模式动机:当某个接口可能有多种实现方式时,一般会使用继承来解决。但是继承总是体现出与具体的平台相关,无法很好地进行扩充,此时需要将接口与实现类最大可能地解耦,使其二者都可以独立发展而不受影响,增大系统的灵活性。
模式定义(Bridge Pattern):将抽象接口与具体实现分离开来,使得两部分都可以独立变化而不受影响。
模式结构图:
模式代码:
bt_桥接模式.h:
1 #ifndef BP_H 2 #define BP_H 3 #include <iostream> 4 using namespace std; 5 6 /* 7 具体操作接口 8 */ 9 class Implementor 10 { 11 public: 12 virtual ~Implementor(){ } 13 virtual void operationImpl() = 0; 14 }; 15 class ConcreteImplementorA : public Implementor 16 { 17 public: 18 virtual void operationImpl() 19 { 20 cout << "调用A类具体实现方法" << endl; 21 } 22 }; 23 class ConcreteImplementorB : public Implementor 24 { 25 public: 26 virtual void operationImpl() 27 { 28 cout << "调用B类具体实现方法" << endl; 29 } 30 }; 31 32 /* 33 抽象功能接口 34 */ 35 class Abstraction 36 { 37 public: 38 virtual ~Abstraction(){ } 39 virtual void operation() = 0; 40 virtual void setImpl(Implementor* impl) = 0; 41 42 protected: 43 Implementor* impl; 44 }; 45 46 class RefinedAbstraction : public Abstraction 47 { 48 public: 49 virtual void setImpl(Implementor* impl) 50 { 51 this->impl = impl; 52 } 53 virtual void operation() 54 { 55 impl->operationImpl(); 56 } 57 }; 58 59 #endif // BP_H
测试用例.cpp:
1 #include "bt_桥接模式.h" 2 3 int main() 4 { 5 cout << "***** 桥接模式测试 *****" << endl; 6 Implementor* pI = new ConcreteImplementorA; 7 Abstraction* pA = new RefinedAbstraction; 8 pA->setImpl(pI); 9 pA->operation(); 10 11 return 0; 12 }
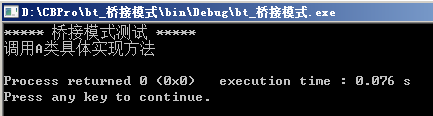
模式分析:桥接模式的优点主要包含如下三部分:
1> 分离接口及其实现:抽象类的具体实现部分可以延迟到运行时进行配置,一个对象即使在运行时也能改变其实现部分。同时客户只需知道Abstraction和Implementor接口即可,无需了解其他类的细节。
2> 可扩展性增强:可以独立地对Abstraction和Implementor层次结构进行扩充,二者是相互独立的,耦合性比较小。
3> 实现细节对于客户透明。