题目链接:http://poj.org/problem?id=1753
参考链接:http://blog.csdn.net/lyy289065406/article/details/6642595
刚开始写的恶心的要命,每次得往上下左右每个节点判访问与搜索,直接超时。实际上只需要一个一个节点挨着搜索,要么同一行下个节点,要么下一行第一个节点。
另外就是用常规思路做的时候不要全部搜索比较最小值。实际上很容易看出来最多只需要走16步,多余的步数其实没有意义。因此从小到大枚举步数,一旦遇见可行解直接退出。
还有就是代码应该是有点小bug-第46 67行的stai < n - 1改成 stai < n 竟然答案会错,得思考思考。
该题还有一个比较有技巧性的方法,晚些时候看看再说。
代码:
1 #include <cstdio> 2 #include <iostream> 3 #include <cstdlib> 4 #include <cmath> 5 #include <cstring> 6 #include <string> 7 #include <algorithm> 8 #include <queue> 9 using namespace std; 10 #define inf 0x3f3f3f3f 11 12 int iadd[] = {0, 1, 0, -1}, jadd[] = {1, 0, -1, 0}; 13 14 int g[4][4]; 15 int n = 4; 16 int rd = 0, minrd = inf; 17 bool flag = false; 18 19 //all 1 20 int solve(int stai, int staj, int lezeron){ 21 if(flag) return 0; 22 if((lezeron == 0 || lezeron == 16) && rd == minrd){ 23 flag = true; 24 return 0; 25 } 26 if(rd >= minrd) return 0; 27 if(stai == n || staj == n) return 0; 28 //reverse it 29 rd++; 30 int tmlezero = lezeron; 31 g[stai][staj] = !g[stai][staj]; 32 if(g[stai][staj]) tmlezero--; 33 else tmlezero++; 34 for(int i = 0; i < n; i++){ 35 int nexti = stai + iadd[i], nextj = staj + jadd[i]; 36 if(nexti < 0 || nexti >= n || nextj < 0 || nextj >= n) 37 continue; 38 g[nexti][nextj] = !g[nexti][nextj]; 39 40 if(g[nexti][nextj]) tmlezero--; 41 else tmlezero++; 42 } 43 44 if(staj < n - 1){ 45 solve(stai, staj + 1, tmlezero); 46 } 47 else{ 48 solve(stai + 1, 0, tmlezero); 49 } 50 51 //no reverse 52 rd--; 53 g[stai][staj] = !g[stai][staj]; 54 55 for(int i = 0; i < n; i++){ 56 int nexti, nextj; 57 nexti = stai + iadd[i], nextj = staj + jadd[i]; 58 if(nexti < 0 || nexti >= n || nextj < 0 || nextj >= n) 59 continue; 60 g[nexti][nextj] = !g[nexti][nextj]; 61 } 62 63 tmlezero = lezeron; 64 65 if(staj < n - 1){ 66 solve(stai, staj + 1, tmlezero); 67 } 68 else{ 69 solve(stai + 1, 0, tmlezero); 70 } 71 72 return 0; 73 } 74 75 int main(){ 76 int tp = 0; 77 for(int i = 0; i < n; i++){ 78 for(int j = 0; j < n; j++){ 79 char tm; 80 scanf(" %c", &tm); 81 if(tm == 'b'){ 82 g[i][j] = 1; 83 } 84 else{ 85 g[i][j] = 0; 86 tp++; 87 } 88 } 89 } 90 for(int i = 0; i <= 16; i++){ 91 minrd = i; 92 rd = 0; 93 flag = false; 94 solve(0, 0, tp); 95 if(flag) 96 break; 97 } 98 if(flag) 99 printf("%d ", minrd); 100 else 101 printf("Impossible "); 102 }
题目:
Flip Game
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 44864 | Accepted: 19228 |
Description
Flip game is played on a rectangular 4x4 field with two-sided pieces placed on each of its 16 squares. One side of each piece is white and the other one is black and each piece is lying either it's black or white side up. Each round you flip 3 to 5 pieces, thus changing the color of their upper side from black to white and vice versa. The pieces to be flipped are chosen every round according to the following rules:
Consider the following position as an example:
bwbw
wwww
bbwb
bwwb
Here "b" denotes pieces lying their black side up and "w" denotes pieces lying their white side up. If we choose to flip the 1st piece from the 3rd row (this choice is shown at the picture), then the field will become:
bwbw
bwww
wwwb
wwwb
The goal of the game is to flip either all pieces white side up or all pieces black side up. You are to write a program that will search for the minimum number of rounds needed to achieve this goal.
- Choose any one of the 16 pieces.
- Flip the chosen piece and also all adjacent pieces to the left, to the right, to the top, and to the bottom of the chosen piece (if there are any).
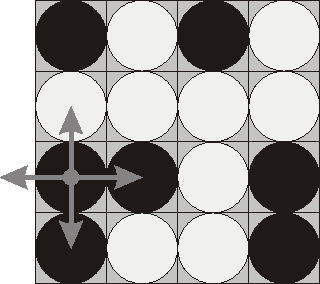
bwbw
wwww
bbwb
bwwb
Here "b" denotes pieces lying their black side up and "w" denotes pieces lying their white side up. If we choose to flip the 1st piece from the 3rd row (this choice is shown at the picture), then the field will become:
bwbw
bwww
wwwb
wwwb
The goal of the game is to flip either all pieces white side up or all pieces black side up. You are to write a program that will search for the minimum number of rounds needed to achieve this goal.
Input
The input consists of 4 lines with 4 characters "w" or "b" each that denote game field position.
Output
Write to the output file a single integer number - the minimum number of rounds needed to achieve the goal of the game from the given position. If the goal is initially achieved, then write 0. If it's impossible to achieve the goal, then write the word "Impossible" (without quotes).
Sample Input
bwwb bbwb bwwb bwww
Sample Output
4