因在于GPU的使用,如果不定义共享的话,那么当GPU调用这些变量时,遇到一次就要调用一次,这样就会花费大量时间在数据存取上,导致使用GPU代码运行很慢,甚至比仅用CPU还慢。共享变量的类型必须为floatX
因为GPU要求在floatX上操作,所以所有的共享变量都要声明为floatX类型
- shared_x = theano.shared(numpy.asarray(data_x, dtype=theano.config.floatX))
-
- self.A = theano.shared(name = "A", value = E.astype(theano.config.floatX))
创建tensor variables
在创建变量时可以给变量命名,命名能够加快debugging的过程,所有的构造器中都有name参数供命名,下面三种创建变量的方式都是创建一个整型的标量,命名为‘myvar’
- x = scalar('myvar',dtype='int32')
- x = iscalar('myvar')
- x = TensorType(dtype = 'int32', broadcastable=())('myvar')
典型构造器
- theano.tensor.scalar(name=None,dtype=config.floatX)
- theano.tensor.vector(name=None,dtype=config.floatX)
- theano.tensor.row(name=None, dtype=config.floatX)
- theano.tensor.col(name=None,dtype=config.floatX)
- theano.tensor.matrix(name=None,dtype=config.floatX)
- theano.tensor.tensor3(name=None,dtype=config.floatX) #3D张量
- theano.tensot.tensor4(name=None,dtype=config.floatX) #4D张量
导入的命名空间 from theano.tensor import *
创建新的Tensor Type
- dtensor5 = TensorType('float64',(False,)*5)
- x=dtensor5()
- z=dtensor5('z')
-
Theano requires that the inputs to all expressions be Variable instances, so Theano automatically wraps them in a TensorConstant. Thus, when you use a numpy ndarray or a python number together with TensorVariable instances in arithmetic expressions, the result is a TensorVariable.
-
broadcastable
这个东西是一个布尔有元素组成的元组,比如[False,True,False]等
broadcastable 一方面指定了类型的大小,另一方面也指定了那个维度上size只能为1(True,表示对应维度上长度只能为1,matlab: size(A,broadcasrable(i)==True)=1)
- [] scalar
- [True] 1D scalar (vector of length 1)
- [True, True] 2D scalar (1x1 matrix)
- [False] vector
- [False, False] matrix
- [False] * n nD tensor
- [True, False] row (1xN matrix)
- [False, True] column (Mx1 matrix)
- [False, True, False] A Mx1xP tensor (a)
- [True, False, False] A 1xNxP tensor (b)
- [False, False, False] A MxNxP tensor (pattern of a + b)
For dimensions in which broadcasting is False, the length of this dimension can be 1 or more.
For dimension in which broadcasting is True, the length of this dimension must be 1.
另外,broadcastable,顾名思义,与传播运算有关,当两个维度不同的参数进行元素运算时,broadcastable pattern 可以通过在tuple的左侧填充True,凑齐维度。
比如 a vector's pattern [False],可以扩展成 [True, False]; a matrix pattern [False,False]可以扩展成 [True, False,False]
然后对应的True维度上就可以broad了
这样学起来实在是太慢了。。。。。。。。。。。
总觉得python乱,还是读代码吧,边读边学。。。
- import theano
- import theano.tensor as T
- x=T.matrix('x')
- s=1/(1+t.exp(-x))
- logistic = theano.function([x],s)
- logistic([[1,2,3],[4,3,0.5]])
结果

学到的点:
声明符号变量x,名称为'x',这个我们也说了主要是为了GPU调度方便
+,-,*,/,包括exp等函数都是元素操作的
logistic相当于一个函数句柄,这个函数的输入是x,输出是s, x到s的关系根据前面定义可得
- import theano
- import theano.tensor as T
- a,b=T.matrices('a','b')
- diff = a-b
- abs_diff = abs(diff)
- diff_squared = diff**2
- f = theano.function([a,b],[diff,abs_diff,diff_squared])
点:
theano 可以定义多输入多输出,类似的matlab代码
- function [diff,abs_diff,diff_squared]=f(a,b)
- ...
- end
matrices 是同时声明参数个数个变量,类似的还有scalars,vectors等
- f([[1,2,3],[2,1,1]])
输出结果

可以看到输出的是个tuple,正对应输出指定变量
- import theano
- import theano.tensor as T
- from theano import In
- x,y = T.scalars('x','y')
- z=x+y
- f= theano.function([x,In(y,value=1)],z)
- f(2)
- f(2,5)
输出结果
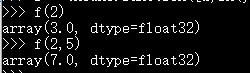
新知识点:
In 这个类能够更详细的指定函数的参数,比如默认值。 函数中给定默认值的参数应该放在没给定默认值参数的后面,c++也这样。
In 还能够重新给参数命名,这样就能够使函数输入,假设2-4个输入是有默认值的,但我2,3想使用默认值,第4个不想使用默认值时,不用对2,3赋值了,比如
- x,y,w= T.scalars('x','y','w')
- z=(x+y)*w
- f=T.function([x,In(y,value=1),In(w,value=2,name='w_byname')],z)
那么f(33,1,5)和f(33,w_by_name=5)结果时一样的
这里为什么w已经有名字'w'了,还要再定义名字呢?
主要是由于In并不知道局部变量x,y,w的名字,函数输入参数其实就是一个字典,keyword是scalar默认的姓名属性,这里In是重写了参数的keyword
- from theano import shared
- from theano import In
- import theano
- import theano.tensor as T
- state = shared(0)
- inc = T.scalar('inc')
- accumular = function([inc],state,update=[(state,state+inc)])
新知识点:
shared 这个函数声明的是一个符号和变量的结合体,也就说他直接赋值了,内部有一定的值参与运算。另外之所以称为shared是因为这个量是可以多个函数共享的,有点像c++里面的静态变量,在一个函数里面修改了这个量,后面使用这个量的函数就是在新的值上运算了。
shared变量可以通过.set_value()和.get_value()设置和读取状态值updates是一个list量,他的元素是(shared_variable,new expression)对,有点像字典,每次对keyword对应的值更新,注意
这里是先返回的state,再进行的state=state+inc,看下面的代码结果
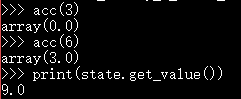
- >>> fn_of_state = state * 2 + inc
- >>> # The type of foo must match the shared variable we are replacing
- >>> # with the ``givens``
- >>> foo = T.scalar(dtype=state.dtype)
- >>> skip_shared = function([inc, foo], fn_of_state, givens=[(state, foo)])
- >>> skip_shared(1, 3) # we're using 3 for the state, not state.value
- array(7)
- >>> print(state.get_value()) # old state still there, but we didn't use it
- 0
知识点:
这个蛮有意思的,就是说我现在有个函数,函数里面的计算牵涉到state变量,但是我不想使用state当前的值,那么我就可以使用function的givens参数另一个值暂且替代state
看上面这个代码,我使用state表示的fn_of_state,但是计算时不想使用当前state的值,而是使用3,于是使用givens令新变量foo替代state占的位置,所以得到的结果fn_of_state=foo*2+inc=7
而state.get_value()值仍然为state的原先值,这里是0这里的givens不仅仅可以适用于shared,还可以适用于任意的symbolic variable,要注意的是在givens的list里面替代的量要相互独立,要不然就不知道怎么替代了
shared变量默认的是broadcastable=False,所以要想使用broadcasrable pattern,需要特别指定,如
theano.shared(...,broadcastable=(True,False))
1