Binary Tree Traversals
Time Limit: 1000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 9283 Accepted Submission(s):
4193
Problem Description
A binary tree is a finite set of vertices that is
either empty or consists of a root r and two disjoint binary trees called the
left and right subtrees. There are three most important ways in which the
vertices of a binary tree can be systematically traversed or ordered. They are
preorder, inorder and postorder. Let T be a binary tree with root r and subtrees
T1,T2.
In a preorder traversal of the vertices of T, we visit the root r followed by visiting the vertices of T1 in preorder, then the vertices of T2 in preorder.
In an inorder traversal of the vertices of T, we visit the vertices of T1 in inorder, then the root r, followed by the vertices of T2 in inorder.
In a postorder traversal of the vertices of T, we visit the vertices of T1 in postorder, then the vertices of T2 in postorder and finally we visit r.
Now you are given the preorder sequence and inorder sequence of a certain binary tree. Try to find out its postorder sequence.
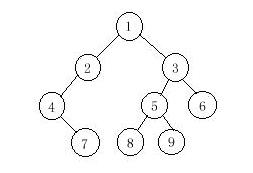
In a preorder traversal of the vertices of T, we visit the root r followed by visiting the vertices of T1 in preorder, then the vertices of T2 in preorder.
In an inorder traversal of the vertices of T, we visit the vertices of T1 in inorder, then the root r, followed by the vertices of T2 in inorder.
In a postorder traversal of the vertices of T, we visit the vertices of T1 in postorder, then the vertices of T2 in postorder and finally we visit r.
Now you are given the preorder sequence and inorder sequence of a certain binary tree. Try to find out its postorder sequence.
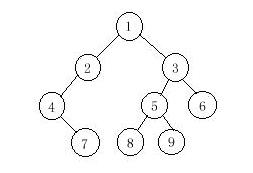
Input
The input contains several test cases. The first line
of each test case contains a single integer n (1<=n<=1000), the number of
vertices of the binary tree. Followed by two lines, respectively indicating the
preorder sequence and inorder sequence. You can assume they are always
correspond to a exclusive binary tree.
Output
For each test case print a single line specifying the
corresponding postorder sequence.
Sample Input
9
1 2 4 7 3 5 8 9 6
4 7 2 1 8 5 9 3 6
Sample Output
7 4 2 8 9 5 6 3 1
Source
Recommend
总结:记录下根结点,再拆分左右子树,一直搜下去。
1 #include<iostream> 2 #include <cstring> 3 #include <string> 4 #include <algorithm> 5 using namespace std; 6 7 typedef struct tree 8 { 9 int v; 10 tree *l, *r; 11 }; 12 tree *root; 13 14 tree *build(int *a, int *b, int n)//函数不能少了* 15 { 16 tree *s; 17 int i; 18 for (i = 1; i <= n; i++) 19 { 20 if (a[1] == b[i]) 21 { 22 s = (tree*)malloc(sizeof(tree));//开辟空间 23 s->v = b[i]; 24 s->l = build(a+1, b, i-1); 25 s->r = build(a + i, b + i, n - i ); 26 return s;//要记得返回 27 } 28 } 29 return NULL; 30 } 31 32 void postorder(tree *ro) 33 { 34 if (ro == NULL) return; 35 postorder(ro->l); 36 postorder(ro->r); 37 if (ro == root) 38 { 39 printf("%d ", ro->v); 40 } 41 else 42 { 43 printf("%d ", ro->v); 44 } 45 } 46 47 int main() 48 { 49 int n, a[1005], b[1005]; 50 while (cin>>n) 51 { 52 int i; 53 for (i = 1; i <=n; i++) 54 { 55 cin >> a[i]; 56 } 57 for (i = 1; i <= n; i++) 58 { 59 cin >> b[i]; 60 } 61 root = build(a, b, n); 62 postorder(root); 63 } 64 return 0; 65 }
另一种不建树的方法
1 #include <iostream> 2 #include <cstring> 3 #include <algorithm> 4 #define maxn 1111 5 int n, pre[maxn], in[maxn], post[maxn], id[maxn], res;//pre表示前序遍历序列,in表示中序遍历序列 6 void print(int a, int b, int c, int d)//a,b,c,d分别表示前序和中序遍历序列的起点和终点 7 { 8 int i = id[pre[a]];//根节点 9 int j = i - c;//中序遍历序列的左子树 10 int k = d - i;//中序遍历序列的右子树 11 if (j) print(a + 1, a + j, c, i - 1);//左子树非空则递归左子树 12 if (k) print(a + j + 1, b, i + 1, d);//右子树非空则递归右子树 13 post[res++] = pre[a]; 14 } 15 int main() 16 { 17 while (~scanf("%d", &n)) 18 { 19 res = 0; 20 for (int i = 0; i<n; i++) scanf("%d", &pre[i]); 21 for (int i = 0; i<n; i++) scanf("%d", &in[i]), id[in[i]] = i; 22 print(0, n - 1, 0, n - 1); 23 for (int i = 0; i<n; i++) 24 printf("%d%c", post[i], i == n - 1 ? ' ' : ' '); 25 } 26 return 0; 27 }

1 #include <stdio.h> 2 3 static int pre[1005]; 4 static int mid[1005]; 5 6 /** 7 每次处理数组中的一个小块 8 特点:先序和后序遍历任意子树都是连续的块 9 **/ 10 void post(int pre_index, int mid_index, int size, int is_root) 11 { 12 if (!size) { 13 return; 14 } 15 16 if (size == 1) 17 { 18 //打印先序 19 printf("%d ", pre[pre_index]); 20 return; 21 } 22 23 //每个(子)树根的位置 24 int root; 25 26 //找到根节点 27 for (root = 0; root < size && pre[pre_index] != 28 mid[mid_index + root]; root++); 29 30 //处理根的左边 31 post(pre_index + 1, mid_index, root, 0); 32 //处理根的右边 33 post(pre_index + root + 1, mid_index + root + 1, 34 size - root - 1, 0); 35 //是否是总根,打印根(相对) 36 is_root ? printf("%d ", pre[pre_index]) : 37 printf("%d ", pre[pre_index]); 38 } 39 40 int main() 41 { 42 int n, i; 43 n = 9; 44 while (~scanf("%d", &n)) 45 { 46 for (i = 0; i < n; i++) 47 scanf("%d", &pre[i]); 48 for (i = 0; i < n; i++) 49 scanf("%d", &mid[i]); 50 post(0, 0, n, 1); 51 } 52 return 0; 53 }