Description
Several startup companies have decided to build a better Internet, called the "FiberNet". They have already installed many nodes that act as routers all around the world. Unfortunately, they started to quarrel about the connecting lines, and ended up with every company laying its own set of cables between some of the nodes.
Now, service providers, who want to send data from node A to node B are curious, which company is able to provide the necessary connections. Help the providers by answering their queries.
Now, service providers, who want to send data from node A to node B are curious, which company is able to provide the necessary connections. Help the providers by answering their queries.
Input
The
input contains several test cases. Each test case starts with the
number of nodes of the network n. Input is terminated by n=0. Otherwise,
1<=n<=200. Nodes have the numbers 1, ..., n. Then follows a list
of connections. Every connection starts with two numbers A, B. The list
of connections is terminated by A=B=0. Otherwise, 1<=A,B<=n, and
they denote the start and the endpoint of the unidirectional connection,
respectively. For every connection, the two nodes are followed by the
companies that have a connection from node A to node B. A company is
identified by a lower-case letter. The set of companies having a
connection is just a word composed of lower-case letters.
After the list of connections, each test case is completed by a list of queries. Each query consists of two numbers A, B. The list (and with it the test case) is terminated by A=B=0. Otherwise, 1<=A,B<=n, and they denote the start and the endpoint of the query. You may assume that no connection and no query contains identical start and end nodes.
After the list of connections, each test case is completed by a list of queries. Each query consists of two numbers A, B. The list (and with it the test case) is terminated by A=B=0. Otherwise, 1<=A,B<=n, and they denote the start and the endpoint of the query. You may assume that no connection and no query contains identical start and end nodes.
Output
For
each query in every test case generate a line containing the
identifiers of all the companies, that can route data packages on their
own connections from the start node to the end node of the query. If
there are no companies, output "-" instead. Output a blank line after
each test case. 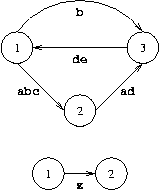
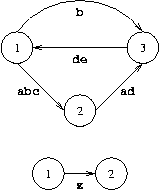
Sample Input
3 1 2 abc 2 3 ad 1 3 b 3 1 de 0 0 1 3 2 1 3 2 0 0 2 1 2 z 0 0 1 2 2 1 0 0 0
Sample Output
ab d - z -
Source
传递闭包,不过在TOJ超时了。应该有种更加牛X的做法。
1 #include <stdio.h> 2 #include <string.h> 3 #define MAXN 220 4 5 int n; 6 int f[MAXN][MAXN][26]; 7 8 void floyd(){ 9 for(int k=1; k<=n; k++){ 10 for(int i=1; i<=n; i++){ 11 for(int j=1; j<=n; j++){ 12 for(int c=0; c<26; c++){ 13 if( f[i][k][c] && f[k][j][c]) 14 f[i][j][c]=1; 15 } 16 } 17 } 18 } 19 } 20 int main() 21 { 22 while( scanf("%d" ,&n)!=EOF && n){ 23 memset(f,0,sizeof(f)); 24 int u,v; 25 char ch[30]; 26 while( scanf("%d %d",&u ,&v) ){ 27 if(u==0 && v==0)break; 28 scanf("%s",ch); 29 for(int i=0; ch[i]!='