The Same Game
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 4585 | Accepted: 1699 |
Description
The game named "Same" is a single person game played on a 10 Theta 15 board. Each square contains a ball colored red (R), green (G), or blue (B). Two balls belong to the same cluster if they have the same color, and one can be reached from another by following balls of the same color in the four directions up, down, left, and right. At each step of the game, the player chooses a ball whose cluster has at least two balls and removes all balls in the cluster from the board. Then, the board is "compressed" in two steps:
1. Shift the remaining balls in each column down to fill the empty spaces. The order of the balls in each column is preserved.
2. If a column becomes empty, shift the remaining columns to the left as far as possible. The order of the columns is preserved.
For example, choosing the ball at the bottom left corner in the sub-board below causes:
The objective of the game is to remove every ball from the board, and the game is over when every ball is removed or when every cluster has only one ball. The scoring of each game is as follows. The player starts with a score of 0. When a cluster of m balls is removed, the player's score increases by (m-2)^2 . A bonus of 1000 is given if every ball is removed at the end of the game.
You suspect that a good strategy might be to choose the ball that gives the largest possible cluster at each step, and you want to test this strategy by writing a program to simulate games played using this strategy. If there are two or more balls to choose from, the program should choose the leftmost ball giving the largest cluster. If there is still a tie, it should choose the bottommost ball of these leftmost balls.
1. Shift the remaining balls in each column down to fill the empty spaces. The order of the balls in each column is preserved.
2. If a column becomes empty, shift the remaining columns to the left as far as possible. The order of the columns is preserved.
For example, choosing the ball at the bottom left corner in the sub-board below causes:
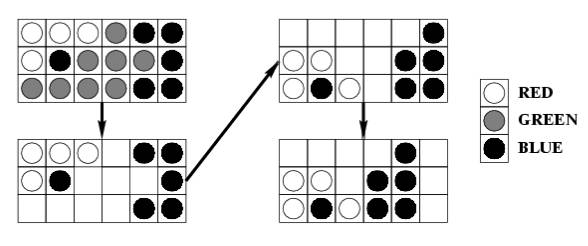
The objective of the game is to remove every ball from the board, and the game is over when every ball is removed or when every cluster has only one ball. The scoring of each game is as follows. The player starts with a score of 0. When a cluster of m balls is removed, the player's score increases by (m-2)^2 . A bonus of 1000 is given if every ball is removed at the end of the game.
You suspect that a good strategy might be to choose the ball that gives the largest possible cluster at each step, and you want to test this strategy by writing a program to simulate games played using this strategy. If there are two or more balls to choose from, the program should choose the leftmost ball giving the largest cluster. If there is still a tie, it should choose the bottommost ball of these leftmost balls.
Input
You will be given a number of games in the input. The first line of input contains a positive integer giving the number of games to follow. The initial arrangement of the balls of each game is given one row at a time, from top to bottom. Each row contains 15 characters, each of which is one of "R", "G", or "B", specifying the colors of the balls in the row from left to right. A blank line precedes each game.
Output
For each game, print the game number, followed by a new line, followed by information about each move, followed by the final score. Each move should be printed in the format:
Move x at (r,c): removed b balls of color C, got s points.
where x is the move number, r and c are the row number and column number of the chosen ball, respectively. The rows are numbered from 1 to 10 from the bottom, and columns are numbered from 1 to 15 from the left. b is the number of balls in the cluster removed. C is one of "R", "G", or "B", indicating the color of the balls removed. s is the score for this move. The score does not include the 1000 point bonus if all the balls are removed after the move.
The final score should be reported as follows:
Final score: s, with b balls remaining.
Insert a blank line between the output of each game. Use the plural forms "balls" and "points" even if the corresponding value is 1.
Move x at (r,c): removed b balls of color C, got s points.
where x is the move number, r and c are the row number and column number of the chosen ball, respectively. The rows are numbered from 1 to 10 from the bottom, and columns are numbered from 1 to 15 from the left. b is the number of balls in the cluster removed. C is one of "R", "G", or "B", indicating the color of the balls removed. s is the score for this move. The score does not include the 1000 point bonus if all the balls are removed after the move.
The final score should be reported as follows:
Final score: s, with b balls remaining.
Insert a blank line between the output of each game. Use the plural forms "balls" and "points" even if the corresponding value is 1.
Sample Input
3 RGGBBGGRBRRGGBG RBGRBGRBGRBGRBG RRRRGBBBRGGRBBB GGRGBGGBRRGGGBG GBGGRRRRRBGGRRR BBBBBBBBBBBBBBB BBBBBBBBBBBBBBB RRRRRRRRRRRRRRR RRRRRRGGGGRRRRR GGGGGGGGGGGGGGG RRRRRRRRRRRRRRR RRRRRRRRRRRRRRR GGGGGGGGGGGGGGG GGGGGGGGGGGGGGG BBBBBBBBBBBBBBB BBBBBBBBBBBBBBB RRRRRRRRRRRRRRR RRRRRRRRRRRRRRR GGGGGGGGGGGGGGG GGGGGGGGGGGGGGG RBGRBGRBGRBGRBG BGRBGRBGRBGRBGR GRBGRBGRBGRBGRB RBGRBGRBGRBGRBG BGRBGRBGRBGRBGR GRBGRBGRBGRBGRB RBGRBGRBGRBGRBG BGRBGRBGRBGRBGR GRBGRBGRBGRBGRB RBGRBGRBGRBGRBG
Sample Output
Game 1: Move 1 at (4,1): removed 32 balls of color B, got 900 points. Move 2 at (2,1): removed 39 balls of color R, got 1369 points. Move 3 at (1,1): removed 37 balls of color G, got 1225 points. Move 4 at (3,4): removed 11 balls of color B, got 81 points. Move 5 at (1,1): removed 8 balls of color R, got 36 points. Move 6 at (2,1): removed 6 balls of color G, got 16 points. Move 7 at (1,6): removed 6 balls of color B, got 16 points. Move 8 at (1,2): removed 5 balls of color R, got 9 points. Move 9 at (1,2): removed 5 balls of color G, got 9 points. Final score: 3661, with 1 balls remaining. Game 2: Move 1 at (1,1): removed 30 balls of color G, got 784 points. Move 2 at (1,1): removed 30 balls of color R, got 784 points. Move 3 at (1,1): removed 30 balls of color B, got 784 points. Move 4 at (1,1): removed 30 balls of color G, got 784 points. Move 5 at (1,1): removed 30 balls of color R, got 784 points. Final score: 4920, with 0 balls remaining. Game 3: Final score: 0, with 150 balls remaining.
