结构型模式
核心作用:是从程序的结构上实现松耦合,从而可以扩大整体的 类结构,用来解决更大的问题
适配器模式adapter
实际生活中的例子:转换器
适配器的两种方式:
1,类适配器(继承)
/**需要适配的对象
* @author 小帆敲代码
*
*/
public class Adaptee {
public void request() {
System.out.println("can do something");
}
}
/**
*适配器(类适配器)
* @author 小帆敲代码
*
*/
public class Adapter extends Adaptee implements Target {
*适配器(类适配器)
* @author 小帆敲代码
*
*/
public class Adapter extends Adaptee implements Target {
@Override
public void handleReq() {
super.request();
}
public void handleReq() {
super.request();
}
}
/**
* 客户端
* @author 小帆敲代码
*
*/
public class Client {
public void test(Target t) {
t.handleReq();
}
public static void main(String[] args) {
Client c=new Client();
Target t=new Adapter();
c.test(t);
}
}
* 客户端
* @author 小帆敲代码
*
*/
public class Client {
public void test(Target t) {
t.handleReq();
}
public static void main(String[] args) {
Client c=new Client();
Target t=new Adapter();
c.test(t);
}
}
public interface Target {
public void handleReq();
public void handleReq();
}
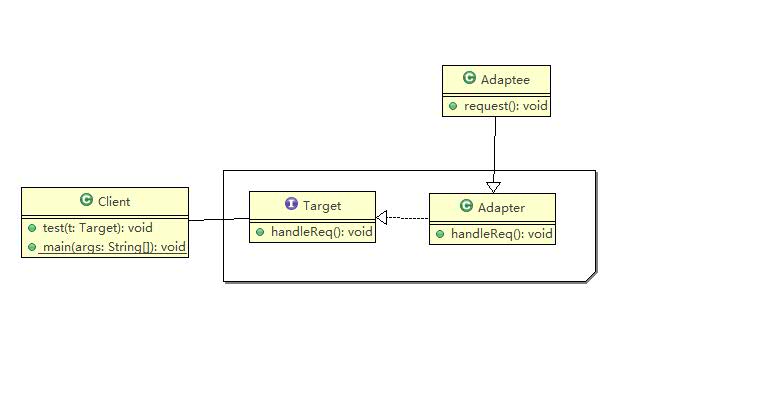
2,对象适配器(组合)
/**需要适配的对象
* @author 小帆敲代码
*
*/
public class Adaptee {
public void request() {
System.out.println("can do something");
}
}
* @author 小帆敲代码
*
*/
public class Adaptee {
public void request() {
System.out.println("can do something");
}
}
/**
*适配器(对象适配器)
* @author 小帆敲代码
*
*/
public class Adapter2 extends Adaptee implements Target {
private Adaptee a;
@Override
public void handleReq() {
a.request();
}
public Adapter2() {
}
public Adapter2(Adaptee a) {
this.a=a;
}
}
*适配器(对象适配器)
* @author 小帆敲代码
*
*/
public class Adapter2 extends Adaptee implements Target {
private Adaptee a;
@Override
public void handleReq() {
a.request();
}
public Adapter2() {
}
public Adapter2(Adaptee a) {
this.a=a;
}
}
public class Client2 {
public void test(Target t) {
t.handleReq();
}
public static void main(String[] args) {
Client c=new Client();
Adaptee a=new Adaptee();
Target t=new Adapter2(a);
c.test(t);
}
}
public void test(Target t) {
t.handleReq();
}
public static void main(String[] args) {
Client c=new Client();
Adaptee a=new Adaptee();
Target t=new Adapter2(a);
c.test(t);
}
}
public interface Target {
public void handleReq();
public void handleReq();
}
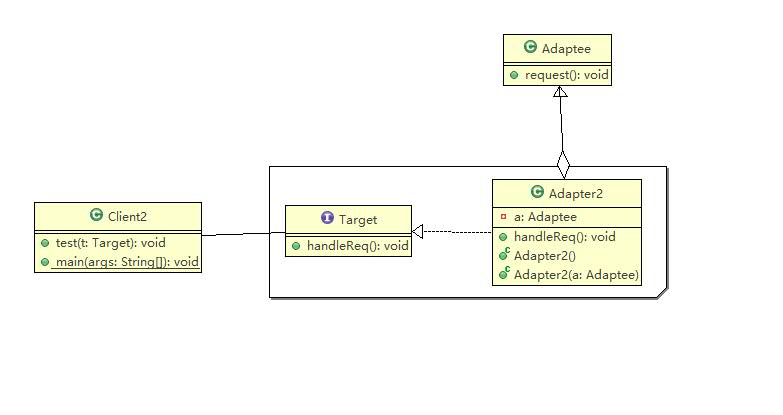
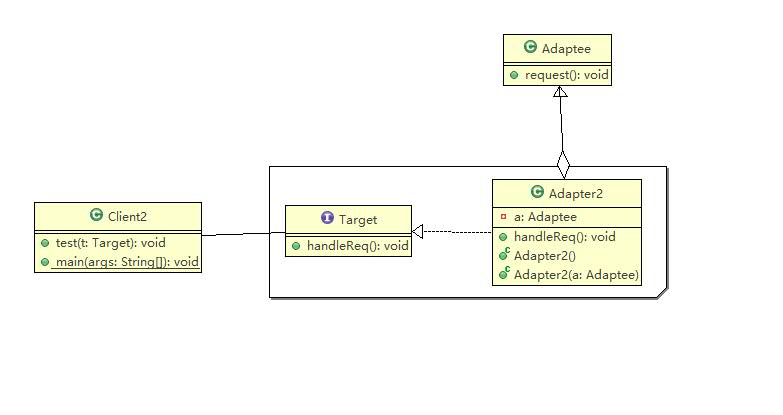