Ultra-QuickSort POJ 2299
Time Limit: 7000MS | Memory Limit: 65536K | |
Total Submissions: 50495 | Accepted: 18525 |
Description
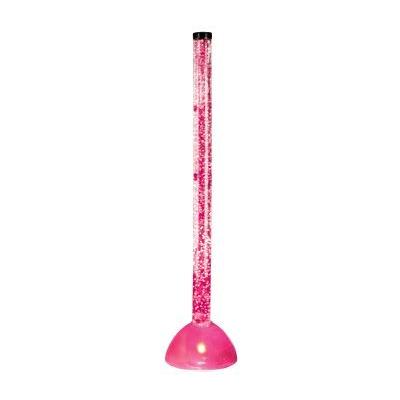
Ultra-QuickSort produces the output
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.
Input
The input contains several test cases. Every test case begins with a line that contains a single integer n < 500,000 -- the length of the input sequence. Each of the the following n lines contains a single integer 0 ≤ a[i] ≤ 999,999,999, the i-th input sequence element. Input is terminated by a sequence of length n = 0. This sequence must not be processed.
Output
For every input sequence, your program prints a single line containing an integer number op, the minimum number of swap operations necessary to sort the given input sequence.
Sample Input
5
9
1
0
5
4
3
1
2
3
0
Sample Output
6
0
树状数组维护:c[i]存储比i小的数目.
离散化: 比如输入9 1 0 5 4,将其转化为 5 2 1 4 3,方便存储。
参考别人的代码,看了好半天!
1 #include <cstdio> 2 #include <iostream> 3 #include <algorithm> 4 #include <cstring> 5 #define LL long long 6 #define Max 500000+10 7 using namespace std; 8 struct node 9 { 10 int num,order; 11 }; 12 int n; 13 int c[Max]; 14 node in[Max]; 15 int t[Max]; 16 int cmp(node a,node b) 17 { 18 return a.num<b.num; 19 } 20 void add(int i,int a) 21 { 22 while(i<=n) 23 { 24 t[i]+=a; 25 i+=i&-i; 26 } 27 } 28 int sum(int i) 29 { 30 int s=0; 31 while(i>=1) 32 { 33 s+=t[i]; 34 i-=i&-i; 35 } 36 return s; 37 } 38 int main() 39 { 40 int i,j; 41 freopen("in.txt","r",stdin); 42 while(~scanf("%d",&n)&&n) 43 { 44 memset(t,0,sizeof(t)); 45 for(i=1;i<=n;i++) 46 { 47 scanf("%d",&in[i].num); 48 in[i].order=i; 49 } 50 sort(in+1,in+1+n,cmp); 51 for(i=1;i<=n;i++) /*离散化*/ 52 c[in[i].order]=i; 53 LL s=0; 54 for(i=1;i<=n;i++) 55 { 56 add(c[i],1); 57 s+=i-sum(c[i]); 58 // cout<<s<<endl; 59 60 } 61 printf("%lld ",s); 62 } 63 }