令人恶心的模拟,也许在大神们看来是水题,但我还是WA了很久,回头想想也不是太难,就是各种小错误,各种想不到的情况,最后用了别人的测试数据才找出错误,哎。
1 #include <iostream> 2 #include <cstring> 3 #include <stdio.h> 4 5 using namespace std; 6 7 int step[4][2]={{1,0},{-1,0},{0,1},{0,-1}}; 8 9 int board[11][16],cnt; 10 bool vis[11][16],marked[11][16]; 11 12 void cnt_ball(int x,int y,int cl) 13 { 14 int i; 15 vis[x][y]=true; 16 for(i=0;i<4;i++) 17 { 18 int tx=x+step[i][0],ty=y+step[i][1]; 19 if(tx>=0&&tx<10&&ty>=0&&ty<15&&!vis[tx][ty]&&board[tx][ty]==cl) 20 { 21 vis[tx][ty]=true; 22 cnt++; 23 cnt_ball(tx,ty,cl); 24 } 25 } 26 return; 27 } 28 29 void mark(int x,int y,int cl) 30 { 31 int i; 32 marked[x][y]=true; 33 for(i=0;i<4;i++) 34 { 35 int tx=x+step[i][0],ty=y+step[i][1]; 36 if(tx>=0&&tx<10&&ty>=0&&ty<15&&board[tx][ty]==cl&&!marked[tx][ty]) 37 { 38 marked[tx][ty]=true; 39 mark(tx,ty,cl); 40 } 41 } 42 return; 43 } 44 45 void eliminate() 46 { 47 int i,j,k; 48 for(i=0;i<10;i++) 49 { 50 for(j=0;j<15;j++) 51 { 52 if(marked[i][j]) 53 { 54 board[i][j]=0; 55 } 56 } 57 } 58 for(j=0;j<15;j++) 59 { 60 for(i=9;i>=0;i--) 61 { 62 if(board[i][j]==0) 63 { 64 int t=0,ti=i; 65 while(ti>=0&&board[ti][j]==0) 66 { 67 t++; 68 ti--; 69 } 70 if(ti==-1) 71 break; 72 for(k=i;k>=t;k--) 73 { 74 board[k][j]=board[k-t][j]; 75 } 76 for(k=0;k<t;k++) 77 { 78 board[k][j]=0; 79 } 80 } 81 } 82 } 83 // for(i=0;i<10;i++) 84 // { 85 // for(j=0;j<15;j++) 86 // cout<<board[i][j]; 87 // cout<<endl; 88 // } 89 // cout<<endl; 90 for(j=0;j<15;j++) 91 { 92 if(board[9][j]==0) 93 { 94 int t=0,tj=j; 95 while(tj<15&&board[9][tj]==0) 96 { 97 t++; 98 tj++; 99 } 100 if(tj==15) 101 break; 102 for(k=j;k<15-t;k++) 103 { 104 for(i=0;i<10;i++) 105 { 106 board[i][k]=board[i][k+t]; 107 } 108 } 109 for(k=14;k>14-t;k--) 110 { 111 for(i=0;i<10;i++) 112 { 113 board[i][k]=0; 114 } 115 } 116 } 117 } 118 // for(i=0;i<10;i++) 119 // { 120 // for(j=0;j<15;j++) 121 // cout<<board[i][j]; 122 // cout<<endl; 123 // } 124 } 125 126 int main() 127 { 128 // freopen("in.txt","r",stdin); 129 int T,cas=0; 130 scanf("%d",&T); 131 while(T--) 132 { 133 cas++; 134 int i,j; 135 memset(board,0,sizeof(board)); 136 char tmp[16]; 137 for(i=0;i<10;i++) 138 { 139 scanf("%s",tmp); 140 for(j=0;j<15;j++) 141 { 142 if(tmp[j]=='R') 143 board[i][j]=1; 144 else if(tmp[j]=='G') 145 board[i][j]=2; 146 else if(tmp[j]=='B') 147 board[i][j]=3; 148 } 149 } 150 printf("Game %d: ",cas); 151 int mov=0,tot=0; 152 while(true) 153 { 154 int process[2],num=0,color=0; 155 memset(vis,false,sizeof(vis)); 156 for(j=0;j<15;j++) 157 { 158 for(i=9;i>=0;i--) 159 { 160 if(board[i][j]!=0&&!vis[i][j]) 161 { 162 cnt=1; 163 cnt_ball(i,j,board[i][j]); 164 if(cnt>num) 165 { 166 memset(marked,false,sizeof(marked)); 167 mark(i,j,board[i][j]); 168 process[0]=10-i; 169 process[1]=j+1; 170 num=cnt; 171 color=board[i][j]; 172 } 173 174 } 175 } 176 } 177 if(num<=1) 178 break; 179 else 180 { 181 int score=(num-2)*(num-2); 182 tot+=score; 183 mov++; 184 eliminate(); 185 char c; 186 if(color==1) 187 c='R'; 188 else if(color==2) 189 c='G'; 190 else if(color==3) 191 c='B'; 192 printf("Move %d at (%d,%d): removed %d balls of color %c, got %d points. ",mov,process[0],process[1],num,c,score); 193 } 194 } 195 int rem=0; 196 for(i=9;i>=0;i--) 197 { 198 for(j=0;j<15;j++) 199 { 200 if(board[i][j]) 201 rem++; 202 } 203 } 204 if(rem==0) 205 tot+=1000; 206 printf("Final score: %d, with %d balls remaining. ",tot,rem); 207 } 208 return 0; 209 }
附上大神的测试数据:
20 BBRRBRGGRBBBRGR BRBGGGGBGRRGBBB BGBGGRBRRGBGBGG BGRBRBBRGGRBGRR RBBGGRRBRRBGBGR BGGGBGBGRGBRBBR GGBRBRBGGBGBRGG GGBBGBBBRGGBGGG RGBGBBGRBRBRGRR RGBRGRBGGBBRBBG Game 1: Move 1 at (3,1): removed 11 balls of color G, got 81 points. Move 2 at (5,1): removed 15 balls of color B, got 169 points. Move 3 at (2,5): removed 7 balls of color B, got 25 points. Move 4 at (2,13): removed 6 balls of color G, got 16 points. Move 5 at (1,12): removed 9 balls of color R, got 49 points. Move 6 at (1,10): removed 10 balls of color B, got 64 points. Move 7 at (3,10): removed 8 balls of color B, got 36 points. Move 8 at (2,10): removed 7 balls of color G, got 25 points. Move 9 at (1,13): removed 6 balls of color G, got 16 points. Move 10 at (1,10): removed 6 balls of color R, got 16 points. Move 11 at (4,4): removed 4 balls of color G, got 4 points. Move 12 at (4,3): removed 4 balls of color R, got 4 points. Move 13 at (5,6): removed 4 balls of color B, got 4 points. Move 14 at (4,7): removed 5 balls of color G, got 9 points. Move 15 at (3,7): removed 8 balls of color R, got 36 points. Move 16 at (1,8): removed 4 balls of color G, got 4 points. Move 17 at (1,7): removed 6 balls of color B, got 16 points. Move 18 at (2,4): removed 3 balls of color G, got 1 points. Move 19 at (1,7): removed 3 balls of color G, got 1 points. Move 20 at (1,6): removed 4 balls of color R, got 4 points. Move 21 at (2,6): removed 3 balls of color R, got 1 points. Move 22 at (1,6): removed 3 balls of color G, got 1 points. Move 23 at (1,1): removed 2 balls of color R, got 0 points. Move 24 at (1,2): removed 2 balls of color G, got 0 points. Move 25 at (1,2): removed 3 balls of color R, got 1 points. Move 26 at (1,1): removed 4 balls of color B, got 4 points. Move 27 