一.作业部分
1.负载均衡程序
2.演示视频
3.程序分工
- 小组:incredible five
- 构建拓扑:俞鋆
- 编写程序:陈绍纬、周龙荣
- 程序调试和视频录制:陈辉、林德望
4.个人工作
- 在期末作业中我和绍伟主要负责程序代码的编写,在做期末作业的过程中我们小组聚在一起开了两次会议,第一次确定要做的内容并分工,第二次将自己的部分和别人的对接。关于代码部分,我们参考了学长的代码,然后对于我们的拓扑结构进行了初步的构思,慢慢开始和队友合作一起编写代码。由于对python语言的不熟悉,编写代码的过程中还是查找了不少资料也和队友一起讨论了多次,最终完成了代码,并虚拟机上运行,和大家一起完成了验收。
- 程序思路
场景二默认包从s1-s4路径发送,所以先给s2、s3下发流表,使之通行。(s2、s3流表链接)。我们小组没有去底层交换机收集信息,再对数据处理得到动态负载均衡,最后经过小组讨论,将s1-s4、s1-s2-s4、s1-s3-s4三条线路默认1:2:2的关系,以经历的线路为基准进行负载均衡,对s4下发流表,使用hardtime机制,让3条线路在一段时间内的占比为2:1:1以达到负载均衡。
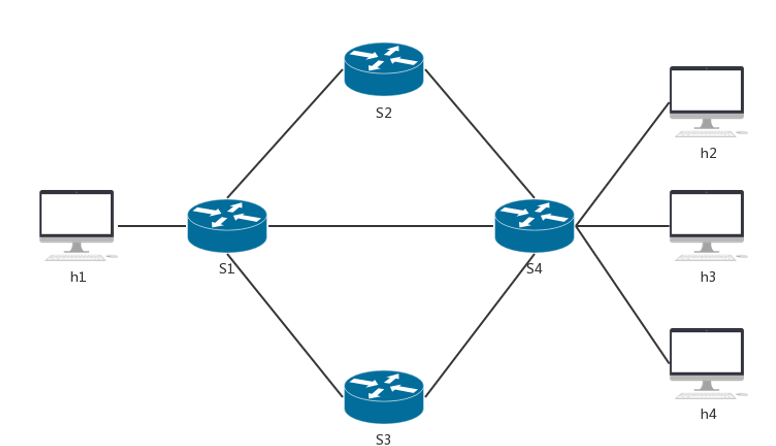
from mininet.topo import Topo
class MyTopo( Topo ):
def __init__( self ):
# Initialize topology
Topo.__init__( self )
# Add hosts and switches
h1 = self.addHost( 'h1' )
h2 = self.addHost( 'h2' )
h3 = self.addHost( 'h3' )
h4 = self.addHost( 'h4' )
s1 = self.addSwitch( 's1' )
s2 = self.addSwitch( 's2' )
s3 = self.addSwitch( 's3' )
s4 = self.addSwitch( 's4' )
# Add links
self.addLink( s1, h1, 1, 0 )
self.addLink( s1, s2, 2, 1 )
self.addLink( s1, s3, 3, 1 )
self.addLink( s1, s4, 4, 1 )
self.addLink( s2, s4, 2, 2 )
self.addLink( s3, s4, 2, 3 )
self.addLink( s4, h2, 4, 0 )
self.addLink( s4, h3, 5, 0 )
self.addLink( s4, h4, 6, 0 )
topos = { 'mytopo': ( lambda: MyTopo() ) }
#!/usr/bin/python
# -*- coding:UTF-8 -*-
import httplib2
import time
class OdlUtil:
http = httplib2.Http()
url = ''
def __init__(self, host, port):
self.url = 'http://' + host + ':' + port
def install_flow(self, container_name='default',username="admin", password="admin"):
self.http.add_credentials(username, password)
flow_name = 'flow_' + str(int(time.time()*1000))
h2_s4_s2_1 = '''
{"flow-node-inventory:flow": [
{"id": "1","flow-name": "s4_s2_1","cookie": 256,
"hard-timeout":4,"instructions": {
"instruction": [{"order": 0,"apply-actions": {
"action": [{"order": 0,"output-action": {
"output-node-connector": "2"}}]}}]},
"priority": 1000,"table_id": 0,"match": {
"ipv4-destination": "10.0.0.1/32","in-port": "4",
"ethernet-match": {"ethernet-type": {"type": 2048}}}}]} '''
h2_s4_s1_1 = '''
{"flow-node-inventory:flow": [
{"id": "0","flow-name": "s4_s2_1","cookie": 256,
"hard-timeout":2,"instructions": {
"instruction": [{"order": 0,"apply-actions": {
"action": [{"order": 0,"output-action": {
"output-node-connector": "1"}}]}}]},
"priority": 1005,"table_id": 0,"match": {
"ipv4-destination": "10.0.0.1/32","in-port": "4",
"ethernet-match": {"ethernet-type": {"type": 2048}}}}]} '''
h2_s4_s3_1 = '''
{"flow-node-inventory:flow": [
{"id": "2","flow-name": "s4_s2_1","cookie": 256,
"hard-timeout":3,"instructions": {
"instruction": [{"order": 0,"apply-actions": {
"action": [{"order": 0,"output-action": {
"output-node-connector": "3"}}]}}]},
"priority": 1002,"table_id": 0,"match": {
"ipv4-destination": "10.0.0.1/32","in-port": "4",
"ethernet-match": {"ethernet-type": {"type": 2048}}}}]} '''
h3_s4_s2_1='''
{"flow-node-inventory:flow": [
