Easy
A binary tree is univalued if every node in the tree has the same value.
Return true
if and only if the given tree is univalued.
Example 1:
Input: [1,1,1,1,1,null,1]
Output: true
Example 2:
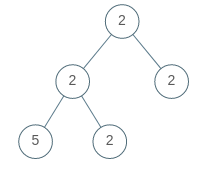
Input: [2,2,2,5,2]
Output: false
Note:
- The number of nodes in the given tree will be in the range
[1, 100]
. - Each node's value will be an integer in the range
[0, 99]
.
题目大意:
如果二叉树内的所有节点的值都一样,那么称之为univalued 是统一值的。
方法:这道题不需要按照某个顺序来遍历,只要随便按照一个顺序遍历整个二叉树,比较他们的节点值是不是有不同的就好了。
把所有的节点值都和根节点的值对比,很快最佳情况下只要一次比较就能结束。
方法一:递归
代码如下:
class Solution { public: bool isUnivalTree(TreeNode* root) { int cur=root->val; bool res=true; if(root->left){ res=res && helper(root->left,cur); } if(root->right){ res = res && helper(root->right,cur); } return res; } bool helper(TreeNode* root,int val){ if(root->val!=val)return false; bool res=true; if(root->left){ res=res && helper(root->left,val); } if(root->right){ res = res && helper(root->right,val); } return res; } };
方法二:迭代
使用堆栈记录节点,这里的遍历方式是层遍历,也可以使用其他的遍历方式。
代码如下:
class Solution { public: bool isUnivalTree(TreeNode* root) { int cur=root->val; stack<TreeNode*> s{{root}}; while(!s.empty()){ TreeNode* temp=s.top(); s.pop(); if(cur!=temp->val){ return false; } if(temp->left){ s.push(temp->left); } if(temp->right){ s.push(temp->right); } } return true; } };