main线程跑三个任务:
package android.java.thread2; class Demo { private String name; public Demo(String name) { this.name = name; } public void showMethod() { for (int i = 0; i < 10; i++) { System.out.println("Demo showMethod >>>>>>>" + name + " " + i); } } } public class Test { public static void main(String[] args) { Demo demo1 = new Demo("步惊云"); Demo demo2 = new Demo("秦霜"); // 任务1 >>>main线程在执行 demo1.showMethod(); // 任务2 >>>main线程在执行 demo2.showMethod(); // 任务3 >>>main线程在执行 for (int i = 0; i < 10; i++) { System.out.println("Test main " + i); } } }
执行的结果:
Demo showMethod >>>>>>>步惊云 0
Demo showMethod >>>>>>>步惊云 1
Demo showMethod >>>>>>>步惊云 2
Demo showMethod >>>>>>>步惊云 3
Demo showMethod >>>>>>>步惊云 4
Demo showMethod >>>>>>>步惊云 5
Demo showMethod >>>>>>>步惊云 6
Demo showMethod >>>>>>>步惊云 7
Demo showMethod >>>>>>>步惊云 8
Demo showMethod >>>>>>>步惊云 9
Demo showMethod >>>>>>>秦霜 0
Demo showMethod >>>>>>>秦霜 1
Demo showMethod >>>>>>>秦霜 2
Demo showMethod >>>>>>>秦霜 3
Demo showMethod >>>>>>>秦霜 4
Demo showMethod >>>>>>>秦霜 5
Demo showMethod >>>>>>>秦霜 6
Demo showMethod >>>>>>>秦霜 7
Demo showMethod >>>>>>>秦霜 8
Demo showMethod >>>>>>>秦霜 9
Test main 0
Test main 1
Test main 2
Test main 3
Test main 4
Test main 5
Test main 6
Test main 7
Test main 8
Test main 9
从以上结果可以看出:
这三个任务都是被main这一个线程来完成的,这样会造成 demo1.showMethod(); 执行的时候 demo2.showMethod(); 任务3处于等待状态
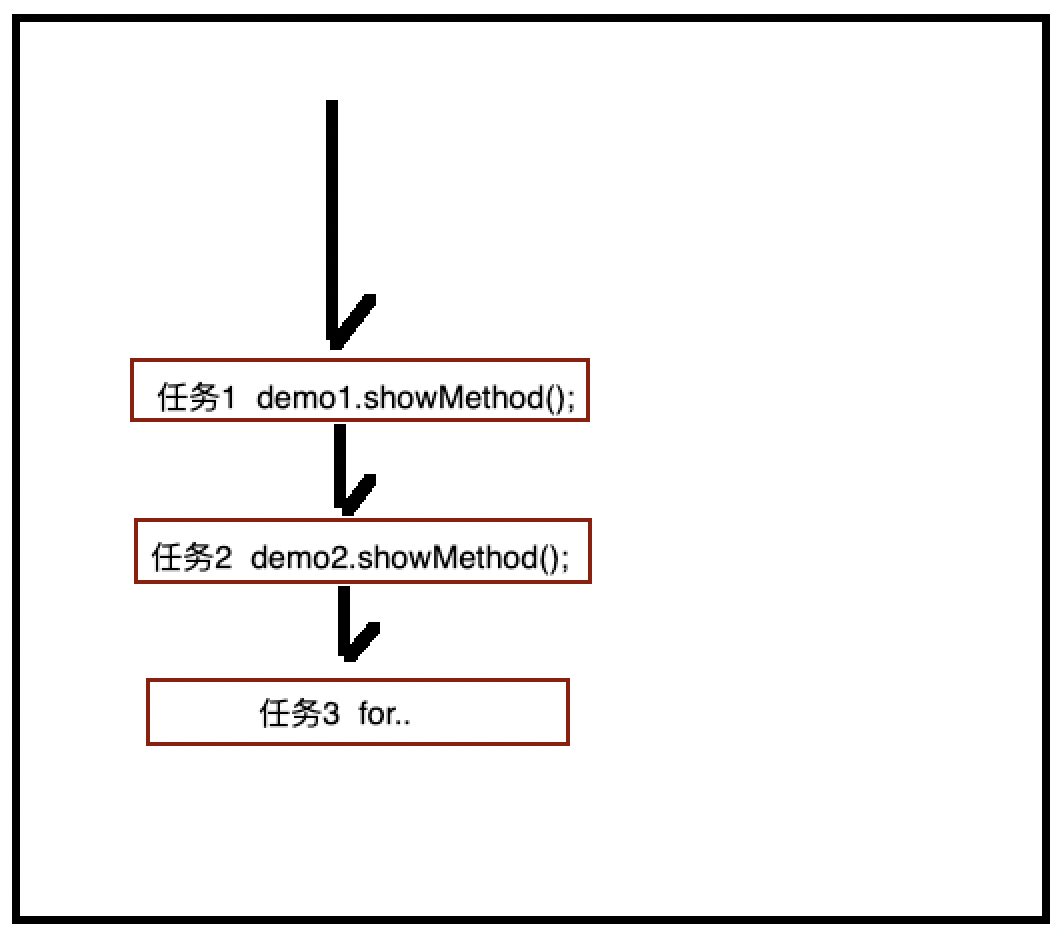
三个任务同时执行,使用Thread
package android.java.thread2; class Demo extends Thread { // 继承Thread 并重写 run() 方法,属于定义一个线程子类 private String name; public Demo(String name) { this.name = name; } /** * run方法里面执行的是start线程里面执行任务 */ @Override public void run() { super.run(); showMethod(); } public void showMethod() { for (int i = 0; i < 10; i++) { System.out.println("Demo showMethod >>>>>>>" + name + " " + i); } } } public class Test { public static void main(String[] args) { Demo demo1 = new Demo("步惊云"); Demo demo2 = new Demo("秦霜"); // 任务1 >>>启动一个新线程去执行任务 demo1.start(); // 任务2 >>>启动一个新线程去执行任务 demo2.start(); // 任务3 >>>main线程在执行 for (int i = 0; i < 10; i++) { System.out.println("Test main " + i); } } }
第一次执行结果:
