Binary Tree Traversals
Time Limit: 1000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 2484 Accepted Submission(s): 1078
Problem Description
A binary tree is a finite set of vertices that is either empty or consists of a root r and two disjoint binary trees called the left and right subtrees. There are three most important ways in which the vertices of a binary tree can be systematically traversed or ordered. They are preorder, inorder and postorder. Let T be a binary tree with root r and subtrees T1,T2.
In a preorder traversal of the vertices of T, we visit the root r followed by visiting the vertices of T1 in preorder, then the vertices of T2 in preorder.
In an inorder traversal of the vertices of T, we visit the vertices of T1 in inorder, then the root r, followed by the vertices of T2 in inorder.
In a postorder traversal of the vertices of T, we visit the vertices of T1 in postorder, then the vertices of T2 in postorder and finally we visit r.
Now you are given the preorder sequence and inorder sequence of a certain binary tree. Try to find out its postorder sequence.
In a preorder traversal of the vertices of T, we visit the root r followed by visiting the vertices of T1 in preorder, then the vertices of T2 in preorder.
In an inorder traversal of the vertices of T, we visit the vertices of T1 in inorder, then the root r, followed by the vertices of T2 in inorder.
In a postorder traversal of the vertices of T, we visit the vertices of T1 in postorder, then the vertices of T2 in postorder and finally we visit r.
Now you are given the preorder sequence and inorder sequence of a certain binary tree. Try to find out its postorder sequence.
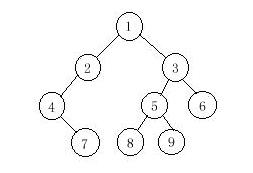
Input
The input contains several test cases. The first line of each test case contains a single integer n (1<=n<=1000), the number of vertices of the binary tree. Followed by two lines, respectively indicating the preorder sequence and inorder sequence. You can assume they are always correspond to a exclusive binary tree.
Output
For each test case print a single line specifying the corresponding postorder sequence.
Sample Input
9
1 2 4 7 3 5 8 9 6
4 7 2 1 8 5 9 3 6
Sample Output
7 4 2 8 9 5 6 3 1
Source
大概意思:给你先序序列和中序序列,求后序序列(二叉树)
import java.util.Scanner; public class Main{//AC static String str; public static void main(String[] args) { Scanner input=new Scanner(System.in); while(input.hasNext()){ //输入 str=""; String n=input.nextLine(); String pre1=input.nextLine(); String pre[]=pre1.split(" "); String rin1=input.nextLine(); String rin[]=rin1.split(" "); //创建根节点 Node node=BT(pre,rin); //后序遍历 PO(node); System.out.println(str.trim()); } } private static void PO(Node node) {//后序遍历 if(node!=null){ PO(node.lchild); PO(node.rchild); str+=" "+node.data; } } private static Node BT(String[] pre, String[] rin) {//递归 if(pre.length<=0) return null; //获取根节点 String s=pre[0]; //创建新节点 Node genjiedian=new Node(s); int index=0,i; //寻找根节点在中序中的位置 for(i=0;i<rin.length;i++){ if(s.equals(rin[i])){ index=i; break; } } //根据根节点,把中序分成左右两个子树 String lrin[]=new String[index]; String rrin[]=new String[rin.length-index-1]; for(i=0;i<index;i++) lrin[i]=rin[i]; i=index+1; for(int e=0;i<rin.length;i++) rrin[e++]=rin[i]; //除去开头的根节点,根据中序的左右子树的长度,把先序也分成等长度的左右子树 String lpre[]=new String[index]; String rpre[]=new String[pre.length-index-1]; for(i=0;i<index;i++){ lpre[i]=pre[i+1]; } for(i=0;i<pre.length-index-1;i++) rpre[i]=pre[i+index+1]; //新的递归,直到把每个分成单个根节点 genjiedian.lchild=BT(lpre,lrin); genjiedian.rchild=BT(rpre,rrin); //返回子树 return genjiedian; } } //创建二叉树 class Node{ String data; Node lchild; Node rchild; Node(String data){ this.data=data; this.lchild=null; this.rchild=null; } }
第二种方法,原理差不多
//8790508 2013-08-01 15:43:22 Accepted 1710 984MS 4872K 1822 B Java zhangyi import java.util.ArrayList; import java.util.List; import java.util.Scanner; public class Main { /** * @param args */ static String str; public static void main(String[] args) { // TODO Auto-generated method stub Scanner input=new Scanner(System.in); while(input.hasNext()){ str=""; List<Integer> pre=new ArrayList<Integer>(); List<Integer> in=new ArrayList<Integer>(); int n=input.nextInt(); for(int i=0;i<n;i++) pre.add(input.nextInt()); //将前序存到LinkedList pre里 for(int i=0;i<n;i++) in.add(input.nextInt());//将后序存到LinkedList in里 //建树 Node node=BT(pre,in); //遍历树,后序输出 PO(node); System.out.println(str.trim()); } } private static void PO(Node node) { // TODO Auto-generated method stub if(node!=null){ PO(node.lchild); PO(node.rchild); str+=" "+node.data; } } private static Node BT(List<Integer> pre, List<Integer> in) { // TODO Auto-generated method stub if(pre.size()<=0) return null; //添加节点 Node genjiedian=new Node(pre.get(0)); //获得根节点在中序中的位置 int d=in.indexOf(pre.get(0)); List<Integer> lpre=new ArrayList<Integer>(); List<Integer> lin=new ArrayList<Integer>();////新的左子树的前序、中序 List<Integer> rpre=new ArrayList<Integer>(); List<Integer> rin=new ArrayList<Integer>();////新的右子树的前序、中序 //获得新的左右子树的前序、中序 lpre=pre.subList(1,d+1); lin=in.subList(0,d); rpre=pre.subList(d+1,pre.size()); rin=in.subList(d+1,in.size()); genjiedian.lchild=BT(lpre,lin); genjiedian.rchild=BT(rpre,rin); return genjiedian; } } //树的数据类型结构类 class Node{ int data; Node lchild; Node rchild; Node(int data){ this.data=data; this.lchild=null; this.rchild=null; } }