字符流和字节流在写出的时候,如果文件不存在是会自动创建的,不需要手动创建文件。
并且,在写入文件时,是具有缓存的,如果没有关闭流,那么需要手动刷新。
1.1 字节输出流 OutputStream
OutputStream 此抽象类,是表示输出字节流的所有类的超类。操作的数据都是字节,定义了输出字节流的基本共性功能方法。
输出流中定义都是写 write 方法,如下图:
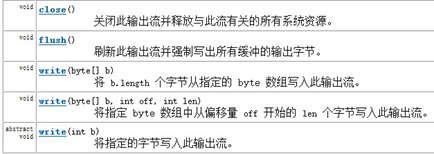
1.1.1 FileOutputStream 类
OutputStream 有很多子类,其中子类 FileOutputStream 可用来写入数据到文件。
FileOutputStream 类,即文件输出流,是用于将数据写入 File的输出流。
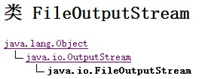
构造方法:

1.1.3在文件中续写和换行
我们直接new FileOutputStream(file)这样创建对象,写入数据,会覆盖原有的文件,
查阅API文档

在输出流的构造函数中,可以传入一个boolean类型的参数,来指定是否续写,如果值true,就会在文件末位继续添加。
代码演示:
public class FileOutputStreamDemo2 { public static void main(String[] args) throws Exception { File file = new File("c:\file.txt"); //创建的时候添加boolean值说明 FileOutputStream fos = new FileOutputStream(file, true); //添加转义字符 String str = " "+"itcast"; fos.write(str.getBytes()); fos.close(); } }
1.1.4- IO异常的处理
在前面编写代码中都发生了IO的异常。我们在实际开发中,对异常时如何处理的,我们来演示一下。
public class FileOutputStreamDemo3 { public static void main(String[] args) { File file = new File("c:\file.txt"); //定义FileOutputStream的引用 FileOutputStream fos = null; try { //创建FileOutputStream对象 fos = new FileOutputStream(file); //写出数据 fos.write("abcde".getBytes()); } catch (IOException e) { System.out.println(e.toString() + "----"); } finally { //一定要判断fos是否为null,只有不为null时,才可以关闭资源 if (fos != null) { try { fos.close(); } catch (IOException e) { throw new RuntimeException(""); } } } } }
字节输入流InputStream
通过前面的学习,我们可以把内存中的数据写出到文件中,那如何想把内存中的数据读到内存中,我们通过InputStream可以实现。InputStream此抽象类,是表示字节输入流的所有类的超类。,定义了字节输入流的基本共性功能方法。

-
int read():读取一个字节并返回,没有字节返回-1.
-
int read(byte[]): 读取一定量的字节数,并存储到字节数组中,返回读取到的字节数。
FileInputStream类
InputStream有很多子类,其中子类FileInputStream可用来读取文件内容。
FileInputStream 从文件系统中的某个文件中获得输入字节。
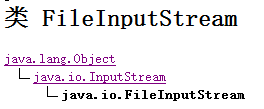
-
构造方法

FileInputStream类读取数据read方法
在读取文件中的数据时,调用read方法,实现从文件中读取数据

-
从文件中读取数据,代码演示:
public class FileInputStreamDemo { public static void main(String[] args) throws IOException { File file = new File("c:\file.txt"); //创建一个字节输入流对象,必须明确数据源,其实就是创建字节读取流和数据源相关联。 FileInputStream fis = new FileInputStream(file); //读取数据。使用 read();一次读一个字节。 int ch = 0; while((ch=fis.read())!=-1){ System.out.println("ch="+(char)ch); } // 关闭资源。 fis.close(); } }
读取数据read(byte[])方法
在读取文件中的数据时,调用read方法,每次只能读取一个,太麻烦了,于是我们可以定义数组作为临时的存储容器,这时可以调用重载的read方法,一次可以读取多个字符。

复制文件练习
1.直接复制的方法
public class CopyFileTest { public static void main(String[] args) throws IOException { //1,明确源和目的。 File srcFile = new File("c:\YesDir est.JPG"); File destFile = new File("copyTest.JPG"); //2,明确字节流 输入流和源相关联,输出流和目的关联。 FileInputStream fis = new FileInputStream(srcFile); FileOutputStream fos = new FileOutputStream(destFile); //3, 使用输入流的读取方法读取字节,并将字节写入到目的中。 int ch = 0; while((ch=fis.read())!=-1){ fos.write(ch); } //4,关闭资源。 fos.close(); fis.close(); } }
2.使用数组缓冲接收的方法
public class CopyFileByBufferTest { public static void main(String[] args) throws IOException { File srcFile = new File("c:\YesDir est.JPG"); File destFile = new File("copyTest.JPG"); // 明确字节流 输入流和源相关联,输出流和目的关联。 FileInputStream fis = new FileInputStream(srcFile); FileOutputStream fos = new FileOutputStream(destFile); //定义一个缓冲区。 byte[] buf = new byte[1024]; int len = 0; while ((len = fis.read(buf)) != -1) { fos.write(buf, 0, len);// 将数组中的指定长度的数据写入到输出流中。 } // 关闭资源。 fos.close(); fis.close(); } }