G.String Transformation
题目描述
Bobo has a string S = s1 s2...sn consists of letter a , b and c . He can transform the string by inserting or deleting substrings aa , bb and abab .
Formally, A = u ? w ? v (“ ? ” denotes string concatenation) can be transformed into A 0 = u ? v and vice versa where u , v are (possibly empty) strings and w ∈ { aa , bb , abab } .
Given the target string T = t1 t2 . . . tm , determine if Bobo can transform the string S into T .
Formally, A = u ? w ? v (“ ? ” denotes string concatenation) can be transformed into A 0 = u ? v and vice versa where u , v are (possibly empty) strings and w ∈ { aa , bb , abab } .
Given the target string T = t1 t2 . . . tm , determine if Bobo can transform the string S into T .
输入
The input consists of several test cases and is terminated by end-of-file.
The first line of each test case contains a string s1 s2 ...sn . The second line contains a string t1 t2 . . . tm .
The first line of each test case contains a string s1 s2 ...sn . The second line contains a string t1 t2 . . . tm .
输出
For each test case, print Yes if Bobo can. Print No otherwise.
• 1 ≤ n, m ≤ 104
• s1 , s2 ,..., sn , t1 , t2 , . . . , tm ∈ { a , b , c }
• The sum of n and m does not exceed 250,000.
• 1 ≤ n, m ≤ 104
• s1 , s2 ,..., sn , t1 , t2 , . . . , tm ∈ { a , b , c }
• The sum of n and m does not exceed 250,000.
样例输入
ab
ba
ac
ca
a
ab
样例输出
Yes
No
No
提示
For the first sample, Bobo can transform as ab => aababb => babb => ba .
题意:给定字符串S和T(均只由a,b,c三个字母组成),可对S进行插入或删除操作(插入或删除的子串只能是"aa","bb"或"abab"),问能否通过操作将S变为T
麻烦的模拟题,不管多麻烦的ababa的式子(不含c时)最终只会化成三种形式:a,b,ab(或ba),题目中插入删除abab的意义所在就是告诉我们ab能够转化成ba
我的思路:将两个式子化解成最优。
eg:abaabcaacabc 化简 accabc,化简使用队列,边放进去边判,队末尾是a,要放的也是a,那就pop(a)
ababaccbac 化简 accbac
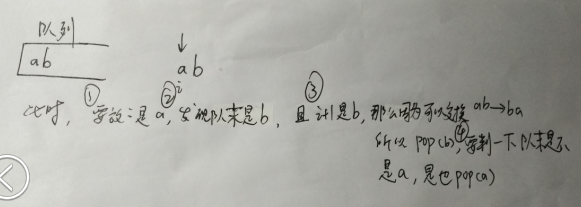
然后在从队首把他们取出来进行比较,特判一下ab和ba是一样的。
#include <iostream> #include<cstring> #include<string> #include<cstdio> #include<algorithm> #include<cmath> #include<deque> #define ll long long using namespace std; char a[10005]; char b[10005]; deque<int>q1,q2; int main() { while(cin>>a>>b) { while(!q1.empty ()) q1.pop_back(); while(!q2.empty ()) q2.pop_back(); int la=strlen(a); int lb=strlen(b); int c1=0; int c2=0; for(int i=0;i<la;i++) { if(a[i]=='c') { q1.push_back(3); c1++; } else if(a[i]=='a') { if(q1.empty ()) { q1.push_back(1); continue; } if(q1.back()==1) { q1.pop_back(); } else if(q1.back()==2) { if(i<la-1&&a[i+1]=='b') { q1.pop_back(); i++; if(q1.empty ()||q1.back ()!=1) { q1.push_back (1); } else if(q1.back ()==1) { q1.pop_back(); } } else q1.push_back (1); } else q1.push_back (1); } else if(a[i]=='b') { if(q1.empty ()) { q1.push_back(2); continue; } if(q1.back()==2) { q1.pop_back(); } else if(q1.back()==1) { if(i<la-1&&a[i+1]=='a') { q1.pop_back(); i++; if(q1.empty ()||q1.back ()!=2) { q1.push_back (2); } else if(q1.back ()==2) { q1.pop_back(); } } else q1.push_back(2); } else q1.push_back(2); } } for(int i=0;i<lb;i++) { if(b[i]=='c') { q2.push_back(3); c2++; } else if(b[i]=='a') { if(q2.empty ()) { q2.push_back(1); continue; } if(q2.back()==1) { q2.pop_back(); } else if(q2.back()==2) { if(i<lb-1&&b[i+1]=='b') { q2.pop_back(); i++; if(q2.empty ()||q2.back ()!=1) { q2.push_back (1); } else if(q2.back ()==1) { q2.pop_back(); } } else q2.push_back (1); } else q2.push_back (1); } else if(b[i]=='b') { if(q2.empty ()) { q2.push_back(2); continue; } if(q2.back()==2) { q2.pop_back(); } else if(q2.back()==1) { if(i<lb-1&&b[i+1]=='a') { q2.pop_back(); i++; if(q2.empty ()||q2.back ()!=2) { q2.push_back (2); } else if(q2.back ()==2) { q2.pop_back(); } } else q2.push_back(2); } else q2.push_back(2); } } bool f=1; if(c1!=c2||q1.size()!=q2.size()) f=0; else { while(!q1.empty ()) { if(q1.front() ==1&&q2.front() ==2) { q1.pop_front (); q2.pop_front (); if(q1.empty ()) { f=0; break; } else if(q1.front() ==2&&q2.front() ==1) { q1.pop_front (); q2.pop_front (); } else { f=0; break; } } else if(q1.front() ==2&&q2.front() ==1) { q1.pop_front (); q2.pop_front (); if(q1.empty ()) { f=0; break; } else if(q1.front() ==1&&q2.front() ==2) { q1.pop_front (); q2.pop_front (); } else { f=0; break; } } else if(q1.front()==q2.front ()) { q1.pop_front(); q2.pop_front(); } else { f=0; break; } } } if(f) printf("Yes "); else printf("No "); } return 0; }