Demo showMethod >>>>>>>步惊云 0
Demo showMethod >>>>>>>秦霜 0
Demo showMethod >>>>>>>秦霜 1
Demo showMethod >>>>>>>秦霜 2
Demo showMethod >>>>>>>秦霜 3
Demo showMethod >>>>>>>步惊云 1
Demo showMethod >>>>>>>步惊云 2
Demo showMethod >>>>>>>步惊云 3
Demo showMethod >>>>>>>步惊云 4
Demo showMethod >>>>>>>步惊云 5
Demo showMethod >>>>>>>步惊云 6
Demo showMethod >>>>>>>步惊云 7
Demo showMethod >>>>>>>秦霜 4
Demo showMethod >>>>>>>秦霜 5
Demo showMethod >>>>>>>秦霜 6
Demo showMethod >>>>>>>秦霜 7
Demo showMethod >>>>>>>秦霜 8
Demo showMethod >>>>>>>秦霜 9
Test main 0
Test main 1
Demo showMethod >>>>>>>步惊云 8
Demo showMethod >>>>>>>步惊云 9
Test main 2
Test main 3
Test main 4
Test main 5
Test main 6
Test main 7
Test main 8
Test main 9
第二次执行结果:
Demo showMethod >>>>>>>步惊云 0
Demo showMethod >>>>>>>步惊云 1
Demo showMethod >>>>>>>步惊云 2
Demo showMethod >>>>>>>步惊云 3
Demo showMethod >>>>>>>步惊云 4
Demo showMethod >>>>>>>步惊云 5
Demo showMethod >>>>>>>步惊云 6
Demo showMethod >>>>>>>步惊云 7
Demo showMethod >>>>>>>步惊云 8
Demo showMethod >>>>>>>步惊云 9
Demo showMethod >>>>>>>秦霜 0
Demo showMethod >>>>>>>秦霜 1
Demo showMethod >>>>>>>秦霜 2
Test main 0
Test main 1
Test main 2
Test main 3
Test main 4
Test main 5
Test main 6
Test main 7
Test main 8
Test main 9
Demo showMethod >>>>>>>秦霜 3
Demo showMethod >>>>>>>秦霜 4
Demo showMethod >>>>>>>秦霜 5
Demo showMethod >>>>>>>秦霜 6
Demo showMethod >>>>>>>秦霜 7
Demo showMethod >>>>>>>秦霜 8
Demo showMethod >>>>>>>秦霜 9
第三次执行结果:
Demo showMethod >>>>>>>步惊云 0
Demo showMethod >>>>>>>秦霜 0
Demo showMethod >>>>>>>秦霜 1
Demo showMethod >>>>>>>秦霜 2
Demo showMethod >>>>>>>秦霜 3
Demo showMethod >>>>>>>秦霜 4
Test main 0
Test main 1
Test main 2
Test main 3
Test main 4
Test main 5
Test main 6
Test main 7
Test main 8
Test main 9
Demo showMethod >>>>>>>秦霜 5
Demo showMethod >>>>>>>秦霜 6
Demo showMethod >>>>>>>秦霜 7
Demo showMethod >>>>>>>秦霜 8
Demo showMethod >>>>>>>秦霜 9
Demo showMethod >>>>>>>步惊云 1
Demo showMethod >>>>>>>步惊云 2
Demo showMethod >>>>>>>步惊云 3
Demo showMethod >>>>>>>步惊云 4
Demo showMethod >>>>>>>步惊云 5
Demo showMethod >>>>>>>步惊云 6
Demo showMethod >>>>>>>步惊云 7
Demo showMethod >>>>>>>步惊云 8
Demo showMethod >>>>>>>步惊云 9