In Spring 3, one of the feature of “mvc:annotation-driven“, is support for convert object to/from XML file, if JAXB is in project classpath.
In this tutorial, we show you how to convert a return object into XML format and return it back to user via Spring @MVC framework.
Technologies used :
- Spring 3.0.5.RELEASE
- JDK 1.6
- Eclipse 3.6
- Maven 3
JAXB is included in JDK6, so, you do not need to include JAXB library manually, as long as object is annotated with JAXB annotation, Spring will convert it into XML format automatically.
2. Model + JAXB
A simple POJO model and annotated with JAXB annotation, later convert this object into XML output.
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement(name = "coffee")
public class Coffee {
String name;
int quanlity;
public String getName() {
return name;
}
@XmlElement
public void setName(String name) {
this.name = name;
}
public int getQuanlity() {
return quanlity;
}
@XmlElement
public void setQuanlity(int quanlity) {
this.quanlity = quanlity;
}
public Coffee(String name, int quanlity) {
this.name = name;
this.quanlity = quanlity;
}
public Coffee() {
}
}
3. Controller
Add “@ResponseBody” in the method return value, no much detail in the Spring documentation.
As i know, when Spring see
- Object annotated with JAXB
- JAXB library existed in classpath
- “mvc:annotation-driven” is enabled
- Return method annotated with @ResponseBody
It will handle the conversion automatically.
package com.mkyong.common.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
import com.mkyong.common.model.Coffee;
@Controller
@RequestMapping("/coffee")
public class XMLController {
@RequestMapping(value="{name}", method = RequestMethod.GET)
public @ResponseBody Coffee getCoffeeInXML(@PathVariable String name) {
Coffee coffee = new Coffee(name, 100);
return coffee;
}
}
4. mvc:annotation-driven
In one of your Spring configuration XML file, enable “mvc:annotation-driven
“.
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.0.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-3.0.xsd">
<context:component-scan base-package="com.mkyong.common.controller" />
<mvc:annotation-driven />
</beans>
Alternatively, you can declares “spring-oxm.jar” dependency and include following MarshallingView
, to handle the conversion. With this method, you don’t need annotate @ResponseBody in your method.
<bean class="org.springframework.web.servlet.view.BeanNameViewResolver" />
<bean id="xmlViewer"
class="org.springframework.web.servlet.view.xml.MarshallingView">
<constructor-arg>
<bean class="org.springframework.oxm.jaxb.Jaxb2Marshaller">
<property name="classesToBeBound">
<list>
<value>com.mkyong.common.model.Coffee</value>
</list>
</property>
</bean>
</constructor-arg>
</bean>
</beans>
5. Demo
URL : http://localhost:8080/SpringMVC/rest/coffee/arabica
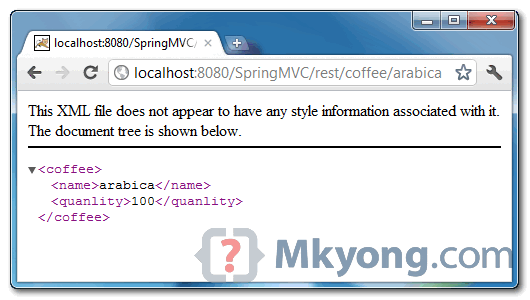