at (1,1): removed 2 balls of color R, got 0 points. Final score: 587, with 1 balls remaining. BRBRGBGBGBRBGBB GRGBBRBBRGGGGGR RGBRGBGBRBBRGRB GGBRRBBBGBBRBRG BRGBRBGRGGRGRBB GGGGBBBBBRRGGRR BBRBBBGRRGBGBGG BRBBBRGGGBGBGRB BGRBBBBBGRBGGGG RRRBRRRGGRBBGBB Game 2: Move 1 at (3,3): removed 26 balls of color B, got 576 points. Move 2 at (1,1): removed 15 balls of color R, got 169 points. Move 3 at (1,7): removed 10 balls of color G, got 64 points. Move 4 at (9,9): removed 7 balls of color G, got 25 points. Move 5 at (2,11): removed 6 balls of color G, got 16 points. Move 6 at (1,10): removed 8 balls of color B, got 36 points. Move 7 at (1,2): removed 5 balls of color G, got 9 points. Move 8 at (1,1): removed 10 balls of color B, got 64 points. Move 9 at (1,6): removed 5 balls of color R, got 9 points. Move 10 at (3,6): removed 7 balls of color R, got 25 points. Move 11 at (4,7): removed 7 balls of color B, got 25 points. Move 12 at (1,5): removed 6 balls of color G, got 16 points. Move 13 at (1,6): removed 5 balls of color G, got 9 points. Move 14 at (1,1): removed 3 balls of color G, got 1 points. Move 15 at (2,1): removed 3 balls of color G, got 1 points. Move 16 at (2,1): removed 4 balls of color R, got 4 points. Move 17 at (1,5): removed 3 balls of color R, got 1 points. Move 18 at (1,1): removed 2 balls of color B, got 0 points. Move 19 at (1,3): removed 2 balls of color B, got 0 points. Move 20 at (1,3): removed 2 balls of color G, got 0 points. Move 21 at (1,2): removed 3 balls of color R, got 1 points. Move 22 at (1,2): removed 2 balls of color B, got 0 points. Move 23 at (1,2): removed 2 balls of color R, got 0 points. Final score: 1051, with 7 balls remaining. BGRGBRBRRBRGRGB GRRRRRRGRRBGRBR BRBGGRBRBBBRGRG BBBBGRRGRRRGBRR GBRBBBGGGBGBBGR GRBBBRBGBBRBRBG GBGBRBBRGBRBBRB BGBBRBRBGRRRBBG RGGBGBBRBBBRBBG BBRRBRBBBRRBBRG Game 3: Move 1 at (7,1): removed 17 balls of color B, got 225 points. Move 2 at (2,6): removed 12 balls of color B, got 100 points. Move 3 at (3,4): removed 14 balls of color R, got 144 points. Move 4 at (1,11): removed 12 balls of color B, got 100 points. Move 5 at (1,9): removed 14 balls of color R, got 144 points. Move 6 at (2,2): removed 9 balls of color G, got 49 points. Move 7 at (3,2): removed 8 balls of color R, got 36 points. Move 8 at (2,5): removed 6 balls of color G, got 16 points. Move 9 at (1,5): removed 8 balls of color B, got 36 points. Move 10 at (1,5): removed 9 balls of color R, got 49 points. Move 11 at (1,7): removed 5 balls of color G, got 9 points. Move 12 at (1,1): removed 4 balls of color B, got 4 points. Move 13 at (3,1): removed 4 balls of color G, got 4 points. Move 14 at (2,7): removed 4 balls of color G, got 4 points. Move 15 at (1,6): removed 4 balls of color B, got 4 points. Move 16 at (1,6): removed 4 balls of color R, got 4 points. Move 17 at (2,3): removed 3 balls of color B, got 1 points. Move 18 at (2,6): removed 3 balls of color G, got 1 points. Move 19 at (2,1): removed 2 balls of color B, got 0 points. Move 20 at (1,4): removed 2 balls of color G, got 0 points. Final score: 930, with 6 balls remaining. BRBRBRRBRGRBRGB RRGRGRBRBGBRGGG RRGRGBGRRBRBBRG BBRRBRRRRBRRGGB GRGBBRBRBBGRRRR BBGGRBRRBGBBRRB GGGGRGRGRRGRRRG BGGGRGGRGBBRBGG BBGGRBRRGBBGBRG RRBBBGBBRBGRBGB Game 4: Move 1 at (7,11): removed 13 balls of color R, got 121 points. Move 2 at (4,1): removed 12 balls of color G, got 100 points. Move 3 at (6,6): removed 12 balls of color R, got 100 points. Move 4 at (1,10): removed 10 balls of color B, got 64 points. Move 5 at (5,8): removed 9 balls of color B, got 49 points. Move 6 at (2,9): removed 9 balls of color G, got 49 points. Move 7 at (2,7): removed 10 balls of color R, got 64 points. Move 8 at (3,4): removed 8 balls of color R, got 36 points. Move 9 at (1,3): removed 7 balls of color B, got 25 points. Move 10 at (2,3): removed 9 balls of color G, got 49 points. Move 11 at (1,4): removed 7 balls of color B, got 25 points. Move 12 at (2,1): removed 6 balls of color B, got 16 points. Move 13 at (1,1): removed 6 balls of color R, got 16 points. Move 14 at (3,1): removed 6 balls of color R, got 16 points. Move 15 at (3,4): removed 6 balls of color G, got 16 points. Move 16 at (4,5): removed 4 balls of color G, got 4 points. Move 17 at (1,6): removed 4 balls of color B, got 4 points. Move 18 at (1,3): removed 3 balls of color G, got 1 points. Move 19 at (1,2): removed 3 balls of color B, got 1 points. Move 20 at (1,2): removed 3 balls of color R, got 1 points. Move 21 at (2,1): removed 2 balls of color B, got 0 points. Final score: 757, with 1 balls remaining. GRRGGBBBGBRRGRR GRBBBGRGRBGBGRG RGBBBRGBGBGRBRB BBRGRBBBBGRBRGG RRGGGRGBBBBGRRG RGRGGBGRRBGBRGB GBRRBRGGRGGRRRR GGRBGGBBRGBRRGG BGBGRBGRGBRGGRB GGRRBBBBBBGBRBB Game 5: Move 1 at (7,6): removed 10 balls of color B, got 64 points. Move 2 at (3,12): removed 10 balls of color R, got 64 points. Move 3 at (3,10): removed 14 balls of color G, got 144 points. Move 4 at (1,5): removed 15 balls of color B, got 169 points. Move 5 at (6,6): removed 10 balls of color G, got 64 points. Move 6 at (3,7): removed 10 balls of color R, got 64 points. Move 7 at (3,4): removed 7 balls of color B, got 25 points. Move 8 at (6,3): removed 8 balls of color G, got 36 points. Move 9 at (1,3): removed 8 balls of color R, got 36 points. Move 10 at (1,1): removed 6 balls of color G, got 16 points. Move 11 at (1,1): removed 8 balls of color B, got 36 points. Move 12 at (1,1): removed 7 balls of color R, got 25 points. Move 13 at (1,13): removed 5 balls of color B, got 9 points. Move 14 at (1,12): removed 6 balls of color R, got 16 points. Move 15 at (1,4): removed 4 balls of color G, got 4 points. Move 16 at (3,1): removed 3 balls of color G, got 1 points. Move 17 at (2,2): removed 3 balls of color B, got 1 points. Move 18 at (2,1): removed 3 balls of color R, got 1 points. Move 19 at (1,5): removed 3 balls of color G, got 1 points. Move 20 at (1,3): removed 2 balls of color R, got 0 points. Move 21 at (1,4): removed 2 balls of color G, got 0 points. Final score: 776, with 6 balls remaining. BBGGRBRGRGGRBRR GGGBBBBBGRGGGGR BGGRRBBBBGBBRGB GRGRRGBBGBGRGRR GBRRGGBBRRGBRRB BGBBGBBGRBBRGRG RRRGBRGBRGGRBRR BGGBGGRGRRGRRGG BGRBGGRBBGRGBRR RRBGRBBBGBRGBRG Game 6: Move 1 at (9,4): removed 16 balls of color B, got 196 points. Move 2 at (9,1): removed 8 balls of color G, got 36 points. Move 3 at (6,3): removed 7 balls of color R, got 25 points. Move 4 at (10,10): removed 7 balls of color G, got 25 points. Move 5 at (6,13): removed 9 balls of color R, got 49 points. Move 6 at (3,9): removed 6 balls of color R, got 16 points. Move 7 at (3,8): removed 7 balls of color G, got 25 points. Move 8 at (1,6): removed 10 balls of color B, got 64 points. Move 9 at (2,5): removed 4 balls of color G, got 4 points. Move 10 at (4,4): removed 6 balls of color G, got 16 points. Move 11 at (1,5): removed 5 balls of color R, got 9 points. Move 12 at (1,6): removed 4 balls of color G, got 4 points. Move 13 at (1,6): removed 4 balls of color R, got 4 points. Move 14 at (1,6): removed 4 balls of color G, got 4 points. Move 15 at (1,7): removed 4 balls of color R, got 4 points. Move 16 at (1,5): removed 7 balls of color B, got 25 points. Move 17 at (4,1): removed 3 balls of color R, got 1 points. Move 18 at (2,2): removed 4 balls of color G, got 4 points. Move 19 at (2,1): removed 5 balls of color B, got 9 points. Move 20 at (1,1): removed 4 balls of color R, got 4 points. Move 21 at (1,2): removed 5 balls of color B, got 9 points. Move 22 at (1,1): removed 3 balls of color G, got 1 points. Move 23 at (1,4): removed 3 balls of color R, got 1 points. Move 24 at (1,3): removed 6 balls of color G, got 16 points. Move 25 at (1,3): removed 3 balls of color B, got 1 points. Move 26 at (1,2): removed 4 balls of color R, got 4 points. Move 27 at (1,1): removed 2 balls of color B, got 0 points. Final score: 1556, with 0 balls remaining. RGRGBGBBGRBGGBR GGRBBGBBGBRBBGG RRBRGGRGRGGBGGG GGGBBBRBRRGRRRR RBRBGBRGRGRGBRR GRBRGRRBGBRBGBB RBGGGRGBGGGRGRG BGGRGRBGBBRGRGR BGRBGRGRRGGRGRB RGRRBGRRGBBBRRR Game 7: Move 1 at (1,2): removed 11 balls of color G, got 81 points. Move 2 at (2,6): removed 8 balls of color R, got 36 points. Move 3 at (2,4): removed 6 balls of color B, got 16 points. Move 4 at (1,5): removed 8 balls of color G, got 36 points. Move 5 at (1,5): removed 8 balls of color B, got 36 points. Move 6 at (2,2): removed 10 balls of color R, got 64 points. Move 7 at (2,1): removed 8 balls of color B, got 36 points. Move 8 at (1,5): removed 8 balls of color G, got 36 points. Move 9 at (7,9): removed 6 balls of color R, got 16 points. Move 10 at (7,10): removed 5 balls of color G, got 9 points. Move 11 at (7,9): removed 7 balls of color B, got 25 points. Move 12 at (6,8): removed 7 balls of color G, got 25 points. Move 13 at (4,7): removed 5 balls of color R, got 9 points. Move 14 at (4,6): removed 6 balls of color G, got 16 points. Move 15 at (1,7): removed 7 balls of color B, got 25 points. Move 16 at (1,6): removed 10 balls of color R, got 64 points. Move 17 at (1,1): removed 3 balls of color R, got 1 points. Move 18 at (1,1): removed 6 balls of color G, got 16 points. Move 19 at (1,1): removed 4 balls of color R, got 4 points. Move 20 at (1,2): removed 4 balls of color B, got 4 points. Move 21 at (2,1): removed 3 balls of color R, got 1 points. Move 22 at (1,1): removed 4 balls of color G, got 4 points. Move 23 at (1,1): removed 2 balls of color R, got 0 points. Final score: 560, with 4 balls remaining. BBGBRGBBBRBBGGR BGGRBRRRBBRGBBR GRBGGBGBGGRGRGR GBGRRBRRGBBGBRR RBRGRBBGBBGRBGB GRGGBRRGBRGBBGG RBGGGGGBBBGRRGG RGGRBRGBBBRBRGR GBBRBRGGRGGGBGR RGGRGRRRRRBGRGG Game 8: Move 1 at (3,2): removed 13 balls of color G, got 121 points. Move 2 at (3,7): removed 12 balls of color B, got 100 points. Move 3 at (1,6): removed 11 balls of color R, got 81 points. Move 4 at (1,14): removed 9 balls of color G, got 49 points. Move 5 at (2,5): removed 7 balls of color B, got 25 points. Move 6 at (3,3): removed 7 balls of color R, got 25 points. Move 7 at (1,2): removed 6 balls of color G, got 16 points. Move 8 at (1,7): removed 6 balls of color G, got 16 points. Move 9 at (2,10): removed 4 balls of color G, got 4 points. Move 10 at (3,11): removed 6 balls of color G, got 16 