{"id": "4","flow-name": "s4_s2_1","cookie": 256,
"hard-timeout":4,"instructions": {
"instruction": [{"order": 0,"apply-actions": {
"action": [{"order": 0,"output-action": {
"output-node-connector": "2"}}]}}]},
"priority": 1000,"table_id": 0,"match": {
"ipv4-destination": "10.0.0.1/32","in-port": "5",
"ethernet-match": {"ethernet-type": {"type": 2048}}}}]} '''
h3_s4_s1_1 = '''
{"flow-node-inventory:flow": [
{"id": "3","flow-name": "s4_s2_1","cookie": 256,
"hard-timeout":2,"instructions": {
"instruction": [{"order": 0,"apply-actions": {
"action": [{"order": 0,"output-action": {
"output-node-connector": "1"}}]}}]},
"priority": 1005,"table_id": 0,"match": {
"ipv4-destination": "10.0.0.1/32","in-port": "5",
"ethernet-match": {"ethernet-type": {"type": 2048}}}}]} '''
h3_s4_s3_1 = '''
{"flow-node-inventory:flow": [
{"id": "5","flow-name": "s4_s2_1","cookie": 256,
"hard-timeout":3,"instructions": {
"instruction": [{"order": 0,"apply-actions": {
"action": [{"order": 0,"output-action": {
"output-node-connector": "3"}}]}}]},
"priority": 1002,"table_id": 0,"match": {
"ipv4-destination": "10.0.0.1/32","in-port": "5",
"ethernet-match": {"ethernet-type": {"type": 2048}}}}]} '''
h4_s4_s2_1 = '''
{"flow-node-inventory:flow": [
{"id": "7","flow-name": "s4_s2_1","cookie": 256,
"hard-timeout":4,"instructions": {
"instruction": [{"order": 0,"apply-actions": {
"action": [{"order": 0,"output-action": {
"output-node-connector": "2"}}]}}]},
"priority": 1000,"table_id": 0,"match": {
"ipv4-destination": "10.0.0.1/32","in-port": "6",
"ethernet-match": {"ethernet-type": {"type": 2048}}}}]}
'''
h4_s4_s1_1 = '''
{"flow-node-inventory:flow": [
{"id": "6","flow-name": "s4_s2_1","cookie": 256,
"hard-timeout":2,"instructions": {
"instruction": [{"order": 0,"apply-actions": {
"action": [{"order": 0,"output-action": {
"output-node-connector": "1"}}]}}]},
"priority": 1005,"table_id": 0,"match": {
"ipv4-destination": "10.0.0.1/32","in-port": "6",
"ethernet-match": {"ethernet-type": {"type": 2048}}}}]} '''
h4_s4_s3_1 = '''
{"flow-node-inventory:flow": [
{"id": "8","flow-name": "s4_s2_1","cookie": 256,
"hard-timeout":3,"instructions": {
"instruction": [{"order": 0,"apply-actions": {
"action": [{"order": 0,"output-action": {
"output-node-connector": "3"}}]}}]},
"priority": 1002,"table_id": 0,"match": {
"ipv4-destination": "10.0.0.1/32","in-port": "6",
"ethernet-match": {"ethernet-type": {"type": 2048}}}}]} '''
headers = {'Content-type': 'application/json'}
num=0
while num < 4 :
response, content = self.http.request(uri='http://192.168.81.133:8181/restconf/config/opendaylight-inventory:nodes/node/openflow:4/flow-node-inventory:table/0/flow/0', body=h2_s4_s1_1, method='PUT',headers=headers)
response, content = self.http.request(uri='http://192.168.81.133:8181/restconf/config/opendaylight-inventory:nodes/node/openflow:4/flow-node-inventory:table/0/flow/1', body=h2_s4_s2_1, method='PUT',headers=headers)
