There are n
bulbs that are initially off. You first turn on all the bulbs, then you turn off every second bulb.
On the third round, you toggle every third bulb (turning on if it's off or turning off if it's on). For the ith
round, you toggle every i
bulb. For the nth
round, you only toggle the last bulb.
Return the number of bulbs that are on after n
rounds.
Example 1:
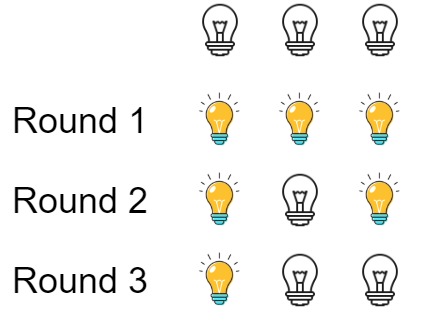
Input: n = 3 Output: 1 Explanation: At first, the three bulbs are [off, off, off]. After the first round, the three bulbs are [on, on, on]. After the second round, the three bulbs are [on, off, on]. After the third round, the three bulbs are [on, off, off]. So you should return 1 because there is only one bulb is on.
Example 2:
Input: n = 0 Output: 0
Example 3:
Input: n = 1 Output: 1
Constraints:
0 <= n <= 109
初始时有 n 个灯泡处于关闭状态。
对某个灯泡切换开关意味着:如果灯泡状态为关闭,那该灯泡就会被开启;而灯泡状态为开启,那该灯泡就会被关闭。
第 1 轮,每个灯泡切换一次开关。即,打开所有的灯泡。
第 2 轮,每两个灯泡切换一次开关。 即,每两个灯泡关闭一个。
第 3 轮,每三个灯泡切换一次开关。
第 i 轮,每 i 个灯泡切换一次开关。 而第 n 轮,你只切换最后一个灯泡的开关。
找出 n 轮后有多少个亮着的灯泡。
来源:力扣(LeetCode)
链接:https://leetcode-cn.com/problems/bulb-switcher
著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。
这是一道数学题。最后的代码很简单,但是理解思路是如何产生的非常重要。这个中文翻译其实是有一点点歧义,从第二轮开始,切换开关的策略如果真的像题目中所说的每几个灯泡里面选一个切换,那么会看不出规律。所以为了看出规律,我们在第 i 轮选择第 i 个灯泡切换开关。这样我们发现,除了第一轮全部打开之外,每一轮会被碰到的灯泡的 index(从1开始)都是当前轮数 i 的质因数。也就是说如果一个灯泡会被切换,那么他的 index 一定可以被当前的轮数 i 整除。除了这一点,我们还需要找到底有哪些 index 下的灯泡是被切换了奇数次的,因为被切换了偶数次的,实际到最后还是亮着的。哪些 index 下的灯泡是被切换了奇数次的呢?数字本身是完全平方数的数字。所以我们只要计算到底有多少个小于轮数 i 的完全平方数即可。
对于 1 - n 中的可以开平方的数字,例如36,它里面可以开平方的数字1*1,2*2...6*6,对于100,它里面可以开平方的数字,1*1,...10*10,可以发现,对于一个数,它里面可以开平方的数字的个数就是它自己的开平方数。
这里有一个小细节,比如如果是12轮吧,12的质因数有1,12,2,6,3,4,一共有六个。但是注意如果轮数本身是一个完全平方数的话,比如16的话,他的质因数就只有1,2,4,8,16,只有奇数个质因数。所以最后亮着的灯泡数量就是轮数的完全平方数。
时间O(1)
空间O(1)
Java实现
1 class Solution { 2 public int bulbSwitch(int n) { 3 return (int) Math.sqrt(n); 4 } 5 }