Prime Path
Description
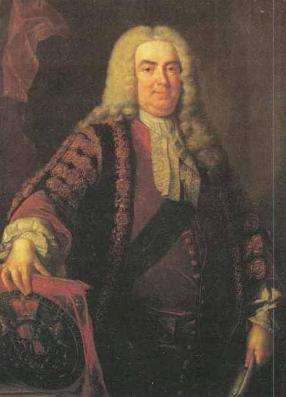
— It is a matter of security to change such things every now and then, to keep the enemy in the dark.
— But look, I have chosen my number 1033 for good reasons. I am the Prime minister, you know!
— I know, so therefore your new number 8179 is also a prime. You will just have to paste four new digits over the four old ones on your office door.
— No, it’s not that simple. Suppose that I change the first digit to an 8, then the number will read 8033 which is not a prime!
— I see, being the prime minister you cannot stand having a non-prime number on your door even for a few seconds.
— Correct! So I must invent a scheme for going from 1033 to 8179 by a path of prime numbers where only one digit is changed from one prime to the next prime.
Now, the minister of finance, who had been eavesdropping, intervened.
— No unnecessary expenditure, please! I happen to know that the price of a digit is one pound.
— Hmm, in that case I need a computer program to minimize the cost. You don't know some very cheap software gurus, do you?
— In fact, I do. You see, there is this programming contest going on... Help the prime minister to find the cheapest prime path between any two given four-digit primes! The first digit must be nonzero, of course. Here is a solution in the case above.
1033The cost of this solution is 6 pounds. Note that the digit 1 which got pasted over in step 2 can not be reused in the last step – a new 1 must be purchased.
1733
3733
3739
3779
8779
8179
Input
One line with a positive number: the number of test cases (at most 100). Then for each test case, one line with two numbers separated by a blank. Both numbers are four-digit primes (without leading zeros).
Output
One line for each case, either with a number stating the minimal cost or containing the word Impossible.
Sample Input
3 1033 8179 1373 8017 1033 1033
Sample Output
6 7 0
题意 将a转换到b,每次换一位,每次交换后的数都是素数,算最短换了多少次
我的思路
找到所有的四位数的素数,打表
用bfs 有四十个方向,找到答案
1 #include <stdio.h> 2 #include <stdlib.h> 3 #include <string.h> 4 int prime[10000],n,m,tail,head; 5 struct que 6 { 7 int x,time; 8 }que[10000]; 9 int row(int x) 10 { 11 int i,s=1; 12 for(i=1;i<=x;i++) 13 s*=10; 14 return s; 15 } 16 int bfs() 17 { 18 int i,j,x; 19 for(i=1000;i<=9999;i++) 20 { 21 for(j=2;j<=i/2;j++) 22 if(i%j==0)break; 23 if(j==i/2+1)prime[i]=1; 24 else prime[i]=0; 25 } 26 prime[n]=0; 27 tail=head=0; 28 que[0].x=n,que[0].time=0; 29 while(head<=tail) 30 { 31 for(i=1;i<=4;i++) 32 { 33 for(j=0;j<=9;j++) 34 { 35 x=que[head].x-(que[head].x/row(i-1)%10)*row(i-1)+j*row(i-1); 36 if(x>=1000&&x<=9999&&prime[x]) 37 { 38 que[++tail].x=x; 39 que[tail].time=que[head].time+1; 40 if(x==m)return que[tail].time; 41 else prime[x]=0; 42 } 43 } 44 } 45 head++; 46 } 47 return 0; 48 } 49 int main() 50 { 51 int i,j,key,g; 52 while(scanf("%d",&g)==1) 53 { 54 for(i=0;i<g;i++) 55 { 56 scanf("%d %d",&n,&m); 57 if(n==m) 58 { 59 printf("0 "); 60 continue; 61 } 62 key=bfs(); 63 if(key) 64 printf("%d ",key); 65 else 66 printf("Impossible "); 67 } 68 } 69 return 0; 70 }