points. Move 11 at (3,10): removed 8 balls of color R, got 36 points. Move 12 at (2,8): removed 15 balls of color B, got 169 points. Move 13 at (1,6): removed 14 balls of color R, got 144 points. Move 14 at (3,1): removed 3 balls of color R, got 1 points. Move 15 at (1,2): removed 6 balls of color B, got 16 points. Move 16 at (2,1): removed 6 balls of color G, got 16 points. Move 17 at (1,1): removed 4 balls of color R, got 4 points. Move 18 at (1,2): removed 5 balls of color B, got 9 points. Move 19 at (1,3): removed 3 balls of color G, got 1 points. Move 20 at (1,1): removed 2 balls of color G, got 0 points. Move 21 at (1,1): removed 2 balls of color B, got 0 points. Final score: 849, with 1 balls remaining. GGGGRGBRRGGRRGG RRBGBGRRBRRBGGR RBRGGGGGBRGRBGG BGGGBGGBRBBBRBR GRGRRBRBGBRBGGB BGGRRRRRGBGRGGG GRBGGRGRRBBRBBR BBRRGRGRRRGGBRG BBBGGBRBRBBGRBB BGGGRGGBRRBGBGB Game 9: Move 1 at (10,1): removed 20 balls of color G, got 324 points. Move 2 at (4,2): removed 22 balls of color R, got 400 points. Move 3 at (1,8): removed 14 balls of color B, got 144 points. Move 4 at (1,1): removed 9 balls of color B, got 49 points. Move 5 at (1,1): removed 9 balls of color G, got 49 points. Move 6 at (9,13): removed 6 balls of color G, got 16 points. Move 7 at (1,11): removed 5 balls of color G, got 9 points. Move 8 at (1,10): removed 6 balls of color R, got 16 points. Move 9 at (1,11): removed 9 balls of color B, got 49 points. Move 10 at (1,2): removed 4 balls of color R, got 4 points. Move 11 at (1,1): removed 5 balls of color B, got 9 points. Move 12 at (1,6): removed 4 balls of color G, got 4 points. Move 13 at (2,4): removed 7 balls of color R, got 25 points. Move 14 at (1,3): removed 5 balls of color G, got 9 points. Move 15 at (1,5): removed 4 balls of color G, got 4 points. Move 16 at (1,5): removed 3 balls of color R, got 1 points. Move 17 at (1,3): removed 4 balls of color B, got 4 points. Move 18 at (1,2): removed 3 balls of color R, got 1 points. Move 19 at (1,1): removed 4 balls of color G, got 4 points. Move 20 at (1,1): removed 3 balls of color B, got 1 points. Move 21 at (1,1): removed 4 balls of color R, got 4 points. Final score: 2126, with 0 balls remaining. GGGRBBBBRBRRGGB GBGGBBRGGBRGRGG BBBRRRBBBGGBRBG BRBRRRRBRBBGBBB BGRBRBGBBRRGGBG GRGGRBBGGBBBGRG RBBRBGRRRGGGRGR RRRGGRRBGBRRGGB BRBGGBGBGBGGGBR BGBRGGBGBBGRBBG Game 10: Move 1 at (7,4): removed 9 balls of color R, got 49 points. Move 2 at (6,4): removed 15 balls of color B, got 169 points. Move 3 at (6,1): removed 7 balls of color B, got 25 points. Move 4 at (1,11): removed 7 balls of color G, got 25 points. Move 5 at (2,4): removed 6 balls of color G, got 16 points. Move 6 at (8,11): removed 6 balls of color G, got 16 points. Move 7 at (3,1): removed 5 balls of color R, got 9 points. Move 8 at (3,1): removed 8 balls of color G, got 36 points. Move 9 at (1,1): removed 6 balls of color B, got 16 points. Move 10 at (1,2): removed 5 balls of color R, got 9 points. Move 11 at (1,6): removed 5 balls of color B, got 9 points. Move 12 at (5,4): removed 4 balls of color G, got 4 points. Move 13 at (3,4): removed 4 balls of color R, got 4 points. Move 14 at (3,4): removed 4 balls of color B, got 4 points. Move 15 at (1,5): removed 4 balls of color G, got 4 points. Move 16 at (1,7): removed 4 balls of color R, got 4 points. Move 17 at (4,6): removed 6 balls of color G, got 16 points. Move 18 at (3,6): removed 10 balls of color B, got 64 points. Move 19 at (2,6): removed 8 balls of color R, got 36 points. Move 20 at (1,9): removed 4 balls of color B, got 4 points. Move 21 at (1,1): removed 3 balls of color G, got 1 points. Move 22 at (1,6): removed 3 balls of color G, got 1 points. Move 23 at (1,1): removed 2 balls of color R, got 0 points. Move 24 at (1,2): removed 2 balls of color B, got 0 points. Move 25 at (1,1): removed 2 balls of color G, got 0 points. Move 26 at (3,1): removed 2 balls of color R, got 0 points. Move 27 at (1,3): removed 2 balls of color R, got 0 points. Move 28 at (1,3): removed 2 balls of color G, got 0 points. Move 29 at (1,2): removed 3 balls of color B, got 1 points. Final score: 522, with 2 balls remaining. GGGBRGGRRBBRGGG BBRRRBGRGRRGGBR GRGBRBRGGBGRBRG RGRGGRBRBRGRGGR BGBGBGRGGBRRRGR BGRGGBRRBRBBGGB BBBGBGGRGGGBBRG BGBBBRGRBRRRRRG GBGRRRRRRRGBGBB GRGGBRBRGGBRBRB Game 11: Move 1 at (2,4): removed 21 balls of color R, got 361 points. Move 2 at (3,1): removed 7 balls of color B, got 25 points. Move 3 at (4,1): removed 13 balls of color G, got 121 points. Move 4 at (2,4): removed 7 balls of color B, got 25 points. Move 5 at (4,9): removed 7 balls of color B, got 25 points. Move 6 at (5,11): removed 11 balls of color G, got 81 points. Move 7 at (3,10): removed 10 balls of color R, got 64 points. Move 8 at (1,8): removed 8 balls of color G, got 36 points. Move 9 at (1,9): removed 10 balls of color B, got 64 points. Move 10 at (1,6): removed 7 balls of color G, got 25 points. Move 11 at (2,4): removed 5 balls of color R, got 9 points. Move 12 at (2,4): removed 5 balls of color B, got 9 points. Move 13 at (1,9): removed 5 balls of color R, got 9 points. Move 14 at (1,3): removed 4 balls