1- 介绍
这篇教程文章是基于:
- Spring 4 MVC
2- 创建一个项目
- File/New/Other..
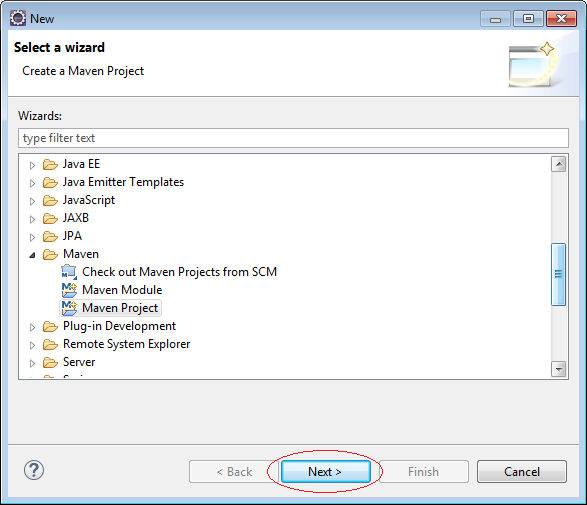

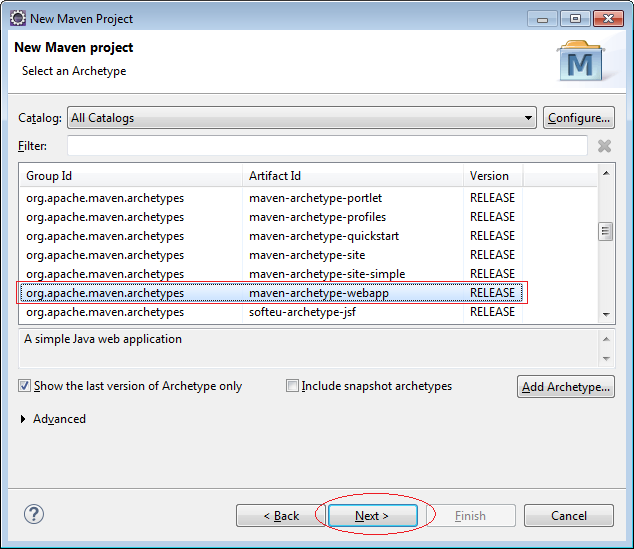
输入:
- Group ID: com.yiibai
- Artifact ID: SpringMVCResource
- Package: com.yiibai.springmvcresource
项目被创建以后如下:

不要担心有错误消息在项目被创建时。原因是,我们还没有声明 Servlet 库。
注意:
Eclipse 4.4(Luna)在创建 Maven 项目结构时可能是有错误的。需要修复。


3- 配置Maven
- pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.yiibai</groupId> <artifactId>SpringMVCResource</artifactId> <packaging>war</packaging> <version>0.0.1-SNAPSHOT</version> <name>SpringMVCResource Maven Webapp</name> <url>http://maven.apache.org</url> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> <!-- Servlet API --> <!-- http://mvnrepository.com/artifact/javax.servlet/javax.servlet-api --> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>3.1.0</version> <scope>provided</scope> </dependency> <!-- Jstl for jsp page --> <!-- http://mvnrepository.com/artifact/javax.servlet/jstl --> <dependency> <groupId>javax.servlet</groupId> <artifactId>jstl</artifactId> <version>1.2</version> </dependency> <!-- JSP API --> <!-- http://mvnrepository.com/artifact/javax.servlet.jsp/jsp-api --> <dependency> <groupId>javax.servlet.jsp</groupId> <artifactId>jsp-api</artifactId> <version>2.2</version> <scope>provided</scope> </dependency> <!-- Spring dependencies --> <!-- http://mvnrepository.com/artifact/org.springframework/spring-core --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>4.1.4.RELEASE</version> </dependency> <!-- http://mvnrepository.com/artifact/org.springframework/spring-web --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-web</artifactId> <version>4.1.4.RELEASE</version> </dependency> <!-- http://mvnrepository.com/artifact/org.springframework/spring-webmvc --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>4.1.4.RELEASE</version> </dependency> </dependencies> <build> <finalName>SpringMVCResource</finalName> <plugins> <!-- Config: Maven Tomcat Plugin --> <!-- http://mvnrepository.com/artifact/org.apache.tomcat.maven/tomcat7-maven-plugin --> <plugin> <groupId>org.apache.tomcat.maven</groupId> <artifactId>tomcat7-maven-plugin</artifactId> <version>2.2</version> <!-- Config: contextPath and Port (Default - /SpringMVCResource : 8080) --> <!-- <configuration> <path>/</path> <port>8899</port> </configuration> --> </plugin> </plugins> </build> </project>
4- 配置Spring
配置 web.xml
SpringContextListener 将读取配置文件参数 contextConfigLocation:


