in this article i just want to explain how we can create progress bar from scratch , the visual design , how to be invokable ,easy to layout ,important methods and finally it's properties .
physical point: the progress bar as you see in figure 1 is consist of tow parts only , first is the backbone or the container of progress line ,the second is the progress line .physically the backbone is Div tag with background repeated-x ,but progress line is span also with background repeated-x but it's width is variable .
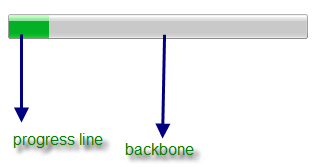
figure1:
here you see four images to accomplish the visual style of progress bar and progress line
all are with one px width , have the same height and border.
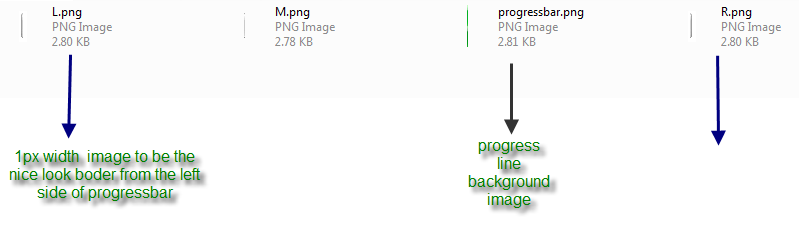
CSS style :
in css style we consider the visual style,but not the layout of progress bar , we will consider that using js latter

/*div , the background image which*/
.progressbar
{
width:300PX;;
height:25px;
position:absolute;
background-image:url('images/M.png');
background-repeat:repeat-x;
}
/*span*/
.progressbar .LeftProgSide
{
width:2px;
height:25px;
margin-left:0px;
background-image:url('images/L.png');
position:absolute;
}
/*span*/
.progressbar .RightProSide
{
width:2px;
height:25px;
float:right;
background-image:url('images/R.png');
}
/* span :line*/
.progressbar .ProgLine
{
width:40px;
height:23px;
margin-left:1px;
margin-top:1px;
position:absolute;
background-image:url('images/progressbar.png');
background-repeat:repeat-x;
}
js code :
with OOP of js we just need to create a class Progressbar. as you know class in js can be created with function keyword ,
in progressbar class we also add some main methods and properties.

function changeState( element ,height ,statNumber)
{
//statNumber the number of state we want to show currently
// elemnt is imageBackground for span
/// 0: normal status,1 mouseMove status,2 mousepress status, 3 fucuse,4 disable ,5 no
var top=height*statNumber;
// alert('100% -'+left+'px');
document.getElementById(element).style.backgroundPosition='100% -'+top+'px';
}
// class. the class pf progress bar
function Progressbar(proLineName,ProgressBar)
{
//proLineName is animation line in the ProgressBar
var visible="";
var maximum_value;// for progresse line, but not for the progressbar
var minimum_value; // for the progress line
var Current_value; // for the porgress line
var width;
var Par=0;
var MarginLeft;
var MarginTop;
// for the progress bar
this.setWidth=function(value)
{
width=value;
maximum_value=value; // the max value for the progress line is the width of progressBar
Par=value/100;
document.getElementById(ProgressBar).style.width=value;
}
this.getMarginLeft=function(){return MarginLeft;}
this.setMarginLeft=function(value)
{
MarginLeft=value;
document.getElementById(ProgressBar).style.marginLeft=value;
}
this.getMarginTop=function(){return MarginTop;}
this.setMarginTop=function(value)
{
MarginTop=value;
document.getElementById(ProgressBar).style.marginTop=value;
}
this.getWidth=function(){alert(width); return width;}
this.getVisible=function() {return visible;}
this.setVisible=function(v)
{
var temp="";
if(v=='false')
{
temp='none'
}
else if( v=='true')
{
temp='block';
}
visible=v;
document.getElementById(proLineName).style.display=temp;
}
this.setValue=function(value)
{
value=value*Par
if(value<width)
{
Current_value=value;
document.getElementById(proLineName).style.width=value;
}
else if(value==maximum_value)
{
Current_value=value;
document.getElementById(proLineName).style.width=value-2;
}
else if(value>width)
{
alert( 'overflow value..');
}
}
this.getValue=function()
{
return Current_value;
}
this.getMaxValue=function(){return maximum_value;}
}
create an object of progress bar
var progLine=new Progressbar('progline','progressbar');
progLine.setVisible('true');
progLine.setWidth(100);
progLine.setMarginLeft(10);
progLine.setMarginTop(10);
progLine.setVisible('true');
progLine.setWidth(100);
progLine.setMarginLeft(10);
progLine.setMarginTop(10);
html code :

<div id="progressbar" class="progressbar">
<span class="LeftProgSide"></span>
<span class="RightProSide"></span>
<span id="progline" class="ProgLine" onmouseover="changeState('progline',23,1);" onmouseout="changeState('progline',23,0);"></span>
</div>

<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta content="text/html; charset=utf-8" http-equiv="Content-Type" />
<link rel="stylesheet" type="text/css" href="progressebar.css"/>
<script language="javascript" type="text/javascript" src="progressebar.js"></script>
<title>Untitled 1</title>
</head>
<body>
<div id="progressbar" class="progressbar">
<span class="LeftProgSide"></span>
<span class="RightProSide"></span>
<span id="progline" class="ProgLine" onmouseover="changeState('progline',23,1);" onmouseout="changeState('progline',23,0);"></span>
</div>
<script language="javascript" type="text/javascript">
var progLine=new Progressbar('progline','progressbar');
progLine.setVisible('true');
progLine.setWidth(100);
progLine.setMarginLeft(10);
progLine.setMarginTop(10);
</script>
<a style="top:100px;position: fixed;" href="#" onclick="progLine.setValue(0);">0</a>
<a style="top:150px;position: fixed;" href="#" onclick="progLine.setValue(30);">30</a>
<a style="top:180px;position: fixed;" href="#" onclick="progLine.setValue(50);">50</a>
<a style="top:200px;position: fixed;" href="#" onclick="progLine.setValue(100);">100</a>
</body>
</html>