Description
Astronomers often examine star maps where stars are represented by points on a plane and each star has Cartesian coordinates. Let the level of a star be an amount of the stars that are not higher and not to the right of the given star. Astronomers want to know
the distribution of the levels of the stars.
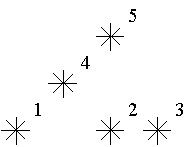
For example, look at the map shown on the figure above. Level of the star number 5 is equal to 3 (it's formed by three stars with a numbers 1, 2 and 4). And the levels of the stars numbered by 2 and 4 are 1. At this map there are only one star of the level 0, two stars of the level 1, one star of the level 2, and one star of the level 3.
You are to write a program that will count the amounts of the stars of each level on a given map.
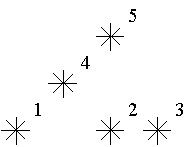
For example, look at the map shown on the figure above. Level of the star number 5 is equal to 3 (it's formed by three stars with a numbers 1, 2 and 4). And the levels of the stars numbered by 2 and 4 are 1. At this map there are only one star of the level 0, two stars of the level 1, one star of the level 2, and one star of the level 3.
You are to write a program that will count the amounts of the stars of each level on a given map.
Input
The first line of the input file contains a number of stars N (1<=N<=15000). The following N lines describe coordinates of stars (two integers X and Y per line separated by a space, 0<=X,Y<=32000). There can be only one star at one point of the plane. Stars
are listed in ascending order of Y coordinate. Stars with equal Y coordinates are listed in ascending order of X coordinate.
Output
The output should contain N lines, one number per line. The first line contains amount of stars of the level 0, the second does amount of stars of the level 1 and so on, the last line contains amount of stars of the level N-1.
Sample Input
5 1 1 5 1 7 1 3 3 5 5
Sample Output
1 2 1 1 0
Hint
This problem has huge input data,use scanf() instead of cin to read data to avoid time limit exceed.
Source
题意:
给你n个点的坐标,统计小于每个点的x、y坐标的点有几个。
思路:让我想到了求逆序数的题目。
按照y轴进行排序,每次将x坐标代表的点插入到树状数组中,然后查询总和。
还有就是坐标从0开始的,0&-0会超时,所以所有坐标要加上1。
#include <cstdio> #include <cmath> #include <vector> #include <iostream> #include <cstring> #include <algorithm> #define space " " #define LOCAL using namespace std; //typedef __int64 Int; //typedef long long Long; const int INF = 0x3f3f3f3f; const int MAXN = 32000 + 10; int bit[MAXN], ar[MAXN]; struct node{ int x, y, lev; }data[MAXN]; int n, maxx; void add(int x, int y) { while (x <= maxx) { bit[x] += y; x += x&-x; } } int sum(int x) { int s = 0; while (x >= 1) { s += bit[x]; x -= x&-x; } return s; } bool cmp(node a, node b) { if (a.y == b.y) return a.x < b.x; return a.y < b.y; } int main() { while (scanf("%d", &n) != EOF) { maxx = 0; memset(bit, 0, sizeof(bit)); for (int i = 1; i <= n; i++) { scanf("%d%d", &data[i].x, &data[i].y); data[i].x++; maxx = max(maxx, data[i].x); } sort(data + 1, data + 1 + n, cmp); for (int i = 1; i <= n; i++) { data[i].lev = sum(data[i].x); add(data[i].x, 1); } memset(ar, 0, sizeof(ar)); for (int i = 1; i <= n; i++) { ar[data[i].lev]++; } for (int i = 0; i < n; i++) { printf("%d ", ar[i]); } } return 0; }