开发自定义异常和配置
- 自定义异常 继承RuntimeException
- 开发异常处理器ExceptionHandle
YBException.java
package net.ybclass.online_ybclass.exception;
/**
* 自定义异常类
*/
public class YBException extends RuntimeException {
private Integer code;
private String msg;
public YBException(Integer code, String msg) {
this.code = code;
this.msg = msg;
}
public Integer getCode() {
return code;
}
public void setCode(Integer code) {
this.code = code;
}
public String getMsg() {
return msg;
}
public void setMsg(String msg) {
this.msg = msg;
}
}
CustomExceptionHandle.java
package net.ybclass.online_ybclass.exception;
import net.ybclass.online_ybclass.utils.JsonData;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseBody;
/**
* 异常处理类
*/
@ControllerAdvice
public class CustomExceptionHandle {
private final static Logger logger = LoggerFactory.getLogger(CustomExceptionHandle.class);
//监听这个异常
@ExceptionHandler(value = Exception.class)
@ResponseBody
public JsonData handle(Exception ex){
logger.error("[ 系统异常 ]{}",ex);
if (ex instanceof YBException){
YBException ybException=(YBException)ex;
return JsonData.buildError(ybException.getCode(),ybException.getMsg());
}else {
return JsonData.buildError("全局异常,未知从错误");
}
}
}
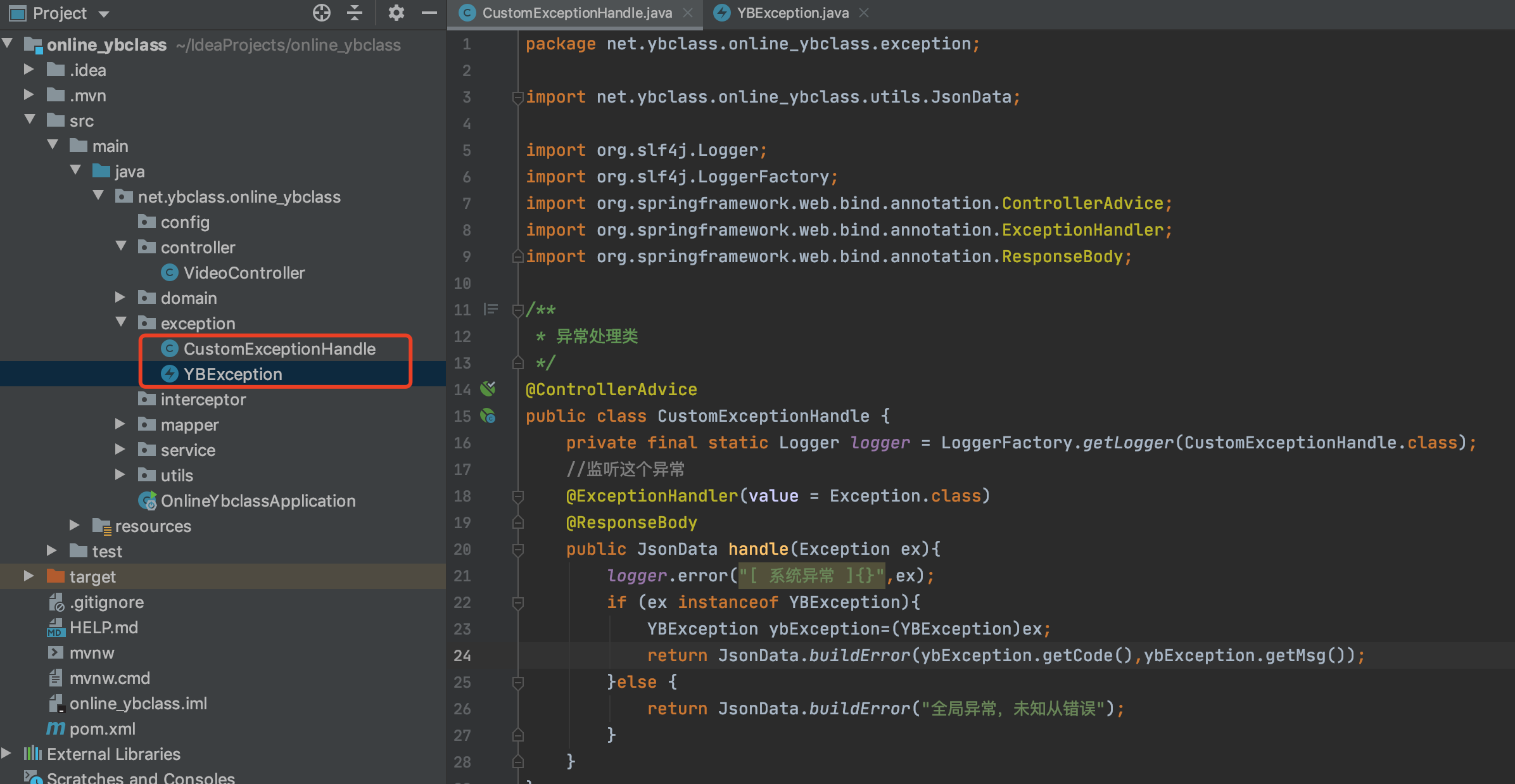
JsonData.java
package net.ybclass.online_ybclass.utils;
public class JsonData {
/**
* 状态码,0表示成功过,1表示处理中,-1表示失败
*/
private Integer code;
/**
* 业务数据
*/
private Object data;
/**
* 信息描述
*/
private String msg;
public JsonData() {
}
public JsonData(Integer code, Object data, String msg) {
this.code = code;
this.data = data;
this.msg = msg;
}
/**
* 成功,不用返回数据
* @return
*/
public static JsonData buildSuccess() {
return new JsonData(0, null, null);
}
/**
* 成功,返回数据
* @param data 返回数据
* @return
*/
public static JsonData buildSuccess(Object data) {
return new JsonData(0, data, null);
}
/**
* 失败,返回信息
* @param msg 返回信息
* @return
*/
public static JsonData buildError(String msg) {
return new JsonData(-1, null, msg);
}
/**
* 失败,返回信息和状态码
* @param code 状态码
* @param msg 返回信息
* @return
*/
public static JsonData buildError(Integer code, String msg) {
return new JsonData(code, null, msg);
}
public Integer getCode() {
return code;
}
public void setCode(Integer code) {
this.code = code;
}
public Object getData() {
return data;
}
public void setData(Object data) {
this.data = data;
}
public String getMsg() {
return msg;
}
public void setMsg(String msg) {
this.msg = msg;
}
}