of color G, got 4 points. Move 15 at (1,2): removed 6 balls of color R, got 16 points. Move 16 at (1,4): removed 4 balls of color G, got 4 points. Move 17 at (1,2): removed 5 balls of color B, got 9 points. Move 18 at (1,2): removed 4 balls of color R, got 4 points. Move 19 at (1,1): removed 3 balls of color G, got 1 points. Move 20 at (1,3): removed 3 balls of color G, got 1 points. Final score: 893, with 5 balls remaining. RRBBBRGGGRGBBRR GBRBBRGGRGBRGBB RRRRBBBBBGBBRBB GGGGRGRGGBBBBBB RRRRRRGRGBGGRRR BBRBRGGGBBRBGRR BBGRRGRRGRBRBRB BRRGRGRBRRRGGBR RGGBBBBRBBGRRGR GGGGRBBRGGGRBRR Game 12: Move 1 at (5,9): removed 16 balls of color B, got 196 points. Move 2 at (3,6): removed 14 balls of color G, got 144 points. Move 3 at (6,1): removed 26 balls of color R, got 576 points. Move 4 at (2,4): removed 16 balls of color B, got 196 points. Move 5 at (6,12): removed 10 balls of color R, got 64 points. Move 6 at (1,1): removed 8 balls of color G, got 36 points. Move 7 at (1,1): removed 5 balls of color R, got 9 points. Move 8 at (1,1): removed 5 balls of color B, got 9 points. Move 9 at (1,8): removed 5 balls of color G, got 9 points. Move 10 at (1,1): removed 4 balls of color G, got 4 points. Move 11 at (1,1): removed 4 balls of color R, got 4 points. Move 12 at (1,2): removed 4 balls of color B, got 4 points. Move 13 at (1,9): removed 4 balls of color R, got 4 points. Move 14 at (1,3): removed 3 balls of color G, got 1 points. Move 15 at (1,2): removed 3 balls of color R, got 1 points. Move 16 at (1,4): removed 3 balls of color R, got 1 points. Move 17 at (3,4): removed 3 balls of color B, got 1 points. Move 18 at (2,5): removed 3 balls of color G, got 1 points. Move 19 at (1,5): removed 3 balls of color B, got 1 points. Move 20 at (1,1): removed 2 balls of color G, got 0 points. Move 21 at (1,2): removed 2 balls of color B, got 0 points. Move 22 at (1,2): removed 4 balls of color G, got 4 points. Move 23 at (1,1): removed 2 balls of color R, got 0 points. Final score: 1265, with 1 balls remaining. GGBRGBGGGGRBGRR GRGRBRRGRBGRGGG BRGRBGRGGGRRRBB GGBBGGRRBGBBBBG RBGBBBRBRBRRRRR RRGBRGGBBBBBGRG BGRRBBRRBBRBRBG RGRGRBRGRRBGGBB BBRRBGBGBBBBGBR GBGGRBBBBGRBGBG Game 13: Move 1 at (1,6): removed 11 balls of color B, got 81 points. Move 2 at (4,8): removed 13 balls of color B, got 121 points. Move 3 at (1,9): removed 11 balls of color R, got 81 points. Move 4 at (1,8): removed 13 balls of color G, got 121 points. Move 5 at (2,13): removed 6 balls of color B, got 16 points. Move 6 at (2,3): removed 5 balls of color R, got 9 points. Move 7 at (3,2): removed 7 balls of color G, got 25 points. Move 8 at (2,1): removed 10 balls of color B, got 64 points. Move 9 at (1,4): removed 5 balls of color R, got 9 points. Move 10 at (6,5): removed 5 balls of color R, got 9 points. Move 11 at (4,4): removed 8 balls of color G, got 36 points. Move 12 at (3,3): removed 6 balls of color B, got 16 points. Move 13 at (1,6): removed 5 balls of color R, got 9 points. Move 14 at (1,9): removed 5 balls of color R, got 9 points. Move 15 at (4,1): removed 4 balls of color R, got 4 points. Move 16 at (3,10): removed 4 balls of color G, got 4 points. Move 17 at (4,1): removed 3 balls of color G, got 1 points. Move 18 at (1,5): removed 3 balls of color G, got 1 points. Move 19 at (3,1): removed 2 balls of color B, got 0 points. Move 20 at (3,1): removed 2 balls of color G, got 0 points. Move 21 at (1,3): removed 2 balls of color G, got 0 points. Move 22 at (1,3): removed 2 balls of color B, got 0 points. Move 23 at (1,2): removed 3 balls of color R, got 1 points. Move 24 at (1,5): removed 2 balls of color G, got 0 points. Move 25 at (1,4): removed 3 balls of color B, got 1 points. Move 26 at (1,4): removed 2 balls of color R, got 0 points. Move 27 at (1,3): removed 2 balls of color G, got 0 points. Move 28 at (1,2): removed 2 balls of color B, got 0 points. Move 29 at (1,1): removed 2 balls of color G, got 0 points. Move 30 at (1,1): removed 2 balls of color R, got 0 points. Final score: 1618, with 0 balls remaining. GGBBGRBGBBRBBBR RGGRRRBRBBBBBRR RBGRBRGGRGRRGGB GGBGRGGGRBGGGRG RGRBRRBGBBGGRGR GBBBGRGRGRGRGGG GGBGGGGRBRGGGBG RGBBRBRRGGGGRRR GGGBRGRGRGRBGRG GBGGRBBRBBRRBBB Game 14: Move 1 at (3,9): removed 21 balls of color G, got 361 points. Move 2 at (2,9): removed 15 balls of color R, got 169 points. Move 3 at (1,9): removed 15 balls of color B, got 169 points. Move 4 at (1,1): removed 10 balls of color G, got 64 points. Move 5 at (1,2): removed 7 balls of color B, got 25 points. Move 6 at (4,2): removed 10 balls of color G, got 64 points. Move 7 at (4,4): removed 9 balls of color R, got 49 points. Move 8 at (2,7): removed 7 balls of color R, got 25 points. Move 9 at (4,6): removed 7 balls of color G, got 25 points. Move 10 at (1,5): removed 7 balls of color B, got 25 points. Move 11 at (3,2): removed 6 balls of color B, got 16 points. Move 12 at (2,6): removed 5 balls of color R, got 9 points. Move 13 at (2,8): removed 5 balls of color B, got 9 points. Move 14 at (1,6): removed 4 balls of color G, got 4 points. Move 15 at (1,1): removed 2 balls of color R, got 0 points. Move 16 at (1,1): removed 