## 函数的四种调用模式
1.函数模式
this--->window
this--->window
function test(){ console.log(this); } test();
this---->调用方法的对象
var obj1 = { test:function(){ console.log(this); } } obj1.test();
this----->new出来的对象
function Person(){ console.log(this); } var obj =new Person();
function Person(name,age){ var o = { name:name, age:age, sayHello:function(){ } } return o; } var p = Person("张三", 18); console.log(p); //object //简单工厂模式的构造函数 创建出来的对象 跟该构造函数无关 //简单工厂模式的构造函数,实际的调用模式是 函数模式
寄生模式
function Person(name,age){ var o = { name:name, age:age, sayHello:function(){ } } return o; } var p = new Person();
this----->指定的是谁就是谁 此模式下可以修改this的值,也就是说可以修改函数的调用方式。
修改方式:
call 函数.call(对象,arg1,arg2,arg3,...argn)
apply 函数.apply(对象,数组)
都可以用来改变this的指向为参数的第一个值
call是使用单独的每一个参数来传参
apply是使用数组进行传参的,这个数组在调用的时候,会被意义拆解,当做函数的每一个采参数
apply 函数.apply(对象,数组)
都可以用来改变this的指向为参数的第一个值
call是使用单独的每一个参数来传参
apply是使用数组进行传参的,这个数组在调用的时候,会被意义拆解,当做函数的每一个采参数
call在函数的形参个数确定的情况下使用
apply在函数的形参个数不确定的情况下使用
apply在函数的形参个数不确定的情况下使用
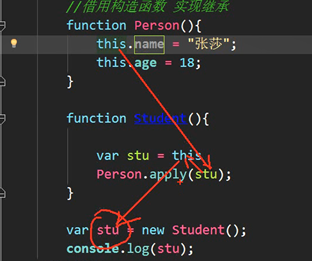
上下文: //案例:求一个数组中的最大值 var arr = [9, 1, 4, 10, 7, 22, 8]; //apply方法和call方法第一个参数传递null的时候,都表示为函数调用模式 //也就是将this指向window var max = Math.max.apply(null, arr); console.log(max); //案例:将传入的参数打印,参数之间用-相互连接 function foo() { // return arguments.join("-"); //伪数组不具有join方法,所以这个时候就要考虑去借用一下数组的join方法 // var str = Array.prototype.join.apply(arguments,["-"]); var str = [].join.apply(arguments,["-"]); return str; } var str = foo(1, 3, "abc", "ffff", 99) // 1-3-abc-ffff-99 console.log(str); // var arr = [1,2,3,4]; // console.log(arr.join("-")); window.onload = function () { //案例:给页面上所有的 div 和 p 标签添加背景色 var divs = document.getElementsByTagName("div");//divs是伪数组 var ps = document.getElementsByTagName("p"); var arr = []; //little tip: push方法可以传多个参数 //arr.push(1,2,3,4,4,5) arr.push.apply(arr,divs); arr.push.apply(arr,ps); //如果使用arr.push()直接把divs传进来 //那么相当于在arr中的第一个元素中存储了一个divs数组 //但是我们需要把divs中的每一个元素单独的存入arr中 //所以需要调用push方法的如下形式 push(1,2,4,4,5) //要实现这个形式的调用,就用到了apply方法的第二个参数的特性 //在调用的时候,会第二个参数的数组,拆成每一个元素以(a,b,c,d,e,f,g) 传入函数 //相当于 arr.push(divs[0],divs[1],divs[..]) // arr.push(divs) for (var k = 0; k < arr.length; k++) { var ele = arr[k]; ele.style.backgroundColor = "yellow"; }