- WEB-INF/web.xml
<web-app xmlns="http://java.sun.com/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" version="3.0"> <display-name>Archetype Created Web Application</display-name> <servlet> <servlet-name>spring-mvc</servlet-name> <servlet-class> org.springframework.web.servlet.DispatcherServlet </servlet-class> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>spring-mvc</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping> <!-- Other XML Configuration --> <!-- Load by Spring ContextLoaderListener --> <context-param> <param-name>contextConfigLocation</param-name> <param-value> /WEB-INF/root-context.xml </param-value> </context-param> <!-- Spring ContextLoaderListener --> <listener> <listener-class>org.springframework.web.context.ContextLoaderListener</listener-class> </listener> </web-app>
配置Spring MVC:
- WEB-INF/spring-mvc-servlet.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.1.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.1.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-4.1.xsd"> <!-- Package Scan --> <context:component-scan base-package="com.yiibai.springmvcresource" /> <!-- Enables the Spring MVC Annotation Configuration --> <context:annotation-config /> <!-- Important!! --> <!-- Default Servlet Handler (For Resources *.css, *.js, image,..) --> <mvc:default-servlet-handler /> <mvc:annotation-driven /> <!-- Config resource mapping --> <mvc:resources mapping="/styles/**" location="/WEB-INF/resources/css/" /> <!-- Config Properties file --> <bean id="appProperties" class="org.springframework.beans.factory.config.PropertiesFactoryBean"> <property name="locations"> <list><value>classpath:ApplicationRB.properties</value></list> </property> </bean> <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="prefix"> <value>/WEB-INF/pages/</value> </property> <property name="suffix"> <value>.jsp</value> </property> <property name="exposedContextBeanNames"> <list><value>appProperties</value></list> </property> </bean> </beans>
- WEB-INF/root-context.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <!-- Empty --> </beans>
配置静态资源:
<!-- /WEB-INF/spring-mvc-servlet.xml --> <!-- Important!! --> <!-- Default Servlet Handler (For Resources *.css, *.js, image,..) --> <mvc:default-servlet-handler /> <mvc:annotation-driven />
资源映射 <mvc:resources mapping ... >:


配置Properties文件:
5- Java类
- MyController.java
package com.yiibai.springmvcresource; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; @Controller public class MyController { @RequestMapping(value = "/staticResourceTest") public String staticResource(Model model) { return "staticResourceTest"; } @RequestMapping(value = "/resourceBundleTest") public String resourceBundle(Model model) { return "resourceBundleTest"; } }
6- 资源包,静态资源和视图
Resource Bundle (Properties file):
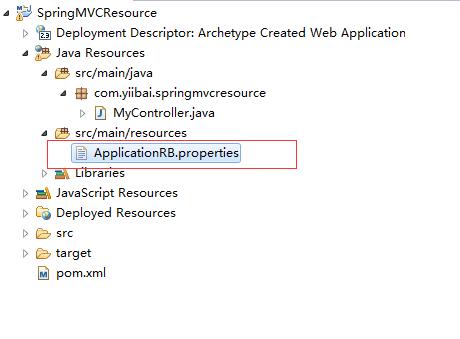
- ApplicationRB.properties
text.loginPrompt=Enter user name and password text.userName=User Name text.password=Password
静态资源