3 balls of color G, got 1 points. Move 17 at (1,1): removed 3 balls of color R, got 1 points. Move 18 at (1,1): removed 2 balls of color G, got 0 points. Move 19 at (1,1): removed 2 balls of color R, got 0 points. Move 20 at (1,1): removed 2 balls of color G, got 0 points. Move 21 at (1,1): removed 2 balls of color R, got 0 points. Move 22 at (1,2): removed 2 balls of color G, got 0 points. Move 23 at (1,1): removed 2 balls of color B, got 0 points. Move 24 at (1,1): removed 2 balls of color R, got 0 points. Final score: 2016, with 0 balls remaining. GRBGBBRGBRBGGBG BBGGGBBBRGRRGGR BGRRRRBGBGRGBBB RBGRBGBGBRBRGRR RRGGGGGGRBBBBGB RBRBRRRGRRGRRBG RBBGRGBGGRRBBBR RGRGGBBBGGRBGRG GRGBGBRBGBRGRRR BBRGBGGGBGGGGRB Game 15: Move 1 at (6,3): removed 16 balls of color G, got 196 points. Move 2 at (3,1): removed 11 balls of color R, got 81 points. Move 3 at (2,6): removed 10 balls of color B, got 64 points. Move 4 at (3,4): removed 9 balls of color G, got 49 points. Move 5 at (2,5): removed 15 balls of color R, got 169 points. Move 6 at (2,4): removed 12 balls of color B, got 100 points. Move 7 at (1,7): removed 8 balls of color G, got 36 points. Move 8 at (3,1): removed 7 balls of color B, got 25 points. Move 9 at (2,1): removed 5 balls of color G, got 9 points. Move 10 at (2,3): removed 5 balls of color G, got 9 points. Move 11 at (1,9): removed 5 balls of color R, got 9 points. Move 12 at (1,7): removed 8 balls of color B, got 36 points. Move 13 at (1,5): removed 5 balls of color R, got 9 points. Move 14 at (2,6): removed 4 balls of color R, got 4 points. Move 15 at (1,7): removed 4 balls of color G, got 4 points. Move 16 at (1,4): removed 6 balls of color B, got 16 points. Move 17 at (2,2): removed 4 balls of color R, got 4 points. Move 18 at (1,3): removed 4 balls of color G, got 4 points. Move 19 at (1,1): removed 5 balls of color B, got 9 points. Move 20 at (1,2): removed 2 balls of color G, got 0 points. Move 21 at (1,1): removed 2 balls of color R, got 0 points. Final score: 833, with 3 balls remaining. GRRRGRGBBGBBRGB RGBRRRBRBGBBBGR RGRBGBBGRBBGBRB RGGGGBBRGBGBBRB RBGBRBGBBRBGBRG RGBRBRGBRGBGGGG RGRRRGGGRRRGBGB GRRRBRBGGBRBBBR RGRGRGRBGRGGGRB BBRRRRGGGRRBBBB Game 16: Move 1 at (3,2): removed 13 balls of color R, got 121 points. Move 2 at (7,10): removed 12 balls of color B, got 100 points. Move 3 at (7,9): removed 13 balls of color G, got 121 points. Move 4 at (1,6): removed 11 balls of color G, got 81 points. Move 5 at (4,6): removed 12 balls of color B, got 100 points. Move 6 at (1,6): removed 13 balls of color R, got 121 points. Move 7 at (3,1): removed 7 balls of color G, got 25 points. Move 8 at (2,1): removed 8 balls of color R, got 36 points. Move 9 at (2,11): removed 6 balls of color B, got 16 points. Move 10 at (3,13): removed 6 balls of color R, got 16 points. Move 11 at (1,12): removed 8 balls of color B, got 36 points. Move 12 at (1,10): removed 6 balls of color G, got 16 points. Move 13 at (3,3): removed 5 balls of color B, got 9 points. Move 14 at (1,1): removed 4 balls of color B, got 4 points. Move 15 at (1,1): removed 4 balls of color G, got 4 points. Move 16 at (1,1): removed 4 balls of color R, got 4 points. Move 17 at (4,2): removed 4 balls of color R, got 4 points. Move 18 at (2,2): removed 3 balls of color G, got 1 points. Move 19 at (1,3): removed 3 balls of color G, got 1 points. Move 20 at (1,3): removed 3 balls of color B, got 1 points. Move 21 at (1,2): removed 3 balls of color R, got 1 points. Final score: 818, with 2 balls remaining. RRGGGBBGBGBGBRG RBRGRRGRBGGBGBG GBBBBRBBGRRRGBB GBBBGGRRGRGRBGR BBBGGBRGRRBRGRB GRRBRGGBGBRBRBB GRGGGGGGGRBRGRR BBBGBGBRRGRBRGR RGRBRRGBGRRGGBG BRRBRRRRGRGRGGB Game 17: Move 1 at (4,3): removed 12 balls of color G, got 100 points. Move 2 at (6,1): removed 10 balls of color B, got 64 points. Move 3 at (3,1): removed 9 balls of color B, got 49 points. Move 4 at (1,2): removed 8 balls of color R, got 36 points. Move 5 at (4,6): removed 8 balls of color R, got 36 points. Move 6 at (1,5): removed 7 balls of color R, got 25 points. Move 7 at (1,2): removed 9 balls of color G, got 49 points. Move 8 at (1,1): removed 6 balls of color B, got 16 points. Move 9 at (1,3): removed 7 balls of color G, got 25 points. Move 10 at (2,1): removed 5 balls of color G, got 9 points. Move 11 at (1,1): removed 4 balls of color R, got 4 points. Move 12 at (1,4): removed 4 balls of color R, got 4 points. Move 13 at (2,2): removed 4 balls of color B, got 4 points. Move 14 at (1,2): removed 6 balls of color R, got 16 points. Move 15 at (1,2): removed 4 balls of color G, got 4 points. Move 16 at (2,3): removed 4 balls of color G, got 4 points. Move 17 at (3,3): removed 5 balls of color R, got 9 points. Move 18 at (1,1): removed 5 balls of color B, got 9 points. Move 19 at (2,1): removed 6 balls of color R, got 16 points. Move 20 at (1,1): removed 7 balls of color G, got 25 points. Move 21 at (1,1): removed 8 balls of color B, got 36 points. Move 22 at (1,1): removed 3 balls of color G, got 1 points. Move 23 at (2,2): removed 3 balls of color B, got 1 points. Move 24 at (1,2): removed 3 balls of color R, got 1 points. Move 