本文使用地址:http://ws.webxml.com.cn/WebServices/MobileCodeWS.asmx?wsdl
为开放免费地址
1.eclipse 生成代码
生成的代码:
调用样例:
package test; import cn.com.WebXml.MobileCodeWSSoapProxy; public class test { public static void main(String[] args) { String result=""; try { MobileCodeWSSoapProxy mobileCodeWSSoap = new MobileCodeWSSoapProxy("http://ws.webxml.com.cn/WebServices/MobileCodeWS.asmx?wsdl"); result = mobileCodeWSSoap.getMobileCodeInfo("188****9277", ""); System.out.println(result); } catch (Exception e) { e.printStackTrace(); } } }
输出结果:
188****9277:河北 邯郸 河北移动全球通卡
2. wsimport生成客户端代码
在{JAVA_HOME} in路径下有个wsimport.exe文件,该文件是jdk专为webservice生成代码用的
打开dos界面进入java的bin目录下:
wsimport -keep -d F:projectjava -s F:projectclass -p cn.itcast.mobile -verbose http://ws.webxml.com.cn/WebServices/MobileCodeWS.asmx?wsdl
参数意义:
-keep:是否生成java源文件
-d:指定.class文件的输出目录
-s:指定.java文件的输出目录
-p:定义生成类的包名,不定义的话有默认包名
-verbose:在控制台显示输出信息
如图:
生成代码如图:
代码实例:
package test; import java.net.MalformedURLException; import java.net.URL; import cn.itcast.mobile.MobileCodeWS; import cn.itcast.mobile.MobileCodeWSSoap; public class test { public static void main(String[] args) { try { MobileCodeWS mobileCodeWS= new MobileCodeWS(new URL("http://ws.webxml.com.cn/WebServices/MobileCodeWS.asmx?wsdl")); MobileCodeWSSoap soap = mobileCodeWS.getPort(MobileCodeWSSoap.class); String result = soap.getMobileCodeInfo("188****9277", ""); System.out.println(result); } catch (Exception e) { e.printStackTrace(); } } }
输出结果:
188****9277:河北 邯郸 河北移动全球通卡
3.编码方式
代码样例:
package test; import java.net.URL; import javax.xml.namespace.QName; import javax.xml.ws.Service; import cn.itcast.mobile.MobileCodeWSSoap; public class test { public static void main(String[] args) { String result=""; try { URL url = new URL("http://ws.webxml.com.cn/WebServices/MobileCodeWS.asmx?wsdl"); QName qname = new QName("http://WebXml.com.cn/","MobileCodeWS"); Service service = Service.create(url,qname); MobileCodeWSSoap mobileCodeWSSoap = service.getPort(MobileCodeWSSoap.class); result = mobileCodeWSSoap.getMobileCodeInfo("188****9277", ""); System.out.println(result); } catch (Exception e) { e.printStackTrace(); } } }
其中MobileCodeWSSoap方法是2中生成的类。
注掉的两行可以没有
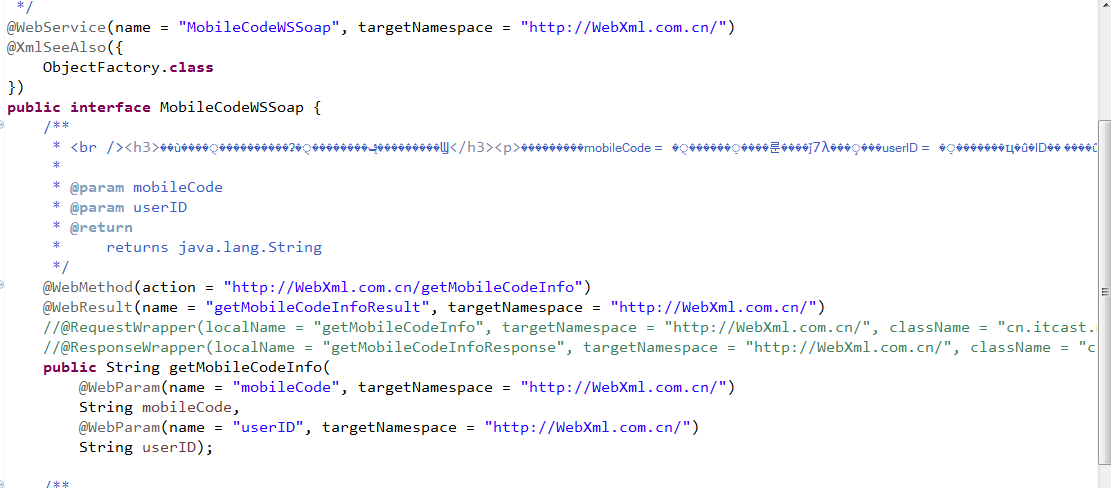
输出结果:
188****9277:河北邯郸河北移动全球通卡
4.4 通过http方式调用:
package test; import java.io.BufferedReader; import java.io.InputStream; import java.io.InputStreamReader; import java.io.OutputStream; import java.net.HttpURLConnection; import java.net.URL; import javax.xml.namespace.QName; import javax.xml.ws.Service; import cn.itcast.mobile.MobileCodeWSSoap; public class test { public static void main(String[] args) { String result=""; try { //第一步:创建服务地址,不是WSDL地址 URL url = new URL("http://webservice.webxml.com.cn/WebServices/MobileCodeWS.asmx"); //第二步:打开一个通向服务地址的连接 HttpURLConnection connection = (HttpURLConnection) url.openConnection(); //第三步:设置参数 //3.1发送方式设置:POST必须大写 connection.setRequestMethod("POST"); //3.2设置数据格式:content-type connection.setRequestProperty("content-type", "text/xml;charset=utf-8"); //3.3设置输入输出,因为默认新创建的connection没有读写权限, connection.setDoInput(true); connection.setDoOutput(true); //第四步:组织SOAP数据,发送请求 String soapXML = getXML("188****9277"); OutputStream os = connection.getOutputStream(); os.write(soapXML.getBytes()); //第五步:接收服务端响应,打印 int responseCode = connection.getResponseCode(); if(200 == responseCode){//表示服务端响应成功 InputStream is = connection.getInputStream(); InputStreamReader isr = new InputStreamReader(is); BufferedReader br = new BufferedReader(isr); StringBuilder sb = new StringBuilder(); String temp = null; while(null != (temp = br.readLine())){ sb.append(temp); } System.out.println(sb.toString()); is.close(); isr.close(); br.close(); } os.close(); } catch (Exception e) { e.printStackTrace(); } } public static String getXML(String phoneNum){ String soapXML = "<?xml version="1.0" encoding="utf-8"?>" +"<soap:Envelope xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/">" +"<soap:Body>" +"<getMobileCodeInfo xmlns="http://WebXml.com.cn/">" +"<mobileCode>"+phoneNum+"</mobileCode>" +"<userID></userID>" +"</getMobileCodeInfo>" +"</soap:Body>" +"</soap:Envelope>"; return soapXML; } }
输出结果:
<?xml version="1.0" encoding="utf-8"?><soap:Envelope xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema"><soap:Body><getMobileCodeInfoResponse xmlns="http://WebXml.com.cn/"><getMobileCodeInfoResult>188****9277:河北邯郸河北移动全球通卡</getMobileCodeInfoResult></getMobileCodeInfoResponse></soap:Body></soap:Envelope>
其中getMobileCodeInfoResult标签中的内容就是我们需要的返回结果。
5.ajax调用