response, content = self.http.request(uri='http://192.168.81.133:8181/restconf/config/opendaylight-inventory:nodes/node/openflow:4/flow-node-inventory:table/0/flow/2', body=h2_s4_s3_1, method='PUT',headers=headers)
response, content = self.http.request(uri='http://192.168.81.133:8181/restconf/config/opendaylight-inventory:nodes/node/openflow:4/flow-node-inventory:table/0/flow/3', body=h3_s4_s1_1, method='PUT',headers=headers)
response, content = self.http.request(uri='http://192.168.81.133:8181/restconf/config/opendaylight-inventory:nodes/node/openflow:4/flow-node-inventory:table/0/flow/4', body=h3_s4_s2_1, method='PUT',headers=headers)
response, content = self.http.request(uri='http://192.168.81.133:8181/restconf/config/opendaylight-inventory:nodes/node/openflow:4/flow-node-inventory:table/0/flow/5', body=h3_s4_s3_1, method='PUT',headers=headers)
response, content = self.http.request(uri='http://192.168.81.133:8181/restconf/config/opendaylight-inventory:nodes/node/openflow:4/flow-node-inventory:table/0/flow/6', body=h4_s4_s1_1, method='PUT',headers=headers)
response, content = self.http.request(uri='http://192.168.81.133:8181/restconf/config/opendaylight-inventory:nodes/node/openflow:4/flow-node-inventory:table/0/flow/7', body=h4_s4_s2_1, method='PUT',headers=headers)
response, content = self.http.request(uri='http://192.168.81.133:8181/restconf/config/opendaylight-inventory:nodes/node/openflow:4/flow-node-inventory:table/0/flow/8', body=h4_s4_s3_1, method='PUT',headers=headers)
print("success")
time.sleep(4)
print(content.decode())
if __name__=='__main__':
odl = OdlUtil('192.168.81.133', '8181')
odl.install_flow()
二.课程总结
个人总结
- 在上个学期选这门课的时候并没有对这门课有过多的了解,对于网络编程也是知之甚少,本着了解新的专业知识有一定的必要性的心态选了这门课。在课程开始后随着课程的不断深入,慢慢对于软件定义网络有了一定的深入认识,尤其是理论和实践结合的方式,在实践的过程中更能加深对于理论的理解。老师布置的关于SDN方面课外资料阅读的博客作业,也帮助扩展了我的知识面。回顾整个课程期间的点点滴滴,总的来说还是收获了不少。
关于SDN
- 软件定义网络(Software Defined Network, SDN ),是一种集中控制的新型网络创新架构,是网络虚拟化的一种实现方式,SDN的核心技术OpenFlow通过将网络设备控制面与数据面分离开来,从而实现了网络流量的灵活控制,使网络作为管道变得更加智能
关于控制器
- Ryu控制器:
Ryu是基于Python语言的开源SDN控制器,提供完备的API,支持多种网络管理设备协议如OpenFlow,Netconf,OF-CO NFIG等多种南向协议。
Ryu构架分3层。最上层Quantum与OF Rest分别为OpenStack和Web提供编程接口;中间层为Ryu自行研发的应用组件;最下层为Ryu底层实现的基本组件。
- Floodlight控制器:
Floodlight是基于Java语言的开源SDN控制器,当前支持的南向协议为OpenFlow1.0协议。Floodlight与Big Switch Networks开发的商用控制器Big Switch Controller的API接口完全兼容,具有较好的可移植性。也以其企业级别的优秀性能,开发效率更高的Java语言,模块化的设计等优点得到了喜欢Java语言的SDN研究者的青睐。
- ONOS控制器:
ONOS控制器采用Java编写,由一系列功能模块组成,每个功能模块由一个或多个组件组成,对外提供一种特定服务,核心功能主要包含:北向接口抽象层/APIs,分布式核心,南向接口抽象层/APIs,软件模块化。
ONOS控制器的服务对象是运营商,它具有高可靠性、高扩展性、高实时性以及高性能ONOS控制器的服务对象是运营商,它具有高可靠性、高扩展性、高实时性以及高性能。
- ODL控制器:
OpenDaylight控制器采用Java编写,支持多种南向协议,包括OpenFlow(支持1.0和1.3版本)、Netconf和OVSDB等,是一个广义的SDN控制平台,而不是OpenFlow系的狭义SDN控制器。
Opendayligh构架也分3层:顶层由控制器和监控网络行为的业务和网络逻辑应用构成;中间层是SDN控制器框架层,其南向协议接口可以支持不同南向协议插件,这些协议插件动态链接到SAL,SAL适配后再同意北向接口供上层应用调用;底层由物理或虚拟设备构成。
关于实践