Eight II
Time Limit: 4000/2000 MS (Java/Others) Memory Limit: 130000/65536 K (Java/Others)Total Submission(s): 1645 Accepted Submission(s): 349
Problem Description
Eight-puzzle, which is also called "Nine grids", comes from an old game.
In this game, you are given a 3 by 3 board and 8 tiles. The tiles are numbered from 1 to 8 and each covers a grid. As you see, there is a blank grid which can be represented as an 'X'. Tiles in grids having a common edge with the blank grid can be moved into that blank grid. This operation leads to an exchange of 'X' with one tile.
We use the symbol 'r' to represent exchanging 'X' with the tile on its right side, and 'l' for the left side, 'u' for the one above it, 'd' for the one below it.

A state of the board can be represented by a string S using the rule showed below.
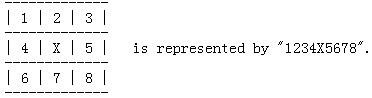
The problem is to operate an operation list of 'r', 'u', 'l', 'd' to turn the state of the board from state A to state B. You are required to find the result which meets the following constrains:
1. It is of minimum length among all possible solutions.
2. It is the lexicographically smallest one of all solutions of minimum length.
In this game, you are given a 3 by 3 board and 8 tiles. The tiles are numbered from 1 to 8 and each covers a grid. As you see, there is a blank grid which can be represented as an 'X'. Tiles in grids having a common edge with the blank grid can be moved into that blank grid. This operation leads to an exchange of 'X' with one tile.
We use the symbol 'r' to represent exchanging 'X' with the tile on its right side, and 'l' for the left side, 'u' for the one above it, 'd' for the one below it.

A state of the board can be represented by a string S using the rule showed below.
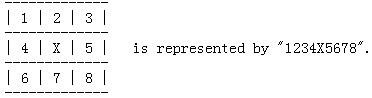
The problem is to operate an operation list of 'r', 'u', 'l', 'd' to turn the state of the board from state A to state B. You are required to find the result which meets the following constrains:
1. It is of minimum length among all possible solutions.
2. It is the lexicographically smallest one of all solutions of minimum length.
Input
The first line is T (T <= 200), which means the number of test cases of this problem.
The input of each test case consists of two lines with state A occupying the first line and state B on the second line.
It is guaranteed that there is an available solution from state A to B.
The input of each test case consists of two lines with state A occupying the first line and state B on the second line.
It is guaranteed that there is an available solution from state A to B.
Output
For each test case two lines are expected.
The first line is in the format of "Case x: d", in which x is the case number counted from one, d is the minimum length of operation list you need to turn A to B.
S is the operation list meeting the constraints and it should be showed on the second line.
The first line is in the format of "Case x: d", in which x is the case number counted from one, d is the minimum length of operation list you need to turn A to B.
S is the operation list meeting the constraints and it should be showed on the second line.
Sample Input
2 12X453786 12345678X 564178X23 7568X4123
Sample Output
Case 1: 2 dd Case 2: 8 urrulldr
八数码问题的升级版,但是思路差不多,这里学习使用IDA*算法来解,对于IDA*算法,为了利用深度搜索的优势解决广度A*的空间问题,其代价是可能会产生重复搜索。
首先,将初始化结点的一个Limit值设置为最大上限,然后进行深度优先搜索,搜索过程中忽略所有H值大于Limit的点,如果没有找到解,则加大Limit值继续搜索,直到找到一个点,在保证Limit值的情况下,可以证明找到的这解是最优解。
公式是:当前局面的估价函数值 + 当前的搜索深度 > 预定义的最大搜索深度时,就进行剪枝。
代码如下:
/************************************************************************* > File Name: Eight_3.cpp > Author: Zhanghaoran > Mail: chilumanxi@xiyoulinux.org > Created Time: Sun 11 Oct 2015 01:14:22 PM CST ************************************************************************/ #include <iostream> #include <algorithm> #include <cstring> #include <cstdio> #include <cstdlib> using namespace std; const int INF = 0x3f3f3f3f; struct Node{ int a, b; Node(int x = 0, int y = 0){ a = x; b = y; } }; int T; const int di[4][2] = {{1, 0}, {0, -1}, {0, 1}, {-1, 0}}; const char dir[4] = {'d', 'l', 'r', 'u'}; bool ok; int Map[3][3]; Node f[11]; int ans[300]; int Limit; int nowx, nowy; int get_h(){ int temp = 0; for(int i = 0; i < 9; i ++){ int x = i / 3; int y = i % 3; if(Map[x][y] == 0) continue; temp += abs(x - f[Map[x][y]].a) + abs(y - f[Map[x][y]].b); } return temp; } bool check(int x, int y){ if(x >= 0 && x < 3 && y < 3 && y >= 0) return true; else return false; } int IDA(int x, int y, int p, int cur){ int r = INF; int temp; int h = get_h(); if(cur + h > Limit) return cur + h; if(h == 0){ ok = true; return cur; } for(int i = 0; i < 4; i ++){ if(i == p) continue; int tx = x + di[i][0]; int ty = y + di[i][1]; if(!check(tx, ty)) continue; swap(Map[x][y], Map[tx][ty]); ans[cur] = i; int lr = IDA(tx, ty, 3 - i, cur + 1); if(ok) return lr; r = min(r, lr); swap(Map[x][y], Map[tx][ty]); } return r; } int main(void){ int N = 1; char ch; cin >> T; getchar(); while(T --){ for(int i = 0; i < 9; i ++){ ch = getchar(); if(ch == 'X' || ch == 'x'){ nowx = i / 3; nowy = i % 3; Map[nowx][nowy] = 0; } if(ch <= '9' && ch >= '0') Map[i / 3][i % 3] = ch - '0'; } getchar(); for(int i = 0; i < 9; i ++){ ch = getchar(); if(ch == 'X' || ch == 'x') continue; else if(ch <= '9' && ch >= '0') f[ch - '0'] = Node(i / 3, i % 3); } getchar(); Limit = get_h(); ok = false; while(!ok) Limit = IDA(nowx, nowy, -10, 0); printf("Case %d: %d ",N++,Limit); for(int i = 0; i < Limit; i ++) putchar(dir[ans[i]]); puts(""); } return 0; }