C# 的 Http 访问可以使用 .net 自带的 HttpWebRequest, WebClient, HttpClient 类。也可以使用开源库 RestSharp 。
RestSharp 的优点很多,最重要的一点是对于新手来说,Postman 直接提供了 RestSharp 的实现代码。
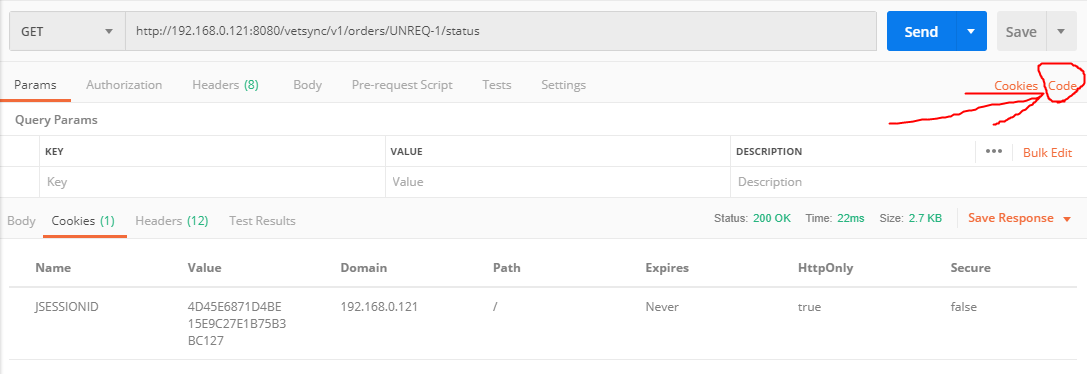
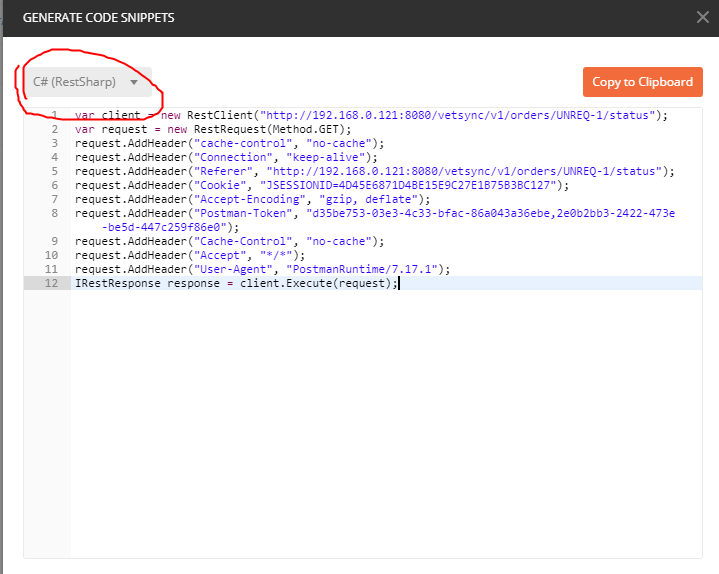
下面给出了网站的登录与 Cookie 的获取使用的方式,分别用 WebRequest 和 RestSharp 实现
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Net;
using System.Threading;
using System.IO;
using System.Net.Http;
using RestSharp;
namespace WebServer
{
class Program
{
static void Main(string[] args)
{
if (true)
{
//使用RestSharp
RestResponseCookie cookie = null;
string html = GetHtml("http://xd:1234@192.168.0.121:8080/vetsync/v1/orders", out cookie);
Console.WriteLine(html);
html = GetHtml("http://192.168.0.121:8080/vetsync/v1/orders", cookie);
Console.WriteLine(html);
}
else
{
//使用WebRequest
string cookie;
string html = GetHtml("http://192.168.0.121:8080/vetsync/v1/orders", "xd", "1234", out cookie);
Console.WriteLine(html);
html = GetHtml("http://192.168.0.121:8080/vetsync/v1/orders", cookie);
Console.WriteLine(html);
}
Console.ReadLine();
}
//第一次使用用户名密码登录(在 url 中),获得cookie
public static string GetHtml(string url, out RestResponseCookie cookie)
{
var client = new RestClient(url);
RestRequest request = new RestRequest(Method.GET);
request.AddHeader("Authorization", "Basic eGQ6MTIzNA==");
IRestResponse response = client.Execute(request);
cookie = response.Cookies[0];
return response.Content;
}
//第二次及以后访问使用cookie验证
public static string GetHtml(string url, RestResponseCookie cookie)
{
var client = new RestClient(url);
RestRequest request = new RestRequest(Method.GET);
request.AddCookie(cookie.Name, cookie.Value);
IRestResponse response = client.Execute(request);
return response.Content;
}
//第一次使用用户名密码登录,获得cookie
public static string GetHtml(string url, string userName, string password, out string cookie)
{
WebRequest request = WebRequest.Create(url);
request.Credentials = CredentialCache.DefaultCredentials;
string code = Convert.ToBase64String(Encoding.Default.GetBytes(string.Format("{0}:{1}", userName, password)));
request.Headers.Add("Authorization", "Basic " + code);
WebResponse wrp = request.GetResponse();
cookie = wrp.Headers.Get("Set-Cookie");
return new StreamReader(wrp.GetResponseStream(), Encoding.Default).ReadToEnd();
}
//第二次及以后访问使用cookie验证
public static string GetHtml(string url, string cookieStr)
{
WebRequest wrt = WebRequest.Create(url);
wrt.Credentials = CredentialCache.DefaultCredentials;
wrt.Headers.Add("Cookie", cookieStr);
WebResponse wrp = wrt.GetResponse();
return new StreamReader(wrp.GetResponseStream(), Encoding.Default).ReadToEnd();
}
}
}