序列化
Struct(结构体) → Json
//结构体序列化为json
p := &People{
Name: "张三",
Age: 16,
}
StructJSON, err := json.Marshal(p)
if err != nil {
fmt.Println("结构体序列化为json失败...", err)
return
}
fmt.Println("↓结构体序列化为json后:↓")
fmt.Println(string(StructJSON))
fmt.Println()
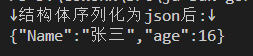
Map集合(key,value) → Json
//map集合序列化为json
MapJSON := make(map[string]interface{})
MapJSON["name"] = "独眼蝙蝠"
MapJSON["lv"] = 1
MapJSON["hp"] = 100
mapJSONRes, err2 := json.Marshal(MapJSON)
if err2 != nil {
fmt.Println("map集合序列化为json失败...", err2)
return
}
fmt.Println("↓map集合序列化为json后:↓")
fmt.Println(string(mapJSONRes))
fmt.Println()
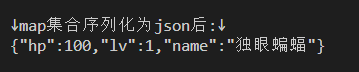
Map切片(多个map(key,value)) → Json
//map切片序列化为json
MapJSONSlice := make([]map[string]interface{}, 2)
if MapJSONSlice[0] == nil {
MapJSONSlice[0] = make(map[string]interface{})
MapJSONSlice[0]["name"] = "独眼蝙蝠"
MapJSONSlice[0]["lv"] = 1
MapJSONSlice[0]["hp"] = 100
MapJSONSlice[0]["goods"] = []string{"棒棒糖(HP恢复60)", "木剑(攻击力+3)", "四点色子"}
}
if MapJSONSlice[1] == nil {
MapJSONSlice[1] = make(map[string]interface{})
MapJSONSlice[1]["name"] = "彭哚菇"
MapJSONSlice[1]["lv"] = 3
MapJSONSlice[1]["hp"] = 300
MapJSONSlice[1]["goods"] = []string{"草莓圣代(HP恢复80)", "铁剑(攻击力+5)", "六点色子"}
}
mapJSONSliceRes, err3 := json.Marshal(MapJSONSlice)
if err3 != nil {
fmt.Println("map切片集合序列化为json失败...", err3)
return
}
fmt.Println("↓map切片集合序列化为json后:↓")
fmt.Println(string(mapJSONSliceRes))
fmt.Println()

反序列化
json.Unmarshal([]byte(json字符串), &接收体指针)
Json → Struct(结构体)
//People 结构体
type People struct {
Name string `jsno:"name"`
Age int `json:"age"`
}
1 //将json字符串 反序列化到 struct结构体
2 jsonStructStr := `{"name":"tom","age":22}`
3 p := &People{}
4 err := json.Unmarshal([]byte(jsonStructStr), &p)
5 if err != nil {
6 fmt.Println("Unmarshal jsonStr err!", err)
7 return
8 }
9 fmt.Println("↓struct结构体↓")
10 fmt.Println(p)
11 fmt.Println(p.Name)
12 fmt.Println()
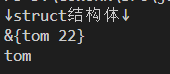
Json → Map(集合,key,value)
1 //将json字符串 反序列化 到map集合
2 jsonMapStr := `{"goodsName":"华为P40","price":5999,"color":"蓝色"}`
3 ResMap := map[string]interface{}{}
4 err2 := json.Unmarshal([]byte(jsonMapStr), &ResMap)
5 if err2 != nil {
6 fmt.Println("Unmarshal jsonMap err!", err2)
7 return
8 }
9 fmt.Println("↓map集合↓")
10 fmt.Println(ResMap)
11 fmt.Println(ResMap["goodsName"])
12 fmt.Println()
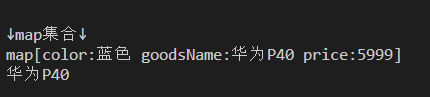
Json → Map切片(多个map集合,key,value)
1 //将json字符串 反序列化 到map切片集合
2 jsonMapSliceStr := `[{"goodsName":"华为Mate40","price":4500,"color":"橄榄绿"},{"goodsName":"小米11","price":5999,"color":"青春蓝"}]`
3 ResMapSlice := make([]map[string]interface{}, 2)
4
5 err3 := json.Unmarshal([]byte(jsonMapSliceStr), &ResMapSlice)
6 if err3 != nil {
7 fmt.Println("Unmarshal jsonMapSlice err!", err3)
8 return
9 }
10 fmt.Println("↓map切片集合↓")
11 fmt.Println(ResMapSlice)
12 fmt.Println(ResMapSlice[0])
13 fmt.Println()