- scripts/common.js
function sayHello() { alert("Hello from JavaScript"); }
- /WEB-INF/resource/css/commons.css
.button { font-size: 20px; background: #ccc; } .red-text { color: red; font-size: 30px; } .green-text { color: green; font-size: 20px; }
视图(两个JSP文件)

- staticResourceTest.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Spring MVC Resource example</title> <script type="text/javascript" src="${pageContext.request.contextPath}/scripts/common.js"></script> <link rel="stylesheet" type="text/css" href="${pageContext.request.contextPath}/styles/common.css"> </head> <body> <div class="red-text">Red text</div> <br> <div class="green-text">Green text</div> <br> <input type="button" class="button" onclick="sayHello();" value="Click me!"> </body> </html>
- resourceBundleTest.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Spring MVC Resource Bundle example</title> </head> <body> <h2>${appProperties['text.loginPrompt']}</h2> ${appProperties['text.userName']} <input type="text" name="userName"> <br> ${appProperties['text.password']} <input type="password" name="password"> <br> </body> </html>
7- 运行应用程序
首先,运行应用程序之前,您需要构建整个项目。
右键单击项目并选择:




运行配置:


输入:
- Name: Run SpringMVCResource
- Base directory: ${workspace_loc:/SpringMVCResource} 可选择“Browse Workspace..." 来选对应项目。
- Goals: tomcat7:run
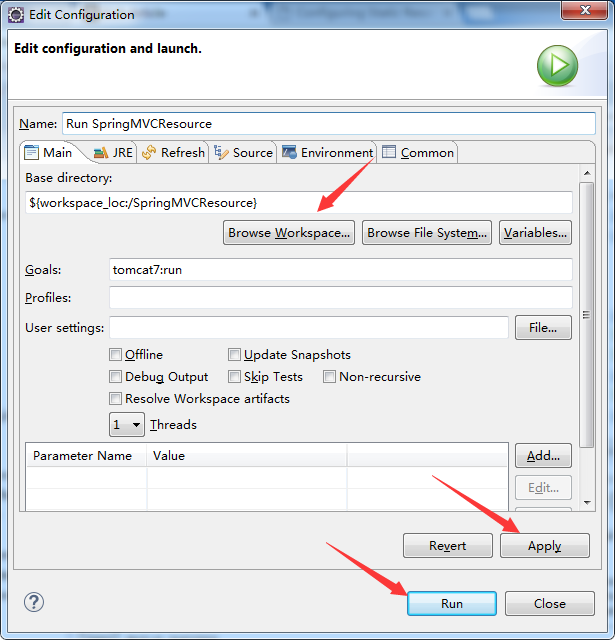
点击 Run
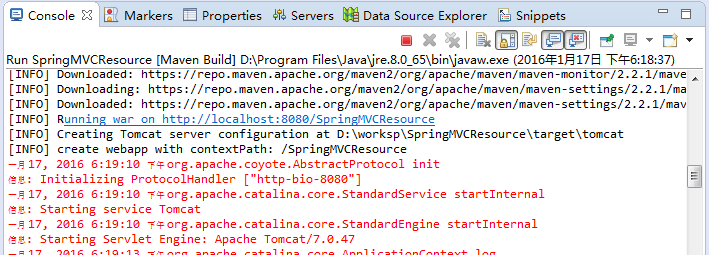
静态资源测试:
- http://localhost:8080/SpringMVCResource/scripts/common.js
- http://localhost:8080/SpringMVCResource/styles/common.css
- http://localhost:8080/SpringMVCResource/staticResourceTest
属性文件测试:
- http://localhost:8080/SpringMVCResource/resourceBundleTest