25 at (1,2): removed 2 balls of color G, got 0 points. Final score: 543, with 1 balls remaining. RRRBBGBRRRGGBBG RBRGBRGRRGRBGBG BBRGGBRGGRGGRBG RRRGBRRRBBGRGGR BGRBRRBBRRGBRRB RGGBRRBBBBGRGBR GRRBGRRRRBBRRBB RBRGBGBRGRBGBBG GRGRBBRRGBGRRRB BGGRRGGBGRBRBRG Game 18: Move 1 at (5,5): removed 15 balls of color R, got 169 points. Move 2 at (7,1): removed 10 balls of color R, got 64 points. Move 3 at (2,5): removed 10 balls of color B, got 64 points. Move 4 at (2,5): removed 9 balls of color G, got 49 points. Move 5 at (4,4): removed 5 balls of color B, got 9 points. Move 6 at (5,2): removed 8 balls of color G, got 36 points. Move 7 at (5,11): removed 5 balls of color G, got 9 points. Move 8 at (1,12): removed 5 balls of color R, got 9 points. Move 9 at (1,13): removed 6 balls of color B, got 16 points. Move 10 at (4,10): removed 5 balls of color B, got 9 points. Move 11 at (3,10): removed 7 balls of color R, got 25 points. Move 12 at (2,11): removed 8 balls of color G, got 36 points. Move 13 at (4,13): removed 5 balls of color B, got 9 points. Move 14 at (6,1): removed 4 balls of color B, got 4 points. Move 15 at (1,4): removed 4 balls of color R, got 4 points. Move 16 at (1,2): removed 3 balls of color G, got 1 points. Move 17 at (1,2): removed 3 balls of color R, got 1 points. Move 18 at (1,1): removed 4 balls of color B, got 4 points. Move 19 at (1,5): removed 3 balls of color R, got 1 points. Move 20 at (1,4): removed 5 balls of color B, got 9 points. Move 21 at (2,4): removed 4 balls of color R, got 4 points. Move 22 at (1,3): removed 3 balls of color G, got 1 points. Move 23 at (1,6): removed 3 balls of color R, got 1 points. Move 24 at (4,7): removed 3 balls of color G, got 1 points. Move 25 at (1,2): removed 2 balls of color R, got 0 points. Move 26 at (1,3): removed 2 balls of color G, got 0 points. Move 27 at (1,2): removed 3 balls of color B, got 1 points. Move 28 at (2,1): removed 3 balls of color R, got 1 points. Move 29 at (1,1): removed 2 balls of color G, got 0 points. Final score: 537, with 1 balls remaining. BGRGBGGGGRBRRRG RBBGBRBGBRBGBBR GGBBBBRRGGBRRRR GGBGBGRRRBRGBGR BGRGRGRBBGBGRGG RBRRGRGRRBRGRGR GRBBRBBBRGGRGRR BRGBBBBGGGRBGRG GGRBBGRRRRGBGGG BBGGRBGBGGRRBBR Game 19: Move 1 at (4,3): removed 11 balls of color B, got 81 points. Move 2 at (4,7): removed 9 balls of color R, got 49 points. Move 3 at (5,4): removed 6 balls of color B, got 16 points. Move 4 at (3,3): removed 6 balls of color G, got 16 points. Move 5 at (3,2): removed 10 balls of color R, got 64 points. Move 6 at (2,5): removed 9 balls of color G, got 49 points. Move 7 at (8,11): removed 6 balls of color R, got 16 points. Move 8 at (2,12): removed 6 balls of color G, got 16 points. Move 9 at (2,12): removed 7 balls of color R, got 25 points. Move 10 at (2,11): removed 6 balls of color B, got 16 points. Move 11 at (3,1): removed 5 balls of color B, got 9 points. Move 12 at (2,1): removed 6 balls of color G, got 16 points. Move 13 at (2,5): removed 5 balls of color R, got 9 points. Move 14 at (2,6): removed 5 balls of color B, got 9 points. Move 15 at (2,5): removed 10 balls of color G, got 64 points. Move 16 at (3,7): removed 8 balls of color R, got 36 points. Move 17 at (1,9): removed 9 balls of color G, got 49 points. Move 18 at (1,5): removed 4 balls of color B, got 4 points. Move 19 at (1,3): removed 4 balls of color G, got 4 points. Move 20 at (1,1): removed 3 balls of color B, got 1 points. Move 21 at (1,3): removed 3 balls of color R, got 1 points. Move 22 at (1,3): removed 4 balls of color B, got 4 points. Move 23 at (3,1): removed 2 balls of color G, got 0 points. Final score: 554, with 6 balls remaining. GGBGGBBRBBGBBBR GRBRGGRBGGRGRRG BGBRBRRBBBGBRRR RBBRGBBGGRBBGRG RGBRBGBRGBBRRGB BGRRGRGGGGRGGRR BRGBBBRBRGGGRBB GGBRRBBGGRGRBBG BGGGBRRBBBRBGGG BBBRGBBGBRGGBGB Game 20: Move 1 at (5,7): removed 13 balls of color G, got 121 points. Move 2 at (3,10): removed 9 balls of color R, got 49 points. Move 3 at (2,8): removed 15 balls of color B, got 169 points. Move 4 at (1,11): removed 12 balls of color G, got 100 points. Move 5 at (4,4): removed 8 balls of color B, got 36 points. Move 6 at (5,3): removed 13 balls of color R, got 121 points. Move 7 at (1,6): removed 9 balls of color B, got 49 points. Move 8 at (3,1): removed 8 balls of color G, got 36 points. Move 9 at (1,1): removed 7 balls of color B, got 25 points. Move 10 at (4,2): removed 6 balls of color B, got 16 points. Move 11 at (2,13): removed 6 balls of color R, got 16 points. Move 12 at (4,1): removed 5 balls of color G, got 9 points. Move 13 at (1,12): removed 5 balls of color B, got 9 points. Move 14 at (1,1): removed 4 balls of color R, got 4 points. Move 15 at (2,5): removed 4 balls of color B, got 4 points. Move 16 at (2,9): removed 4 balls of color R, got 4 points. Move 17 at (1,8): removed 7 balls of color G, got 25 points. Move 18 at (2,6): removed 5 balls of color R, got 9 points. Move 19 at (1,5): removed 3 balls of color G, got 1 points. Move 20 at (1,2): removed 2 balls of color G, got 0 points. Move 21 at (2,3): removed 2 balls of color G, got 0 points. Final score: 803, with 3 balls remaining.